Default parameters in JavaScript are a useful feature that allows developers to set default values for function parameters. When a function is called, if a parameter is not provided, the default value is used instead. This can help simplify code and make it more readable, as well as reduce the likelihood of errors.
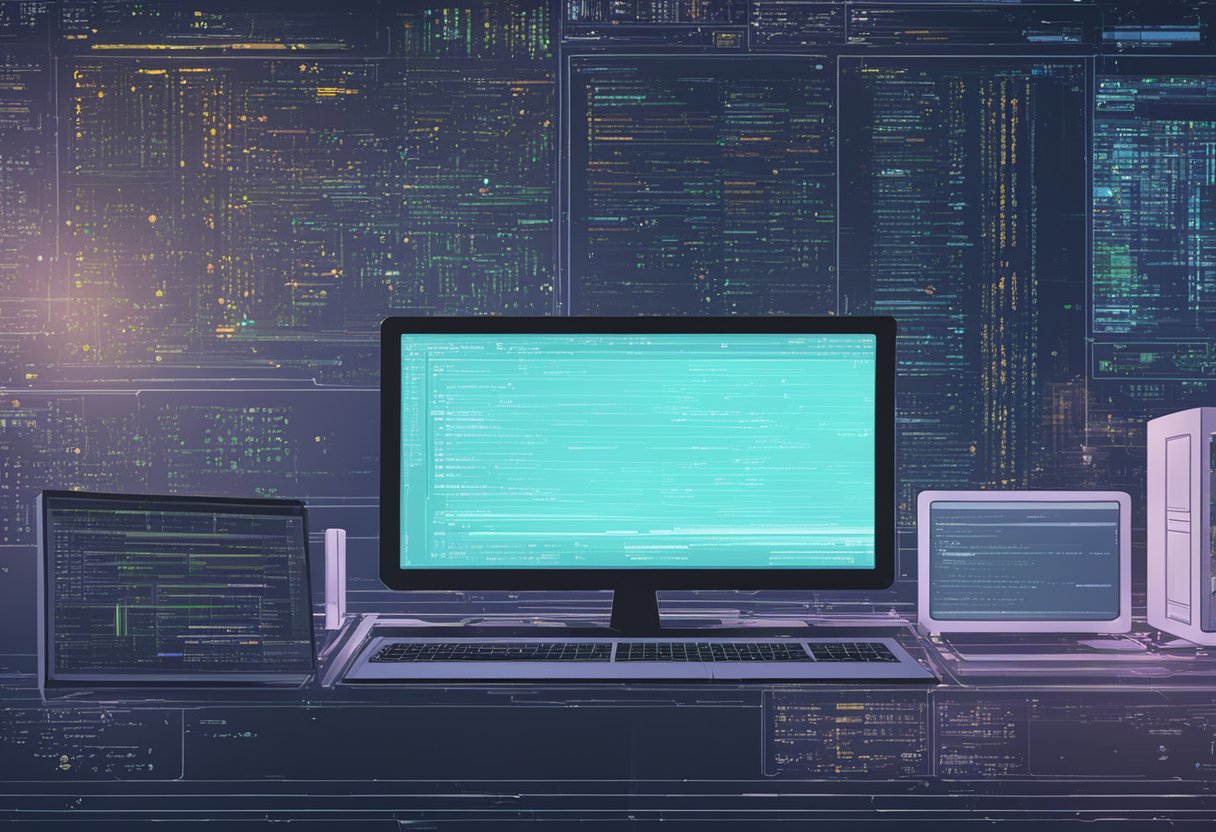
Understanding Default parameters was introduced in ECMAScript 6 (ES6) and are a powerful tool for JavaScript developers. In previous versions of JavaScript, developers had to use workarounds to achieve similar functionality, such as checking for undefined values and manually setting defaults. With default parameters, developers can define default values directly in the function signature, making code simpler, more concise, and easier to read.
Setting Default Values To set a default value for a parameter, simply add an equals sign followed by the default value after the parameter name in the function declaration. For example, to set the default value of a parameter named “x” to 42, the function declaration would look like this:
function myFunction(x = 42) {
// function code here
}
Key Takeaways
- Default parameters are a powerful tool that allows developers to set default values for function parameters.
- Default parameters were introduced in ECMAScript 6 and simplify code by allowing developers to define default values directly in the function signature.
- To set a default value for a parameter, add an equals sign followed by the default value after the parameter name in the function declaration.
Understanding Default Parameters
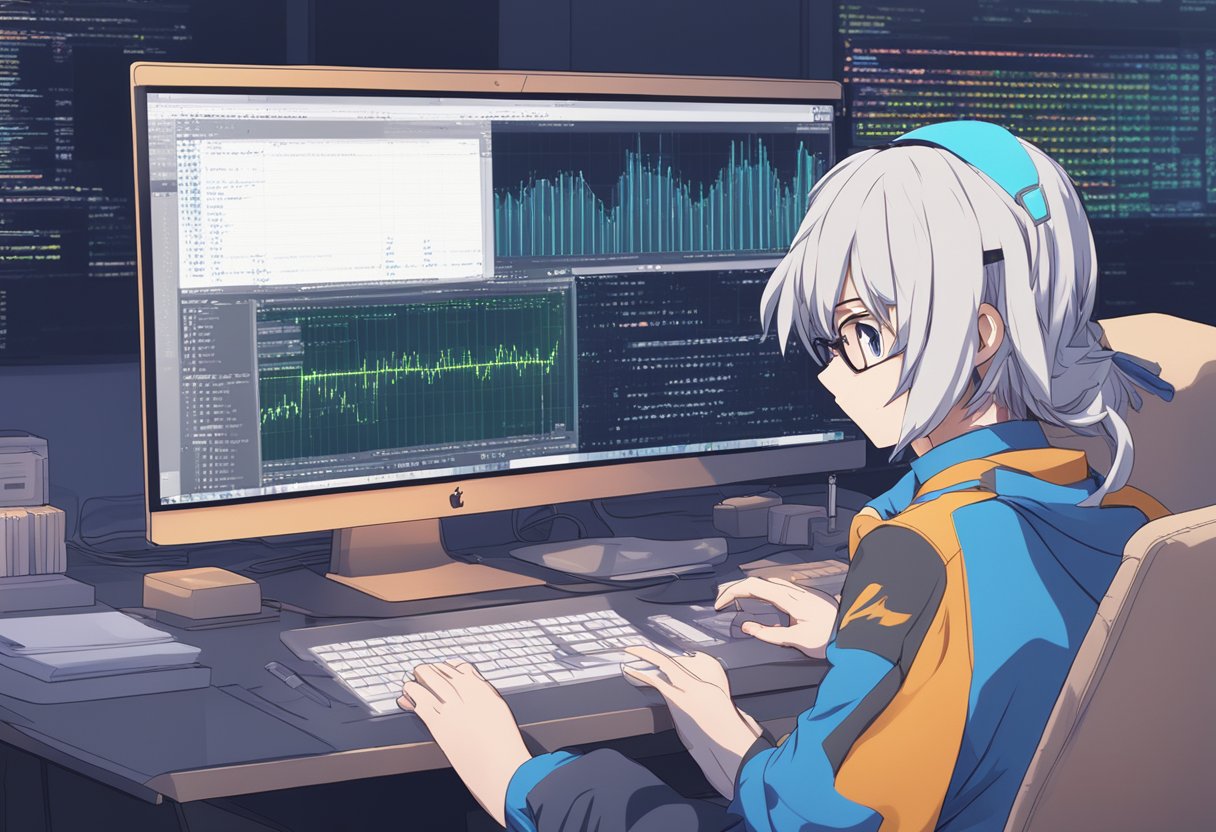
Default parameters are a feature in JavaScript that allows developers to set default values for function parameters. This feature was introduced in ECMAScript 6 (ES6) and has since become a popular tool for simplifying code.
History of Default Parameters
Before the introduction of default parameters in ES6, developers had to manually check if a parameter was undefined and set a default value if necessary. This was often done using a conditional statement like the following:
function exampleFunction(param) {
if (param === undefined) {
param = 'default value';
}
// rest of the function code
}
This approach was cumbersome and made code harder to read and maintain. Default parameters were introduced to simplify this process and make code more concise.
Syntax and Usage
The syntax for default parameters is straightforward. To set a default value for a function parameter, simply assign the value to the parameter in the function declaration. For example:
function exampleFunction(param = 'default value') {
// rest of the function code
}
In this example, if the parameter is not passed or is undefined, it will default to ‘default value’.
Default parameters can also reference other parameters in the same function declaration. For example:
function exampleFunction(param1, param2 = param1 * 2) {
// rest of the function code
}
In this example, if param2 is not passed or is undefined, it will default to twice the value of param1.
It’s important to note that default parameters only apply to parameters that are undefined or not passed. If a value is passed for a parameter, even if it’s null or an empty string, the default value will not be used.
Overall, default parameters are a useful feature that simplify code and make it easier to read and maintain. Developers should use them when appropriate to improve the clarity and efficiency of their code.
Setting Default Values
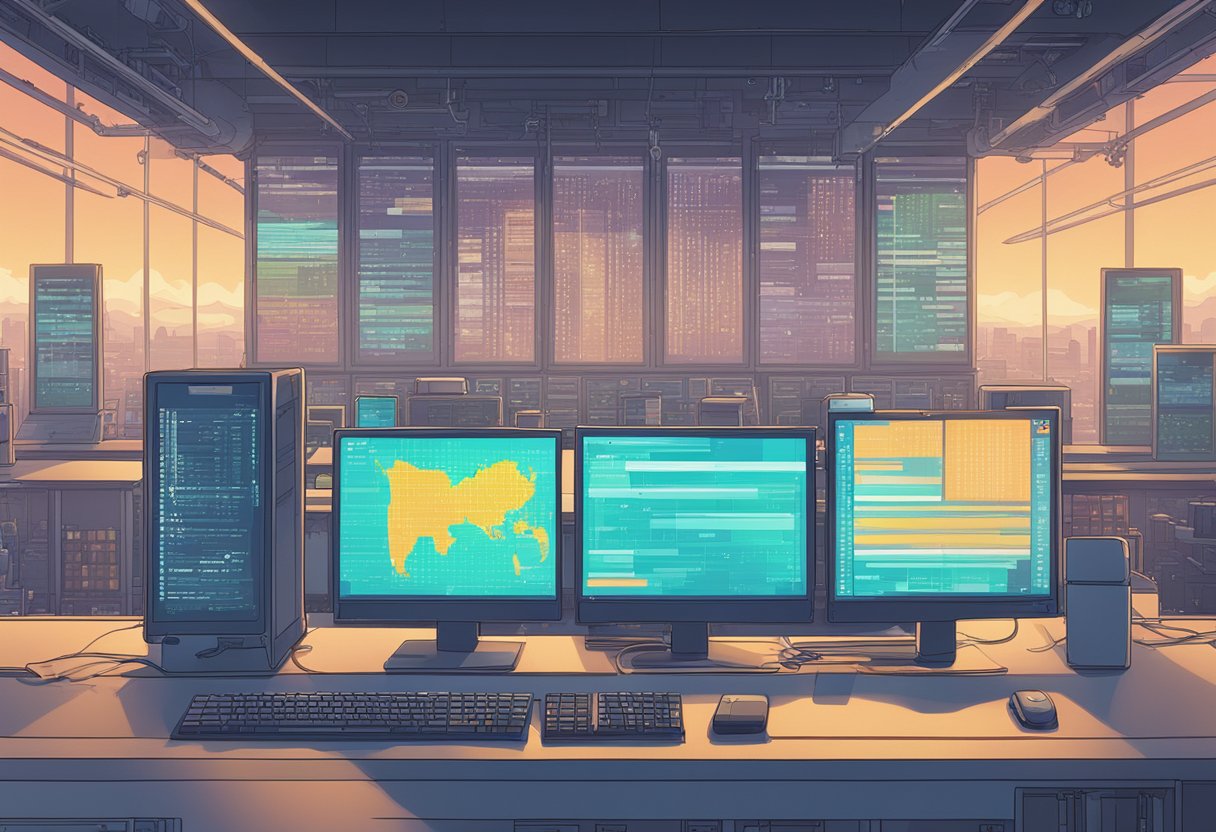
In JavaScript, default function parameters allow named parameters to be initialized with default values if no value or undefined
is passed. This feature was introduced in ES6 and is widely used in modern JavaScript development.
Using Undefined
When a function is called without passing a value for a parameter, the parameter’s value is set to undefined
. To avoid this, default parameter values can be set in the function declaration. For example, consider the following function:
function greet(name) {
console.log(`Hello, ${name}!`);
}
If the function is called without passing a value for the name
parameter, the output will be Hello, undefined!
. To set a default value for the name
parameter, the function can be rewritten as:
function greet(name = "World") {
console.log(`Hello, ${name}!`);
}
Now, if the function is called without passing a value for the name
parameter, the output will be Hello, World!
.
Using Null
It’s important to note that setting a default value for a parameter does not prevent the parameter from being set to null
. If a value of null
is passed to a parameter with a default value, the parameter will be set to null
. For example:
function greet(name = "World") {
console.log(`Hello, ${name}!`);
}
greet(null); // Output: Hello, null!
In this case, the name
parameter is set to null
instead of the default value of "World"
.
Overall, setting default values for function parameters is a useful feature in JavaScript that can help make code more readable and easier to maintain.
Advantages of Default Parameters
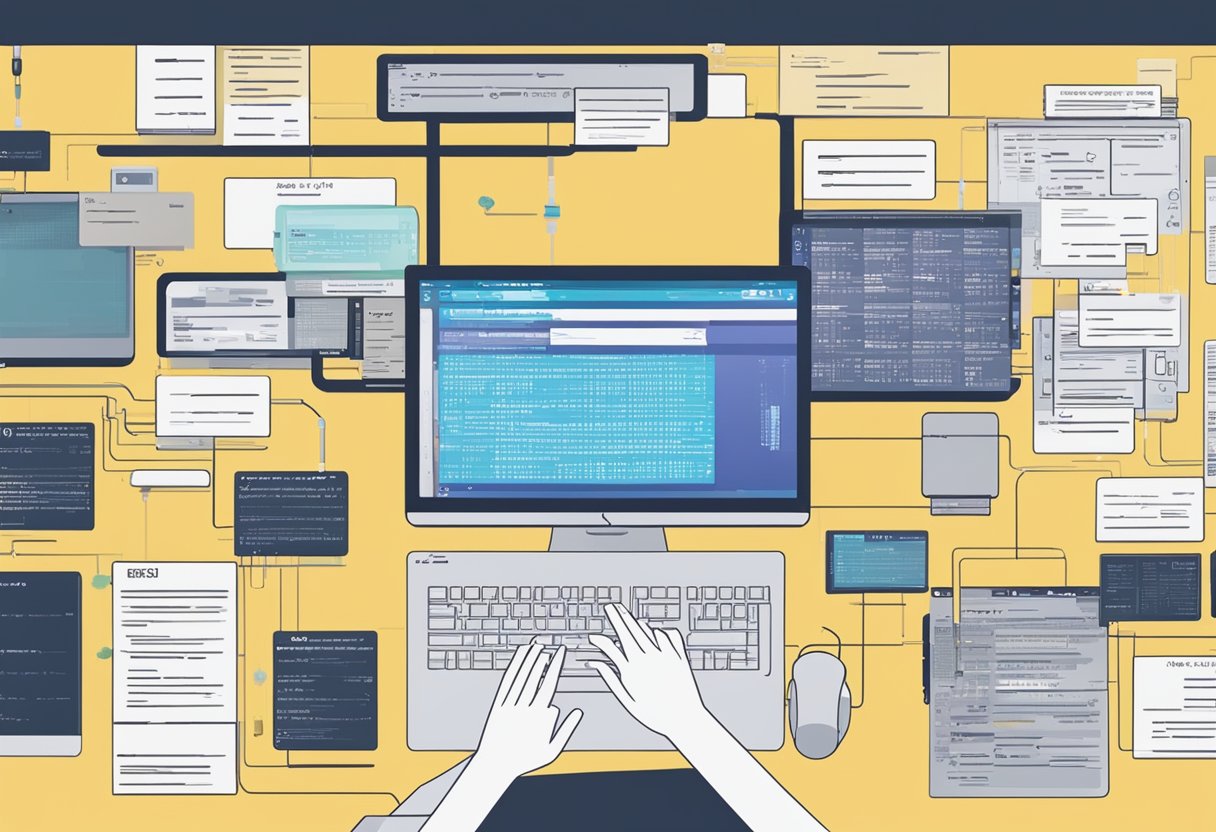
Default parameters in JavaScript provide several advantages that make them a useful feature for developers.
1. Simplifies Function Calls
Default parameters simplify function calls by allowing developers to define default values for parameters. This means that when a function is called, the developer does not have to explicitly pass a value for every parameter. Instead, if a parameter is not passed, the default value is used. This can make function calls more concise and easier to read.
2. Prevents Errors
Default parameters can help prevent errors that occur when a function is called without all the required parameters. When a default value is specified for a parameter, it ensures that the function will always have a value to work with. This can prevent errors that might occur if a function is called with missing parameters.
3. Increases Flexibility
Default parameters increase the flexibility of functions by allowing developers to define optional parameters. This means that a function can be called with different combinations of parameters, and the default values will be used for any missing parameters. This can make functions more versatile and easier to reuse in different contexts.
4. Improves Readability
Default parameters can improve the readability of code by making it easier to understand the purpose of a function. By specifying default values for parameters, developers can make it clear what values a function expects, and what the function will do if those values are not provided. This can make code easier to read and maintain over time.
In summary, default parameters provide several advantages for developers, including simplifying function calls, preventing errors, increasing flexibility, and improving readability. By using default parameters, developers can write more concise and versatile functions that are easier to understand and maintain.
Common Mistakes and Pitfalls

Ignoring Side Effects
One common mistake with default parameters is ignoring the side effects of using them. When a default parameter is set to a mutable object like an array or an object, it can cause unexpected behavior. This is because the default value is only created once when the function is defined, and not every time the function is called. As a result, if the default parameter is modified in the function, it will affect all future calls of the function.
For example, consider the following code snippet:
function addItem(item, list = []) {
list.push(item);
return list;
}
console.log(addItem('apple')); // Output: ['apple']
console.log(addItem('banana')); // Output: ['apple', 'banana']
In the above code, the default value of the list
parameter is an empty array. However, since the same array is used every time the function is called, the second call to addItem()
returns ['apple', 'banana']
instead of just ['banana']
. To avoid this issue, it is recommended to use undefined
as the default value and create a new array or object inside the function when needed.
Overriding Defaults Unintentionally
Another common mistake is unintentionally overriding the default value of a parameter. This can happen when the function is called with undefined
as the value for a parameter, which will cause the default value to be used instead.
For example, consider the following code snippet:
function greet(name = 'World') {
console.log(`Hello, ${name}!`);
}
greet(undefined); // Output: Hello, World!
In the above code, the greet()
function has a default value of 'World'
for the name
parameter. However, when the function is called with undefined
as the value for name
, the default value is used instead of the intended value. To avoid this issue, it is recommended to always pass a value for every parameter, even if it is the default value.
Overall, default parameters can be a powerful tool in JavaScript, but they should be used with caution to avoid unintended consequences.
Best Practices

When working with default parameters in JavaScript, it’s important to keep in mind some best practices to ensure code readability, maintenance, and performance.
Readability and Maintenance
Default parameters can make code more readable and maintainable by providing a clear indication of what the function expects. It’s a good practice to use descriptive parameter names, especially when the function has more than one parameter. This makes it easier for other developers to understand the purpose of the function and its parameters.
Another best practice is to avoid using complex expressions as default parameter values, as they can make the code harder to understand and maintain. Instead, use simple and concise values that clearly communicate their purpose.
Performance Considerations
While default parameters can improve code readability and maintenance, they can also have an impact on performance. When a function is called with fewer arguments than its defined parameters, default parameters are used to fill in the missing values. This means that default parameters are evaluated every time the function is called, even if they are not used.
To avoid unnecessary evaluations, it’s recommended to use undefined
as the default value instead of a complex expression. This way, the default value is only evaluated when it’s actually needed.
It’s also important to keep in mind that default parameters are not the same as optional parameters. Optional parameters are defined using undefined
as the default value, while default parameters are defined using an actual default value. This means that optional parameters can be omitted when calling the function, while default parameters cannot.
Overall, by following these best practices, developers can ensure that their code is both readable and performant when working with default parameters in JavaScript.
Use Cases and Examples

Default parameters can be very useful in certain situations. For instance, when a function is expected to be called with a certain number of arguments, but some of them are optional, default parameters can be used to provide default values for the optional parameters. This can make the code more concise and easier to read.
In JavaScript, function parameters default to undefined
. However, with the use of default parameters, a different default value can be set. For example, consider the following code:
function greet(name = 'World') {
console.log(`Hello, ${name}!`);
}
In this example, if no argument is passed to the greet
function, it will use the default value of 'World'
for the name
parameter. If an argument is passed, it will use that value instead. This allows for more flexibility in how the function is called.
Another use case for default parameters is when a function is called with an argument that is not provided by the caller. In this case, the default parameter can be used to provide a default value for the missing argument. For example:
function multiply(a, b = 1) {
return a * b;
}
In this example, if the b
parameter is not provided when the multiply
function is called, it will default to 1
. This can be useful in cases where a default value is needed for a certain parameter, but it is not always provided by the caller.
It is also possible to use expressions as default values for parameters. For example:
function sum(x = 1, y = x, z = x + y) {
console.log(x + y + z);
}
In this example, the default value of x
is 1
, the default value of y
is the value of x
, and the default value of z
is the sum of x
and y
. This allows for more complex default values to be used for parameters.
Overall, default parameters can be a powerful tool in JavaScript for making functions more flexible and easier to read. By providing default values for optional parameters, missing arguments, or complex expressions, default parameters can help simplify code and make it more maintainable.
Compatibility and Transpilation

JavaScript is constantly evolving, and new features are being added to the language. However, not all browsers support the latest features of the language. This is where compatibility and transpilation come in.
ES6 Support
ES6 introduced several new features, including default parameters. However, not all browsers support these features. For example, Internet Explorer does not support default parameters. Therefore, if you want to use default parameters in your code, you need to make sure that your code is compatible with all browsers.
Transpiling for Older Browsers
One way to ensure that your code is compatible with all browsers is to use transpilation. Transpilation is the process of converting code written in one version of JavaScript to another version of JavaScript. For example, you can use a transpiler like Babel to convert code written in ES6 to ES5.
Transpiling your code ensures that your code is compatible with older browsers that do not support the latest features of the language. However, transpiling your code can also increase the size of your code, which can slow down your website. Therefore, it is important to weigh the benefits of transpiling your code against the drawbacks.
In conclusion, default parameters are a useful feature in JavaScript, but not all browsers support them. Therefore, it is important to ensure that your code is compatible with all browsers by using transpilation.
Advanced Concepts
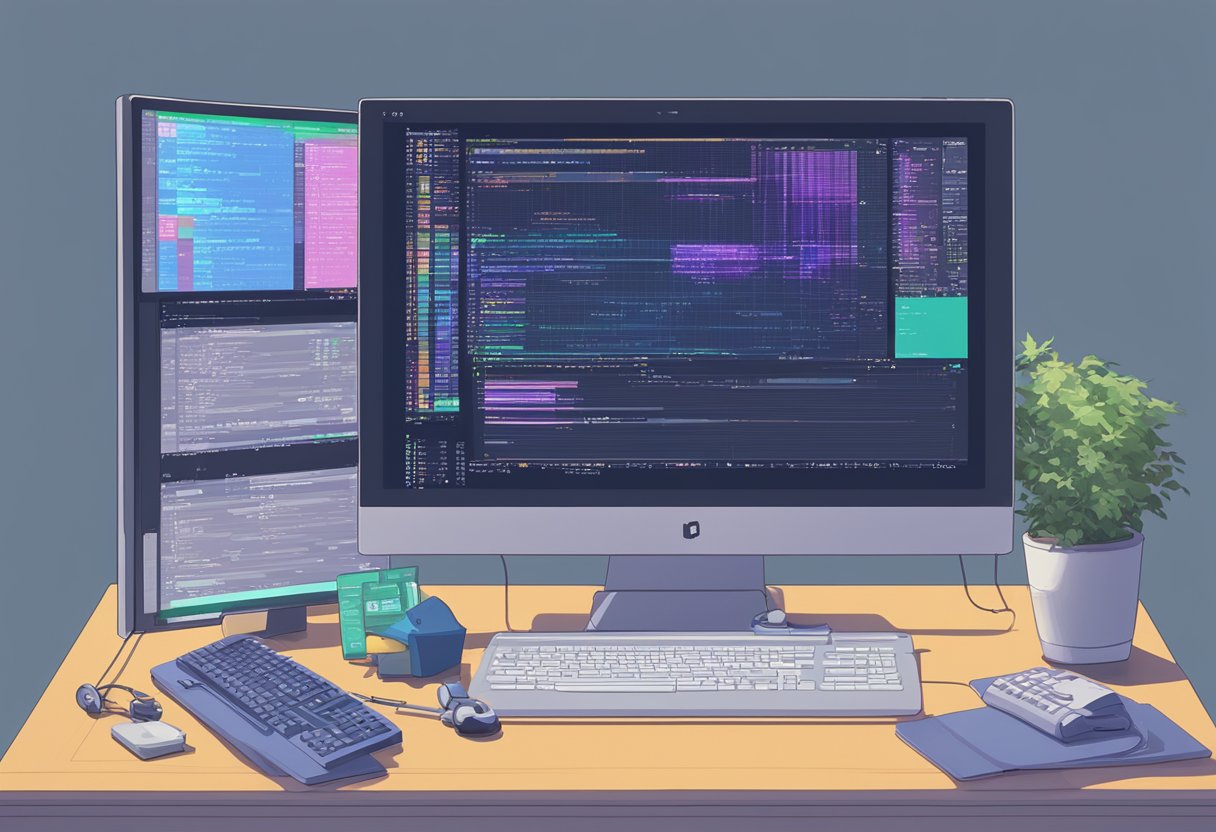
Using Default Parameters with Destructuring
Destructuring is a powerful feature in JavaScript that allows developers to extract values from arrays and objects and assign them to variables. When used in combination with default parameters, destructuring can provide even greater flexibility and control over function behavior.
For example, consider the following function that takes an options object as its parameter:
function getUser({ username = 'anonymous', password = 'password' } = {}) {
// ...
}
This function uses destructuring to extract the username
and password
properties from the options
object. If no options
object is provided, the default value of an empty object is used instead. Notice that both username
and password
have default values of 'anonymous'
and 'password'
, respectively.
Default Parameters and Arrow Functions
Arrow functions are a concise and convenient way to write functions in JavaScript. They also work seamlessly with default parameters.
Consider the following example:
const multiply = (a, b = 1) => {
return a * b;
};
This arrow function takes two parameters, a
and b
. However, b
has a default value of 1
, which means that if no value is provided for b
, it will default to 1
. This function can be called in the following ways:
multiply(5, 2); // returns 10
multiply(5); // returns 5
In the first example, both a
and b
are provided with values. In the second example, only a
is provided with a value, and b
defaults to 1
.
Overall, default parameters in JavaScript provide a powerful tool for writing flexible and robust functions. When used in combination with destructuring and arrow functions, developers can create even more expressive and concise code.
Summary

In JavaScript, function parameters default to undefined
. However, it is often useful to set a different default value. This is where default parameters can help. Default parameters allow developers to define a default value for a function parameter that will be used when no argument is provided for the parameter during function call.
Default parameters were introduced in ECMAScript 2015 (ES6). Prior to ES6, developers had to use conditional statements to check if a parameter was undefined and then assign a default value. With default parameters, developers can now define default values directly in the function signature.
To define a default parameter, simply assign a value to the parameter in the function signature. For example, function multiply(a, b=1) { ... }
defines a default value of 1
for the b
parameter.
Default parameters can also be expressions, not just values. This means that developers can use functions or other expressions to define default parameter values. For example, function multiply(a, b=getDefault()) { ... }
would call the getDefault()
function if no value is provided for b
.
It is important to note that default parameters are only used when no value or undefined
is passed for the parameter. If a value is passed, even if it is null
, the passed value will be used instead of the default parameter value.
Overall, default parameters provide a convenient way to set default values for function parameters, making code more concise and readable.
Frequently Asked Questions

How do you define default parameters for a function in JavaScript?
In JavaScript, default parameters can be defined by assigning a value to a parameter in the function declaration. When a function is called without an argument for that parameter, the default value will be used instead. For example, function multiply(a, b = 1) { return a * b; }
defines a default value of 1 for the parameter b
.
Can you provide an example of using default parameters with arrays in JavaScript?
Yes. Default parameters can be used with arrays in JavaScript by defining an empty array as the default value for a parameter. For example, function logArray(arr = []) { console.log(arr); }
defines an empty array as the default value for the arr
parameter. If the function is called without an argument for arr
, an empty array will be logged to the console.
What is the syntax for using object destructuring with default parameters in JavaScript?
In JavaScript, object destructuring can be used with default parameters by wrapping the destructured object in parentheses and assigning a default value to the entire object. For example, function logPerson({ name = 'John', age = 30 } = {}) { console.log(name, age); }
defines an object with default values for the name
and age
properties. If the function is called without an argument for the destructured object, an empty object will be used instead.
How can default parameters be implemented in TypeScript functions?
In TypeScript, default parameters can be implemented in the same way as in JavaScript. However, TypeScript also allows for optional parameters to be defined with a question mark after the parameter name. For example, function multiply(a: number, b: number = 1) { return a * b; }
defines a default value of 1 for the b
parameter and requires the a
parameter to be a number.
What is the behavior of a function when no arguments are passed to parameters with default values in JavaScript?
When no arguments are passed to parameters with default values in JavaScript, the default value will be used instead. For example, function multiply(a, b = 1) { return a * b; }
defines a default value of 1 for the b
parameter. If the function is called with only one argument for a
, the default value of 1 will be used for b
.
How do you handle optional parameters in JavaScript functions using default values?
In JavaScript, optional parameters can be handled using default values. By defining a default value for a parameter, the parameter becomes optional. For example, function logName(firstName, lastName = '') { console.log(firstName, lastName); }
defines the lastName
parameter as optional by providing a default value of an empty string. If the function is called with only one argument for firstName
, an empty string will be used for lastName
.