Java’s Files.copy method, a key component of the java.nio.file package, presents a straightforward solution for copying files and directories. This method has streamlined a formerly complex process, making file management more accessible for developers. The following article offers a detailed exploration of how to leverage this capability effectively, emphasizing the method’s flexibility, options for handling file attributes and links, and strategies for robust exception management. As we unpack the layers of Files.copy, the focus remains on practical guidance, aimed at enhancing your programming toolkit with efficient file management techniques.
Understanding the Files.copy Method
Understanding the Files.copy Method in Java
Java’s Files.copy method is a tool designed to simplify the process of copying files and directories in Java. It’s part of the java.nio.file package, a more recent addition to Java designed to handle file operations more efficiently than the older java.io package. The Files.copy method boasts versatility, allowing for the duplication of content from one file to another, or even to a directory, with relative ease. Here’s a breakdown of how it works, geared towards those who have a basic grasp of Java programming.
Usage Basics:
At its core, the Files.copy method requires two primary arguments: the source and the destination. The source is the file or directory you want to copy, while the destination is where you want the copy to be placed. The method follows this simple syntax:
Files.copy(Path source, Path target, CopyOption... options)
– Path source refers to the location of the original file or directory.
– Path target specifies where the copy will go.
– CopyOption… options is an optional parameter that allows you to specify how the copying should be handled, such as overwriting existing files or copying attributes.
Step-by-Step Instructions:
- Setup Your Environment: First, ensure your Java development environment is ready and that you’ve imported the necessary package with the line
import java.nio.file.*;
. - Define Your Source and Destination: Utilize the Paths.get method to define the source and destination paths. For example,
Path sourcePath = Paths.get("/path/to/source"); Path destinationPath = Paths.get("/path/to/destination");
- Execute the Copy: Use the Files.copy method to execute the copy operation. Without additional options, the basic command looks like this:
Files.copy(sourcePath, destinationPath);
- Handle Exceptions: Since file operations can fail due to various reasons (like file not found or access denied), wrap your code block in a try-catch statement to manage potential IOExceptions.
try { Files.copy(sourcePath, destinationPath); } catch (IOException e) { e.printStackTrace(); }
- (Optional) Use Copy Options: If you want to overwrite an existing file at the destination or copy attributes, include the appropriate CopyOption parameters. For overwriting an existing file, the method call would look like this:
Files.copy(sourcePath, destinationPath, StandardCopyOption.REPLACE_EXISTING);
Remember, the Files.copy method is powerful but requires careful handling, especially when working with existing files, to avoid unintentional data loss. Always double-check your source and destination paths and test your code in a safe environment before running it on important files.
This method streamlines what used to be a complex task into a few lines of code, emphasizing Java’s ongoing development towards more efficient file handling. Whether you’re working on a large-scale project or just managing local files, understanding the Files.copy method is a valuable skill in any Java programmer’s toolkit.
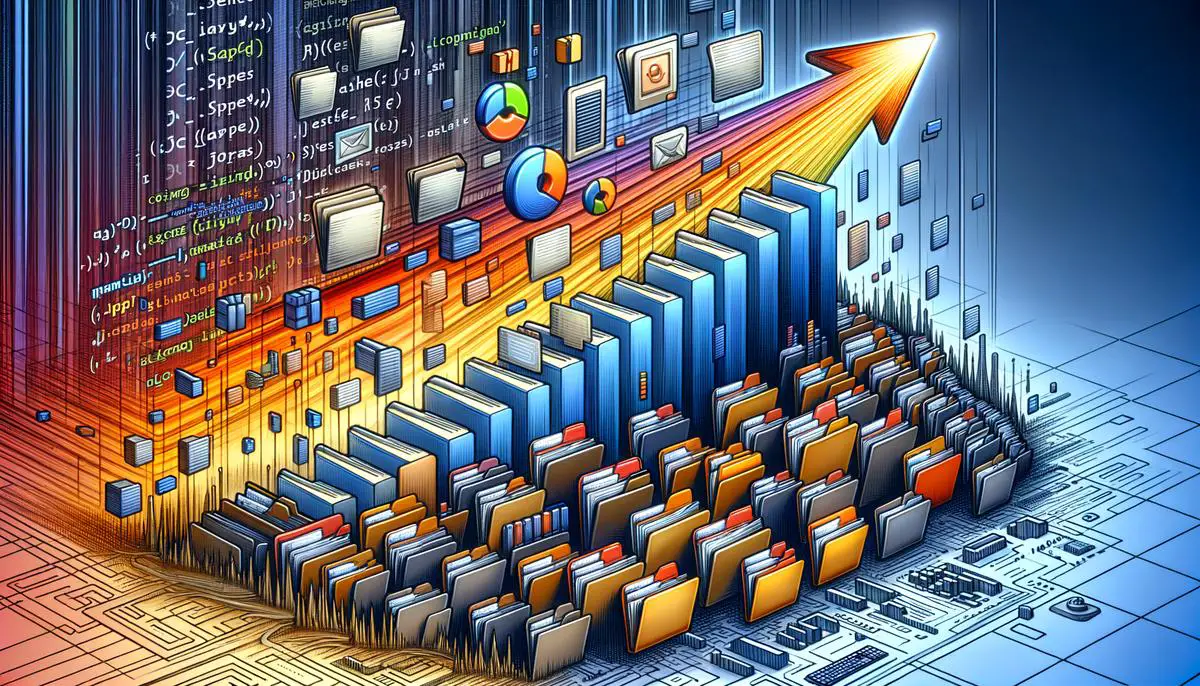
Exploring Copy Options
How Do Copy Options Enhance the Functionality of Files.copy in Java?
Beyond the basic file copying capabilities of the Files.copy
method in Java, the real magic happens with the inclusion of CopyOption
parameters. These options significantly broaden the utility and safety of file operations. Today, we’ll delve into how these options elevate the method’s functionality, focusing on nuances that offer you control and precision in your file management tasks.
Preserving File Attributes with COPY_ATTRIBUTES
One standout feature is the COPY_ATTRIBUTES
option. When you’re transferring files, especially in a backup or archival scenario, maintaining the original file’s attributes is crucial. By using this option, Files.copy
doesn’t just copy the file data; it ensures that attributes like the creation time, modification time, and file permissions are replicated in the destination file. This feature is particularly useful when maintaining the integrity and metadata of files is essential for your application.
Atomic Move with ATOMIC_MOVE
The ATOMIC_MOVE
option introduces an extra layer of reliability, especially in environments where file integrity during transfer is non-negotiable. This option ensures that the file move operation is atomic, meaning it either completes successfully without partial transfers, or it fails entirely, leaving your files in their original, unaltered state. It’s a safeguard against data corruption during critical file operations, especially useful in system-level or transactional applications where consistency is key.
Link Handling with NOFOLLOW_LINKS
When dealing with symbolic links, the NOFOLLOW_LINKS
option offers a pathway to control. This option tells Files.copy
to treat the symbolic link itself as the target rather than the file it points to. It’s essential for applications that need to copy the link structure of a file system without disturbing the actual linked files. This control over link handling is invaluable for complex file management tasks, ensuring that links remain intact and operational after copying.
Concluding Thoughts
Incorporating CopyOption
parameters into your use of Java’s Files.copy
method significantly upgrades your file management capabilities. Whether you need to ensure attribute preservation with COPY_ATTRIBUTES
, guarantee atomicity with ATOMIC_MOVE
, or manage symbolic links with NOFOLLOW_LINKS
, these options offer precision and control. Java’s file API, through these nuanced options, provides a robust toolkit for handling your file operations with the care and specificity they require.
Embracing these enhancements not only streamlines your development workflow but also empowers your applications to handle files with the sophistication and safety modern software demands. With this understanding, you’re well-equipped to leverage Files.copy
to its fullest, tailoring file operations to your precise needs and the demands of your projects.
Remember, powerful tools like these are at your disposal for a reason. Using them thoughtfully will elevate your file management strategies, ensuring your applications are not just functional but resilient and reliable.
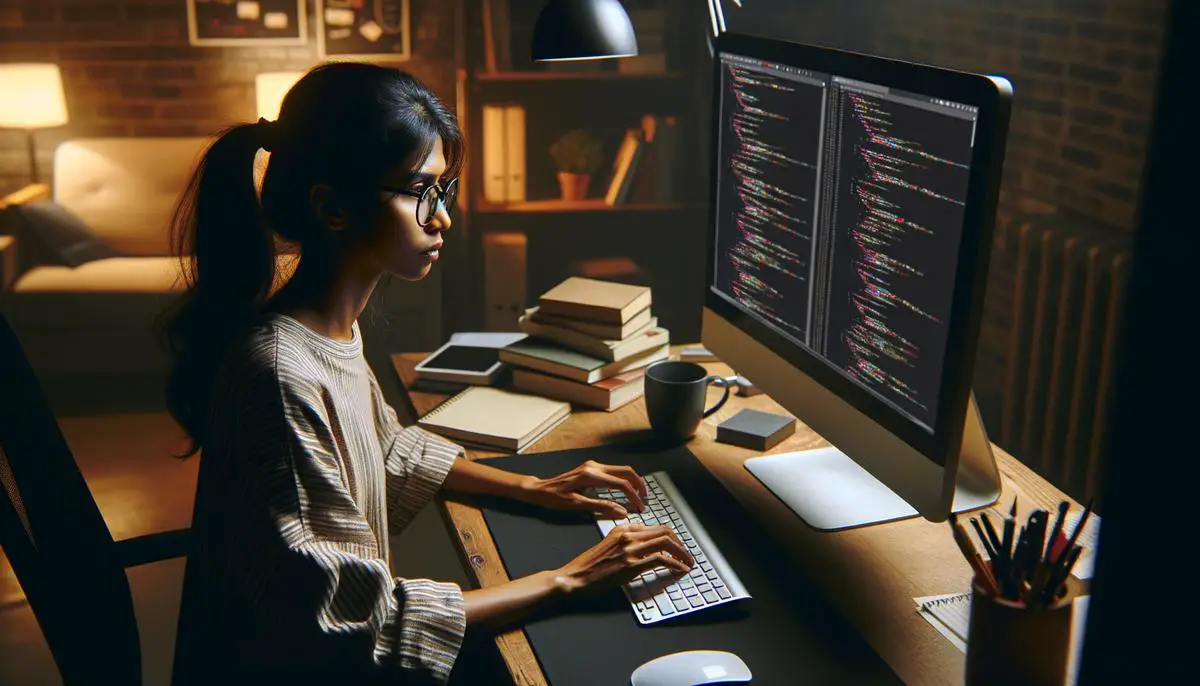
Exception Handling and Best Practices
Moving forward, diving into exception handling for Files.copy
is crucial for robust file management in Java. Exception handling ensures your program remains stable and responsive, even when faced with errors during file operations. Here’s how to master exception handling with Files.copy
effectively, mirroring the tone and style previously set.
1. Understand Common Exceptions
Two primary exceptions can arise when using Files.copy
:
- IOException: This is thrown when an I/O error occurs during file copying. Causes can range from the source file not existing, to lack of access permissions, or issues with the storage device.
- FileAlreadyExistsException: Specifically thrown if the target file exists but is not set to be replaced due to the absence of the
REPLACE_EXISTING
CopyOption.
2. Implement Try-Catch Blocks
To manage these exceptions, wrap your Files.copy
call inside a try-catch
block. This enables your program to catch and handle exceptions gracefully, preventing it from crashing.
try {
Files.copy(sourcePath, destinationPath, StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
System.out.println("An error occurred during file copy: " + e.getMessage());
}
3. Handle Specific Exceptions
For more granular control, you can catch and handle different types of exceptions separately. This is particularly useful for providing specific feedback or taking alternative actions depending on the error.
try {
Files.copy(sourcePath, destinationPath);
} catch (FileAlreadyExistsException e) {
System.out.println("The destination file already exists.");
} catch (IOException e) {
System.out.println("A general I/O error occurred: " + e.getMessage());
}
4. Utilize Finally Block
Optionally, a finally
block can be used to execute cleanup code or other operations that must occur after the try
block completes, regardless of whether an exception was caught.
try {
Files.copy(sourcePath, destinationPath);
} catch (IOException e) {
System.out.println("Error copying file: " + e.getMessage());
} finally {
System.out.println("Operation complete.");
}
5. Employ Best Practices
It’s important to:
- Validate file paths before attempting operations.
- Check file permissions to avoid unnecessary exceptions.
- Use specific catch blocks for precise exception handling.
- Employ the
finally
block for cleanup tasks to ensure the integrity of your application’s operations.
By adhering to these recommended practices for exception handling with Files.copy
, you ensure that your file operations are not only effective but also resilient to errors. Implementing robust exception handling is key to maintaining the stability and reliability of your Java applications, providing a seamless experience for the end-users.
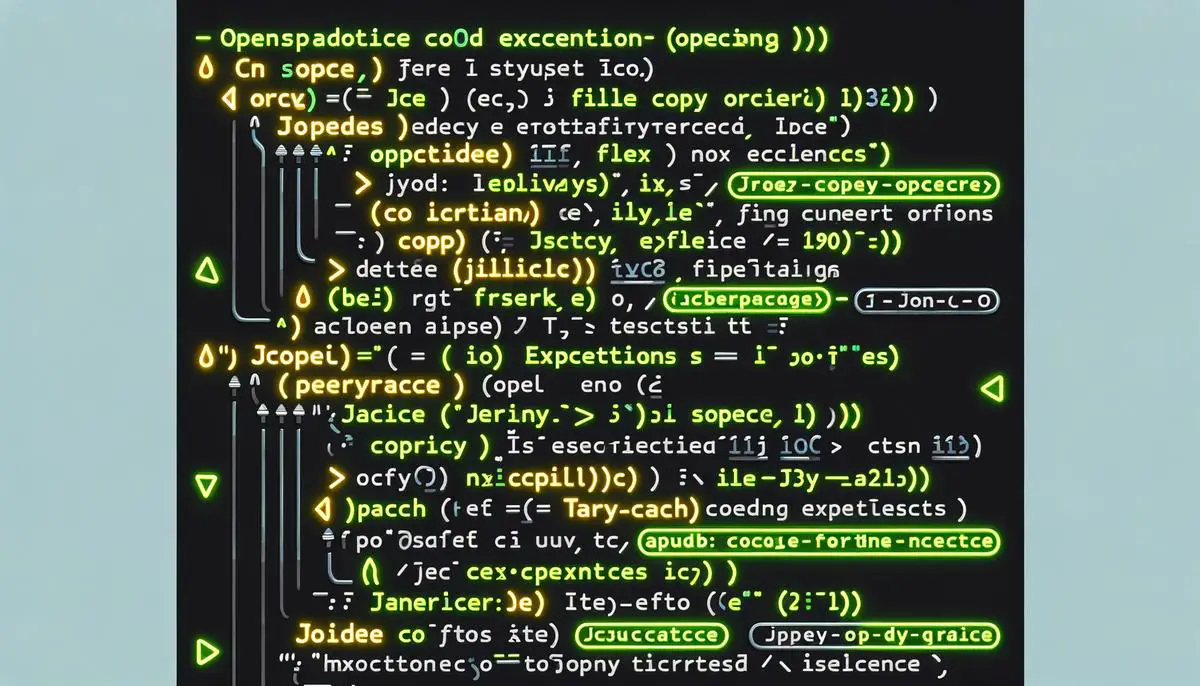
The Files.copy method in Java encapsulates a powerful approach to file management, bridging simplicity with sophistication. Through step-by-step instructions, exploration of CopyOptions, and a dive into exception handling, this article has armed you with the knowledge to employ Files.copy with confidence and precision. By integrating these practices into your development workflow, you not only optimize your file operations but also fortify your applications against potential file-related errors. Remember, mastering the nuances of Files.copy and its accompanying features enables you to handle files with unmatched efficiency and reliability, making your Java applications more robust and user-friendly.
Unraveling the Java Util Package in Java Classes
Ultimate Guide to Java Development Kit
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.