Java is a popular programming language that is used to build a wide range of applications. One of the most important classes in Java is the String class, which is used to represent strings of characters. String initialization in Java is a crucial aspect of programming with Java. In this article, we will explore the different ways to initialize strings in Java.
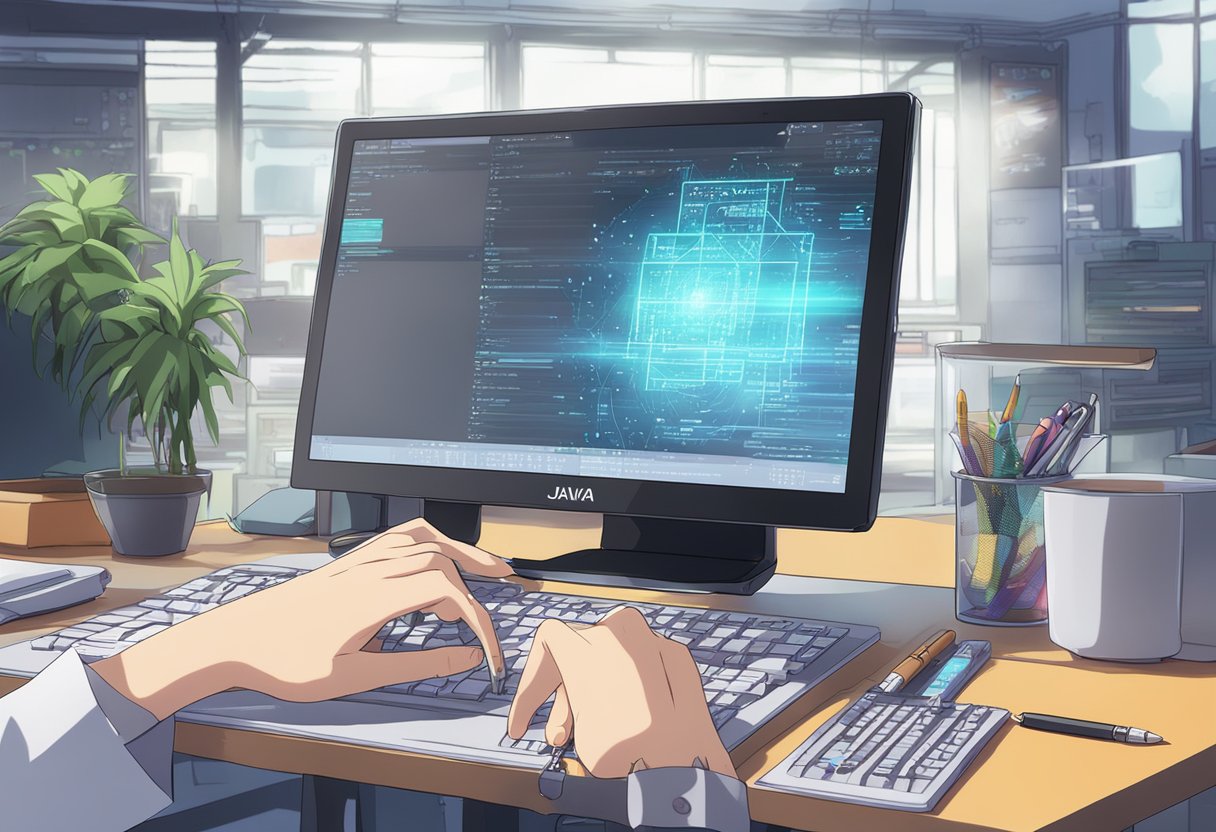
When it comes to creating strings in Java, there are two primary methods: direct initialization and indirect initialization. Direct initialization involves creating a string constant object in the string pool area of memory. This method is used when we want to create a string that cannot be modified. On the other hand, indirect initialization is used when we want to create a string that can be modified. This method involves creating a new string object using the new keyword.
In this article, we will also discuss the different ways to create strings in Java. We will cover the syntax for creating string literals using the new keyword and the different ways to initialize strings in Java. We will also explore the differences between string literals and string objects and when to use each one. Whether you are new to Java or an experienced programmer, understanding string initialization in Java is essential to writing efficient and effective.
Types of String Initialization
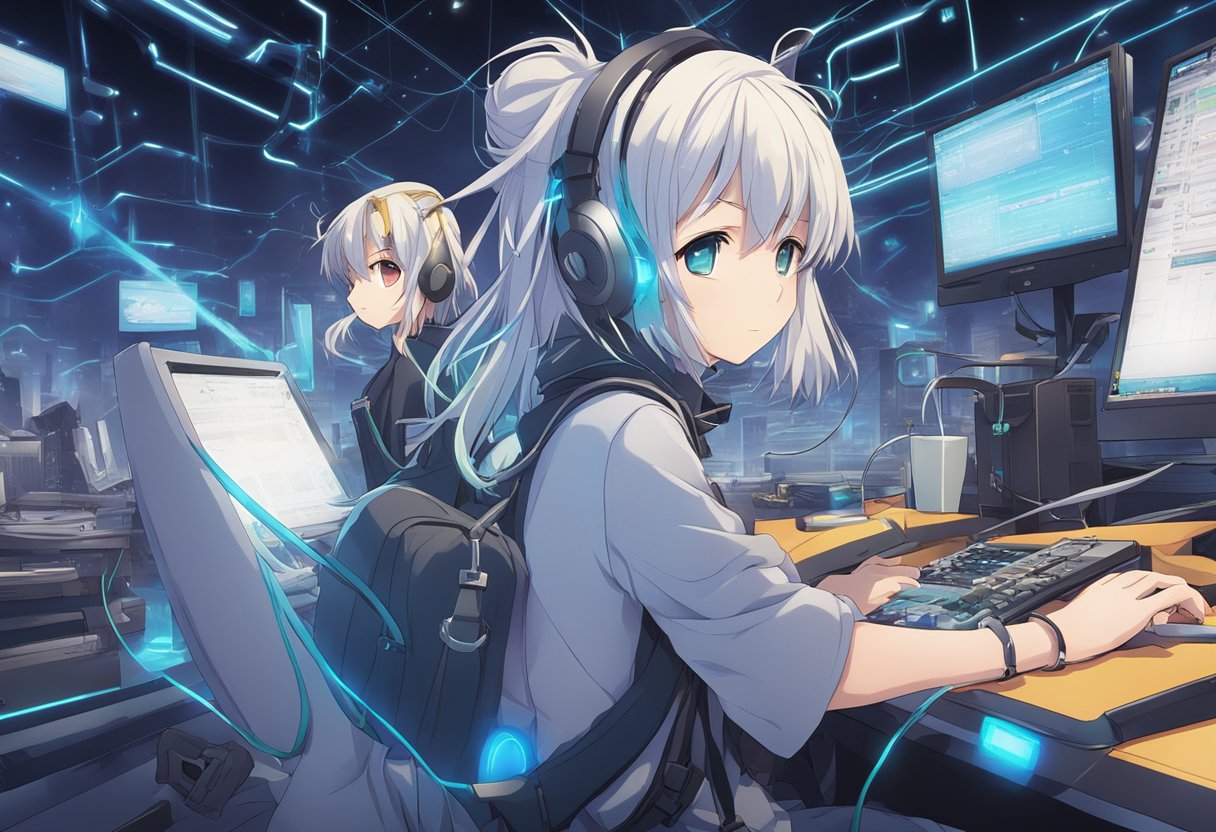
In Java, there are two ways to initialize a string: String Literal and New Keyword.
String Literal
String Literal is the most commonly used way to initialize a string in Java. It is a sequence of characters enclosed in double quotes. When we initialize a string using String Literal, Java creates a new string object in the String Constant Pool. If a string with the same value already exists in the String Constant Pool, Java returns a reference to that object instead of creating a new one.
For example, String str = "Hello World";
creates a new string object in the String Constant Pool with the value “Hello World”. If we create another string object with the same value using String Literal, Java returns a reference to the existing object instead of creating a new one.
New Keyword
Another way to initialize a string in Java is by using the New Keyword. When we initialize a string using the New Keyword, Java creates a new string object in the Heap Memory. Unlike String Literal, the string object initialized using the New Keyword is mutable, which means we can change its value after initialization.
For example, String str = new String("Hello World");
creates a new string object in the Heap Memory with the value “Hello World”. If we create another string object with the same value using the New Keyword, Java creates a new object in the Heap Memory.
In conclusion, both String Literal and New Keyword are valid ways to initialize a string in Java. The choice between the two depends on the requirements of the application. If we need an immutable string object, we can use String Literal. If we need a mutable string object, we can use the New Keyword.
String Immutability
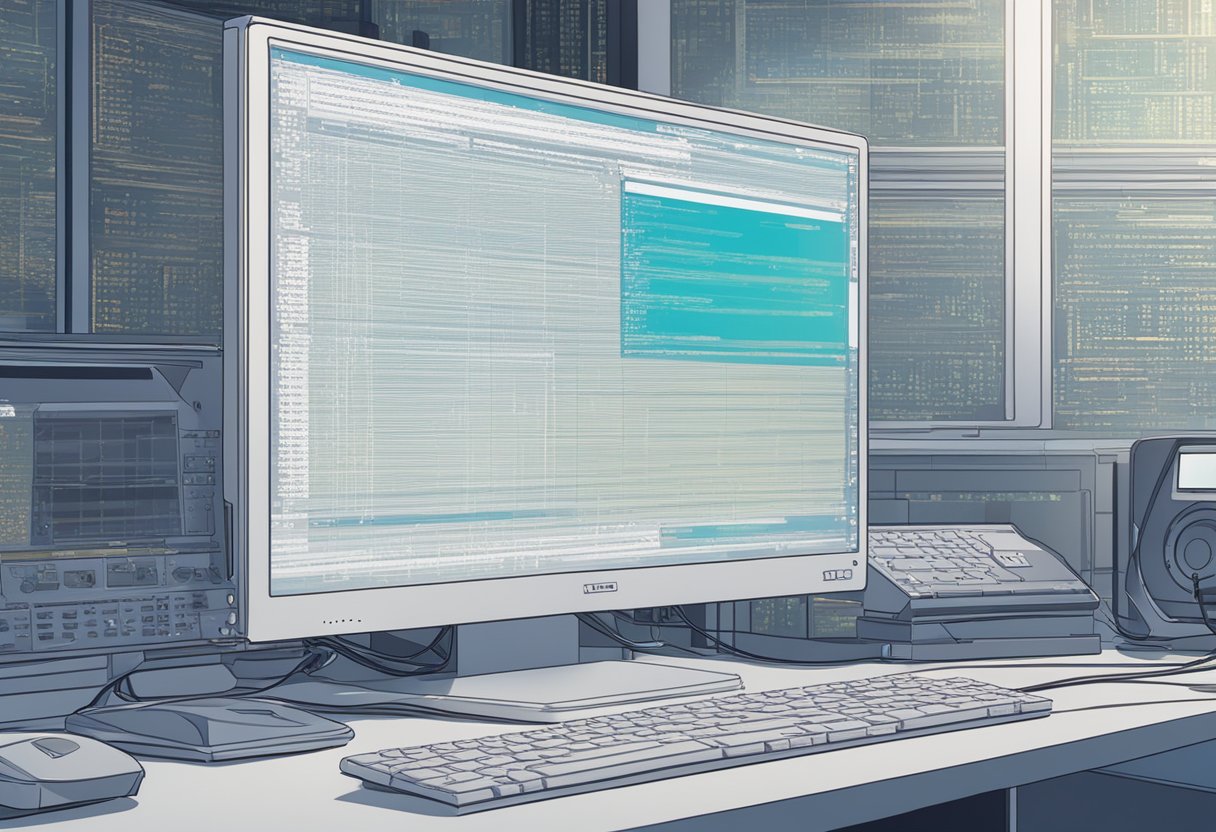
In Java, Strings are immutable. This means that once a String object is created, its value cannot be changed. Any operation that appears to modify the value of a String actually creates a new String object with the modified value. This can lead to performance issues in applications that perform a lot of String manipulation.
Why String Immutable In Java
To understand why String immutability is important, consider the following example:
String s = "hello";
s = s + " world";
In this code, a new String object is created with the value “hello world”. However, the original String object with the value “hello” is not modified. Instead, the variable s
is now pointing to the new String object.
One advantage of String immutability is that it makes Strings thread-safe. Because Strings cannot be modified, multiple threads can safely access the same String object without the risk of race conditions or other synchronization issues.
However, the downside of String immutability is that it can lead to unnecessary object creation and memory usage. To avoid this issue, Java provides the StringBuilder
and StringBuffer
classes, which are mutable alternatives to the String
class.
Overall, understanding String immutability is important for writing efficient and thread-safe Java code that performs a lot of String manipulation.
String Pool
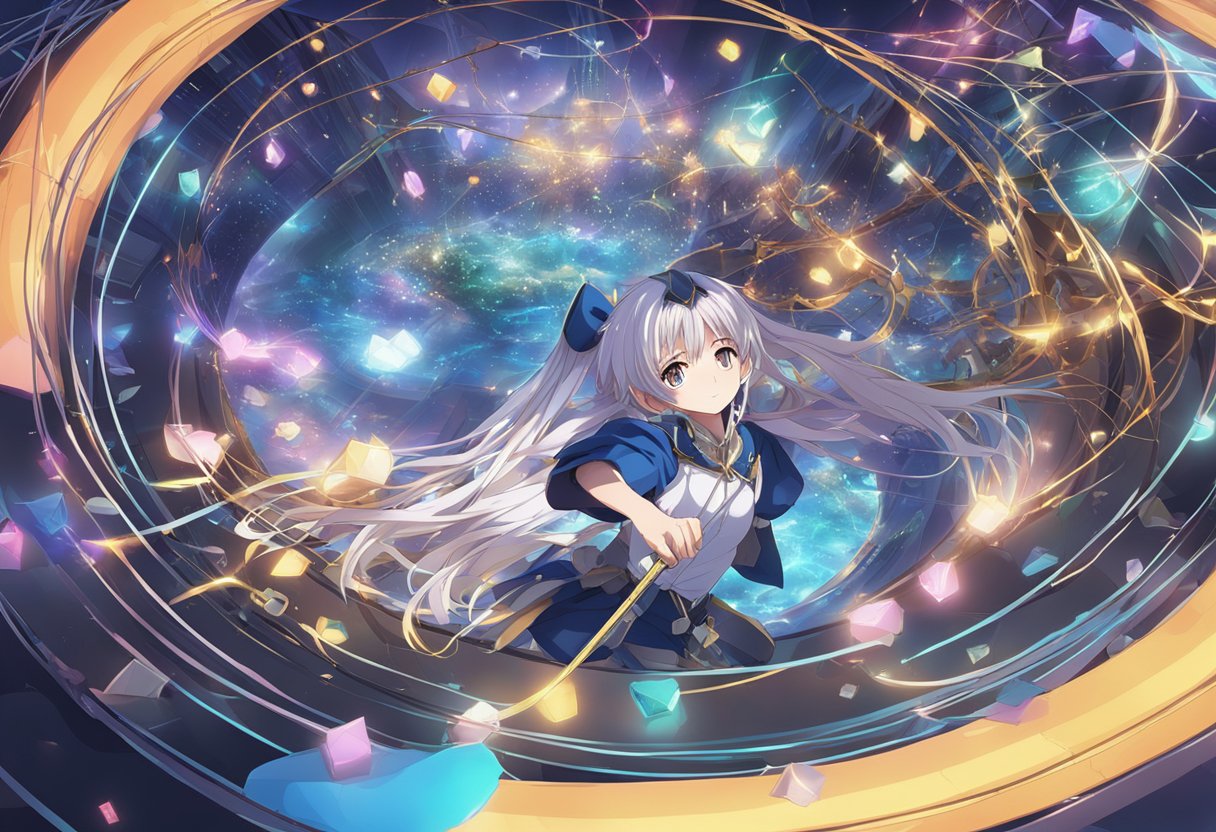
In Java, the String Pool is a special memory region that stores all the String literals. When we create a String variable and assign a value to it, the JVM searches the String Pool for a String of equal value. If it finds one, it returns a reference to that String object. If not, it creates a new String object and adds it to the String Pool.
The String Pool is created by the JVM during the startup of the application and is stored in the heap memory. It is a part of the permanent generation of the heap, which means that the String Pool persists throughout the lifetime of the application.
The String Pool is useful for reducing memory usage and improving performance. Because Strings are immutable in Java, the JVM can optimize the amount of memory allocated for them by storing only one copy of each literal String in the pool. This process is called interning.
It is important to note that not all Strings are stored in the String Pool. Strings that are created using the new
operator are not stored in the String Pool and are allocated in the heap memory. Therefore, it is recommended to use String literals wherever possible to take advantage of the String Pool and improve performance.
String Initialization Examples
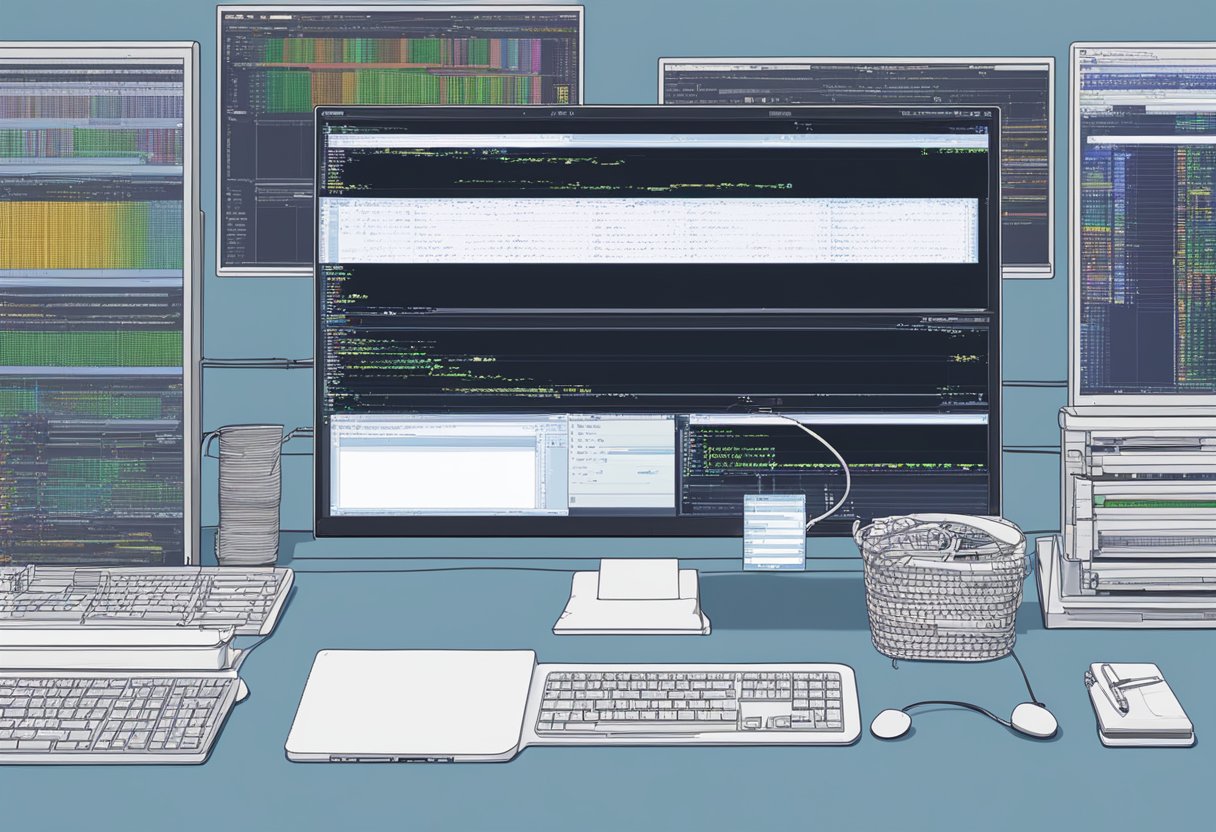
In Java, there are two ways to initialize a string variable: using a string literal or using the new
keyword. We will discuss both methods in this section.
String Literal Example
The most common way to initialize a string variable is by using a string literal. A string literal is a sequence of characters enclosed in double quotes. For example:
String greeting = "Hello, World!";
In this example, the string variable greeting
is initialized with the value “Hello, World!”. This is a simple and efficient way to create a string object.
New Keyword Example
Another way to initialize a string variable is by using the new
keyword. This method creates a new instance of the String
class. For example:
String greeting = new String("Hello, World!");
In this example, the string variable greeting
is initialized with the value “Hello, World!” using the new
keyword. This method is less commonly used than the string literal method, but it can be useful in certain situations.
It’s important to note that strings created using the new
keyword are not stored in the string pool. This means that if you create two string objects using the new
keyword with the same value, they will not be equal. To compare two strings created using the new
keyword, you should use the equals()
method.
In conclusion, there are two ways to initialize a string variable in Java: using a string literal or using the new
keyword. The string literal method is the most common and efficient way to create a string object, while the new
keyword method can be useful in certain situations.
Common Mistakes and How to Avoid Them

When initializing a string in Java, there are some common mistakes that developers make. Here are some of them and how to avoid them:
Using Incorrect Type of Characters
One of the most common mistakes is not using the correct type of characters when initializing a string. If you use a character type that is not supported by Java, it can cause errors or unexpected behavior in your programs. For example, if you use single quotes instead of double quotes to initialize a string, you will get a compilation error. To avoid this mistake, always use double quotes to initialize a string in Java.
Not Properly Escaping Special Characters
Another common mistake is not properly escaping special characters. Special characters are characters that have special meanings in Java, such as the double quote, single quote, backslash, and newline characters. If you don’t escape these characters properly, it can cause errors or unexpected behavior in your programs. To escape a special character, you need to use the backslash character followed by the special character. For example, to include a double quote in a string, you need to use the following syntax: \"
. To include a backslash, you need to use the following syntax: \\
.
Not Initializing String Variables
String variables in Java need to be initialized before they can be used. If you try to use an uninitialized string variable, you will get a compilation error. To avoid this mistake, always initialize your string variables before using them. If you are not sure what value to initialize your string variable with, you can use an empty string (""
) as a default value.
Using new String()
Instead of String Literal
When creating a new string object, it is recommended to use string literals instead of the new String()
constructor. This is because string literals are more efficient and use less memory than creating a new string object. For example, instead of using new String("hello")
, you can use "hello"
directly.
By avoiding these common mistakes, you can ensure that your string initialization in Java is error-free and efficient.
Best Practices

When it comes to initializing strings in Java, there are a few best practices that should be followed to ensure clean and efficient code.
Use String Literals
One of the most common ways to initialize a string in Java is by using string literals. This involves simply assigning a value to a string variable using quotes. For example:
String message = "Hello, world!";
Using string literals is generally preferred over creating new string objects with the new
keyword, as it is more concise and can help to avoid unnecessary object creation.
Declare Variables with Initial Values
It is generally a good practice to declare variables with initial values whenever possible. This helps to avoid null pointer exceptions and can make the code more readable. For example:
String message = ""; // initialize with an empty string
Use StringBuilder for Concatenation
When concatenating multiple strings together, it is recommended to use a StringBuilder
object instead of using the +
operator. This is because the +
operator creates a new string object each time it is used, which can be inefficient for large strings. Using a StringBuilder
allows for efficient string concatenation without creating unnecessary objects.
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(", ");
sb.append("world!");
String message = sb.toString();
By following these best practices, we can write clean and efficient code when initializing strings in Java.
Frequently Asked Questions
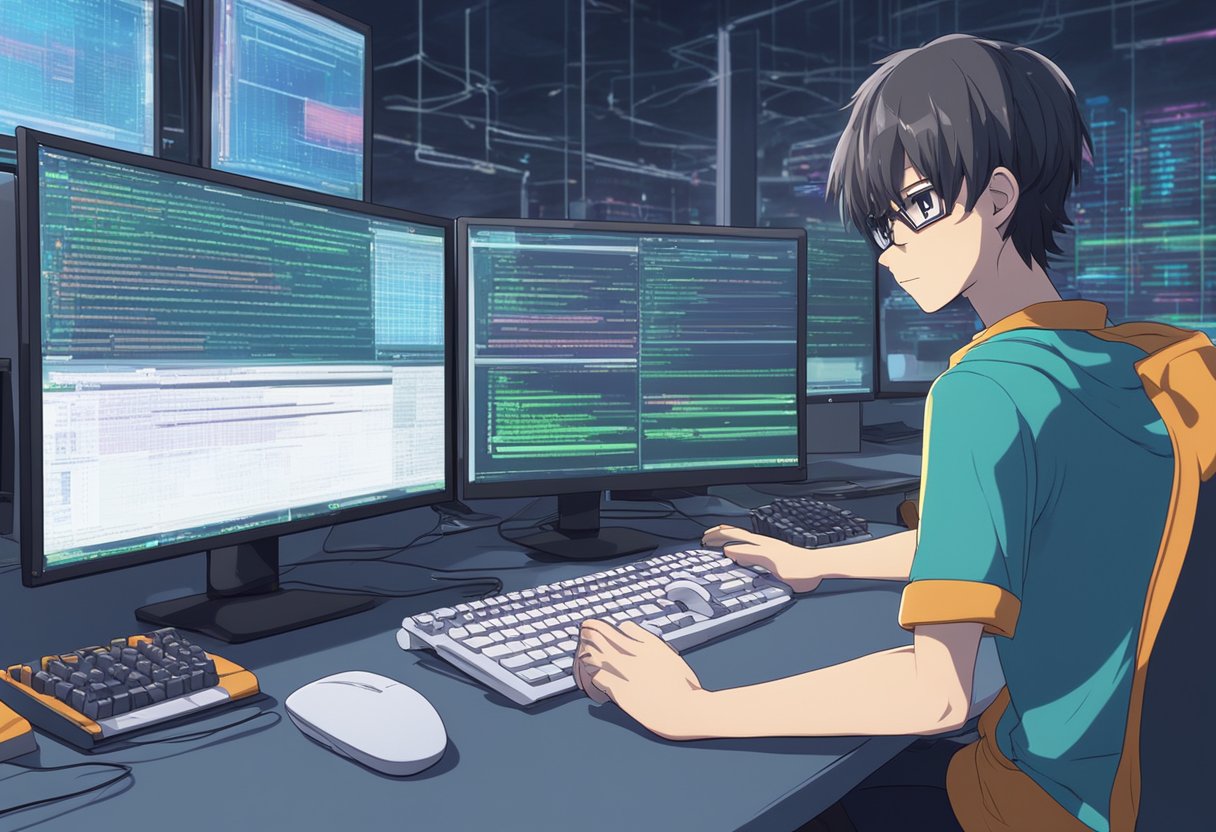
What is the default value of a Java String?
In Java, the default value of a String is null. If you declare a String variable without initializing it, it will have a value of null. This means that you cannot use the variable until you initialize it with a value.
How do you initialize an empty String in Java?
You can initialize an empty String in Java in several ways. One way is to use the empty string literal, which is a pair of double quotes with nothing in between them. For example, String str = "";
initializes an empty String. Another way is to use the String()
constructor with no arguments. For example, String str = new String();
also initializes an empty String.
What are some String initialization methods in Java?
There are several String initialization methods in Java. One way is to use the String
constructor with a character array. For example, String str = new String(new char[]{'H', 'e', 'l', 'l', 'o'});
initializes a String with the value “Hello”. Another way is to use the String
constructor with a byte array and a character set. For example, String str = new String(new byte[]{72, 101, 108, 108, 111}, StandardCharsets.UTF_8);
initializes a String with the value “Hello”. You can also use the valueOf()
method to convert other data types to Strings.
How do you declare a String in Java?
To declare a String variable in Java, you need to use the String
keyword followed by the variable name. For example, String str;
declares a String variable named str
. You can also initialize the String variable at the same time as declaring it. For example, String str = "Hello";
declares and initializes a String variable named str
with the value “Hello”.
How can you add to an empty String in Java?
In Java, you can add to an empty String by concatenating it with another String using the +
operator. For example, String str = ""; str += "Hello";
initializes an empty String and then adds the value “Hello” to it. Alternatively, you can use the StringBuilder
class to build up a String incrementally.
What are some common String methods in Java?
There are many common String methods in Java, including length()
, charAt()
, substring()
, indexOf()
, trim()
, toLowerCase()
, toUpperCase()
, startsWith()
, endsWith()
, replace()
, split()
, and join()
. These methods allow you to manipulate and extract information from Strings in various ways.
String Strip In Java: How to Remove Unwanted Characters in Java Strings