Char values in Java are an essential aspect of programming in this language. They are used to store individual characters, such as letters, digits, and symbols. A char value is represented by a single 16-bit Unicode character, which means that it can contain any Unicode character, including those that require more than one byte to represent.
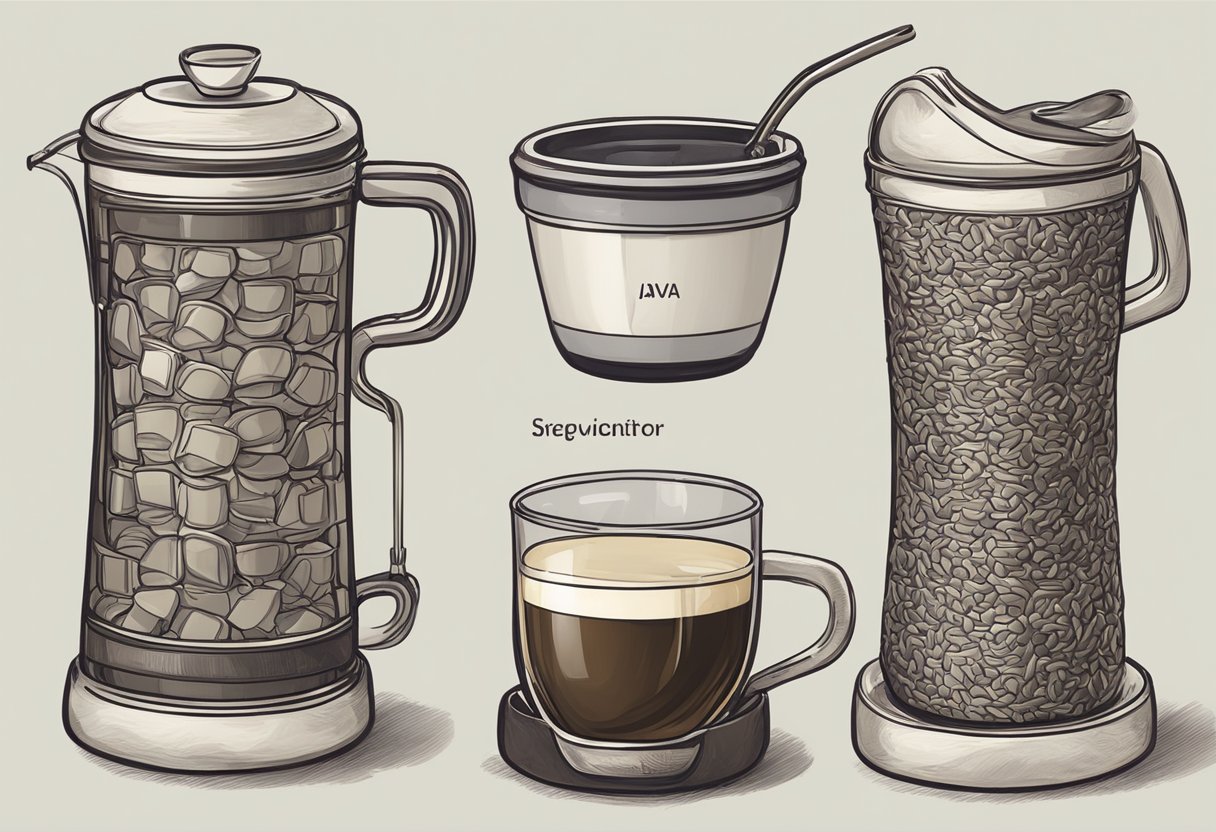
In Java, a char value must be enclosed in single quotes, such as ‘a’, ‘5’, or ‘$’. It is important to note that a char value is not the same as a String value, which is a sequence of characters enclosed in double quotes. While a String value can contain any number of characters, a char value can only store a single character.
String Strip In Java: How to Remove Unwanted Characters in Java Strings
Understanding how to work with char values is crucial for any Java programmer. In this article, we will explore the basics of char values, including how to declare and initialize them, how to convert them to other data types, and how to perform operations on them. We will also look at some common use cases for char values in Java programming. By the end of this article, you will have a solid understanding of char values and how to use them effectively in your Java programs.
Understanding Char in Java

In Java, the char
data type is used to represent a single character. It is a 16-bit unsigned integer with a range of 0 to 65,535.
A char
value can be represented using a single character enclosed in single quotes, such as 'a'
, 'b'
, or '1'
. It can also be represented using its Unicode value, which is a 4-digit hexadecimal number preceded by the escape sequence \u
, such as '\u0041'
for the uppercase letter A.
It is important to note that a char
is not the same as a String
in Java. A String
is a sequence of characters, while a char
represents a single character.
When comparing char
values, it is important to use single quotes, such as if (myChar == 'a')
, instead of double quotes, which are used for String
values.
Here are some other important things to keep in mind when working with char
values in Java:
- A
char
can be used in arithmetic operations, but it will be converted to an integer first. - The
Character
class in Java provides many useful methods for working withchar
values, such asisLetter
,isDigit
, andtoUpperCase
. - Unicode characters beyond the Basic Multilingual Plane (BMP) require two
char
values to represent them, known as a surrogate pair.
Overall, understanding the char
data type in Java is essential for working with individual characters in your programs.
Char Data Type

In Java, the char
data type represents a single character. A character can be a letter, digit, symbol, or whitespace. The char
data type is a primitive data type, meaning it is not an object and does not have any methods.
The size of a char
in Java is 16 bits, which allows it to represent a wide range of characters. The range of char
values is between 0 and 65,535. This range includes the standard ASCII characters, which range from 0 to 127.
To declare a char
variable in Java, use the keyword char
followed by the variable name. For example:
char firstInitial = 'J';
In this example, we declare a char
variable named firstInitial
and assign it the value of the character ‘J’.
You can also assign a char
variable the value of a Unicode character using the escape sequence \u
followed by the Unicode value in hexadecimal. For example:
char euroSymbol = '\u20AC';
In this example, we declare a char
variable named euroSymbol
and assign it the value of the Euro symbol, which has a Unicode value of 20AC
in hexadecimal.
One important thing to note is that char
values are enclosed in single quotes ('
) instead of double quotes ("
), which are used for String
values. This is because char
values represent a single character, while String
values represent a sequence of characters.
Overall, the char
data type in Java is a simple but important data type that allows us to represent individual characters in our programs.
Char Literals

In Java, a char literal represents a single character, enclosed within single quotes. For example, the char literal ‘A’ represents the uppercase letter A.
We can also use Unicode escape sequences to represent characters that cannot be typed on the keyboard. For example, the char literal ‘\u0058’ represents the uppercase letter X, because the Unicode code point for X is 0058.
It is important to note that a char literal is actually an integer type. It stores the 16-bit Unicode integer value of the character in question. You can look at something like ASCII Table to see the different values for different characters.
Here are some examples of valid char literals in Java:
- ‘A’
- ‘B’
- ‘\n’ (newline character)
- ‘\u00A9’ (copyright symbol)
- ‘\u2605’ (star symbol)
It is also possible to use escape sequences to represent certain special characters. Here are some commonly used escape sequences:
- ‘\n’ – newline
- ‘\t’ – tab
- ‘\b’ – backspace
- ‘\r’ – carriage return
- ”’ – single quote
- ‘”‘ – double quote
- ‘\’ – backslash
In summary, char literals in Java are used to represent single characters and are enclosed within single quotes. They are actually an integer type that stores the 16-bit Unicode integer value of the character. We can use Unicode escape sequences or escape sequences to represent characters that cannot be typed on the keyboard or certain special characters.
Char Values and Unicode
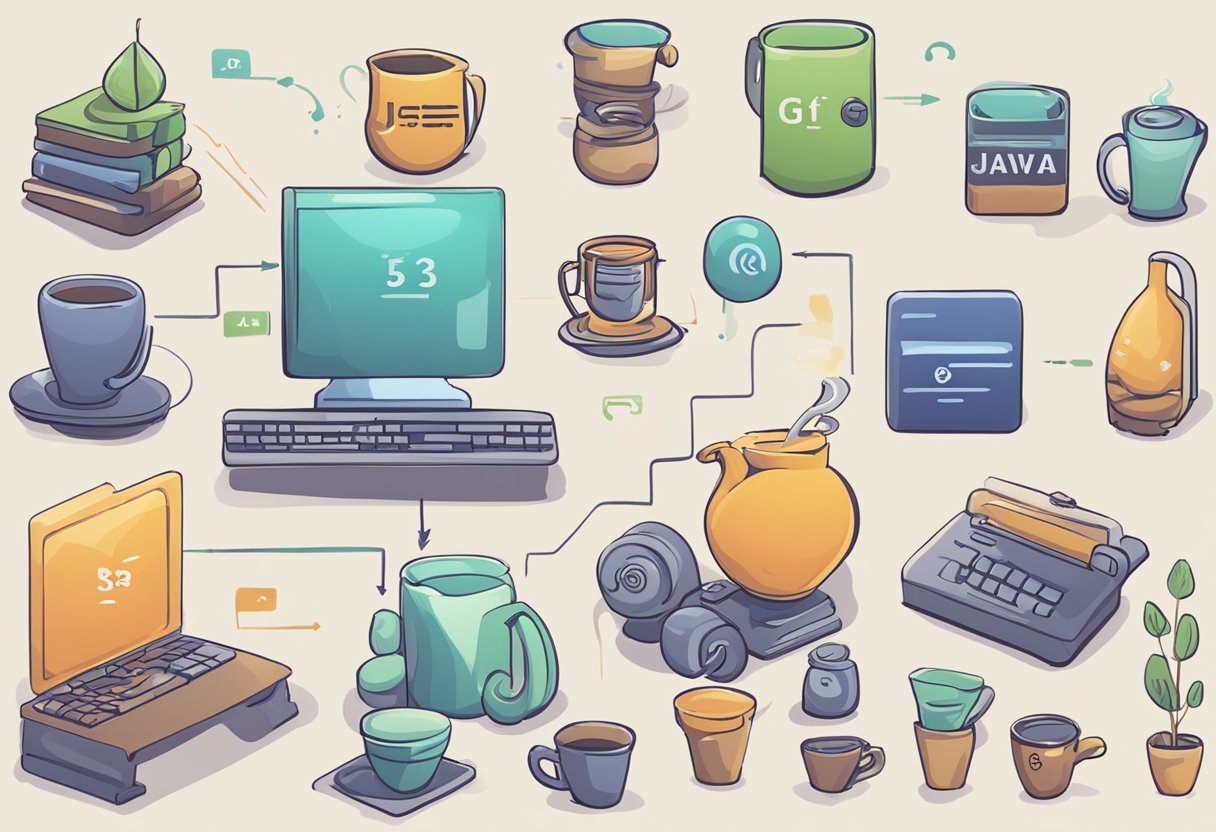
In Java, the char
data type represents a single 16-bit Unicode character. Unicode is a character encoding standard that assigns a unique code point to every character in every language. This means that every character, including letters, numbers, punctuation marks, and special characters, has a unique code point that can be represented by a char
value in Java.
The Unicode standard supports over 140,000 characters, including characters from all major languages and scripts. This means that the char
data type can represent most characters that you might encounter in your Java programs. However, there are some characters that cannot be represented by a single char
value because they require more than 16 bits to encode.
To represent characters that require more than 16 bits, Java uses a surrogate pair of char
values. A surrogate pair is a pair of char
values that together represent a single Unicode code point. The first char
value in the pair is called the high surrogate, and the second char
value is called the low surrogate.
To determine whether a char
value represents a high surrogate or a low surrogate, you can use the Character.isHighSurrogate(char ch)
and Character.isLowSurrogate(char ch)
methods, respectively. To convert a surrogate pair to a Unicode code point, you can use the Character.toCodePoint(char high, char low)
method.
In summary, the char
data type in Java represents a single 16-bit Unicode character, and can be used to represent most characters in most languages and scripts. For characters that require more than 16 bits, Java uses a surrogate pair of char
values.
Char Values and ASCII
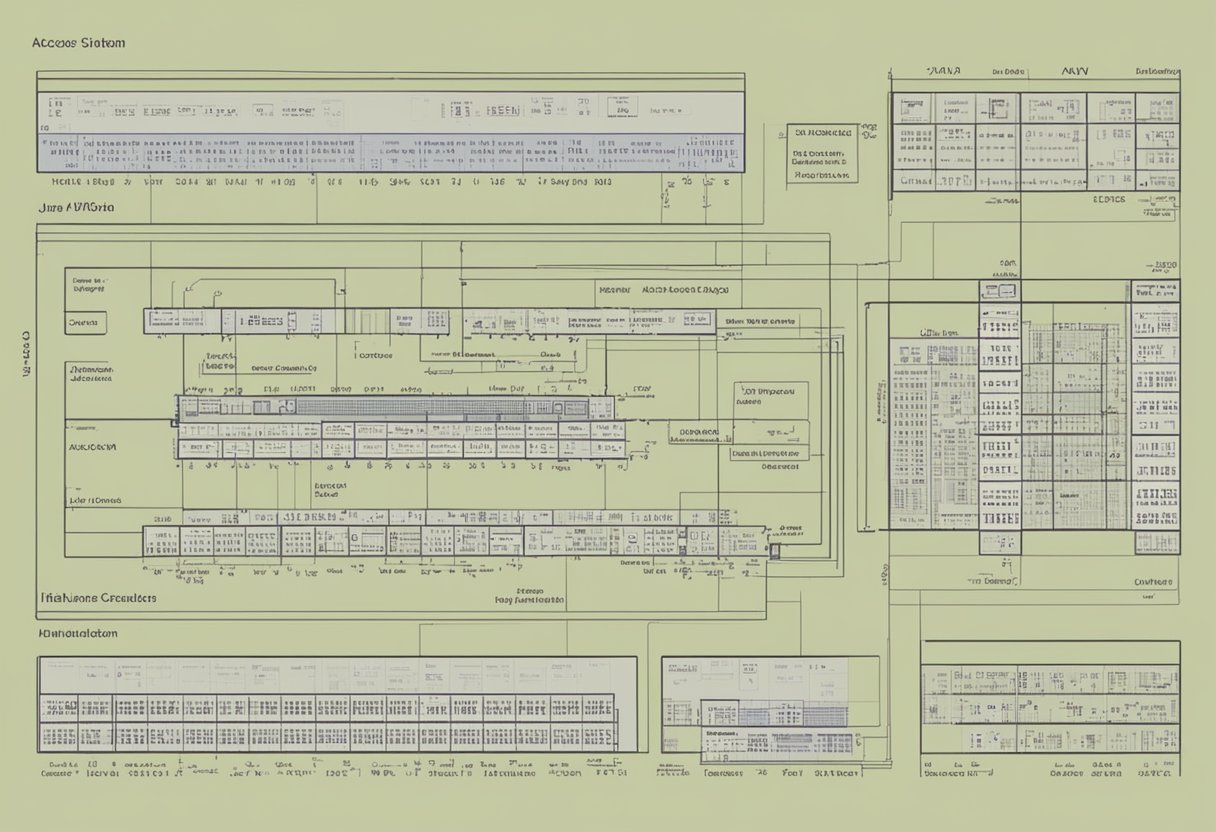
In Java, a char
data type represents a single character. It is a primitive data type that can hold a 16-bit Unicode character. A char
value is enclosed in single quotes, like this: 'a'
.
ASCII (American Standard Code for Information Interchange) is a character encoding standard that assigns a unique number to each character. ASCII is a subset of Unicode and defines codes for 128 characters, including letters, digits, punctuation marks, and control characters. The ASCII values for uppercase letters range from 65 to 90, and for lowercase letters, they range from 97 to 122.
To get the ASCII value of a char
in Java, you can simply cast the char
to an int
using the (int)
operator. For example, to get the ASCII value of the character 'a'
, you can do the following:
char c = 'a';
int ascii = (int) c;
System.out.println(ascii); // Output: 97
You can also use the Character.getNumericValue()
method to get the ASCII value of a char
. This method returns the numeric value of the character, which is equivalent to its ASCII value for ASCII characters. For example:
char c = 'a';
int ascii = Character.getNumericValue(c);
System.out.println(ascii); // Output: 10
Note that the Character.getNumericValue()
method returns -1 for non-ASCII characters.
In Java, you can also use escape sequences to represent non-printable ASCII characters, such as the newline character ('\n'
) and the tab character ('\t'
). For example:
char newline = '\n';
char tab = '\t';
In conclusion, understanding char
values and ASCII is important when working with Java programs that deal with characters and strings. By knowing how to get the ASCII value of a char
, you can perform various operations on characters and manipulate strings in Java.
Char Methods in Java
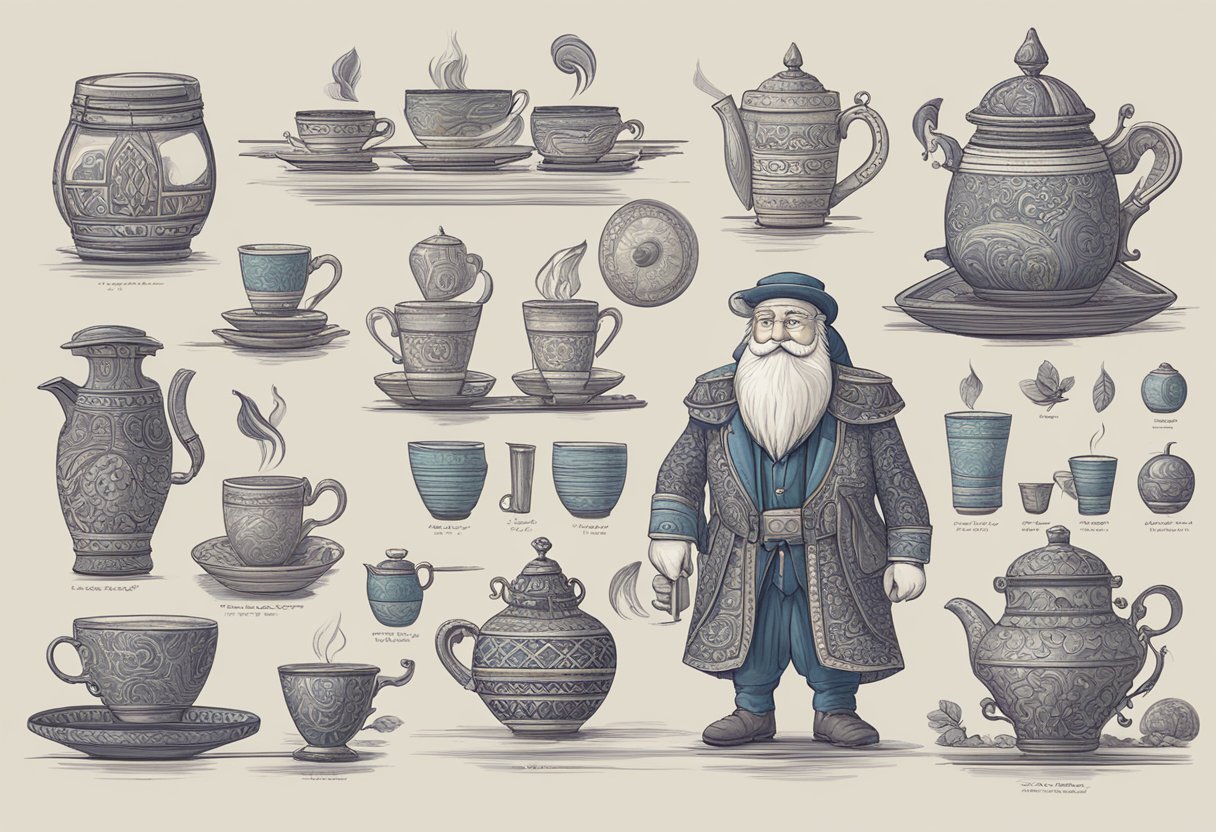
In Java, the char
data type is a primitive data type that represents a single character. The Character
class in Java provides several methods to manipulate char
values. We will discuss some of the most commonly used methods below.
CharAt Method
The charAt
method returns the character at a specified index in a string. It takes an integer argument that represents the index of the character to be returned. For example, the following code snippet returns the character ‘o’ from the string “Hello World!”.
String str = "Hello World!";
char ch = str.charAt(4);
IsDigit Method
The isDigit
method returns true
if a character is a digit (0-9), and false
otherwise. For example, the following code snippet checks if the character ‘5’ is a digit.
char ch = '5';
boolean isDigit = Character.isDigit(ch);
IsLetter Method
The isLetter
method returns true
if a character is a letter (a-z or A-Z), and false
otherwise. For example, the following code snippet checks if the character ‘A’ is a letter.
char ch = 'A';
boolean isLetter = Character.isLetter(ch);
IsUpperCase Method
The isUpperCase
method returns true
if a character is an uppercase letter (A-Z), and false
otherwise. For example, the following code snippet checks if the character ‘B’ is an uppercase letter.
char ch = 'B';
boolean isUpperCase = Character.isUpperCase(ch);
IsLowerCase Method
The isLowerCase
method returns true
if a character is a lowercase letter (a-z), and false
otherwise. For example, the following code snippet checks if the character ‘c’ is a lowercase letter.
char ch = 'c';
boolean isLowerCase = Character.isLowerCase(ch);
These are some of the most commonly used methods provided by the Character
class in Java to manipulate char
values.
Type Casting with Char

In Java, type casting is the process of converting a value of one data type to another data type. The char
data type is a primitive data type that represents a single character. When it comes to type casting with char
, there are a few things to keep in mind.
Widening Casting with Char
Widening casting is the process of converting a smaller data type to a larger data type. In Java, widening casting with char
is done automatically when converting a char
to an int
or a long
. This is because char
is a 16-bit unsigned integer type, and int
and long
are both signed integer types that are larger than char
.
Narrowing Casting with Char
Narrowing casting is the process of converting a larger data type to a smaller data type. In Java, narrowing casting with char
is done manually when converting an int
or a long
to a char
. This is because char
is a 16-bit unsigned integer type, and int
and long
are both signed integer types that are larger than char
.
When narrowing casting with char
, it is important to keep in mind that the resulting char
value may not represent a valid character. This is because char
can only represent Unicode characters in the range of 0 to 65535. If the int
or long
value being converted is outside of this range, the resulting char
value may be an invalid character or a control character.
Example
Here’s an example of type casting with char
:
char c = 'A';
int i = c; // Widening casting, automatically done
System.out.println(i); // Output: 65
int j = 66;
char d = (char) j; // Narrowing casting, manually done
System.out.println(d); // Output: B
In this example, we first assign the char
value 'A'
to the variable c
. We then assign the value of c
to the variable i
using widening casting. The resulting value of i
is the Unicode value of the character 'A'
, which is 65.
We then assign the int
value 66
to the variable j
. We then assign the value of j
to the variable d
using narrowing casting. The resulting value of d
is the character represented by the Unicode value of j
, which is 'B'
.
In conclusion, type casting with char
is an important concept to understand in Java. By keeping in mind the differences between widening and narrowing casting, we can ensure that our code is accurate and efficient.
Char Array in Java
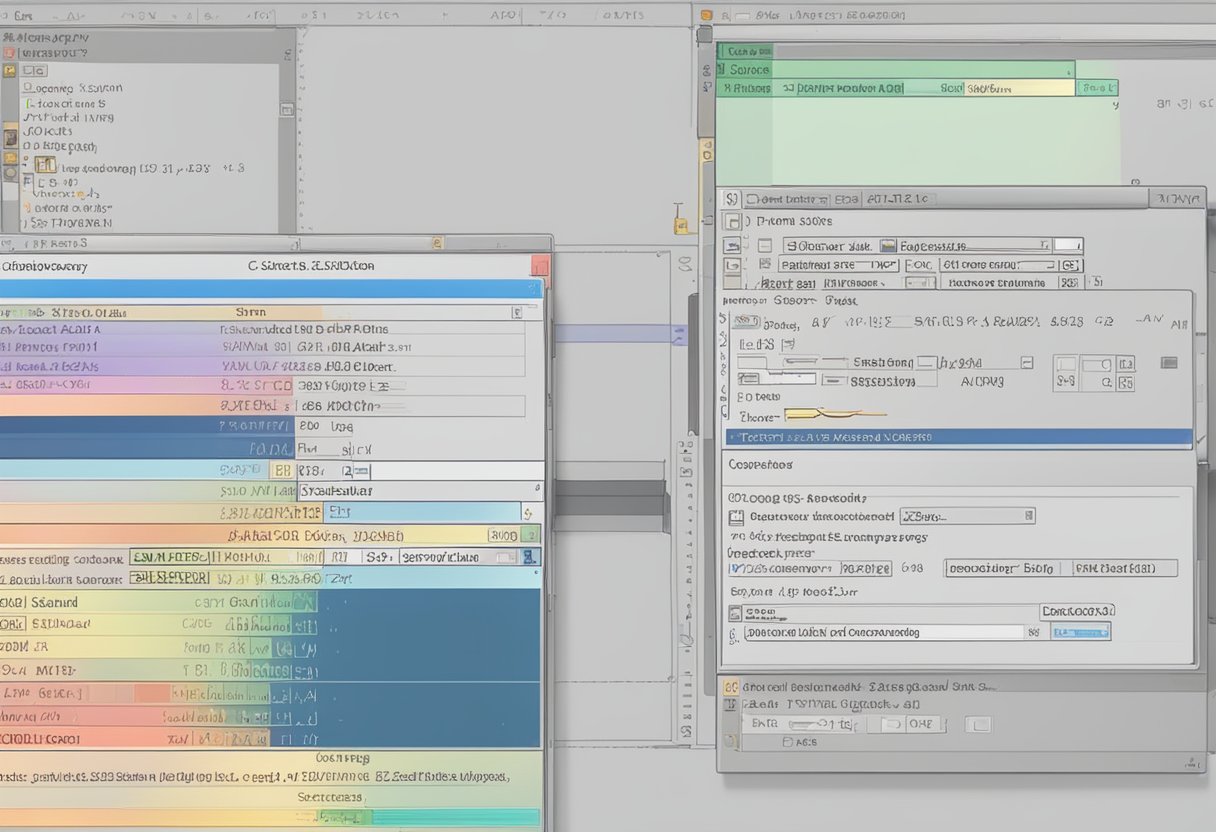
In Java, a character array is a data structure that stores a sequence of characters. Each character is stored in contiguous memory locations and can be accessed by its index, similar to an array of integers or any other data type.
To declare a character array in Java, we use the following syntax:
char[] charArray;
We can also declare and initialize a character array in a single line of code, like this:
char[] charArray = {'a', 'b', 'c', 'd', 'e'};
In Java, a character array is different from a string array, and neither a string nor a character array can be terminated by the NUL character. The Java language uses UTF-16 representation in a character array, string, and StringBuffer classes.
We can perform various operations on a character array, such as concatenation, comparison, and sorting. We can also use the String
class’s toCharArray()
method to convert a string into a character array.
Overall, a character array is a useful data structure in Java for handling character data type values.
Char and String Comparison in Java

In Java, char
and String
are two different data types. A char
is a single character, while a String
is a sequence of characters. When comparing char
and String
values in Java, it is important to understand the differences between these two data types.
Comparing Char Values
To compare char
values in Java, you can use the relational operators such as <
, >
, <=
, >=
, ==
, and !=
. These operators compare the ASCII values of the characters. For example, the ASCII value of the character ‘A’ is 65, while the ASCII value of the character ‘B’ is 66. Therefore, the expression 'A' < 'B'
is true.
Comparing String Values
To compare String
values in Java, you should use the equals()
method. The equals()
method compares the contents of two String
objects, not just their references. For example, the expressions "hello".equals("hello")
and "hello" == "hello"
both return true
.
It is important to note that the ==
operator compares the references of two objects, not their contents. Therefore, the expressions "hello" == new String("hello")
and "hello".equals(new String("hello"))
return false
and true
, respectively.
Converting Char to String
To convert a char
value to a String
object, you can use the Character.toString()
method or concatenate the char
value with an empty String
. For example, Character.toString('A')
and 'A' + ""
both return the String
“A”.
Conclusion
In summary, when comparing char
and String
values in Java, you should use the appropriate methods and operators for each data type. Use the relational operators to compare char
values, and use the equals()
method to compare String
values. Remember that the ==
operator compares object references, not contents.
Conclusion

In conclusion, Char values are an important data type in Java that represent single character values. They can be used to store and manipulate characters such as letters, digits, and symbols.
Throughout this article, we have explored various operations that can be performed on Char values using methods such as isLetter()
, isDigit()
, and toUpperCase()
. We have also discussed how to compare Char values using relational operators and the Character.compare()
method.
It is important to note that Char values are not the same as String values, which represent a sequence of characters. Char values can only store one character at a time, while String values can store multiple characters.
In Java, Char values are represented using Unicode encoding, which allows for the representation of characters from different languages and scripts. This means that developers can use Char values to work with text in different languages and scripts.
Overall, Char values are a fundamental part of the Java programming language, and understanding how to work with them is essential for any Java developer.
Frequently Asked Questions

What is the default value of a char in Java?
The default value of a char in Java is ‘\u0000’. It is a null character.
How do you assign a value to a char in Java?
You can assign a value to a char in Java by using single quotes. For example, char myChar = ‘A’; assigns the value ‘A’ to the variable myChar.
What is the range of char values in Java?
The range of char values in Java is from ‘\u0000’ to ‘\uffff’. It means that a char can hold any Unicode character.
What is the ASCII value of ‘A’ to ‘Z’ in Java?
The ASCII value of ‘A’ to ‘Z’ in Java is from 65 to 90. ‘A’ has the ASCII value of 65, ‘B’ has 66, and so on until ‘Z’ which has the ASCII value of 90.
What is the ASCII value of special characters in Java?
The ASCII value of special characters in Java depends on the character. For example, the ASCII value of ‘@’ is 64, ‘#’ is 35, and ‘$’ is 36.
How do you find the ASCII value of a character in Java?
To find the ASCII value of a character in Java, you can use the type casting. For example, int asciiValue = (int) ‘A’; assigns the ASCII value of ‘A’ to the variable asciiValue.