When working with programming languages, it is essential to have a clear understanding of enumerations, which define a set of named constants. Enumerations are useful for defining a list of related values that don’t change, such as days of the week or colors. In addition, enums can be used to define a set of options for a user to choose from in a program.
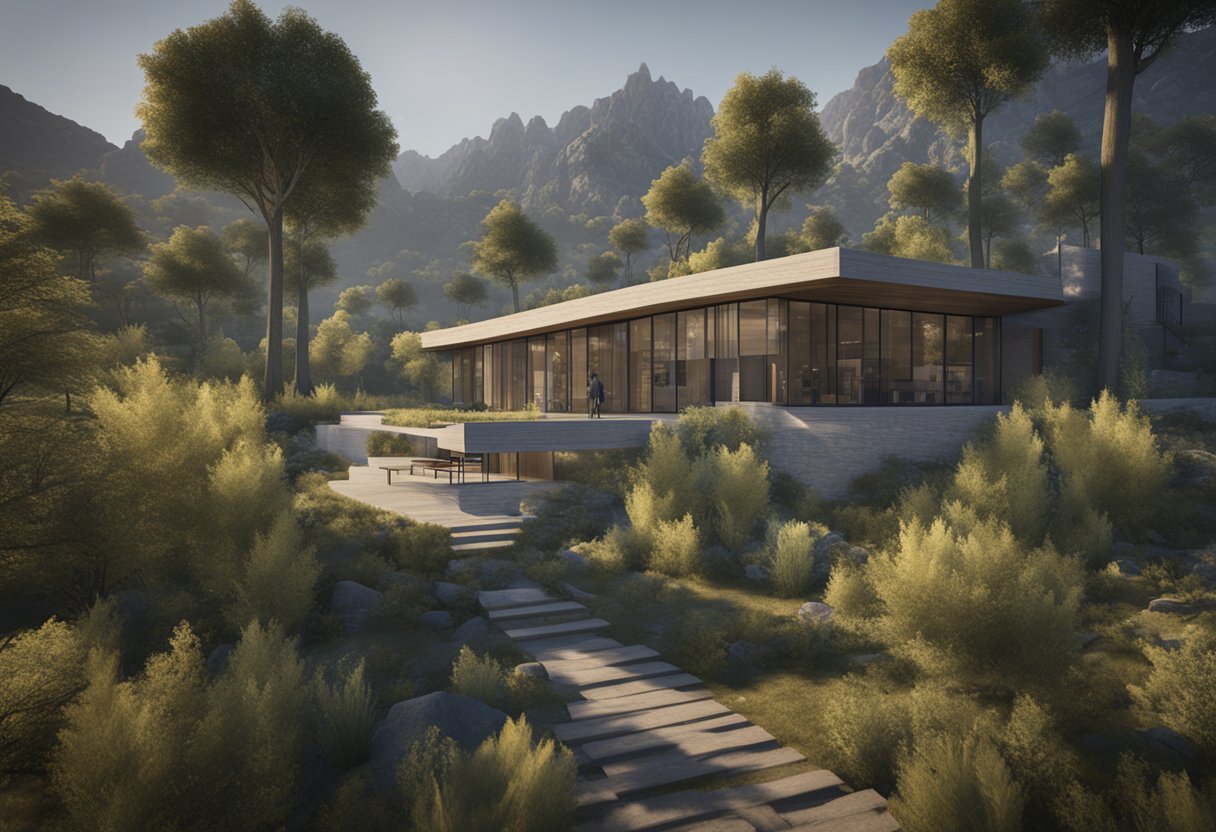
One common use case for enumerations is to define them with string values. This allows developers to assign a string value to each constant in the enumeration. For example, an enumeration of colors could be defined with string values like “Red”, “Green”, and “Blue”. Defining enums with string values can be useful when working with user interfaces or when the values need to be displayed to the user.
Char Values in Java: Understanding the Basics
To define an enum with string values, developers can use a variety of programming languages, including C++, Java, and Swift. The exact syntax may differ slightly between languages, but the basic concept is the same. By defining an enum with string values, developers can create more readable and maintainable code.
Key Takeaways
- Enumerations are useful for defining a set of named constants that don’t change.
- Defining enums with string values can be useful for displaying values to the user or working with user interfaces.
- By defining an enum with string values, developers can create more readable and maintainable code.
Understanding Enumerations
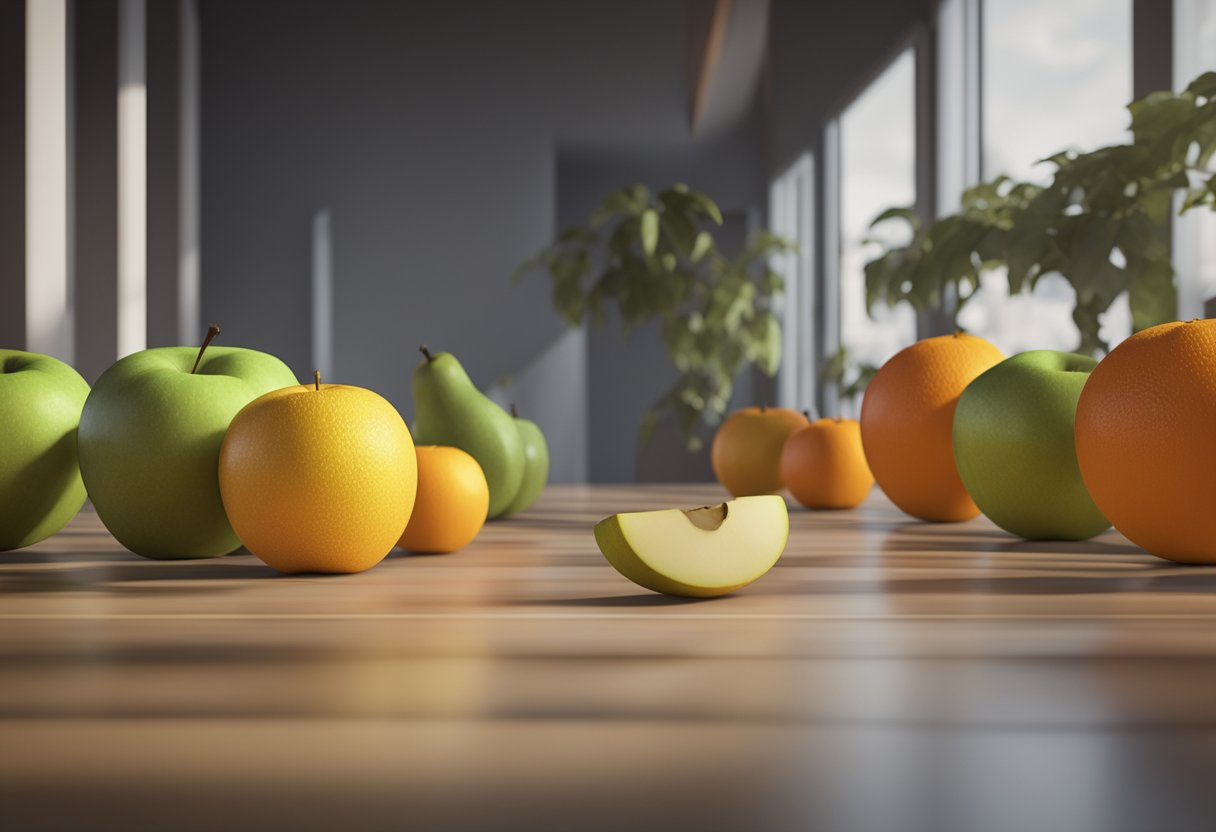
Definition and Use Cases
An enumeration, or enum for short, is a user-defined type in programming languages that consists of a set of named values. Enumerations are commonly used to define a fixed set of values that a variable can take. They are useful in situations where there are a limited number of options or when the data needs to be restricted to a certain set of values.
One of the most common use cases for enumerations is to define a set of string values that a variable can take. This is useful when the programmer wants to restrict the input to a specific set of strings. For example, an enumeration of strings could be used to define the days of the week or the months of the year.
Underlying Data Types
Under the hood, enumerations are represented by an underlying data type. This data type can vary depending on the programming language, but it is usually an integer. Each named value in the enumeration is assigned a unique integer value, starting from 0 and incrementing by 1.
When defining an enumeration of strings, each named value is assigned a string value instead of an integer. This allows the programmer to use the named values as strings in their code.
In some programming languages, it is also possible to assign custom integer values to the named values in the enumeration. This can be useful when the programmer wants to assign specific integer values to the named values.
Overall, enumerations are a powerful tool in programming languages that allow programmers to define a fixed set of values that a variable can take. When defining an enumeration of strings, each named value is assigned a string value instead of an integer.
Defining Enums with String Values
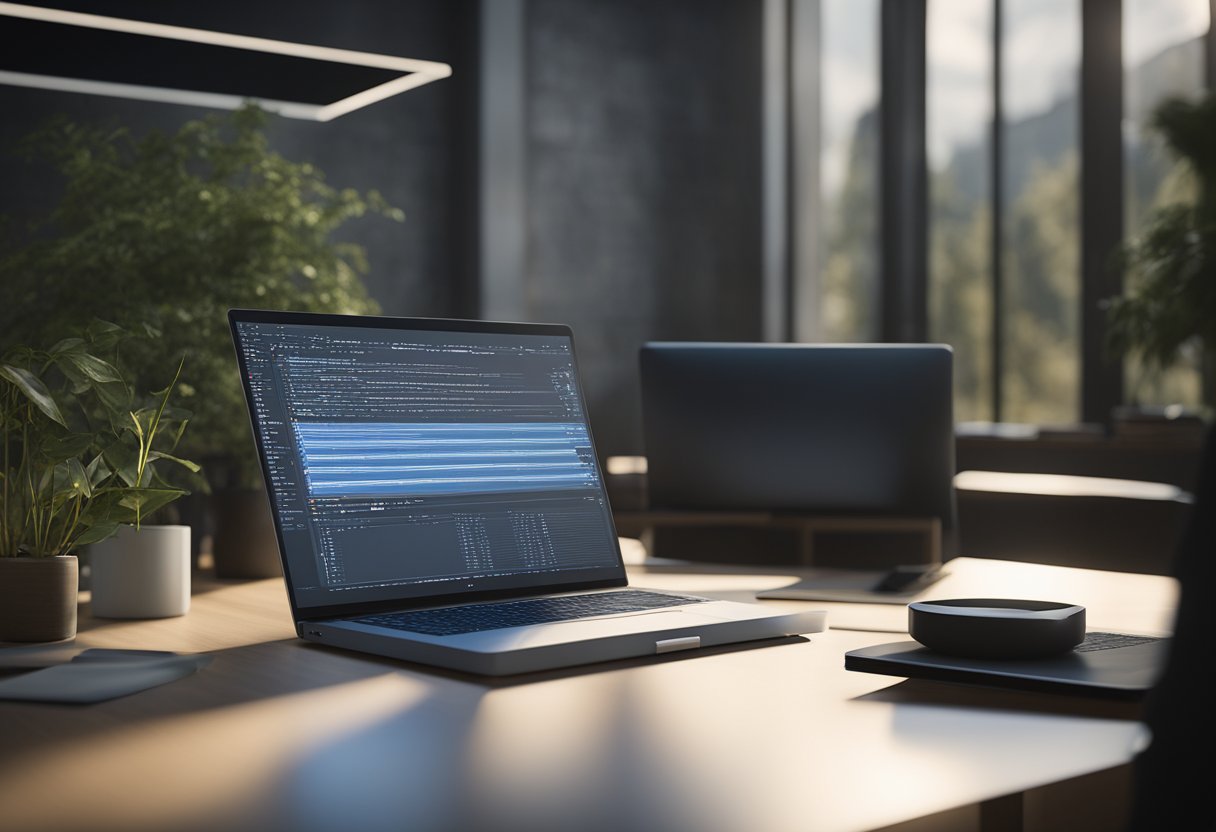
Enums are a powerful feature in many programming languages, including C++, that allow developers to define a set of named constants. In C++, enums are typically defined with integer values, but it is also possible to define enums with string values. This section provides an overview of how to define enums with string values in C++.
Syntax Overview
The syntax for defining an enum with string values in C++ is similar to that of a regular enum. The main difference is that the enum class keyword is used instead of just enum. Here is an example:
enum class Color {
RED,
GREEN,
BLUE
};
In this example, the Color enum is defined with three named constants, each with a string value. The underlying type of the enum is implicitly set to int by default, but it can be explicitly set to another integer type if needed.
Assigning String Values
To assign string values to enum constants, the constants must be defined with an initializer. In the example above, the initializer is the string literal “red”, “green”, and “blue”. It is important to note that the string values must be unique, just like the integer values in a regular enum.
Once an enum with string values is defined, the values can be accessed using the scope resolution operator (::). For example, to access the string value of the RED constant in the Color enum, you would use the following code:
std::string colorString = static_cast<std::underlying_type<Color>::type>(Color::RED);
In this example, the static_cast operator is used to convert the Color::RED constant to an integer value, which is then implicitly converted to a string value. The resulting string value is then assigned to the colorString variable.
In conclusion, defining enums with string values in C++ can be a useful technique for creating a set of named constants with string values. By following the syntax and guidelines outlined in this section, developers can easily define and use enums with string values in their C++ code.
Working with String Enums
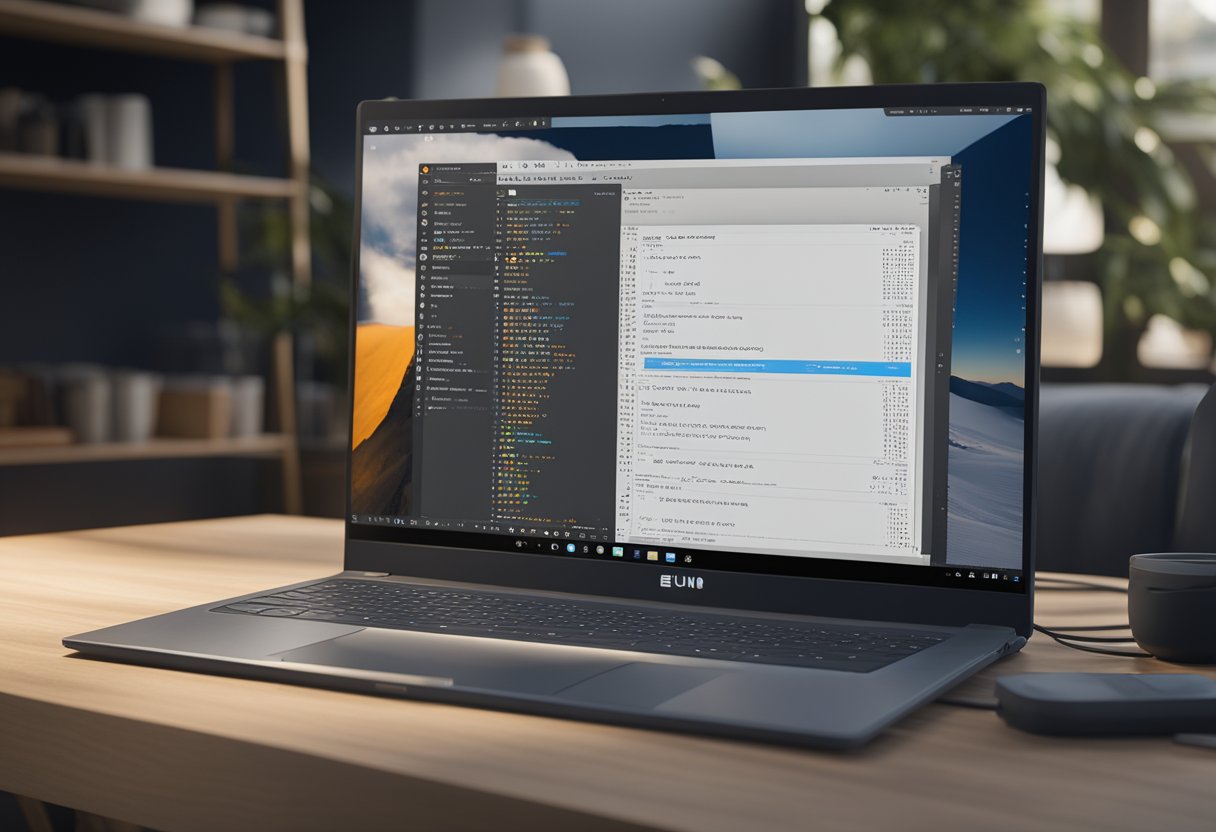
An enum is a user-defined type that consists of a set of named constants. In TypeScript, enums can be defined with string values. This allows developers to define enums with human-readable values, making the code more readable and easier to understand.
Accessing Enum Members
Accessing enum members is similar to accessing members of any other object in TypeScript. Enum members can be accessed using either the dot notation or the bracket notation. For example, consider the following enum:
enum Color {
Red = "RED",
Green = "GREEN",
Blue = "BLUE"
}
To access the Red
member of the Color
enum, you can use either of the following syntax:
Color.Red
Color["Red"]
Both of these syntaxes will return the string value "RED"
. Similarly, you can access the other members of the Color
enum using the dot or bracket notation.
Iterating Over Enums
Iterating over enums is a common task when working with TypeScript. TypeScript provides several ways to iterate over enums. One way is to use a for...in
loop. For example, consider the following enum:
enum Color {
Red = "RED",
Green = "GREEN",
Blue = "BLUE"
}
To iterate over the Color
enum using a for...in
loop, you can use the following syntax:
for (const key in Color) {
console.log(key, Color[key]);
}
This will output the following:
Red RED
Green GREEN
Blue BLUE
Another way to iterate over enums is to use the Object.keys()
method. For example:
Object.keys(Color).forEach(key => {
console.log(key, Color[key]);
});
This will output the same result as the for...in
loop.
In conclusion, defining an enum with string values in TypeScript is a powerful feature that can make the code more readable and easier to understand. Accessing enum members and iterating over enums are common tasks when working with enums, and TypeScript provides several ways to accomplish these tasks.
Best Practices
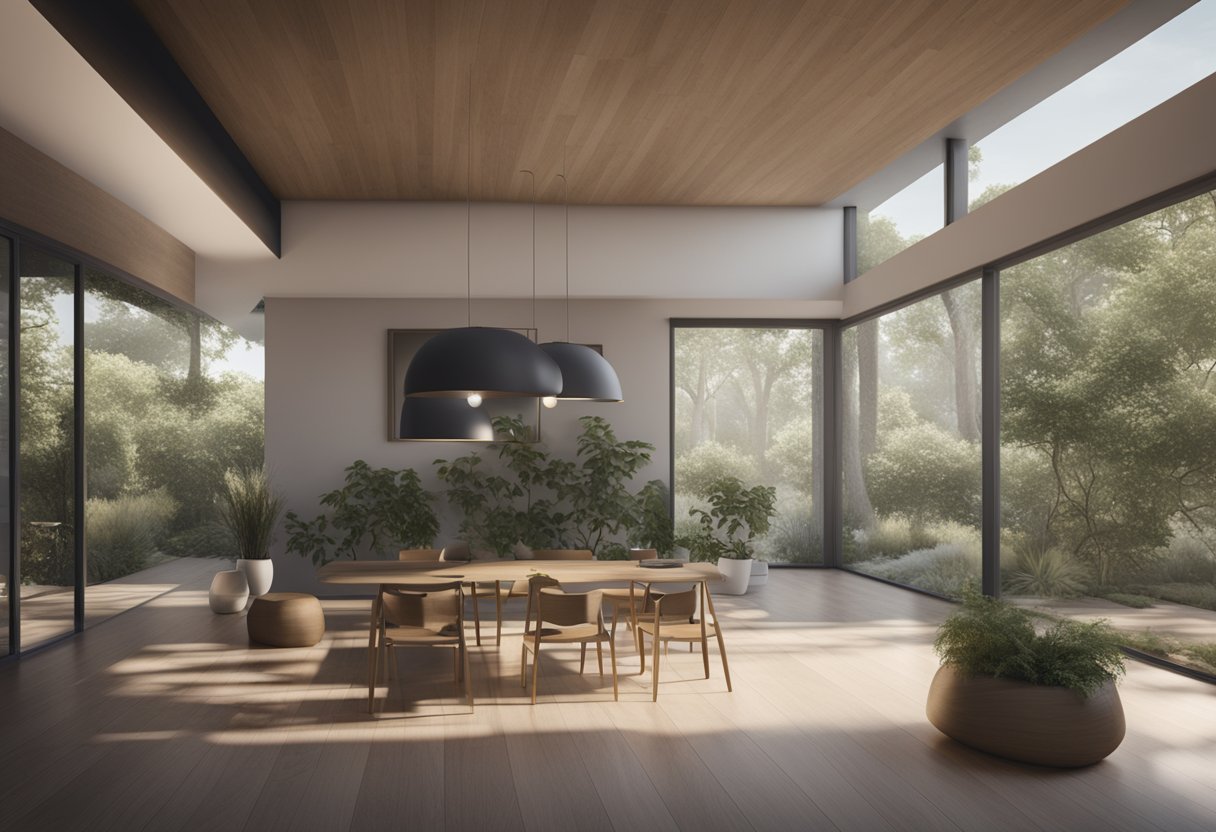
Defining an enum with string value can be a powerful tool in programming. However, it is important to follow some best practices to ensure that the enum is defined correctly and used effectively.
Naming Conventions
When defining an enum with string value, it is important to follow consistent naming conventions. This makes it easier to read and understand the code. The names of the enum values should be descriptive and follow a consistent format. For example, if defining an enum for colors, the values could be named “Red”, “Green”, and “Blue”.
When to Use String Enums
String enums are useful when you have a fixed set of values that are not expected to change frequently. They are also useful when you need to provide a user-friendly name for a value. For example, if you are defining an enum for error messages, the values could be named “InvalidInput”, “MissingParameter”, and “UnauthorizedAccess”. These values provide a clear and concise message to the user.
It is important to note that string enums should not be used for values that are expected to change frequently. If a value needs to be updated, it would require a code change, which can be time-consuming and error-prone. In such cases, it is better to use a configuration file or database to store the values.
Overall, defining an enum with string value can be a powerful tool in programming if used correctly. By following best practices and using string enums only when appropriate, developers can create more efficient and user-friendly code.
Advanced Topics
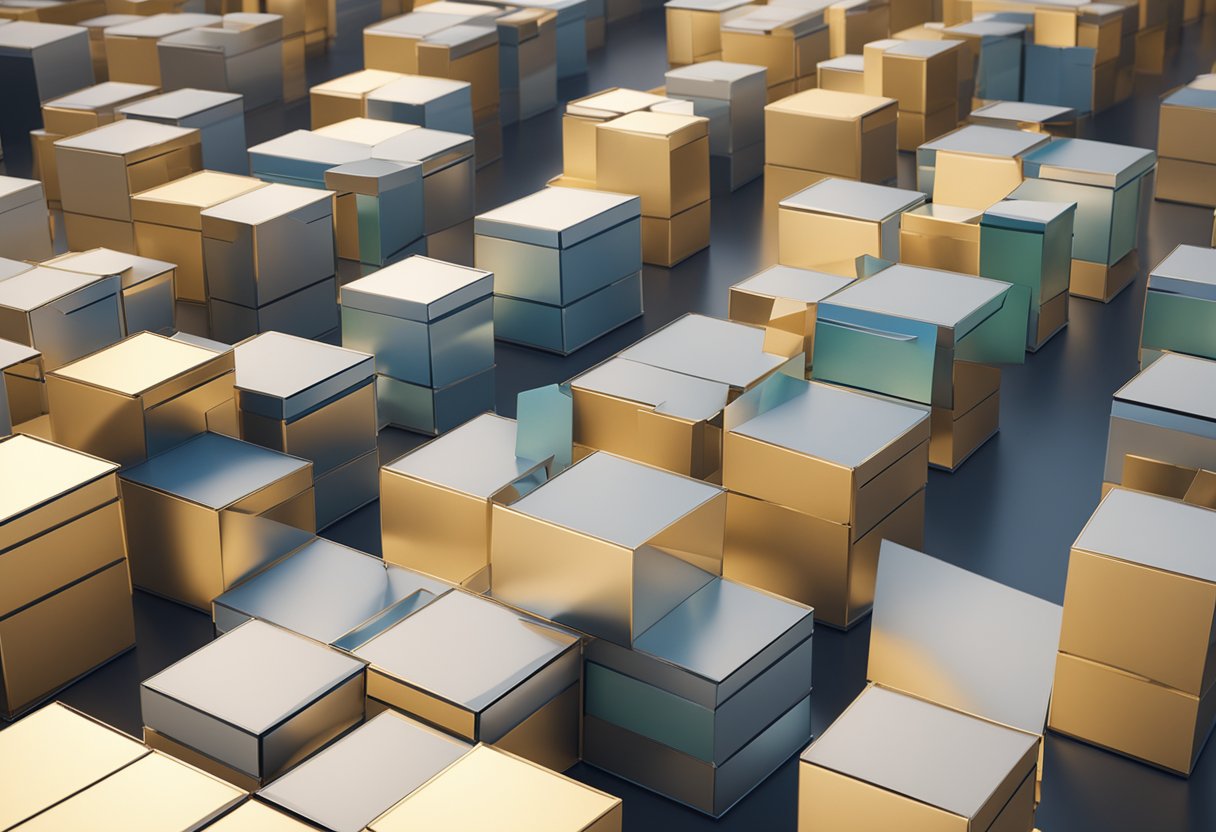
Enum Member Values
In C#, enums are indeed used to define a set of named constants. By default, the underlying type of an enum is int
, and the values of the constants are sequential integers starting from 0. However, it is not possible to define an enum with string values by default. Enum values are expected to be of the same underlying type, and explicitly specifying string values is not supported in the standard enum definition.
For example, the default enum declaration looks like this:
public enum MyEnum {
Value1,
Value2,
Value3
}
If you need string values, you might use attributes or other mechanisms to associate string values with enum members. Alternatively, starting from C# 7.3, you can use the Enum
class with the EnumMember
attribute in combination with a string
-based backing type:
public enum MyStringEnum : Enum {
[EnumMember(Value = "String1")]
Value1,
[EnumMember(Value = "String2")]
Value2,
[EnumMember(Value = "String3")]
Value3
}
This approach allows you to associate string values with enum members.
Frequently Asked Questions
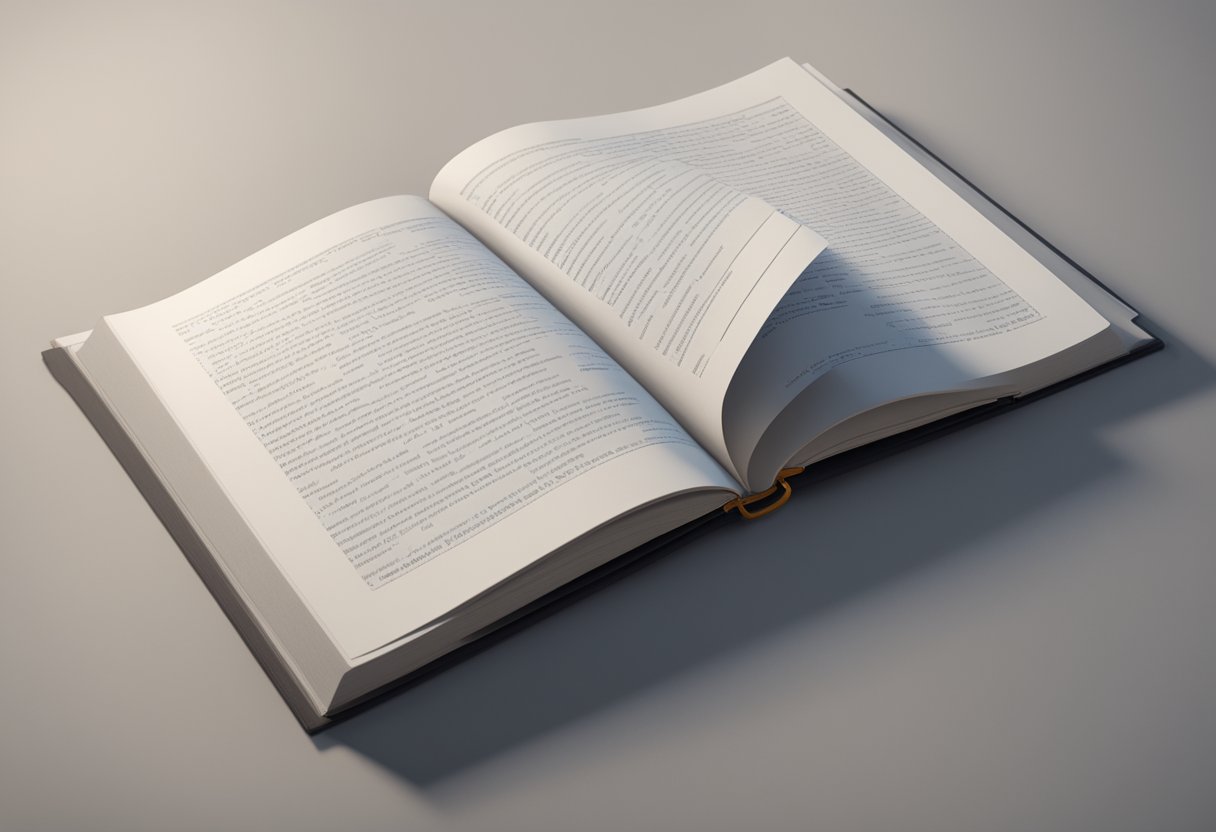
What is the syntax for defining an enum with string values in Java?
To define an enum with string values in Java, the syntax is as follows:
public enum EnumName {
ENUM_VALUE_1("string1"),
ENUM_VALUE_2("string2"),
ENUM_VALUE_3("string3");
private final String stringValue;
EnumName(String stringValue) {
this.stringValue = stringValue;
}
public String getStringValue() {
return stringValue;
}
}
How can I convert an enum to its string representation in C#?
To convert an enum to its string representation in C#, you can use the ToString()
method:
EnumName enumValue = EnumName.ENUM_VALUE_1;
string stringValue = enumValue.ToString();
Is it possible to associate multiple string values with a single enum entry in Java?
No, it is not possible to associate multiple string values with a single enum entry in Java. However, you can define multiple enum entries with the same string value:
public enum EnumName {
ENUM_VALUE_1("string1"),
ENUM_VALUE_2("string1"),
ENUM_VALUE_3("string2");
private final String stringValue;
EnumName(String stringValue) {
this.stringValue = stringValue;
}
public String getStringValue() {
return stringValue;
}
}
Can you create a Java enum that holds a list of string values?
No, you cannot create a Java enum that holds a list of string values. However, you can define multiple enum entries with the same string value, as shown in the previous example.
What is the best way to assign string values to enums in Java?
The best way to assign string values to enums in Java is to define a constructor for the enum that takes a string parameter, as shown in the first example.
How do you retrieve the string value from an enum in Java?
To retrieve the string value from an enum in Java, you can define a method in the enum that returns the string value:
EnumName enumValue = EnumName.ENUM_VALUE_1;
String stringValue = enumValue.getStringValue();
String Strip In Java: How to Remove Unwanted Characters in Java Strings