JavaScript is a popular programming language used for creating dynamic web pages and web applications. It is a versatile language that allows developers to create complex applications easily. One of the most important features of JavaScript is the ability to control program flow using statements like break and continue. These statements are used to alter the behavior of loops and switch statements.
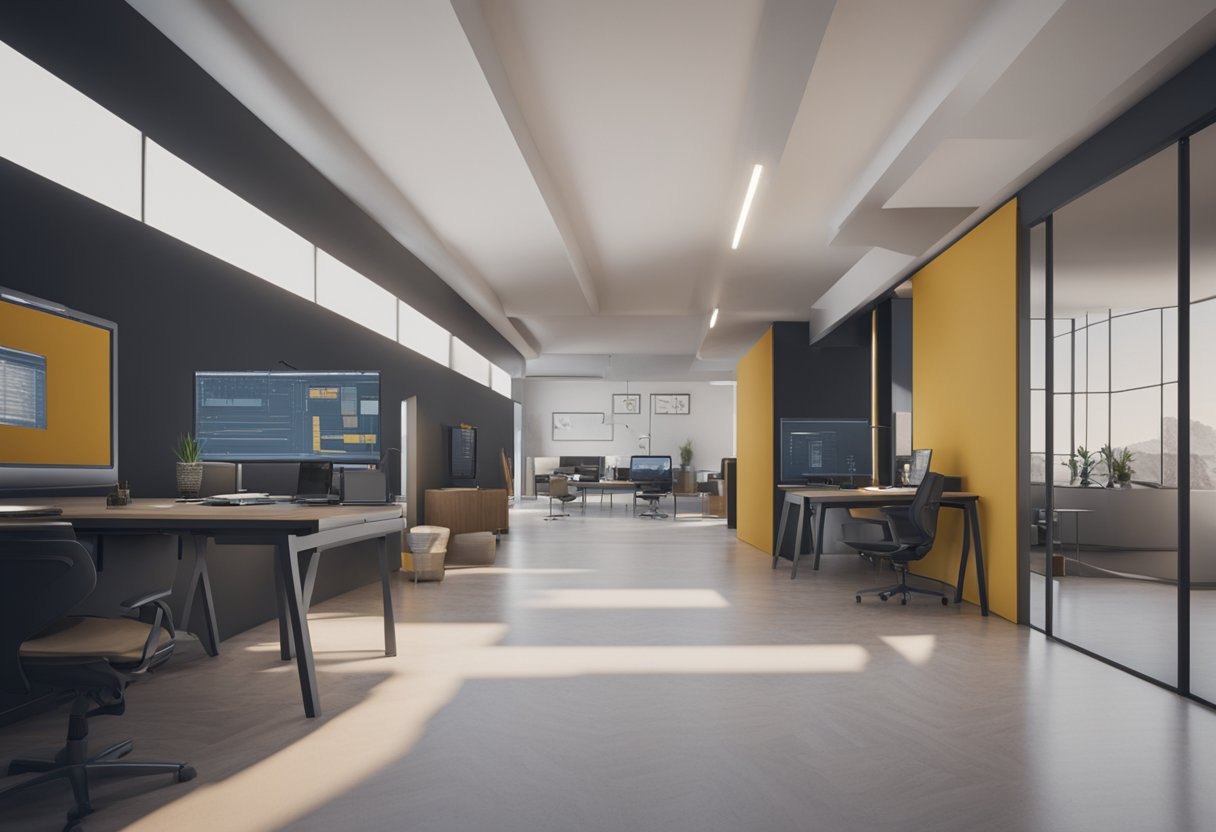
The break statement is used to exit a loop or switch statement. When executed, it terminates the current loop or switch statement and transfers control to the statement following the terminated statement. On the other hand, the continue statement is used to skip the current iteration of a loop and move on to the next iteration. It is useful when you want to skip the execution of a particular iteration based on some condition.
Key Takeaways
- Break and continue statements are essential for controlling program flow in JavaScript.
- The break statement is used to exit a loop or switch statement, while the continue statement is used to skip the current iteration of a loop.
- Best practices for using break and continue include avoiding nested loops, using descriptive labels, and avoiding infinite loops.
Understanding the Break Statement
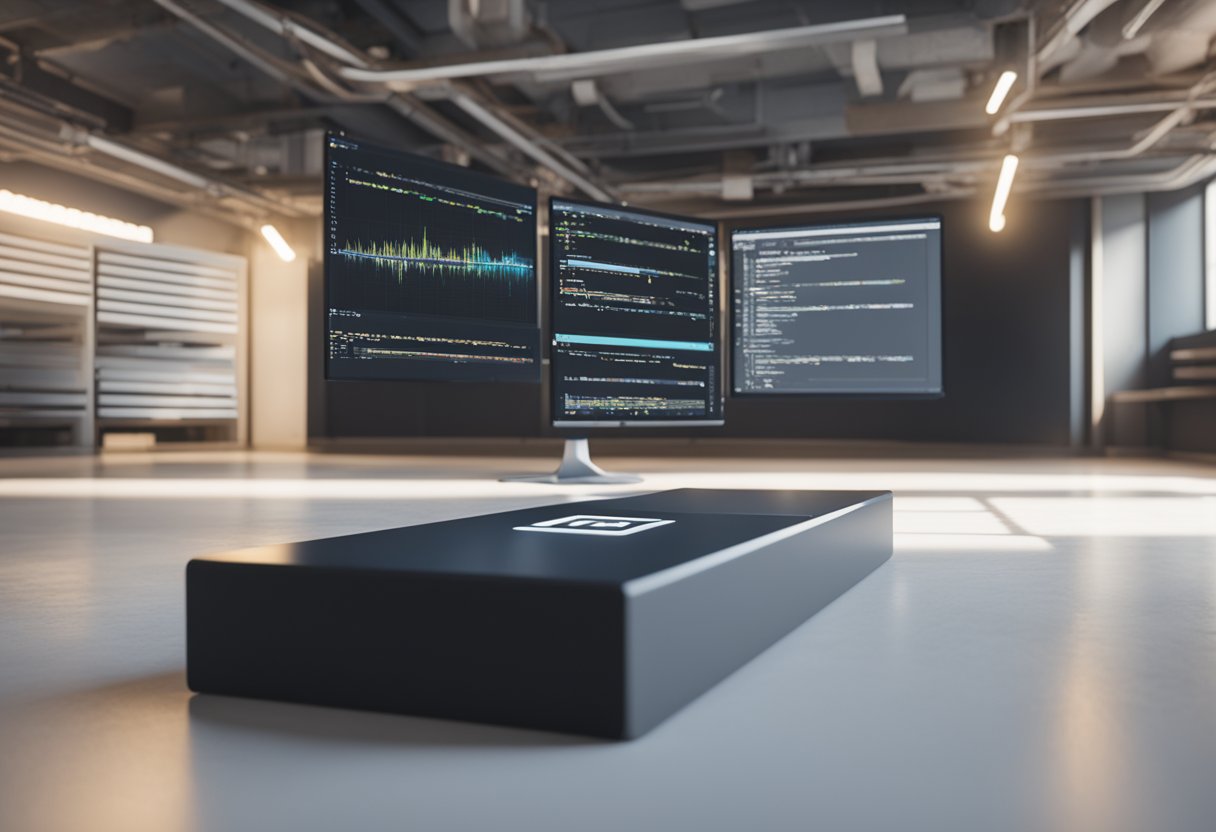
In JavaScript, the break
statement is used to exit a loop. It can be used inside a for
loop, while
loop, or switch
statement. When the break
statement is encountered inside a loop, the loop is immediately terminated, and the program execution continues with the statement following the loop.
The break
statement is often used in combination with an if
statement to exit a loop when a certain condition is met. For example, consider the following code snippet:
for (var i = 0; i < 10; i++) {
if (i === 5) {
break;
}
console.log(i);
}
In this code, the for
loop iterates from 0 to 9. When i
is equal to 5, the break
statement is executed, and the loop is terminated. The output of this code is:
0
1
2
3
4
As you can see, the loop terminates when i
is equal to 5, and the number 5 is not printed to the console.
The break
statement can also be used to exit a switch
statement. When the break
statement is encountered inside a switch
statement, the program execution continues with the statement following the switch
statement. If the break
statement is not used inside a switch
statement, the program execution will “fall through” to the next case statement.
In summary, the break
statement is a powerful tool for controlling the flow of a program. It is used to exit a loop or switch
statement when a certain condition is met. When used correctly, the break
statement can make your code more efficient and easier to read.
Understanding the Continue Statement
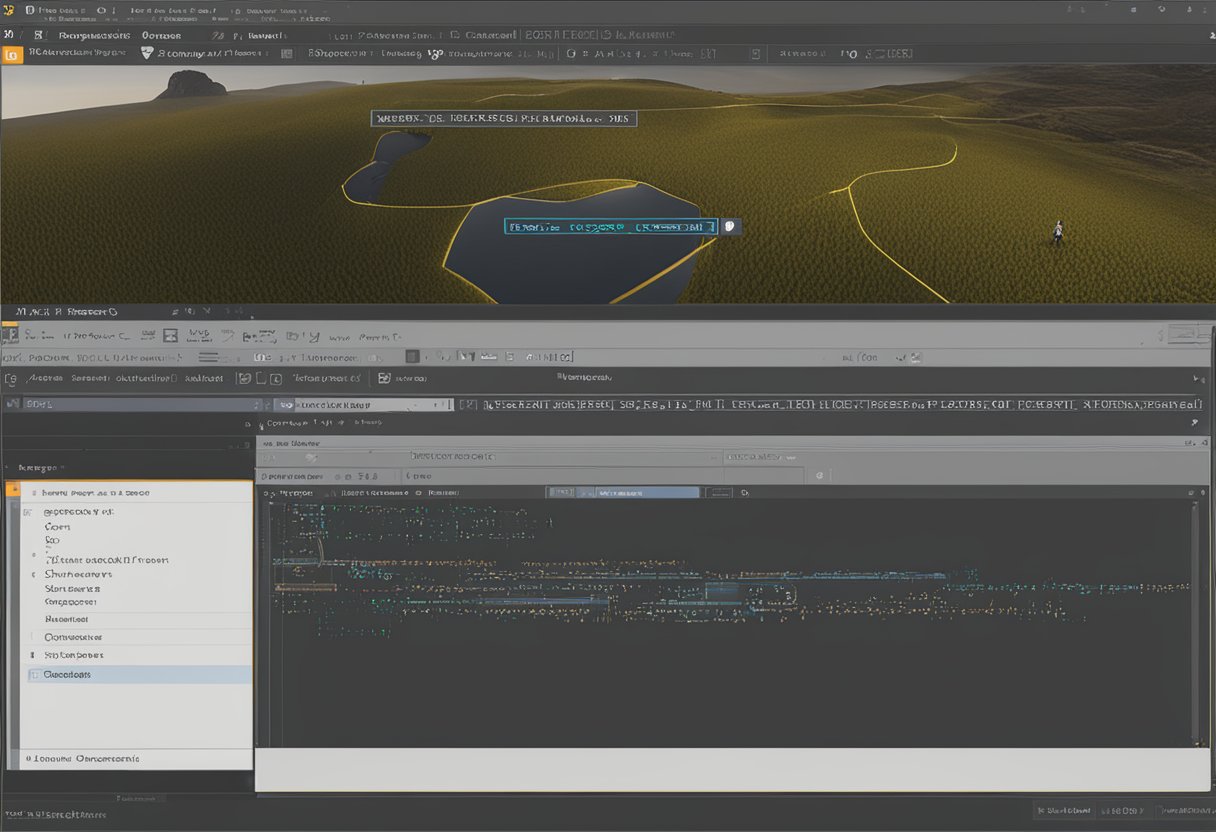
In JavaScript, the continue
statement is used to skip the current iteration of a loop and move on to the next iteration. It is often used in combination with the if
statement to skip certain iterations based on a condition.
The continue
statement can only be used inside a loop, such as for
, while
, or do-while
loops. When the continue
statement is encountered, the remaining statements inside the loop for that iteration are skipped, and the loop starts again with the next iteration.
Here is an example of using the continue
statement inside a for
loop:
for (let i = 0; i < 10; i++) {
if (i === 3) {
continue;
}
console.log(i);
}
In this example, the loop will iterate from 0 to 9. However, when i
is equal to 3, the continue
statement is executed, and the remaining statements inside the loop for that iteration are skipped. Therefore, the number 3 will not be printed to the console.
The continue
statement can be useful when working with arrays or other collections of data. For example, if you want to loop over an array and skip certain elements based on a condition, you can use the continue
statement to skip those elements:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
continue;
}
console.log(numbers[i]);
}
In this example, the loop iterates over the numbers
array and prints only the odd numbers to the console. The continue
statement is used to skip the even numbers and move on to the next iteration.
Overall, the continue
statement is a powerful tool for controlling the flow of a loop in JavaScript. By using continue
, you can skip certain iterations of a loop based on a condition, which can be useful for processing collections of data or performing other complex tasks.
Break Statement Syntax
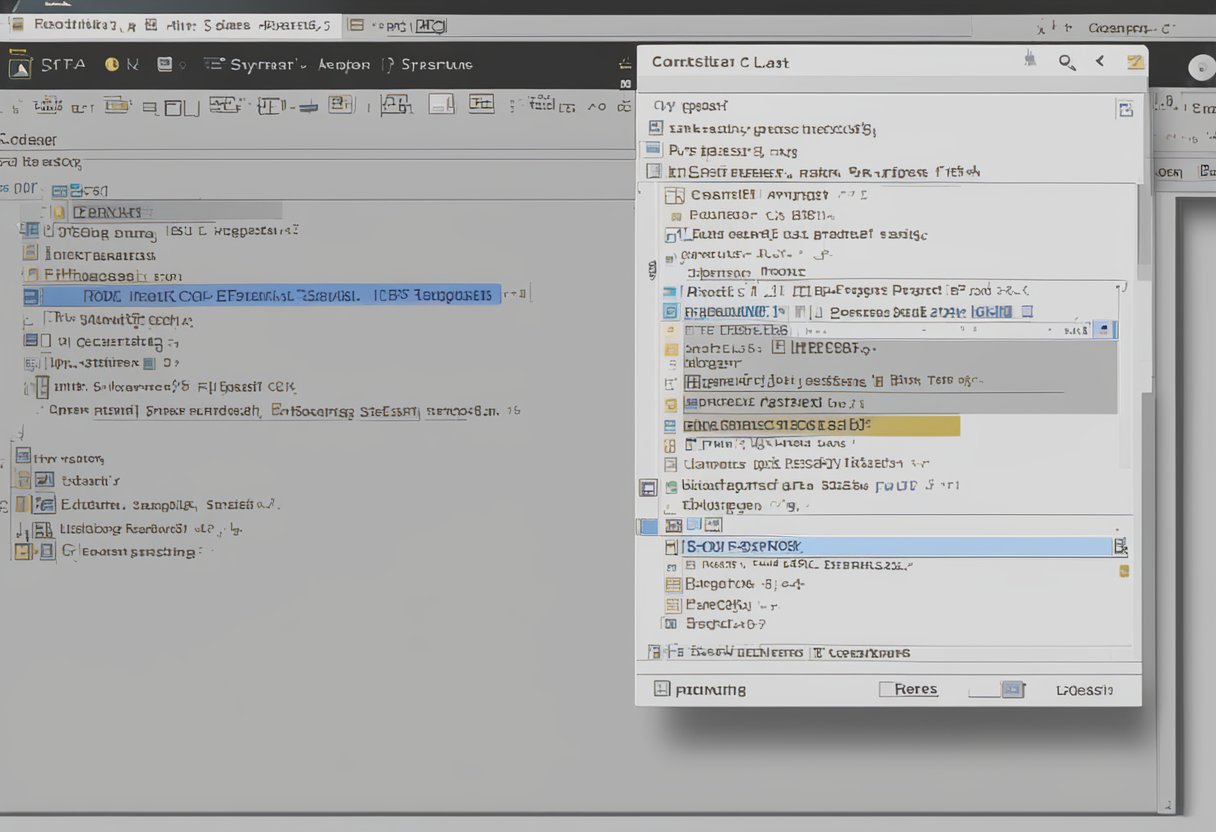
The break
statement is used to terminate a loop or switch statement and transfer control to the statement immediately following the terminated statement. It is commonly used in conjunction with if
statements to exit a loop when a certain condition is met.
The syntax of the break
statement in JavaScript is as follows:
break;
When the break
statement is encountered within a loop, the loop is terminated and program control is transferred to the statement following the loop. If the break
statement is used within a nested loop, only the innermost loop is terminated.
The break
statement can also be used with a label to terminate a statement outside of the current loop. The label must be placed before the statement to be terminated, and the break
statement must include the label name. For example:
outerloop: for (var i = 0; i < 10; i++) {
for (var j = 0; j < 10; j++) {
if (i * j >= 30) {
break outerloop;
}
}
}
This code will terminate both loops when i * j
is greater than or equal to 30.
In summary, the break
statement is a useful tool for controlling program flow in JavaScript. It allows for the termination of loops and switch statements when certain conditions are met, and can be used with labels to terminate statements outside of the current loop.
Continue Statement Syntax
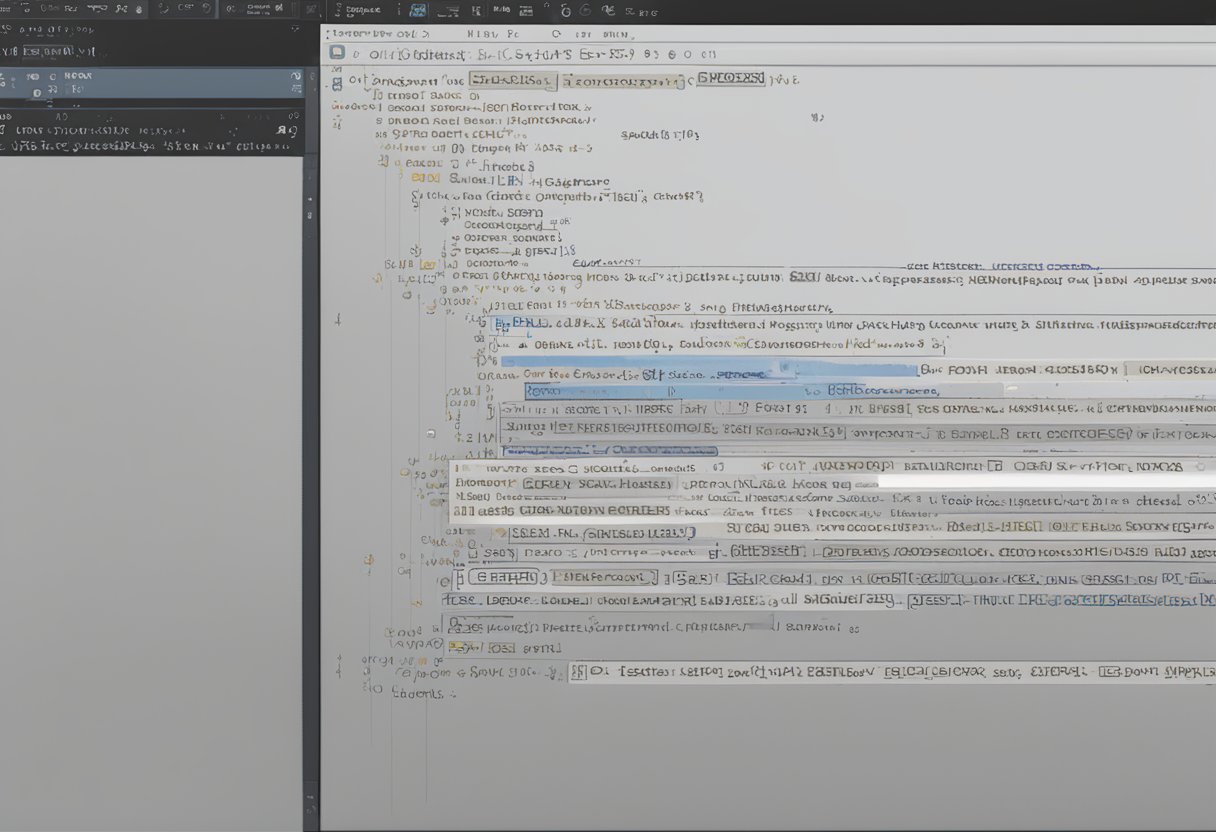
The continue
statement is used in JavaScript to skip the current iteration of a loop and move on to the next iteration. It is often used inside loops to skip certain values or conditions that meet specific criteria.
The syntax for using the continue
statement in a loop is as follows:
for (initialization; condition; increment) {
// code to be executed
if (condition) {
continue;
}
// more code to be executed
}
The continue
statement is placed inside the loop block and is executed when the specified condition is met. It causes the loop to skip the current iteration and move on to the next iteration.
Here is a brief explanation of the different parts of the for
loop syntax:
- Initialization: This is where you set the initial value of the loop variable.
- Condition: This is where you specify the condition that must be true for the loop to continue executing.
- Increment: This is where you specify how the loop variable should be incremented or decremented after each iteration.
When the continue
statement is executed, the loop variable is incremented or decremented based on the specified increment value and the loop continues executing from the top with the next iteration.
It is important to note that the continue
statement only skips the current iteration of the loop and not the entire loop. The loop will continue executing until the specified condition becomes false.
Using Break in Loops
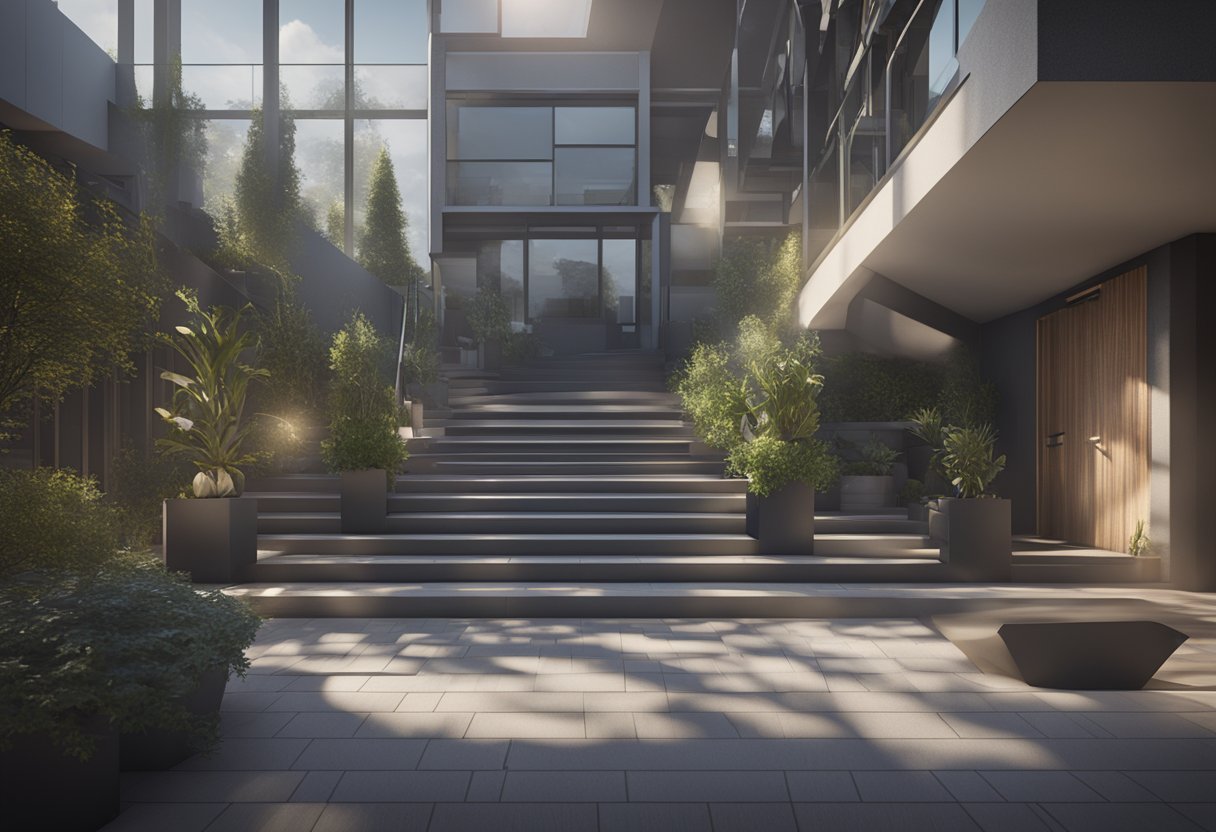
In JavaScript, the break
statement is used to exit a loop prematurely. When a break
statement is executed inside a loop, the loop is immediately terminated, and the program execution continues with the next statement after the loop.
Break in For Loops
In a for
loop, the break
statement is often used to exit the loop early when a certain condition is met. For example, consider the following code:
for (let i = 0; i < 10; i++) {
if (i === 5) {
break;
}
console.log(i);
}
In this code, the loop will terminate when i
is equal to 5, and the program will continue with the next statement after the loop.
Break in While Loops
A while
loop is another type of loop that can be exited early using the break
statement. For example, consider the following code:
let i = 0;
while (i < 10) {
if (i === 5) {
break;
}
console.log(i);
i++;
}
In this code, the loop will terminate when i
is equal to 5, and the program will continue with the next statement after the loop.
Break in Do-While Loops
A do-while
loop is similar to a while
loop, but the loop body is executed at least once before the condition is checked. The break
statement can also be used to exit a do-while
loop prematurely. For example, consider the following code:
let i = 0;
do {
if (i === 5) {
break;
}
console.log(i);
i++;
} while (i < 10);
In this code, the loop will terminate when i
is equal to 5, and the program will continue with the next statement after the loop.
Using the break
statement can be a powerful tool when working with loops in JavaScript. However, it should be used judiciously to avoid creating code that is difficult to read and understand.
Using Continue in Loops
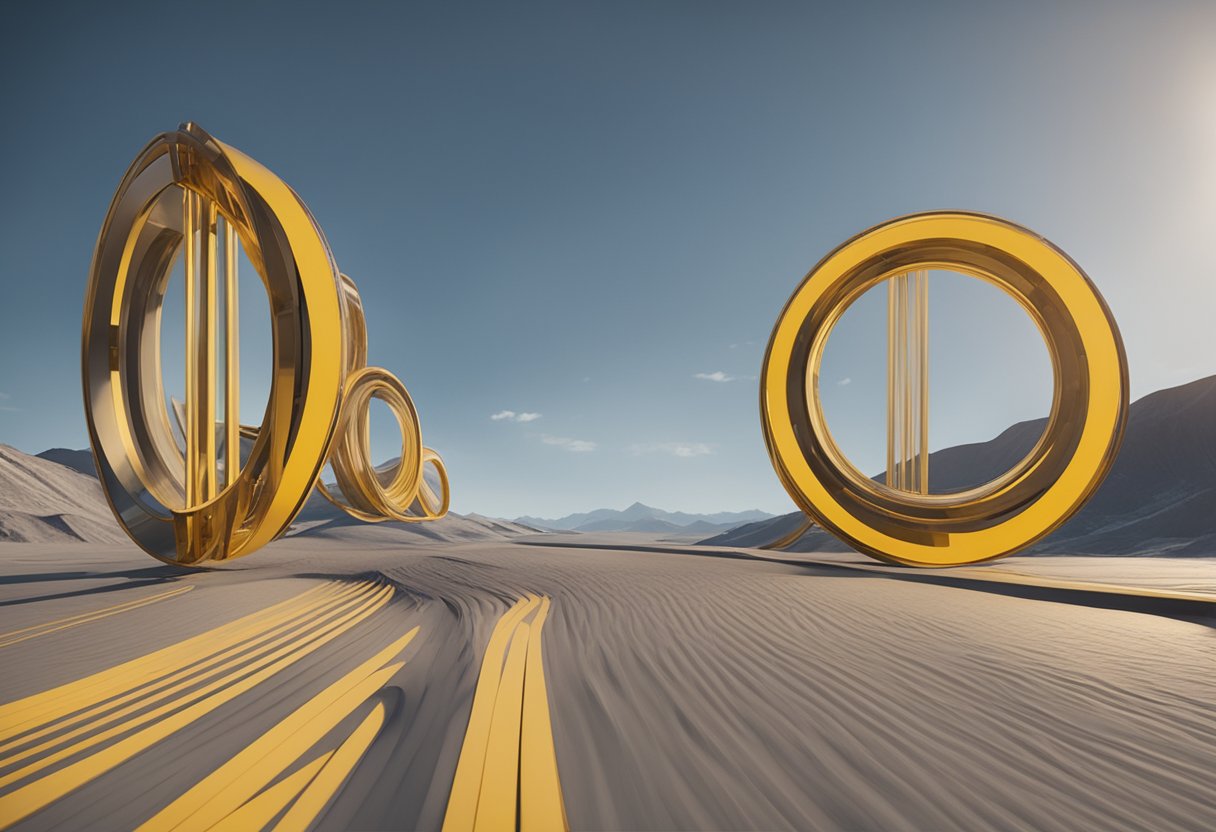
When working with loops in JavaScript, sometimes it is necessary to skip over certain iterations based on a condition. This is where the continue
statement comes in. The continue
statement allows the program to skip to the next iteration of the loop immediately, without executing any further statements in the current iteration.
Continue in For Loops
The continue
statement can be used in a for
loop to skip over certain iterations based on a condition. Here’s an example:
for (let i = 1; i <= 10; i++) {
if (i % 2 === 0) {
continue;
}
console.log(i);
}
In this example, the continue
statement is used to skip over even numbers and print only odd numbers. The output of this code would be:
1
3
5
7
9
Continue in While Loops
The continue
statement can also be used in a while
loop to skip over certain iterations based on a condition. Here’s an example:
let i = 0;
while (i < 10) {
i++;
if (i % 2 === 0) {
continue;
}
console.log(i);
}
In this example, the continue
statement is used to skip over even numbers and print only odd numbers. The output of this code would be the same as the previous example:
1
3
5
7
9
Continue in Do-While Loops
The continue
statement can also be used in a do-while
loop to skip over certain iterations based on a condition. Here’s an example:
let i = 0;
do {
i++;
if (i % 2 === 0) {
continue;
}
console.log(i);
} while (i < 10);
In this example, the continue
statement is used to skip over even numbers and print only odd numbers. The output of this code would be the same as the previous examples:
1
3
5
7
9
Using the continue
statement in loops can help make your code more efficient and concise by skipping over unnecessary iterations.
Break in Switch Statements
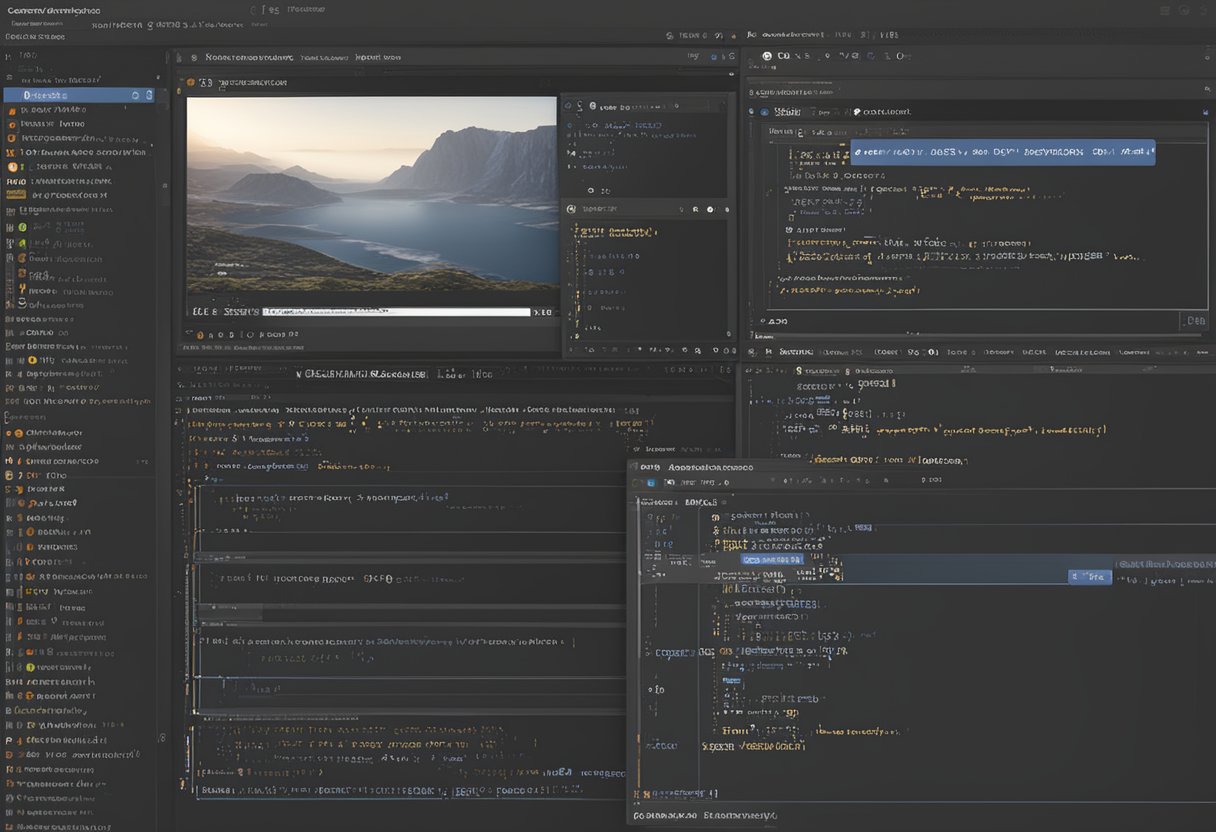
In JavaScript, the switch
statement is used to execute different actions based on different conditions. When a switch
statement is executed, the code block associated with the first matching case
is executed. If no matching case
is found, the default
code block is executed.
The break
statement is used to exit a switch
statement. When a break
statement is encountered, the code execution inside the switch
statement is terminated and the control is transferred to the next statement after the switch
block. If a break
statement is not used, the code execution will continue to the next case
statement until a break
statement is encountered or the default
statement is executed.
Here is an example of how to use break
statement in a switch
statement:
let color = "red";
switch(color) {
case "red":
console.log("The color is red.");
break;
case "green":
console.log("The color is green.");
break;
default:
console.log("The color is neither red nor green.");
}
In this example, if the value of color
is "red"
, the code block associated with the first case
statement is executed and the output will be "The color is red."
. The break
statement is used to exit the switch
statement. If the value of color
is "green"
, the code block associated with the second case
statement is executed and the output will be "The color is green."
. If the value of color
is neither "red"
nor "green"
, the code block associated with the default
statement is executed and the output will be "The color is neither red nor green."
.
It is important to use the break
statement in a switch
statement to avoid executing unnecessary code blocks and to ensure that the correct code block is executed based on the condition.
Loop Control vs. Flow Control
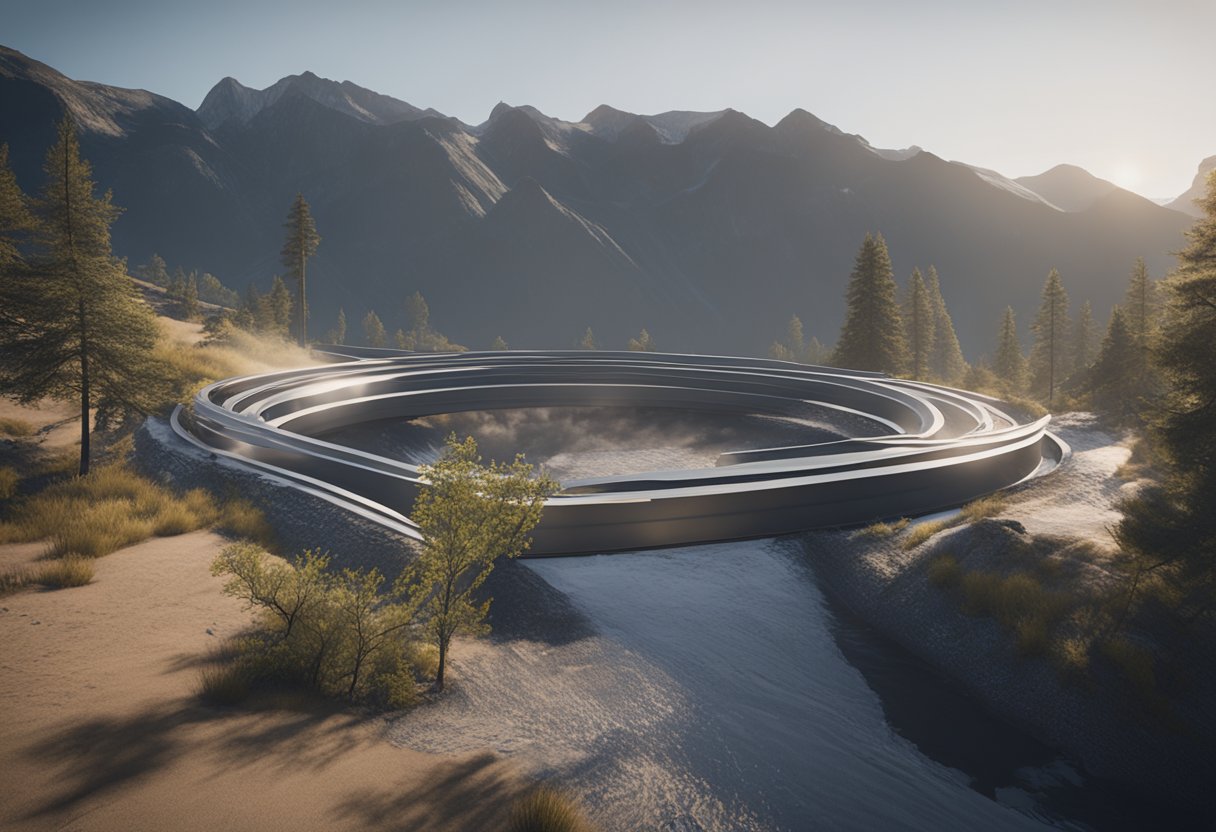
In JavaScript, there are two types of control statements that allow developers to manipulate the flow of code execution: loop control statements and flow control statements.
Loop control statements, such as break
and continue
, are used to control the behavior of loops. When a break
statement is encountered within a loop, the loop is terminated and control is passed to the next statement after the loop. On the other hand, when a continue
statement is encountered, the current iteration of the loop is terminated, and control is passed to the next iteration.
Flow control statements, such as if
, else
, and switch
, are used to control the flow of execution within a program. These statements allow developers to make decisions based on the values of variables or the results of expressions. For example, an if
statement can be used to execute a block of code only if a certain condition is met.
Both loop control and flow control statements are essential for writing efficient and effective JavaScript code. By using loop control statements, developers can optimize the performance of their code by terminating loops early or skipping unnecessary iterations. Similarly, by using flow control statements, developers can ensure that their code executes only when certain conditions are met, improving the overall reliability of their programs.
It’s important to note that while loop control statements are a type of flow control statement, they are distinct from other flow control statements in their behavior and purpose. By understanding the differences between these two types of statements, developers can write cleaner, more efficient, and more reliable code.
Best Practices for Break and Continue
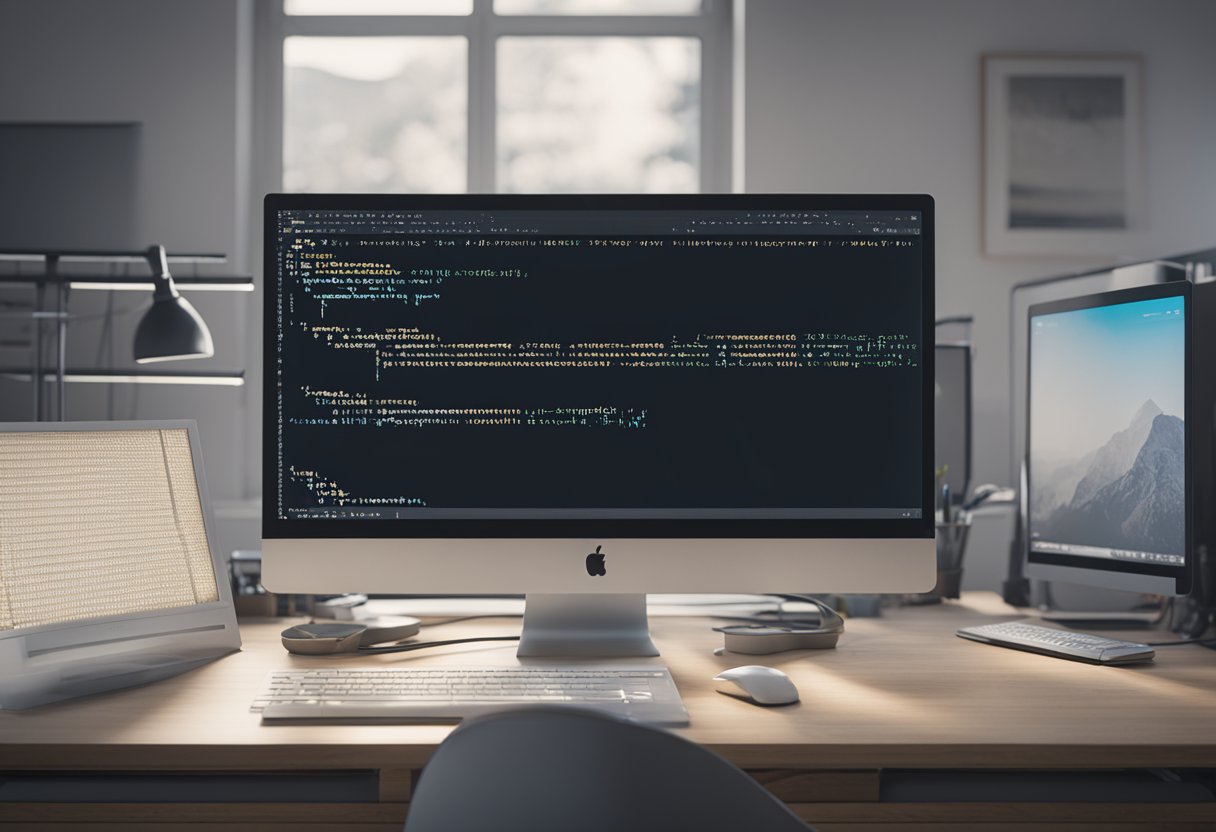
When using break
and continue
statements in JavaScript, it is important to follow best practices to ensure the code is readable, maintainable, and efficient. Here are some tips to keep in mind:
1. Use break
and continue
Sparingly
While break
and continue
can be useful in certain situations, overusing them can make the code harder to read and understand. It is recommended to use them only when necessary and to keep the number of break
and continue
statements to a minimum.
2. Limit Nested Loops
Nested loops can be difficult to read and understand, especially when break
and continue
statements are involved. It is recommended to limit the number of nested loops in the code and to use functions to break down complex logic.
3. Place break
and continue
Statements Carefully
Placing break
and continue
statements in the wrong place can lead to unexpected behavior and bugs. It is recommended to place them at the beginning or end of a loop, depending on the desired behavior.
4. Use Labels with break
and continue
Labels can be used with break
and continue
statements to specify which loop to exit or continue. This can be especially useful when dealing with nested loops. However, it is recommended to use labels sparingly and only when necessary, as they can make the code harder to read.
By following these best practices, developers can ensure that their code is easy to read, maintain, and efficient when using break
and continue
statements in JavaScript.
Common Pitfalls and How to Avoid Them
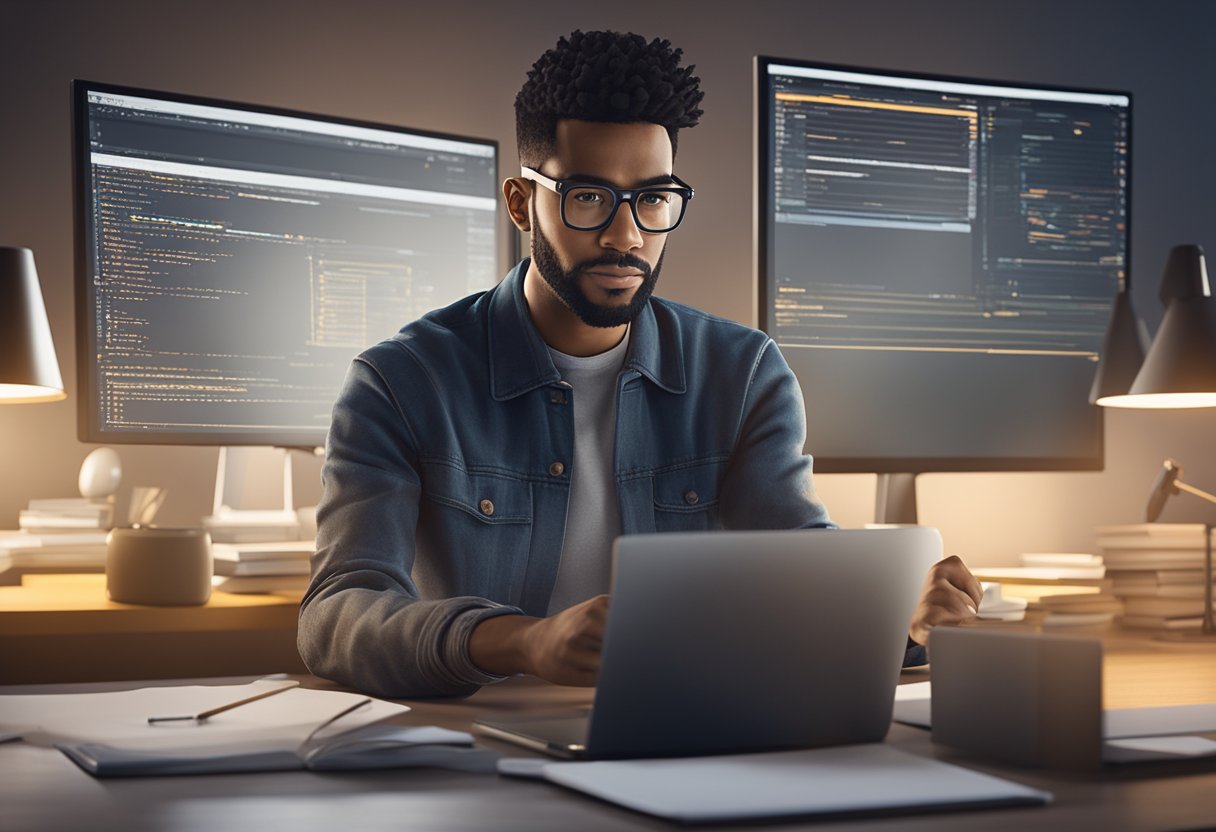
When using the break
and continue
statements in JavaScript, there are common pitfalls that developers should be aware of to avoid unexpected behavior in their code.
Forgetting to Use Labels
One common pitfall is forgetting to use labels when using break
and continue
statements in nested loops. If a label is not used, the break
or continue
statement will only affect the innermost loop, instead of breaking or continuing the outer loop as intended.
To avoid this, developers should always use a label when using break
or continue
statements in nested loops. The label should be placed before the outer loop, followed by a colon. For example:
outerloop:
for (var i = 0; i < 5; i++) {
innerloop:
for (var j = 0; j < 5; j++) {
if (i === 2 && j === 2) {
break outerloop;
} else if (i === 1 && j === 1) {
continue innerloop;
}
console.log("i = " + i + ", j = " + j);
}
}
In this example, the label outerloop
is used before the outer loop, and the break
statement is used with the label to break out of both loops when i
and j
are both equal to 2. The continue
statement is also used with the innerloop
label to skip the iteration when i
and j
are both equal to 1.
Using break
or continue
Outside of a Loop
Another common pitfall is using break
or continue
statements outside of a loop. This will result in a syntax error, as break
and continue
statements can only be used within loops.
To avoid this, developers should ensure that break
and continue
statements are only used within loops. If a break
or continue
statement is needed outside of a loop, developers should consider using a different approach to achieve the desired result.
Using break
or continue
in Switch Statements
Finally, using break
or continue
statements in switch statements can also lead to unexpected behavior. If a break
statement is not used within each case, the code will continue to execute the next case until a break
statement is reached.
To avoid this, developers should always include a break
statement within each case of a switch statement. If a continue
statement is needed, developers should consider using a different approach, as continue
statements are not allowed within switch statements.
By being aware of these common pitfalls and following best practices when using break
and continue
statements, developers can avoid unexpected behavior in their code and improve the overall quality of their JavaScript programs.
Frequently Asked Questions
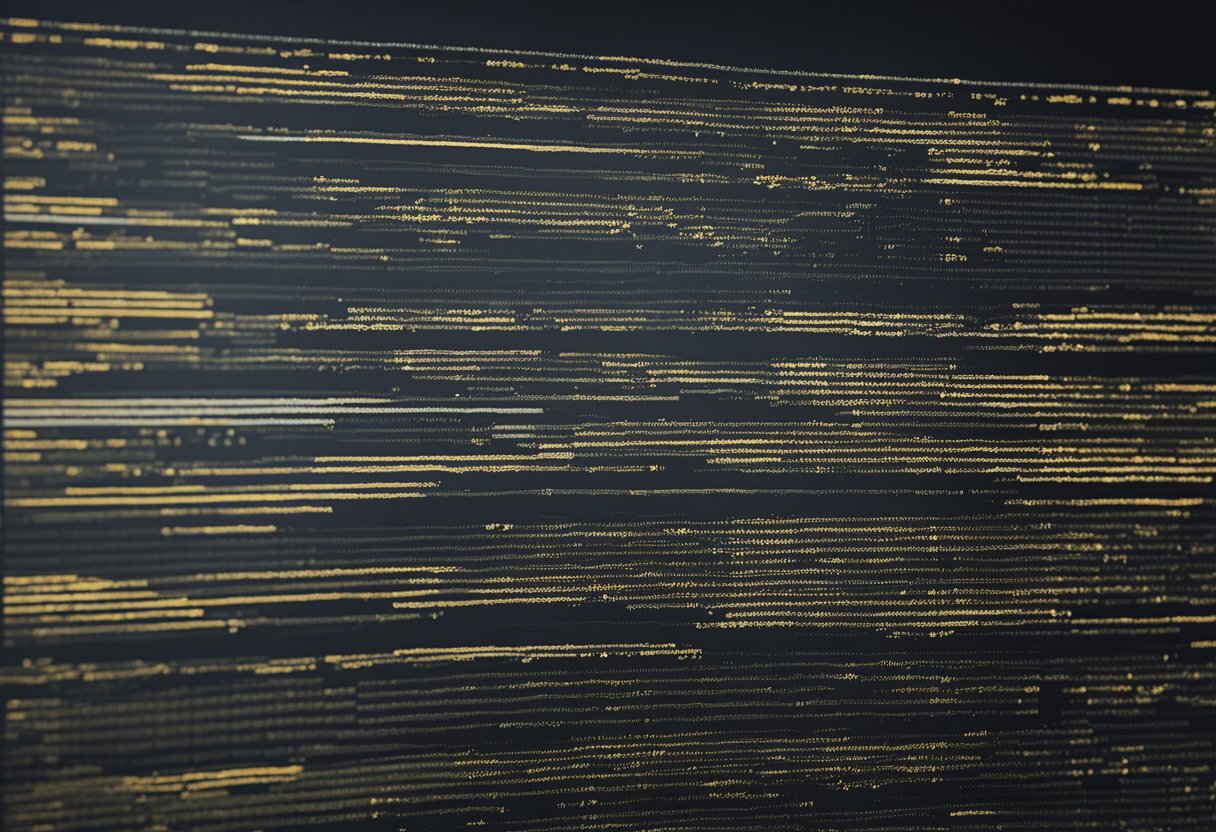
How do you use the continue statement within a loop in JavaScript?
In JavaScript, the continue
statement can be used within a loop to skip the current iteration and move on to the next one. When the continue
statement is encountered, the current iteration of the loop is immediately terminated, and the loop proceeds with the next iteration. The continue
statement is often used in conjunction with an if
statement to skip certain iterations based on a condition.
What is the difference between the break and continue statements in JavaScript?
In JavaScript, the break
and continue
statements are both used to alter the flow of control within a loop. The break
statement is used to exit the loop entirely, while the continue
statement is used to skip the current iteration and move on to the next one.
In which types of loops can the break statement be used in JavaScript?
In JavaScript, the break
statement can be used in any type of loop, including for
, while
, and do-while
loops. When the break
statement is encountered, the loop is immediately terminated, and the program continues executing from the next statement after the loop.
Can you use the continue statement in a forEach loop in JavaScript?
In JavaScript, the forEach
method is used to iterate over the elements of an array, and it does not support the use of the continue
statement. However, you can achieve similar functionality by using the Array.prototype.filter
method to create a new array containing only the elements you want to iterate over.
How can you prematurely exit a loop in JavaScript?
In JavaScript, you can prematurely exit a loop by using the break
statement. When the break
statement is encountered, the loop is immediately terminated, and the program continues executing from the next statement after the loop.
What are the effects of using break and continue in nested loops in JavaScript?
In JavaScript, when break
or continue
statements are used within nested loops, they only affect the innermost loop. When a break
statement is encountered, only the innermost loop is terminated, and the program continues executing from the next statement after the loop. Similarly, when a continue
statement is encountered, only the innermost loop skips the current iteration and proceeds with the next one.