Form submission is an essential element in web development. It allows users to send data to the server for processing and storage. In the past, developers used server-side scripts to handle form submissions. However, with the advent of JavaScript, it is now possible to handle form submissions on the client side. This has made form submission faster and more efficient.

Understanding form submission in JavaScript is crucial for web developers. It involves creating a form, handling form data, and submitting the form to the server. JavaScript event handling is also essential in form submission. It allows developers to trigger specific actions when users interact with the form. Asynchronous form submission is another feature that enhances the user experience by allowing data to be sent to the server without reloading the page.
Understanding Form Submission
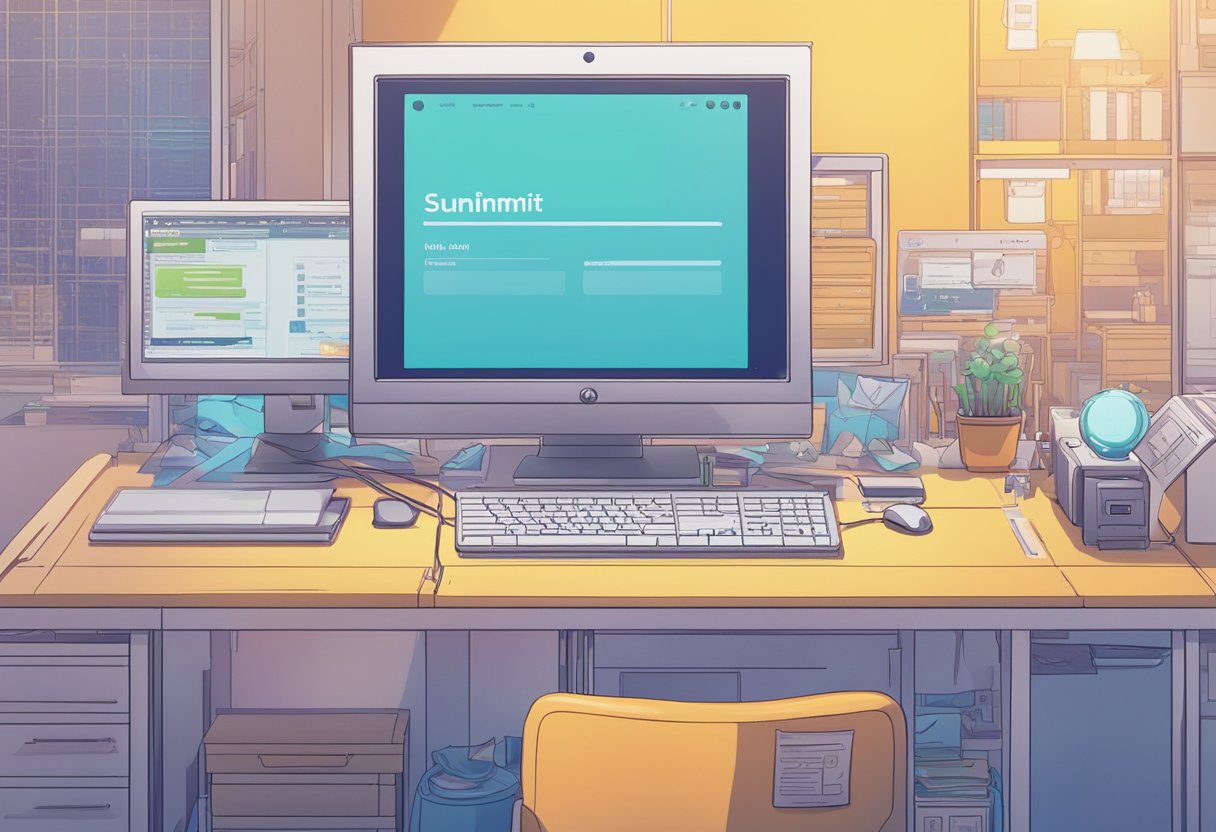
Submitting a form in HTML is a common way to collect user data. When a user submits a form, the data is sent to the server for processing. In this section, we will discuss the basics of HTML form submission and the role of JavaScript in form submission.
HTML Form Basics
HTML forms are used to collect data from users. A form is defined using the <form>
tag, which contains one or more form elements such as text fields, radio buttons, checkboxes, and buttons. When a user submits a form, the data is sent to the server using the HTTP POST method by default.
To submit a form, the user can click on a submit button, which is defined using the <input>
tag with the type attribute set to “submit”. Alternatively, the form can be submitted using JavaScript. In this case, the JavaScript code can be triggered by an event such as a button click or a form submit event.
Here’s an example of an HTML form with a submit button and JavaScript code to submit the form.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Submit Form with JavaScript</title>
<script>
function submitForm() {
// Get the form element
var form = document.getElementById("myForm");
// Submit the form
form.submit();
}
</script>
</head>
<body>
<h2>Submit Form with JavaScript</h2>
<form id="myForm" action="/submit_url" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<!-- Submit button -->
<input type="button" value="Submit Form" onclick="submitForm()">
</form>
</body>
</html>
In this example:
- The form is defined with the
form
tag and given anid
attribute of “myForm”. - It contains two input fields for username and password.
- There’s an input button with its
type
set to “button” and itsvalue
set to “Submit Form”. This button triggers thesubmitForm()
JavaScript function when clicked. - The
submitForm()
function retrieves the form element by its ID and submits it programmatically using thesubmit()
method.
The Role of JavaScript
JavaScript can be used to enhance the functionality of HTML forms. For example, JavaScript can be used to validate user input before the form is submitted. This can help prevent errors and improve the user experience.
JavaScript can also be used to submit a form without reloading the page. This is known as AJAX form submission. In this case, the JavaScript code sends the form data to the server using the XMLHttpRequest object, and the server responds with a JSON object or HTML code that can be used to update the page without reloading it.
In summary, HTML form submission is a basic feature of web development, and JavaScript can be used to enhance the functionality of HTML forms. By understanding the basics of HTML form submission and the role of JavaScript, developers can create more robust and user-friendly web applications.
Here’s an example of JavaScript code for AJAX form submission:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX Form Submission</title>
<script>
function submitForm() {
// Get form data
var formData = new FormData(document.getElementById("myForm"));
// Create XMLHttpRequest object
var xhr = new XMLHttpRequest();
// Configure XMLHttpRequest
xhr.open("POST", "/submit_url", true);
// Set content type header if needed
// xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
// Define function to handle response
xhr.onload = function() {
if (xhr.status === 200) {
// Process response here
console.log(xhr.responseText);
} else {
// Handle error
console.error('Error occurred while submitting the form.');
}
};
// Send form data
xhr.send(formData);
}
</script>
</head>
<body>
<h2>AJAX Form Submission</h2>
<form id="myForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<!-- Submit button -->
<input type="button" value="Submit Form" onclick="submitForm()">
</form>
</body>
</html>
In this code:
- The
submitForm()
function is called when the submit button is clicked. - It gathers the form data using
FormData
. - Creates an
XMLHttpRequest
object to send the form data to the server. - Configures the request with the appropriate method (POST), URL, and whether it’s asynchronous.
- Handles the response in the
onload
event of the request, processing the response or handling errors accordingly. - Finally, it sends the form data using
xhr.send(formData)
.
Creating a Form

When building a website, creating a form is one of the most common tasks. A form allows users to input data that can be submitted to the server for processing. In JavaScript, creating a form is straightforward and involves defining the form elements, input types, and form attributes.
Form Elements
A form element is a container for form controls such as input fields, checkboxes, and radio buttons. The <form>
tag is used to create a form element. The form element can contain one or more form controls.
Here’s an example of an HTML form element containing various form controls:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example Form</title>
</head>
<body>
<h2>Example Form</h2>
<form action="/submit_url" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br><br>
<label for="age">Age:</label>
<input type="number" id="age" name="age"><br><br>
<label for="gender">Gender:</label>
<select id="gender" name="gender">
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select><br><br>
<label for="subscribe">Subscribe to Newsletter:</label>
<input type="checkbox" id="subscribe" name="subscribe"><br><br>
<label for="feedback">Feedback:</label><br>
<textarea id="feedback" name="feedback" rows="4" cols="50"></textarea><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
In this code:
- The
<form>
tag creates the form element, with theaction
attribute specifying the URL to which the form data will be submitted, and themethod
attribute specifying the HTTP method (POST in this case). - Inside the form, various form controls are included, such as text input fields (
<input type="text">
), password input field (<input type="password">
), email input field (<input type="email">
), number input field (<input type="number">
), select dropdown (<select>
), checkbox (<input type="checkbox">
), and textarea (<textarea>
). - Each form control is associated with a label using the
<label>
tag, which improves accessibility and usability.
Input Types
Input types define the type of data that can be entered into an input field. There are several input types available in HTML, including text, password, email, number, and date. Each input type has its own set of attributes that can be used to customize the input field.
Form Attributes
Form attributes are used to define the behavior and appearance of a form. Some of the most commonly used form attributes include action, method, and target. The action attribute specifies the URL of the server-side script that will process the form data. The method attribute specifies the HTTP method used to submit the form data, which can be either GET or POST. The target attribute specifies the name of the window or frame where the server response will be displayed.
In summary, creating a form in JavaScript involves defining the form elements, input types, and form attributes. By using the appropriate form elements, input types, and form attributes, developers can create forms that are easy to use and visually appealing.
Handling Form Data

When working with forms in JavaScript, it is essential to understand how to handle form data. This section will cover two important aspects of handling form data: accessing form values and validation techniques.
Accessing Form Values
To access form values in JavaScript, you can use the document.forms
property. This property returns an array of all the forms on the page. You can then access the form elements by their name or index. For example, to access the value of an input element with the name “username” in the first form on the page, you can use the following code:
var username = document.forms[0].elements["username"].value;
You can also use the querySelector
method to access form elements. For example, to access the value of an input element with the name “username” using querySelector
, you can use the following code:
var username = document.querySelector('input[name="username"]').value;
Validation Techniques
Form validation is the process of checking whether the data entered by the user is valid or not. There are several techniques you can use to validate form data in JavaScript, such as regular expressions, built-in HTML5 validation, and custom validation functions.
Regular Expressions
Regular expressions are a powerful tool for validating form data. They allow you to define a pattern that the input must match. For example, to check whether an input contains only letters and spaces, you can use the following regular expression:
var pattern = /^[a-zA-Z\s]+$/;
You can then use the test
method of the regular expression object to check whether the input matches the pattern. For example, to check whether an input with the name “username” contains only letters and spaces, you can use the following code:
var username = document.forms[0].elements["username"].value;
if (!pattern.test(username)) {
alert("Username can only contain letters and spaces.");
}
Built-in HTML5 Validation
HTML5 provides built-in form validation attributes that you can use to validate form data. For example, you can use the required
attribute to make an input field required, and the type
attribute to specify the type of input expected. HTML5 also provides built-in validation messages that are displayed when the input does not meet the specified requirements.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML5 Form Validation</title>
</head>
<body>
<h2>HTML5 Form Validation</h2>
<form action="/submit_url" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required minlength="6"><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<label for="age">Age:</label>
<input type="number" id="age" name="age" required min="18" max="100"><br><br>
<label for="gender">Gender:</label>
<select id="gender" name="gender" required>
<option value="">Select Gender</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
We can set an error message as we want using This-
<input type="text" name="Myname" oninvalid="setCustomValidity('Please enter a text')" required />
Custom Validation Functions
You can also create custom validation functions to validate form data. These functions can check for specific requirements and display custom error messages. For example, you can create a custom validation function to check whether an input contains a valid email address:
function validateEmail(input) {
var pattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!pattern.test(input.value)) {
input.setCustomValidity("Please enter a valid email address.");
} else {
input.setCustomValidity("");
}
}
You can then call this function on the input element’s oninput
event to check the input value as the user types. For example:
<input type="email" name="email" oninput="validateEmail(this)">
In conclusion, handling form data is an essential part of working with forms in JavaScript. By understanding how to access form values and validate form data, you can create robust and user-friendly forms.
JavaScript Event Handling
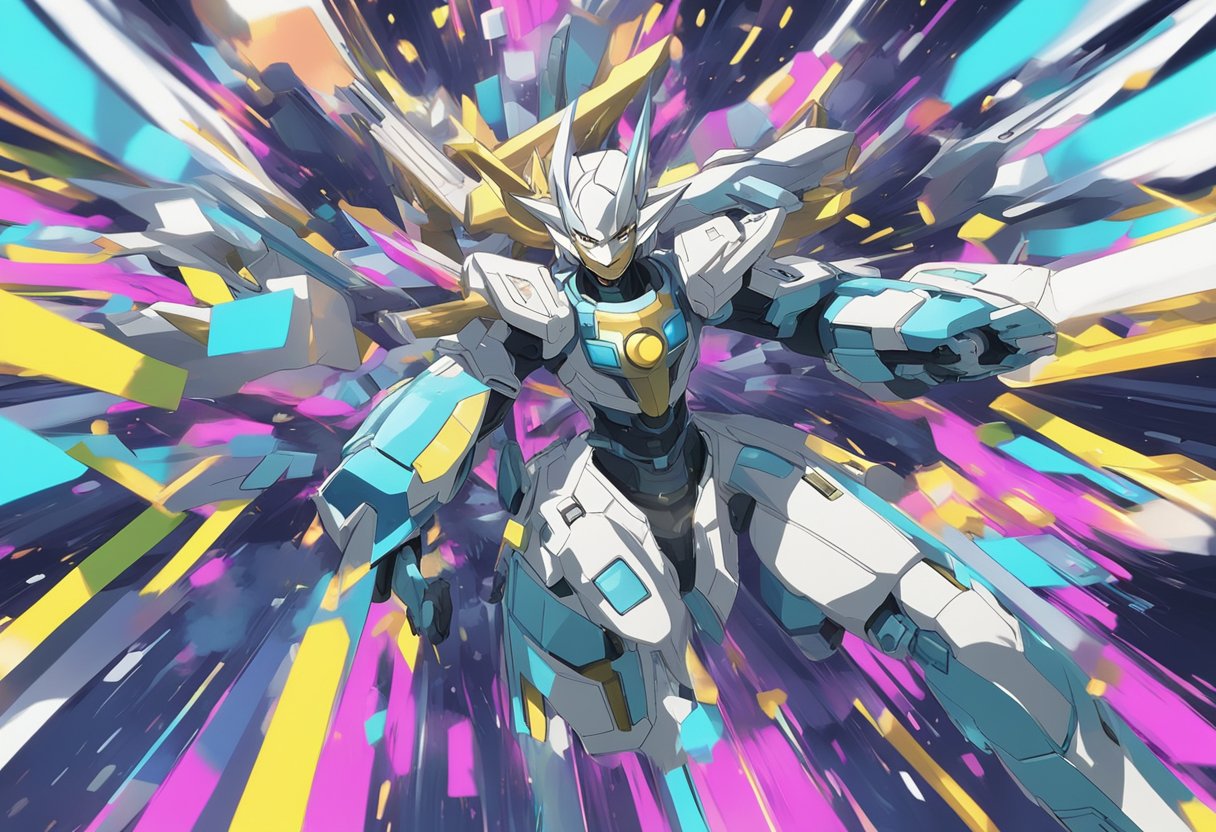
JavaScript event handling is a crucial aspect of web development. It allows developers to create interactive web pages by responding to user actions such as clicks, form submissions, and keystrokes. In this section, we will discuss two important concepts related to JavaScript event handling: the submit event and event propagation.
The Submit Event
The submit event is triggered when a user submits a form. When a form is submitted, the browser sends the form data to the server for processing. However, before the data is sent, the submit event is triggered, allowing developers to perform additional processing or validation.
To handle the submit event in JavaScript, developers can attach an event listener to the form element. The event listener will be triggered when the form is submitted, and the developer can use JavaScript to perform additional processing or validation before the data is sent to the server.
Event Propagation
Event propagation is the process by which an event is passed from one element to another in the DOM tree. When an event is triggered on an element, it can be handled by the element itself or by any of its ancestors in the DOM tree.
Event propagation can be either bubbling or capturing. In bubbling, the event is first handled by the innermost element and then propagated to its ancestors. In capturing, the event is first handled by the outermost element and then propagated to its descendants.
To handle event propagation in JavaScript, developers can use the event.stopPropagation()
method to stop the event from being propagated further up or down the DOM tree. This can be useful in scenarios where the developer wants to handle the event only on a specific element and not on its ancestors or descendants.
In conclusion, JavaScript event handling is an essential aspect of web development that allows developers to create interactive web pages. Understanding the submit event and event propagation is crucial for creating robust and efficient event handling code.
Asynchronous Form Submission
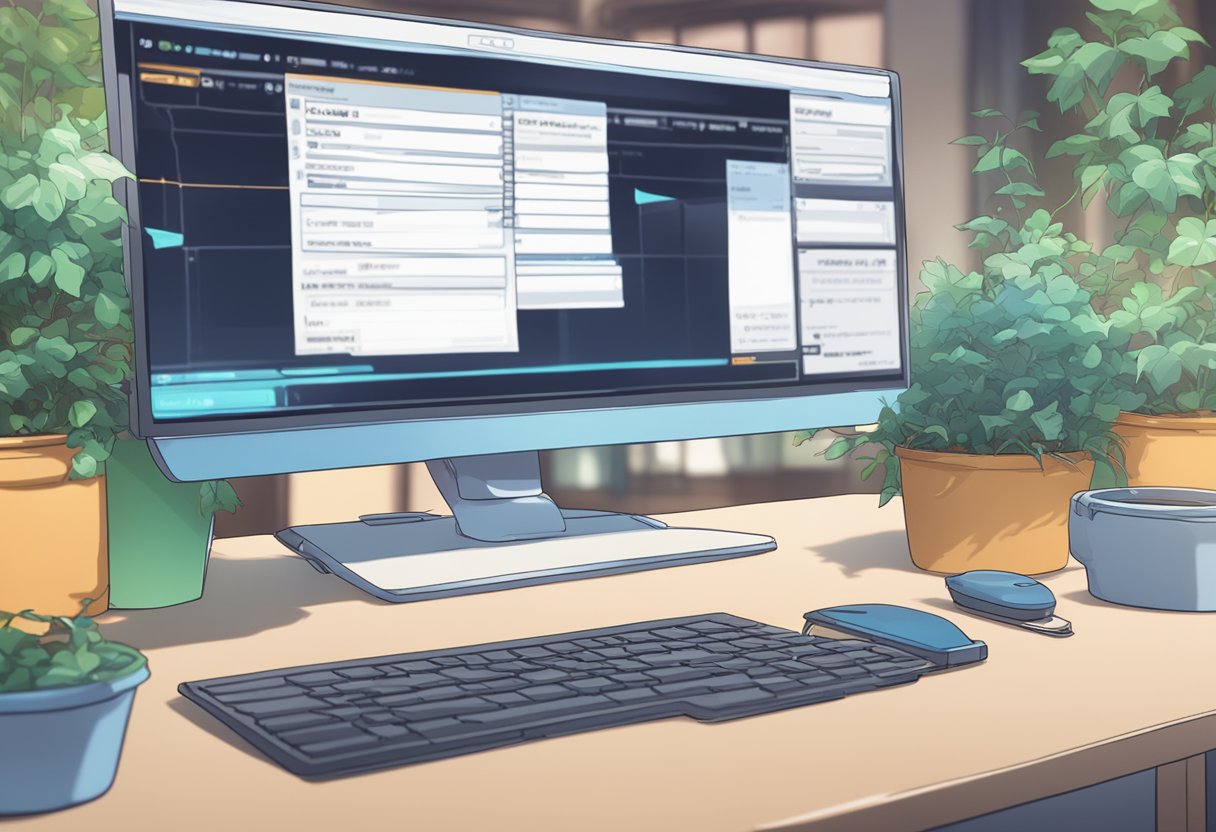
When submitting a form in JavaScript, it is important to consider the various ways to handle the submission asynchronously. This allows for a more efficient and responsive user experience, as the page does not have to reload every time the form is submitted. There are several ways to achieve asynchronous form submission in JavaScript, including AJAX requests, using the Fetch API, and handling responses.
AJAX Requests
One way to achieve asynchronous form submission is by using AJAX requests. AJAX stands for Asynchronous JavaScript and XML, and is a technique used to send and receive data asynchronously between a web browser and a server. When a form is submitted using AJAX, the page does not have to reload, and the user can continue to interact with the page while the form is being submitted.
To use AJAX for form submission, the developer can attach an event listener to the form’s submit event, prevent the default form submission behavior, and then use the XMLHttpRequest object to send the form data to the server. The server can then respond with a JSON object containing the status of the submission, which can be used to update the page accordingly.
Using Fetch API
Another way to achieve asynchronous form submission is by using the Fetch API. The Fetch API is a modern interface for fetching resources, including sending and receiving data from a server. It provides a simpler and more flexible way to make HTTP requests than the traditional XMLHttpRequest object.
To use the Fetch API for form submission, the developer can attach an event listener to the form’s submit event, prevent the default form submission behavior, and then use the fetch() method to send the form data to the server. The server can then respond with a JSON object containing the status of the submission, which can be used to update the page accordingly.
Handling Responses
Regardless of the method used for asynchronous form submission, it is important to handle the server’s response appropriately. This includes checking the status of the response to ensure that the submission was successful, and updating the page accordingly. The response can also include additional data, such as error messages or a confirmation message, which can be displayed to the user.
In conclusion, asynchronous form submission is an important technique for creating a more efficient and responsive user experience. By using AJAX requests or the Fetch API, and handling the server’s response appropriately, developers can create forms that submit data without requiring a page reload.
Enhancing User Experience

When it comes to web forms, user experience is paramount. A poorly designed form can lead to user frustration, errors, and even abandonment. Luckily, JavaScript provides several tools to enhance the user experience of form submission.
Progress Indicators
One way to enhance the user experience of form submission is by providing progress indicators. Progress indicators let the user know that their form submission is being processed and can help reduce user frustration and confusion.
There are several ways to implement progress indicators in JavaScript. One common method is to use a spinner or loading icon that appears while the form is being processed. Another method is to use a progress bar that fills up as the form is being submitted.
Form Reset and Clear
Another way to enhance the user experience of form submission is by providing the ability to reset or clear the form. This can be especially useful for long or complex forms where the user may want to start over or make changes.
JavaScript provides several methods for resetting or clearing a form. One common method is to use the reset()
method on the form element. This method clears all form fields and resets them to their default values.
Another method is to use the setCustomValidity()
method to set a custom error message on form fields. This can be useful for highlighting errors or guiding the user towards correct input.
In conclusion, JavaScript provides several tools for enhancing the user experience of form submission. By providing progress indicators and the ability to reset or clear the form, developers can create a more user-friendly and intuitive form submission process.
Security Considerations
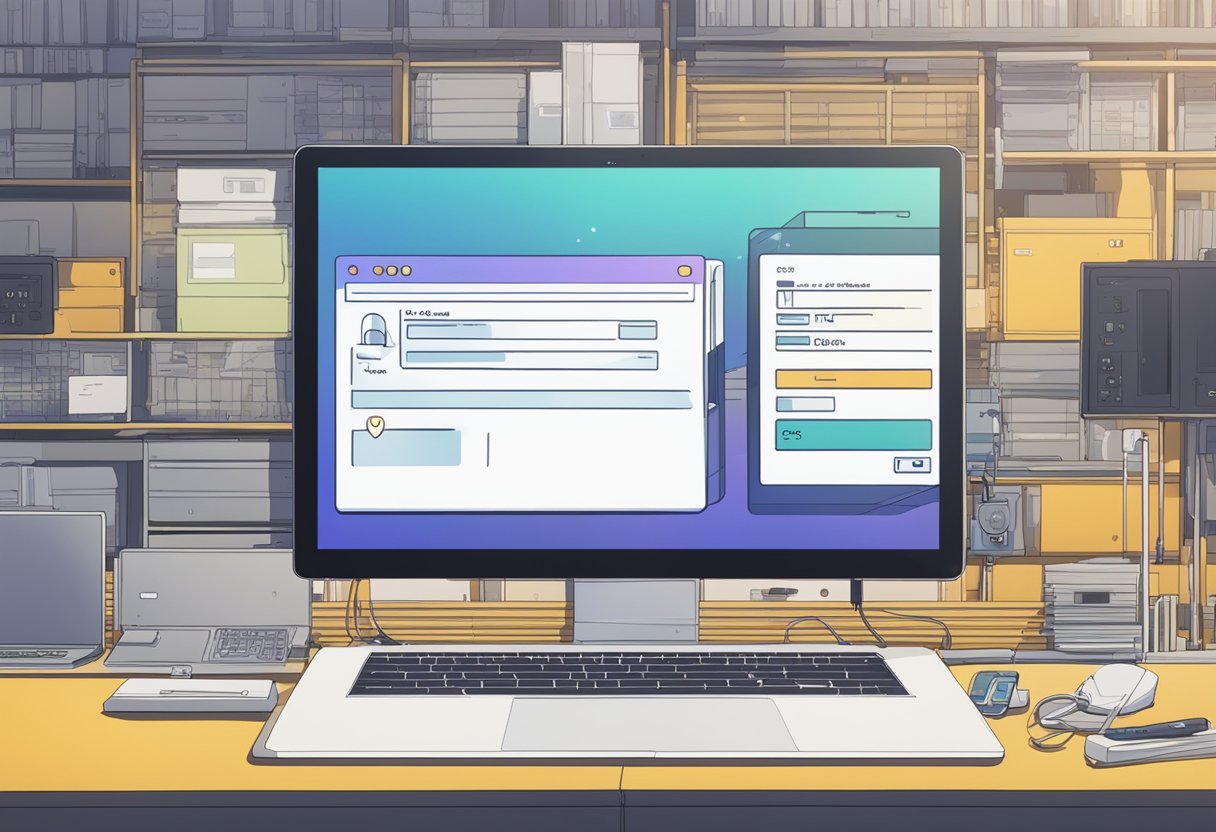
When building web forms, it’s important to take security considerations into account to prevent malicious attacks. Here are some of the most common security issues to keep in mind when working with form submissions in JavaScript.
Cross-Site Scripting (XSS)
Cross-site scripting (XSS) is a type of vulnerability that allows attackers to inject malicious code into a web page. This can happen when user input is not properly sanitized before being displayed on the page. To prevent XSS attacks, developers should always sanitize user input before displaying it on the page. This can be done by using a library like DOMPurify to sanitize HTML, or by using a framework like React that automatically sanitizes user input.
Cross-Site Request Forgery (CSRF)
Cross-site request forgery (CSRF) is another type of vulnerability that can occur when a user is tricked into performing an action on a website without their knowledge or consent. This can happen when an attacker creates a malicious form on their own website that submits data to the target website. To prevent CSRF attacks, developers should always include a CSRF token in their forms. This token is a unique value that is generated for each form submission and is used to verify that the submission is legitimate.
In addition to these specific vulnerabilities, developers should also keep in mind general security best practices when working with form submissions. This includes using HTTPS to encrypt data in transit, validating user input on the server-side, and limiting the amount of data that is collected from users. By following these best practices, developers can ensure that their web forms are secure and protect user data from malicious attacks.
Debugging Form Issues
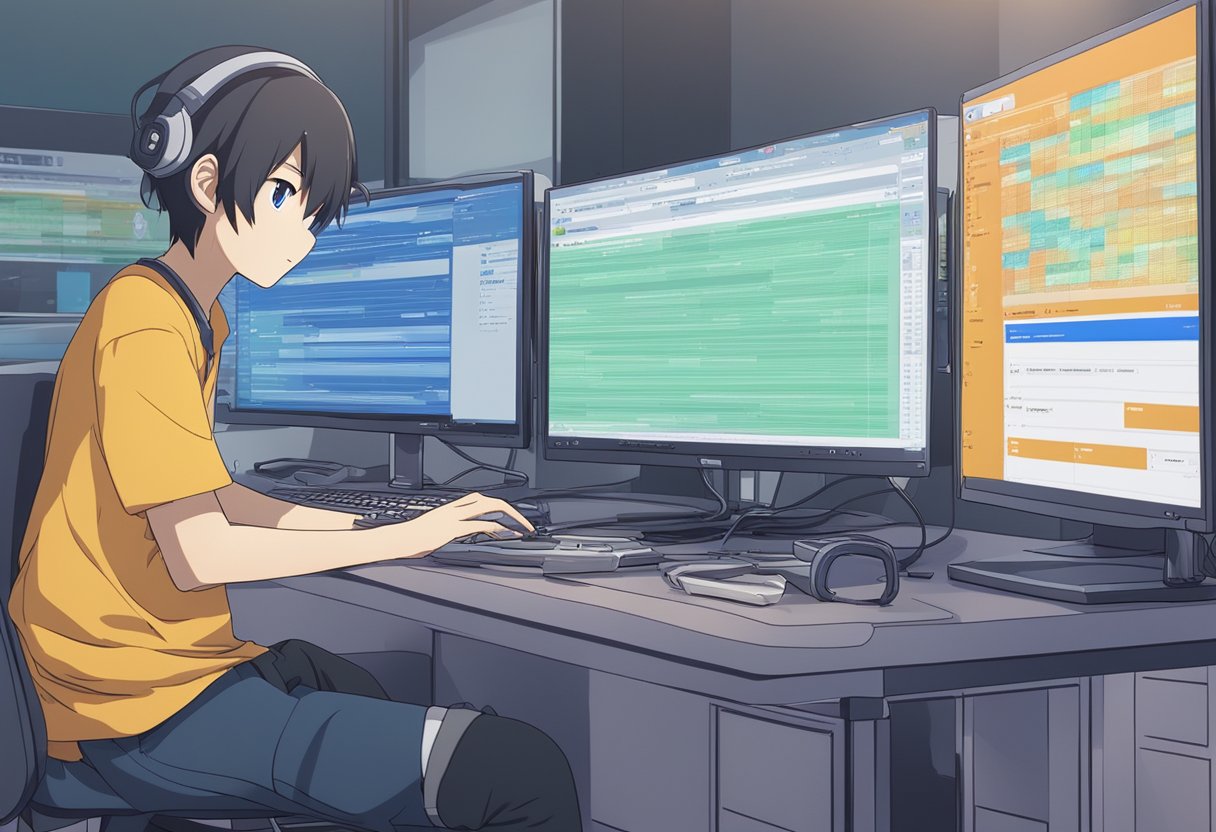
Forms are an integral part of any website, and they allow users to input data that can be sent to a server for processing. However, form submission issues can be a common problem that can lead to frustration for both users and developers. In this section, we will discuss some common form submission errors and how to debug them.
Common Errors
1. Validation Errors
Validation errors occur when the user enters incorrect or incomplete data in the form. For example, if a user forgets to enter their email address or enters an invalid email address, the form will not submit. To debug validation errors, developers can use the browser’s console to check for any error messages and use JavaScript to validate the input data before submitting the form.
2. Network Errors
Network errors occur when there is a problem with the server or the user’s internet connection. For example, if the server is down or the user’s internet connection is lost, the form will not submit. To debug network errors, developers can use the browser’s network tab to check for any error messages and use JavaScript to check the user’s internet connection before submitting the form.
3. Server Errors
Server errors occur when there is a problem with the server-side code that processes the form data. For example, if the server-side code is not configured correctly, the form data may not be processed correctly. To debug server errors, developers can use server logs to check for any error messages and use JavaScript to check the server-side code for any errors.
Debugging Tools
Developers can use various tools to debug form submission issues. Some of the most commonly used tools are:
1. Browser Console
The browser console is a built-in tool that allows developers to view error messages and debug JavaScript code. Developers can use the console to check for any error messages related to form submission and use JavaScript to validate and submit the form.
2. Network Tab
The network tab is a built-in tool that allows developers to view network activity and debug network-related issues. Developers can use the network tab to check for any error messages related to form submission and use JavaScript to check the user’s internet connection before submitting the form.
3. Server Logs
Server logs are logs generated by the server that can be used to debug server-side issues. Developers can use server logs to check for any error messages related to form submission and use JavaScript to check the server-side code for any errors.
In conclusion, form submission issues can be a common problem that can lead to frustration for both users and developers. However, by using the right tools and techniques, developers can easily debug and fix these issues, ensuring a smooth user experience.
Frequently Asked Questions
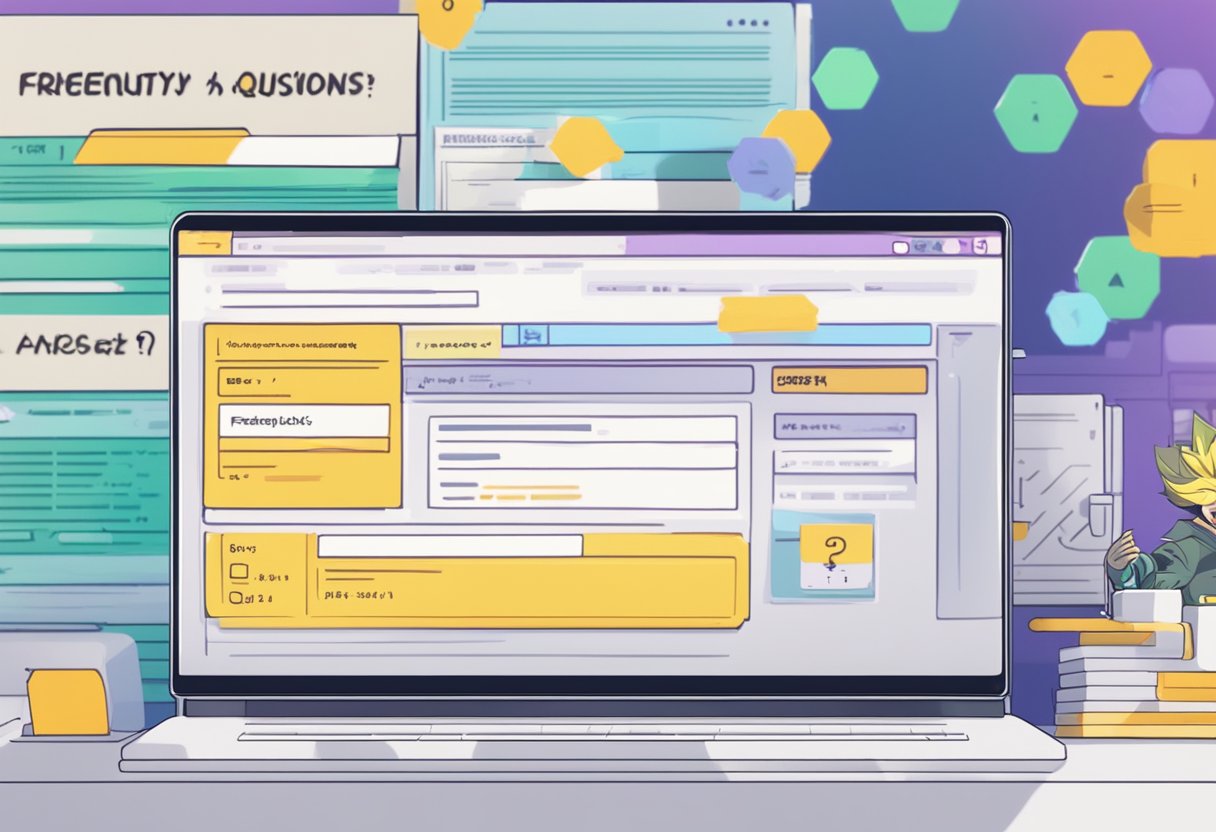
How do I capture form data upon submission using JavaScript?
To capture form data upon submission using JavaScript, you can use the submit
event listener and the FormData
API. The submit
event is triggered when the form is submitted, and the FormData
API allows you to create a key-value pair of the form data. You can then use this data to perform various actions, such as sending it to the server or manipulating it on the client-side.
What is the process for triggering a form submit event in JavaScript?
To trigger a form submit event in JavaScript, you can use the submit()
method. This method can be called on the form element to submit the form programmatically. Alternatively, you can use the click()
method on the submit button to trigger the form submission event.
How can a form be submitted automatically using JavaScript without user interaction?
A form can be submitted automatically using JavaScript without user interaction by calling the submit()
method on the form element. This can be useful in cases where the form submission is triggered by an event other than the user clicking the submit button, such as a timer or a change in the form data.
In what ways can I programmatically create and submit a form with JavaScript?
There are several ways to programmatically create and submit a form with JavaScript. One way is to use the createElement()
method to create a new form element, set its attributes and values, and then append it to the DOM. Another way is to use the XMLHttpRequest
object to send form data to the server without refreshing the page. You can also use the fetch()
API to send form data to the server using the POST method.
What are the differences between submitting a form with vanilla JavaScript versus jQuery?
Submitting a form with vanilla JavaScript versus jQuery is mostly a matter of syntax. jQuery provides a simpler syntax for handling form submission, but it ultimately uses the same underlying JavaScript methods. Vanilla JavaScript requires more code to handle form submission, but it provides more control over the process.
How does the submit() method function in the context of form handling in JavaScript?
The submit()
method is a built-in method in JavaScript that can be called on a form element to submit the form programmatically. When the submit()
method is called, the form data is sent to the server for processing. You can use the preventDefault()
method to prevent the default form submission behavior and handle the form data on the client-side.