The define()
keyword is a fundamental concept in JavaScript programming. It is used to define a new module, which can be imported and used in other parts of the program. The define()
keyword is part of the Asynchronous Module Definition (AMD) API, which is used to load modules asynchronously in the browser.

When a module is defined using the define()
keyword, it is given a name and a list of dependencies. The dependencies are other modules that this module requires in order to function correctly. When the module is loaded, the dependencies are loaded first, and then the module itself is executed. This ensures that all the required dependencies are available before the module is used.
The define()
keyword is an essential part of modern JavaScript programming, especially in the context of web development. It allows developers to break their code into smaller, more manageable modules, which can be loaded and executed independently. This makes it easier to maintain and test code, and also improves performance by reducing the amount of code that needs to be loaded and executed at once.
Understanding Define() in JavaScript
Purpose of Define()
In JavaScript, define()
is a keyword used to define a new module. It is a part of the AMD (Asynchronous Module Definition) API, which allows developers to write modular code for the browser. The main purpose of define()
is to define a module that can be loaded asynchronously, which means that it can be loaded when it is needed, rather than being loaded all at once.
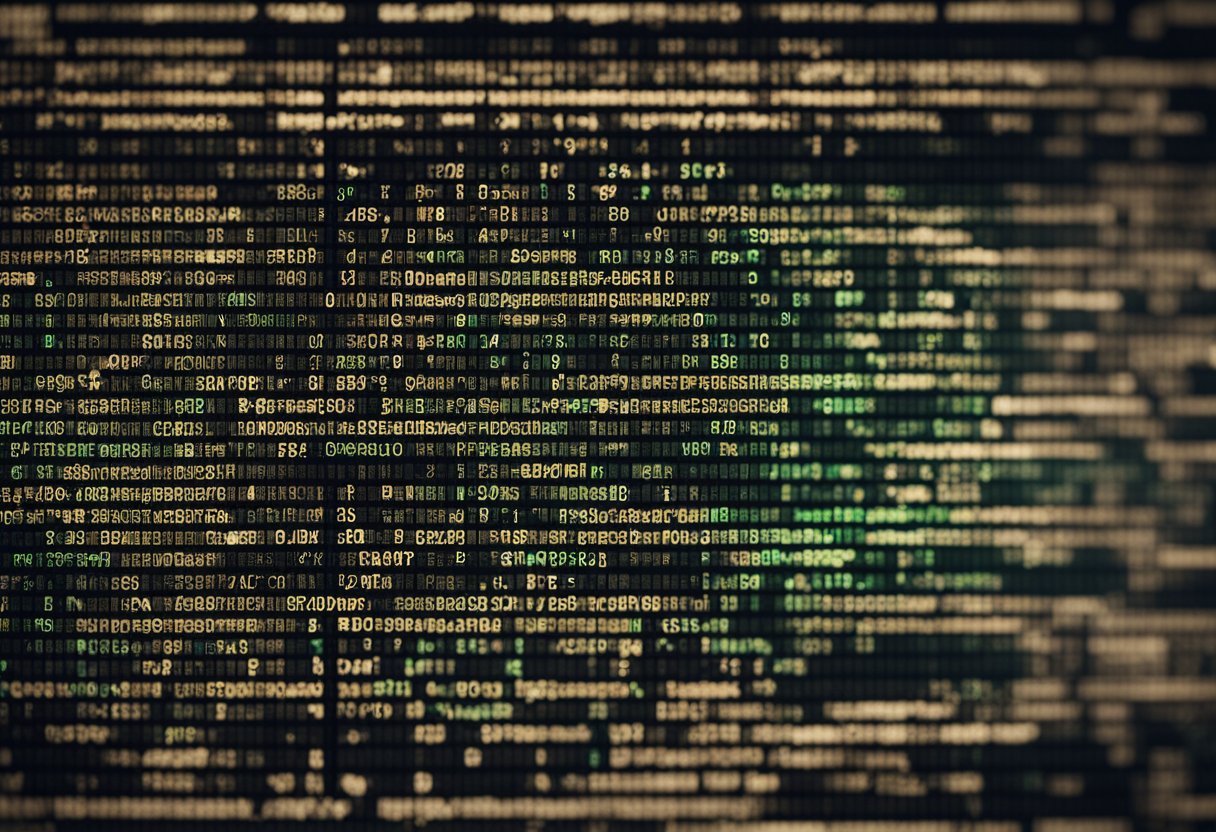
When defining a module using define()
, developers can specify its dependencies and the function that will be executed when the module is loaded. The function can return an object, a function, or a value, which can be used by other modules that depend on it.
Scope of Define()
The scope of define()
is limited to the module that is being defined. It does not affect any other part of the program. When a module is defined using define()
, it is given a unique identifier, which can be used to load the module asynchronously.
Developers can use define()
to define a module that depends on other modules, and they can specify the dependencies using an array of strings. The strings represent the names of the modules that the current module depends on. When the module is loaded, the dependencies are loaded first, and then the module is executed.
Syntax and Parameters
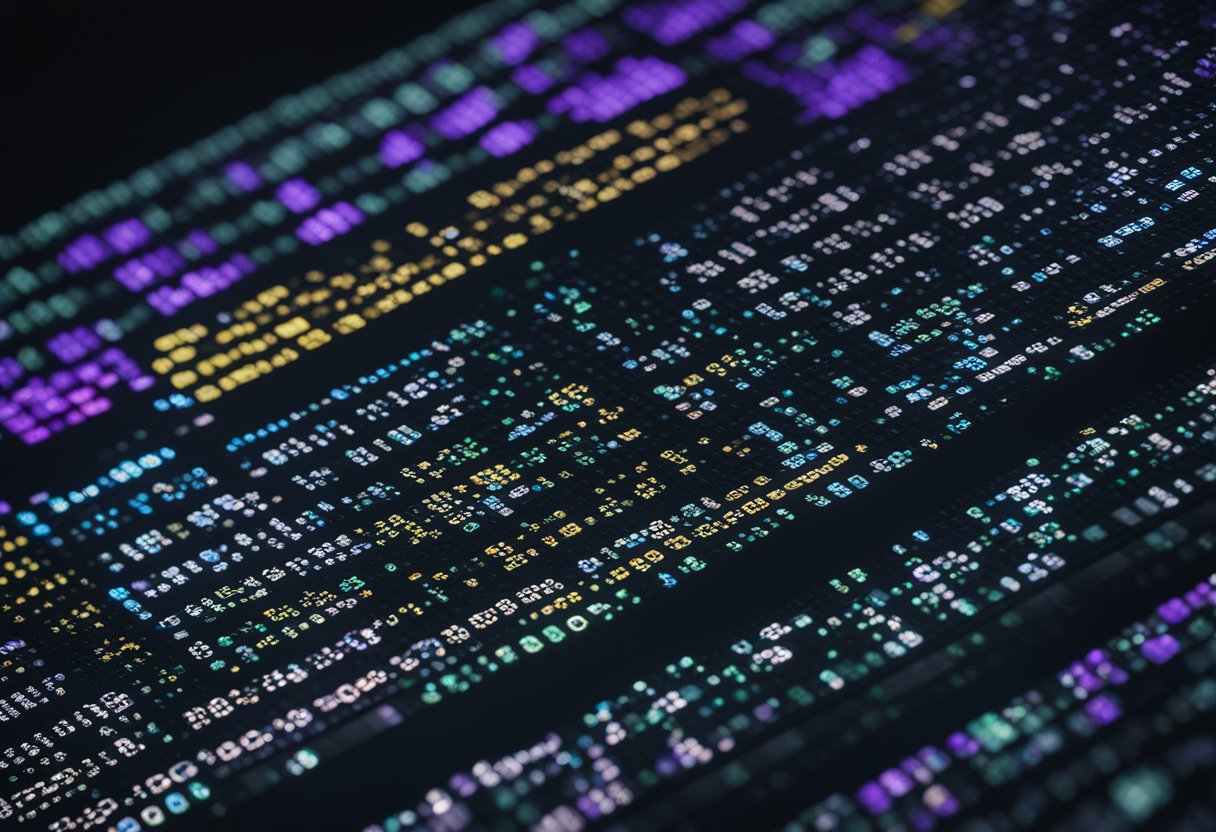
Syntax Overview
define()
is a built-in function in JavaScript that is used to define a new module. The syntax for define()
is as follows:
define(moduleName, dependencies, moduleDefinition);
Here, moduleName
is a string that represents the name of the module being defined. dependencies
is an array of strings that represents the modules that the current module depends on. moduleDefinition
is a function that defines the module.
Parameter Types
The define()
function takes three parameters, all of which are required. The first parameter, moduleName
, is a string that represents the name of the module being defined. This parameter is required and must be a non-empty string.
The second parameter, dependencies
, is an array of strings that represents the modules that the current module depends on. This parameter is optional and can be an empty array if the module does not depend on any other modules.
The third parameter, moduleDefinition
, is a function that defines the module. This parameter is required and must be a function that returns the module. The function can take any number of parameters, but the first parameter is usually reserved for the dependencies of the module.
Return Values
The define()
function returns nothing. Instead, it defines a new module that can be used by other modules in the application.
Default Parameters in JavaScript: A Comprehensive Guide
Implementing Define()
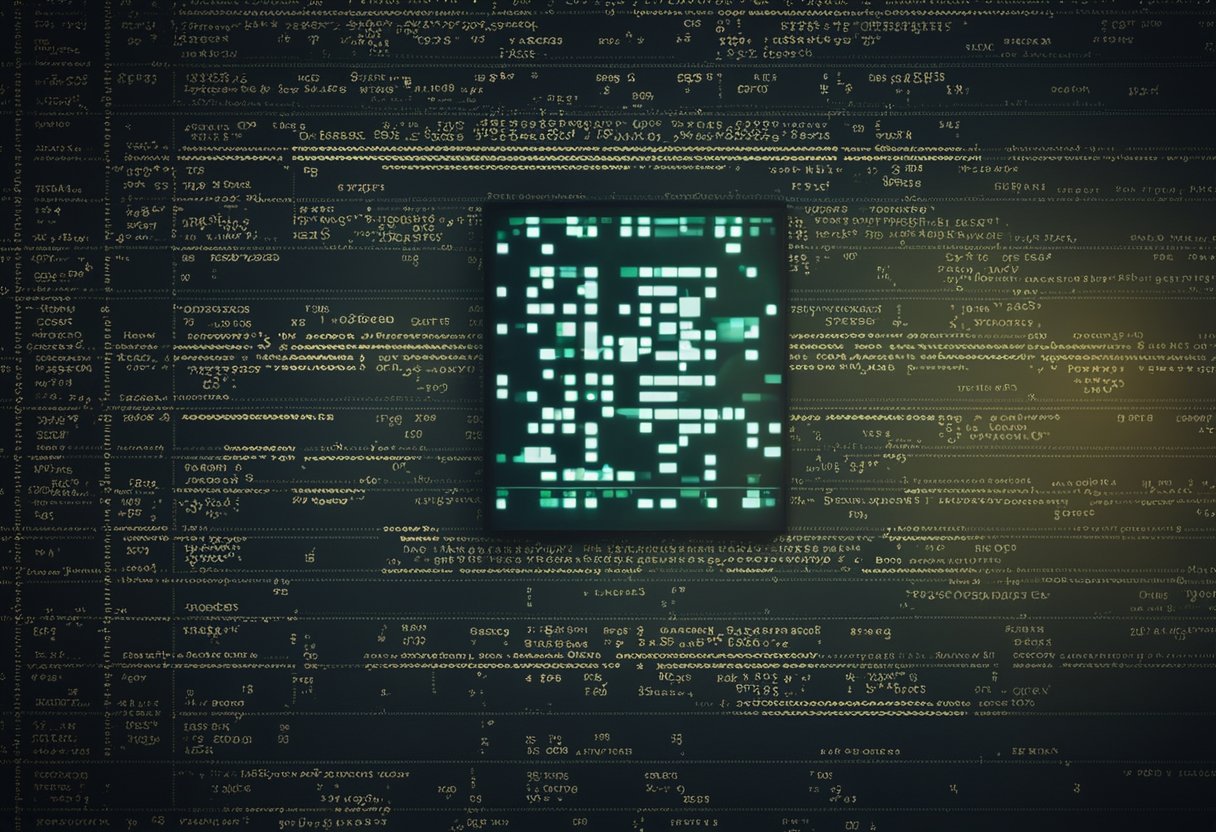
Basic Implementation
In JavaScript, the define()
keyword is used to define a module. A module is a self-contained piece of code that can be reused in different parts of a program. The define()
keyword takes two arguments: the name of the module and an array of dependencies.
Here is a basic example of how to use the define()
keyword:
define('myModule', [], function() {
// code for myModule
});
In this example, we are defining a module called myModule
with no dependencies. The third argument is a function that contains the code for the module.
Advanced Usage
The define()
keyword can also be used to define modules with dependencies. Here is an example:
define('myModule', ['dependency1', 'dependency2'], function(dependency1, dependency2) {
// code for myModule
});
In this example, we are defining a module called myModule
that depends on two other modules: dependency1
and dependency2
. The third argument is a function that takes two arguments, which are the dependencies.
The define()
keyword can also be used to define modules that export values. Here is an example:
define('myModule', [], function() {
var myValue = 'Hello, world!';
return myValue;
});
In this example, we are defining a module called myModule
that exports a value. The third argument is a function that returns the value.
Common Pitfalls and Best Practices
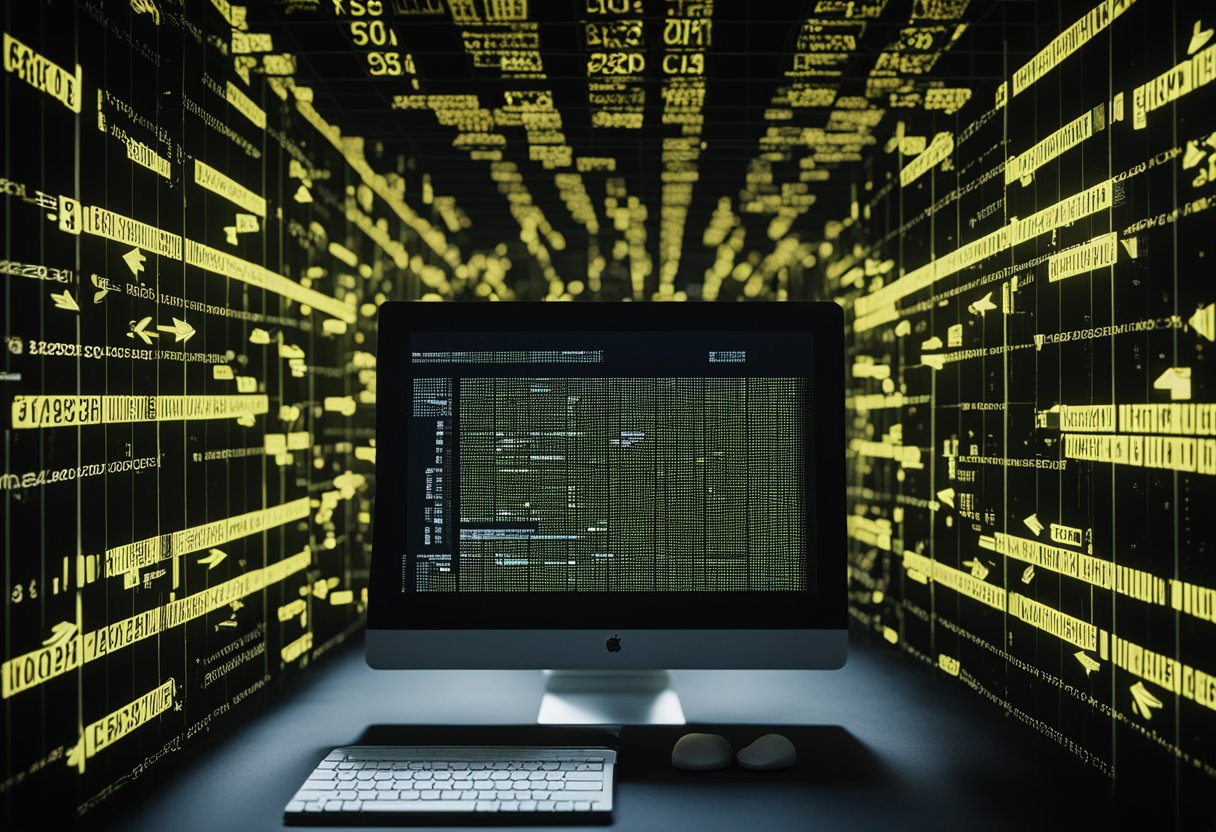
Common Errors
When using the define()
keyword in JavaScript, there are several common errors that developers may encounter. These include:
- Forgetting to include dependencies: When defining a module, it’s important to include all of its dependencies. Failing to do so can result in errors and unexpected behavior.
- Not using a callback function: When defining a module, it’s important to use a callback function to ensure that the module is properly loaded.
- Naming conflicts: When defining modules, it’s important to choose unique names to avoid naming conflicts. This can be particularly challenging when working with large codebases.
- Not using strict mode: When defining modules, it’s important to use strict mode to catch errors and avoid unexpected behavior.
Best Practices
To avoid these common errors and ensure that define()
is used correctly, there are several best practices that developers can follow:
- Use a consistent naming convention: When defining modules, use a consistent naming convention to avoid naming conflicts and make it easier to find and reference modules.
- Use a build tool: Use a build tool like Grunt or Gulp to automate the process of defining and loading modules. This can help to reduce errors and improve performance.
- Use strict mode: Always use strict mode when defining modules to catch errors and avoid unexpected behavior.
- Include dependencies: When defining modules, include all of its dependencies to ensure that the module is properly loaded and avoid errors.
- Use a callback function: Always use a callback function when defining modules to ensure that the module is properly loaded.
By following these best practices, developers can ensure that they are using the define()
keyword correctly and avoid common errors.
Real-World Applications
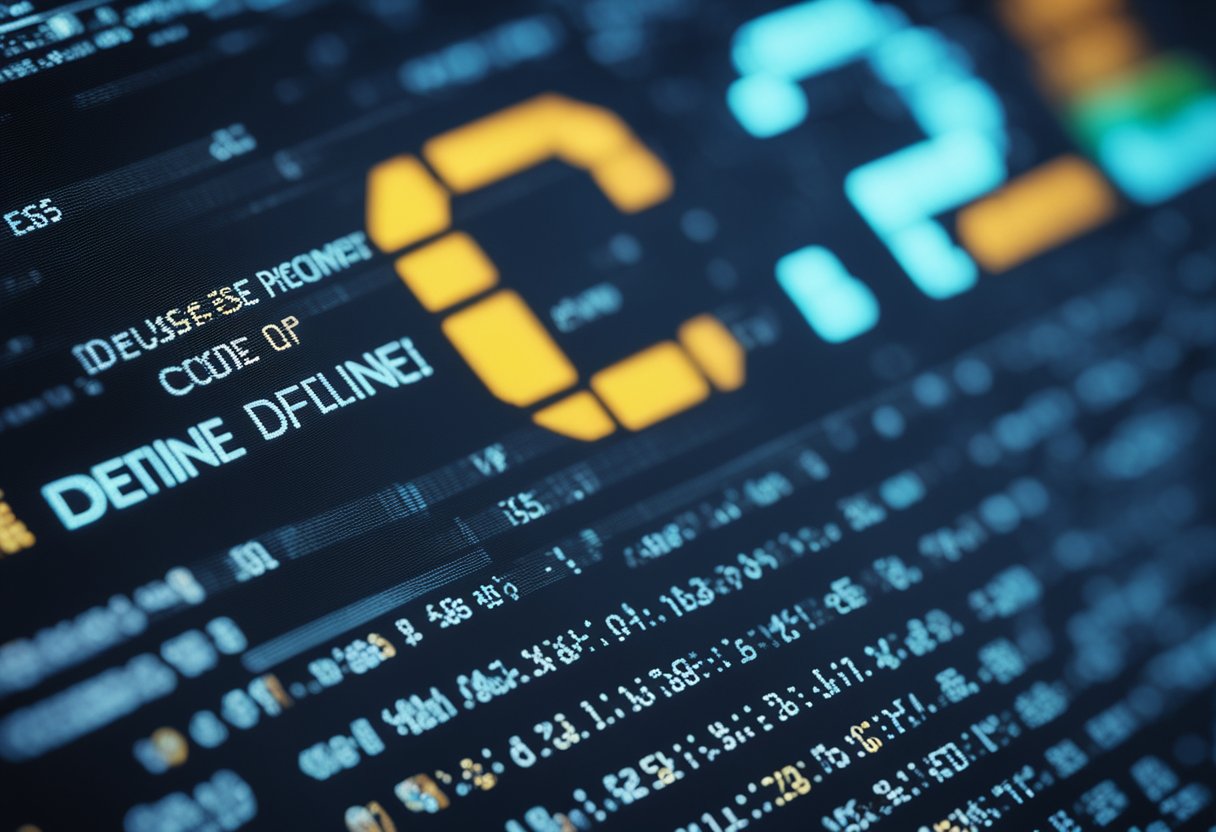
JavaScript’s define()
keyword is a powerful tool that can be used to create modular and reusable code. While the define()
keyword is often used in large-scale web applications, it can be useful for smaller projects as well.
Use Cases
One of the most common use cases for the define()
keyword is to create reusable modules. By defining a module with define()
, developers can create a self-contained unit of code that can be easily reused across different parts of an application. This can help to reduce code duplication and make the codebase easier to maintain.
Another use case for the define()
keyword is to create plugins for popular JavaScript libraries such as jQuery. By defining a plugin with define()
, developers can create a modular and reusable piece of code that can be easily integrated into an existing application.
Case Studies
There are many examples of the define()
keyword being used in real-world applications. One such example is the popular JavaScript library, RequireJS. RequireJS uses the define()
keyword to define modules that can be loaded asynchronously, which can help to improve the performance of web applications.
Another example of the define()
keyword being used in a real-world application is the Adobe Experience Manager (AEM) content management system. AEM uses the define()
keyword to define modules that can be easily reused across different parts of the application. This helps to reduce code duplication and make the codebase easier to maintain.
In conclusion, the define()
keyword is a powerful tool that can be used to create modular and reusable code in JavaScript. It is commonly used in large-scale web applications and can be useful for smaller projects as well. By defining modules with define()
, developers can create self-contained units of code that can be easily reused across different parts of an application.
Frequently Asked Questions
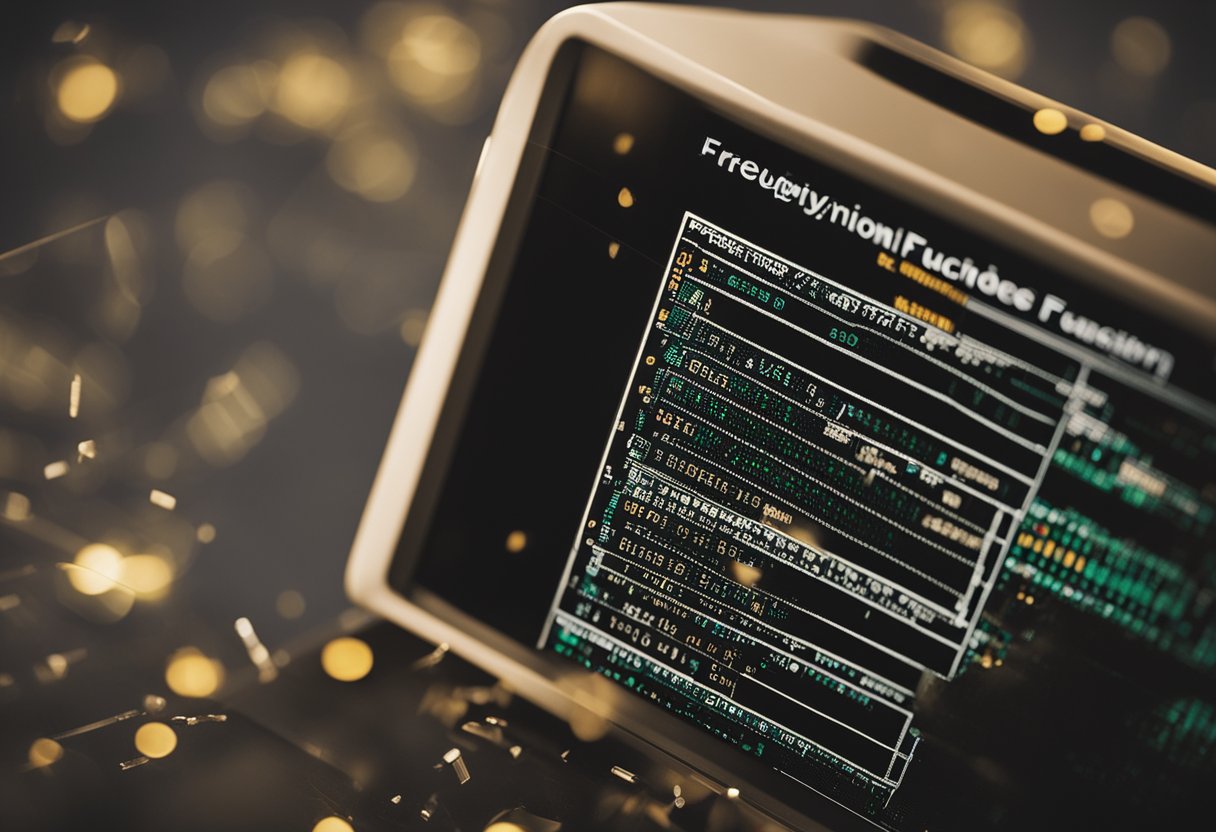
How do you define a function in JavaScript?
To define a function in JavaScript, you can use the function
keyword followed by the name of the function and its parameters in parentheses. The body of the function is enclosed in curly braces {}
. For example, to define a function that adds two numbers, you can write:
function addNumbers(a, b) {
return a + b;
}
What is the purpose of the define() method in RequireJS?
The define()
method in RequireJS is used to define a module. It takes a module name and an array of dependencies as arguments, followed by a factory function that returns the module. The purpose of this method is to allow for modular development in JavaScript, where each module can be loaded as needed, improving performance and maintainability.
How can you define an array in JavaScript?
To define an array in JavaScript, you can use square brackets []
and separate the elements with commas. For example, to define an array of numbers, you can write:
let numbers = [1, 2, 3, 4, 5];
What does customElements.define() do in JavaScript web components?
The customElements.define()
method in JavaScript web components is used to define a new custom element. It takes two arguments: the name of the custom element and an object that defines the behavior of the element. This method allows developers to create their own HTML elements with custom functionality.
In JavaScript, what is the ‘this’ keyword and how is it used?
In JavaScript, the this
keyword refers to the current object. It is used to access properties and methods of the object. The value of this
depends on how the function is called. For example, when a function is called as a method of an object, this
refers to the object. When a function is called with the new
keyword, this
refers to the newly created object.
How do you create a custom keyword in JavaScript?
In JavaScript, you cannot create custom keywords. Keywords are reserved by the language and have a specific meaning. However, you can create custom functions and variables with names that are not reserved keywords. This allows you to create your own functionality in JavaScript.