Understanding Null and Undefined
When working with JavaScript, understanding the types null
and undefined
is crucial because they represent different concepts yet are often mistaken for each other. null
is a deliberate assignment, signifying ‘no value’ and is used when you want to intentionally clear an object or indicate that a given variable should be ’empty’ or ‘non-existent’. Conversely, undefined
signals an uninitialized state, implying that a variable has been declared but hasn’t yet been assigned a value. It’s the default value of any new variables you declare without an explicit initial value.
In code operations, especially comparisons, these types behave in ways that might be unexpected. Both null
and undefined
are falsy values, which means they behave like false
in conditions but are not equivalent to false
explicitly.1 However, when compared with double equals ==
, null
and undefined
are considered equal because JavaScript performs type coercion and considers them similar in this context. This doesn’t hold when using triple equals ===
, as this operator also checks the type, which is different for each.
Additionally, confusion often arises since null
is of type “object”, something to remain aware of because it might lead one to think it carries properties or methods. On the other hand, undefined
is of type undefined
, making its identification straightforward in most debugging situations.
Null or undefined can also lead to multiple types of runtime errors if not handled correctly. For example, accessing properties on null
or undefined
values will throw a TypeError, often leading to script breakdowns if proper checks are not implemented. It’s generally good practice to validate whether a variable is neither undefined nor null before utilizing it further in functions or conditionals.
To enhance code safety and avoid pitfalls with null and undefined, frameworks and enhancements like TypeScript can enforce stricter checks, allowing developers to handle these scenarios more predictively. TypeScript introduces optional typing and strict null checks that can significantly prevent common JavaScript bugs associated with unchecked null or undefined values.
In system design or when interfacing with databases and other languages that may furnish values representing non-existence or void entries differently, understanding and correctly handling null and undefined becomes a necessity for error-resistant code.
Practical Uses of Null and Undefined
In functions, returning null
can be a deliberate action to communicate the absence of a return value meaningfully. Consider a function designed to fetch user data from a database; if no records are found for a given user ID, returning null
might indicate this absence explicitly, allowing the caller to handle the scenario appropriately:
function getUserData(userId) {
const userData = database.find(user => user.id === userId);
if (!userData) {
return null;
}
return userData;
}
In the consuming code, you can specifically check for a null
return to decide the subsequent actions — possibly showing a user-friendly message or logging the incident.
const userData = getUserData(requestedUserId);
if (userData === null) {
console.log('No user found for the provided ID.');
}
Similarly, undefined
often appears with variables that have been declared but not assigned a value. In JavaScript, function parameters that are not passed behave as undefined
. This characteristic can be useful for setting default values in function parameters:
function greet(name = 'Guest') {
console.log(`Hello, ${name}!`);
}
greet(); // Output: "Hello, Guest!"
Here, if no name is provided, name
defaults to "Guest"
because the function parameter name
is undefined
and the default parameter value is utilized.
Error handling is another significant area where null
and undefined
demonstrate their utility. Detecting values explicitly set to null
can help segregate cases where an error or an unusual event needs to be handled differently than a typical “value not found” or uninitialized variable case:
function processPayment(amount) {
if (amount === null) {
throw new Error('Invalid payment amount.');
}
if (typeof amount === 'undefined') {
console.log('Payment amount not initialized.');
amount = setDefaultPayment();
}
// Proceed with processing the payment
}
The checking sequence illustrates using both null
and undefined
to ensure that the amounts are properly captured or handled, serving as gatekeepers before proceeding further into the business logic. This reduces the probability of bugs related to unhandled cases of absence or non-initialization of data.
TypeScript’s Strict Null Checking
TypeScript’s strictNullChecks
configuration plays a critical role in solidifying type safety within your applications, essentially serving as a watchdog against common null
and undefined
bugs that could lead to runtime errors. When enabled, this flag requires all variables and function parameters to be explicitly declared as either nullable or not, thereby fortifying your code by enforcing a stricter set of checks before compilation.
Under strictNullChecks
, TypeScript treats null
and undefined
as types that must be explicitly accounted for in your code logic. For instance, if a variable is intended to hold a string or remain undefined, it must be declared with a union type like string | undefined
. Without the type annotation to accommodate undefined
, trying to assign such a value to the string variable would result in a type error during compilation.2 This prevents a significant class of errors where functions are invoked with missing arguments, or object properties are accessed without prior initialization.
Consider the following scenario in TypeScript without strictNullChecks
enabled:
let user: { name: string; age?: number };
user = { name: 'Alice' };
console.log(user.age.toFixed(2)); // Potential Runtime Error
In this example, accessing age
from user
and performing a method like toFixed
on it would not cause a compilation error. However, it would crash at runtime because age
is undefined
and undefined
doesn’t have a toFixed
method. This illustrates the type of problem strictNullChecks
seeks to mitigate.
With strictNullChecks
enabled, TypeScript injects refined wisdom into your codebase:
let user: { name: string; age?: number | undefined };
user = { name: 'Alice' };
// TypeError: Object is possibly 'undefined'.
console.log(user.age.toFixed(2));
Here, TypeScript raises a compilation error, flagging that age
might be undefined
. This fosters developers to explicitly handle such cases, for instance, by adding checks or using optional chaining (user.age?.toFixed(2)
) which evaluates to undefined
instead of throwing an error if age
is undefined
.
The advantage is clear: using strictNullChecks
guides developers to properly strategize how their variables are initialized and interacted with throughout the application. This promotes error handling and drives home the importance of thoughtful system design choices where certainty about variable states leads to more predictable and stable execution paths.
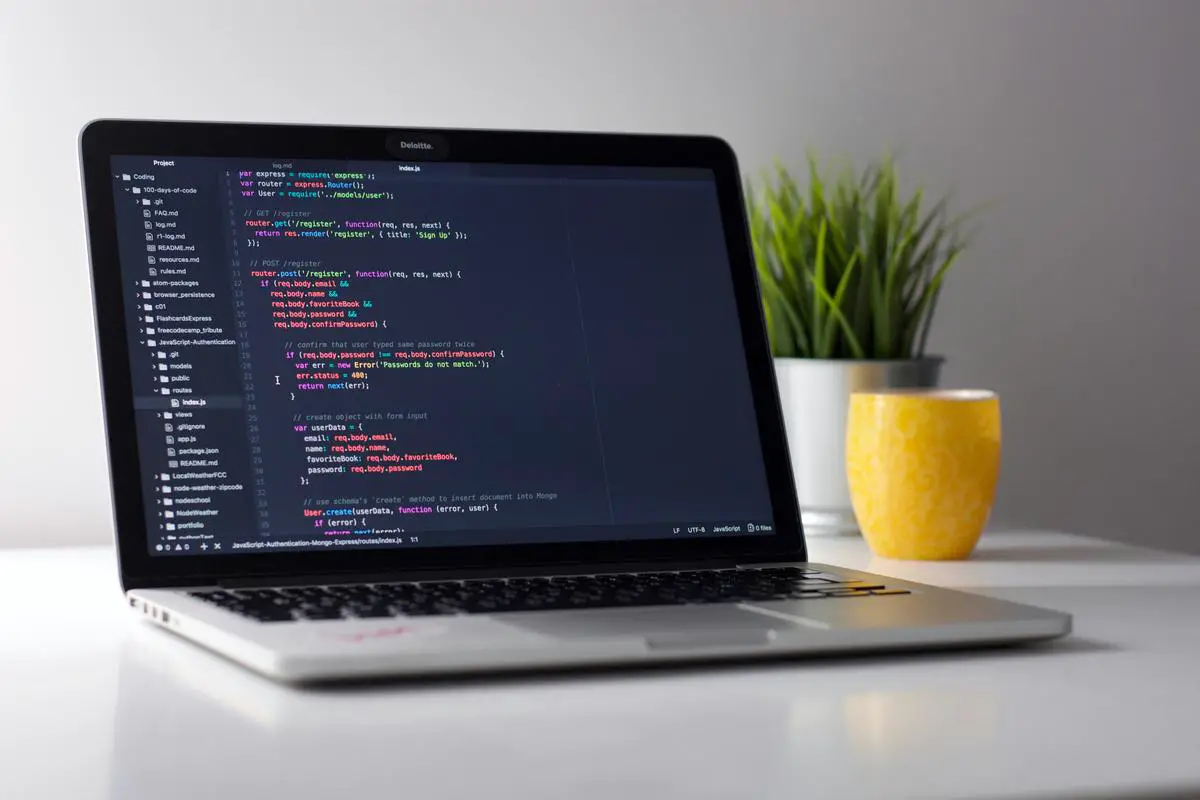
Photo by clemhlrdt on Unsplash
Nullable Types and Optional Chaining
In TypeScript, nullable types allow you to specify that a variable may not only hold a value of a specific type but could also be null. This explicit acknowledgment of nullability enhances type safety because TypeScript ensures that you deal explicitly with the presence of ‘null’. The syntax for declaring a nullable type involves the use of union types, which you can symbolize with the pipe (‘|
‘) character to denote that the variable could either hold a specific type or be null.
For instance, consider a case where you have an object representing a book. A book must always have a name, but publication year might be unknown at the point of creating some instances:
interface Book {
name: string;
publicationYear?: number | null;
}
In this interface definition (Book
), the publicationYear
property is defined as being either a number or null, indicating that the publication year might either have a value or explicitly lack one (denoted by null
), or might not be defined at all (undefined
), implying the lack of even potentially knowable information.
Additionally, when accessing properties with potential null or undefined values, TypeScript enables you to write cleaner code using the optional chaining operator (?.
). Optional chaining simplifies your code when accessing values deep within a chain of connected objects when any of them might be null or undefined.
For example:
let book: Book = {
name: "Demon Haunted World",
publicationYear: null
};
let publicationInfo = book.publicationYear?.toString() ?? "Publication year unknown";
Here, the optional chaining operator (?.
) allows publicationYear
to be safely accessed. If publicationYear
is null
or undefined
, the expression short-circuits, and undefined
is returned. This undefined result is further handled by the nullish coalescing operator (??
), which provides a default value (“Publication year unknown”) when the left-hand operand evaluates to either null
or undefined
.
Another relevant example is removing noisy error handling code when accessing deeply nested properties:
let config = {
network: {
proxy: {
address: null
}
}
};
let proxyAddress = config.network?.proxy?.address ?? "No Proxy Configured";
Here, accessing each level with ?
guards against crashes that can occur when trying to access a property of null
or undefined
. If any segment in this access path evaluates to null
or undefined
, the chain evaluation stops at that point, returning undefined
, and again the nullish coalescing operator (??
) delivers a meaningful default.
Essentially, nullable types and optional chaining in TypeScript provide structural and operational security. It ensures that your type assumptions are clear throughout the code, and potential null
or undefined
values do not cause unforeseen errors, thereby harmonizing error-checking and enhancing code reliability.
Error Handling with Null and Undefined
To manage errors effectively in JavaScript and TypeScript, especially those involving null
and undefined
, developers must adopt practices and tools that enhance error detection and troubleshooting. Proper handling of these values can safeguard applications from pitfalls like type errors and runtime crashes.
Employ Conditional Checks
Before using values that could be null
or undefined
, perform conditional checks to verify their availability:
function processUser(user) {
if (user !== null && user !== undefined) {
console.log(`Processing ${user.name}`);
} else {
console.warn('Attempted to process invalid user.');
}
}
The function checks whether user
is neither null
nor undefined
before proceeding, guarding against processing invalid data.
Apply Default Values with Short-Circuiting
JavaScript’s logical OR operator (||
) allows fallback to default values when dealing with null
or undefined
:
function greet(name) {
const userName = name || 'Guest';
console.log(`Hello, ${userName}!`);
}
greet(); // Output: "Hello, Guest!"
If name
is null
or undefined
, userName
falls back to 'Guest'
, avoiding errors like accessing properties on null
or undefined
.
Leverage TypeScript’s Non-Nullable Types
TypeScript developers should use non-nullable types available through the strictNullChecks
option. It ensures values are not null
or undefined
without explicit handling:
function getLength(text: string | null) {
if (text === null) {
return 0;
}
return text.length;
}
TypeScript forces handling the null
case for text
, preventing potential runtime errors.
Utilize Optional Chaining and Nullish Coalescing in TypeScript
TypeScript’s optional chaining (?.
) and nullish coalescing (??
) operators are useful for dealing with deep object structures which might contain null
or undefined
:
interface User {
details?: {
age: number | null;
};
}
const user: User = {};
const userAge = user.details?.age ?? "Unknown";
console.log(`User age is ${userAge}.`); // Output: "User age is Unknown."
?.
prevents runtime errors by returning undefined
if any part of the chain is null
or undefined
, and ??
provides a default value.
Assert Non-null Conditions
Sometimes, you know more than TypeScript about whether a value is null. TypeScript’s non-null assertion operator (!
) can avoid verbosity while telling the compiler the value is not null
or undefined
:
function handleElement(elementId: string) {
const element = document.getElementById(elementId)!; // Assert element is non-null
console.log(`Handling element with id: ${element.id}`);
}
The !
post-fix expression asserts that the value will not be null or undefined.
Deploy Custom Error Handling Strategies
Incorporate structured error handling in your applications. For complex operations liable to fail due to missing values, use try-catch blocks:
try {
let result = riskyOperation();
} catch (error) {
console.error('Operation failed:', error);
}
A proactive approach to error management, particularly with operations involving potential null
and undefined
errors, safeguards your applications from unexpected failures.1
Following these strategies will bolster your JavaScript and TypeScript code’s reliability when dealing with null
and undefined
. It will also improve your development standard. By defensively coding and employing best practices, you can enhance your software’s efficacy and maintainability.