When it comes to styling a website, CSS is the go-to language for web developers. CSS allows developers to customize the look and feel of a website, from fonts and colors to layout and positioning. However, sometimes the default styles provided by CSS don’t quite fit the design vision for a website. In these cases, it’s necessary to override or change the default styles.
Overriding CSS styles can be a tricky task, especially for beginners. There are several ways to override styles in CSS, including using the !important declaration, specificity, and inheritance. In this article, we will explore different methods for overriding CSS styles and provide examples to help you master the art of styling your website. Whether you’re a seasoned developer or just starting out, understanding how to override CSS styles is an essential skill for creating a visually appealing website.
The Basics of Overriding CSS Style
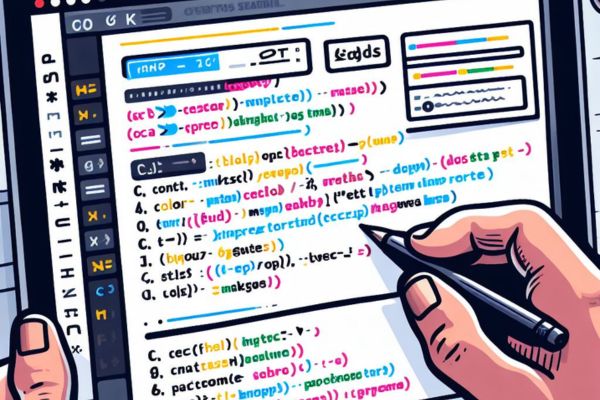
When it comes to styling a webpage, CSS plays a crucial role in determining how the page looks. However, sometimes we may need to override or modify the existing styles to achieve the desired look and feel. In this section, we will cover the basics of overriding CSS styles.
Specificity
CSS styles are applied based on the specificity of the selector. The more specific a selector is, the higher its priority. For example, a style applied to a class selector will have lower specificity than a style applied to an ID selector. Similarly, a style applied to an element selector will have lower specificity than a style applied to a class selector.
Cascading
CSS styles are also applied based on the order in which they are defined. If two styles have the same specificity, the one that comes later in the CSS file will take precedence. However, this can be overridden using the !important
keyword.
Using !important
The !important
keyword can be used to override any other styles applied to an element. However, it should be used sparingly as it can make the CSS code difficult to maintain and debug. It is recommended to use !important
only when absolutely necessary.
Inline Styles
Inline styles are styles applied directly to an HTML element using the style
attribute. Inline styles have the highest priority and will override any other styles applied to the element.
Using Important Declaration
When creating CSS styles, you may encounter situations where you need to override a previously defined style. One way to do this is by using the !important
declaration. In this section, we will discuss the syntax of the !important
declaration and when to use it.
Syntax of Important Declaration
The !important
declaration is added to the end of a CSS property value. It tells the browser that this style should take precedence over any other styles defined for that element. Here is an example:
p {
color: red !important;
}
In the example above, the color
property is set to red
and the !important
declaration is added to the end. This tells the browser that this style should take precedence over any other styles defined for p
elements.
When to Use Important Declaration
It is generally recommended to avoid using the !important
declaration whenever possible. However, there are some situations where it may be necessary. Here are a few examples:
- Overriding styles from third-party libraries: If you are using a third-party library that defines styles with the
!important
declaration, you may need to use it to override those styles. - Specificity issues: If you have two conflicting styles and one has a higher specificity than the other, you may need to use the
!important
declaration to ensure that the desired style is applied. - Accessibility concerns: If you need to ensure that a certain style is always applied for accessibility reasons, you may need to use the
!important
declaration.
In general, it is best to avoid using the !important
declaration whenever possible. Instead, try to use more specific selectors or refactor your CSS to avoid conflicts.
Overriding Inline Styles
Challenge with Inline Styles
Inline styles are styles that are applied directly to an HTML element using the style
attribute. They have the highest specificity, which means that they override styles defined in external style sheets or in the style
section of the HTML document. This can be a challenge when you want to change the style of an element that has an inline style applied to it.
Methods to Override Inline Styles
There are several methods to override inline styles. These include:
Using !important
The easiest way to override an inline style is to use the !important
keyword in your CSS rule. This makes the rule more specific than the inline style, and therefore takes precedence. For example, if you want to change the color of a <div>
element that has an inline style of color: red
, you can use the following CSS rule:
div {
color: blue !important;
}
Using Specificity
Another way to override an inline style is to use a more specific selector. For example, if you have an inline style applied to a <p>
element, you can override it by using a selector that is more specific, such as body p
. This selector has a higher specificity than the inline style and will therefore take precedence.
Using JavaScript
You can also use JavaScript to override inline styles. This can be useful if you want to change the style of an element dynamically, based on user interaction or other events. To override an inline style using JavaScript, you can use the style
property of the element. For example, if you have a <div>
element with an inline style of background-color: red
, you can change the background color to blue using the following JavaScript code:
var div = document.getElementById('myDiv');
div.style.backgroundColor = 'blue';
Overriding External and Internal Styles
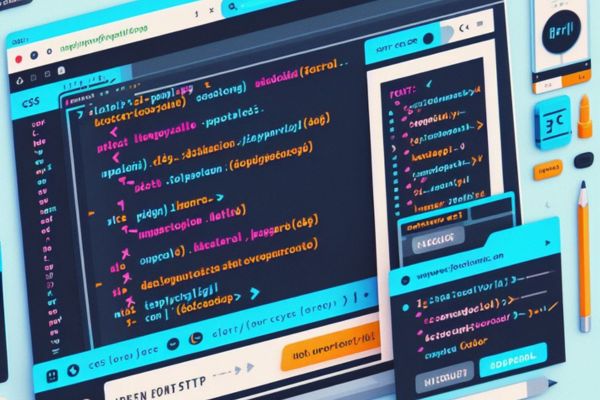
CSS styles can be defined in different ways: inline, internal, and external. Inline styles are applied directly to an HTML element using the style
attribute. Internal styles are defined within the HTML document using the <style>
tag. External styles are defined in a separate CSS file and linked to the HTML document using the <link>
tag.
Overriding External Styles
Overriding external styles is relatively easy. You can create a new CSS rule with the same selector and property as the original rule, but with a different value. The new rule should be placed after the original rule in the external CSS file. If the specificity of the new rule is higher than that of the original rule, the new rule will take precedence.
How To Handle CSS Precedence When Using React And CSS Classes?
For example, suppose we have the following CSS rule in an external file:
p {
color: blue;
}
We can override this rule by creating a new rule with the same selector and property, but a different value:
p {
color: red;
}
If we place this new rule after the original rule in the external CSS file, it will take precedence and all paragraphs will be displayed in red.
Overriding Internal Styles
Overriding internal styles is similar to overriding external styles. You can create a new CSS rule with the same selector and property as the original rule, but with a different value. The new rule should be placed after the original rule in the internal style block. If the specificity of the new rule is higher than that of the original rule, the new rule will take precedence.
For example, suppose we have the following internal style block in an HTML document:
<head>
<style>
p {
color: blue;
}
</style>
</head>
We can override this style block by creating a new rule with the same selector and property, but a different value:
<head>
<style>
p {
color: red;
}
</style>
</head>
If we place this new rule after the original rule in the internal style block, it will take precedence and all paragraphs will be displayed in red.
Specificity in CSS
Understanding CSS Specificity
CSS specificity is a way to determine which CSS rule will be applied to an element when multiple rules target the same element. Specificity is calculated based on the number of selectors used to target an element and the types of selectors used.
In general, the more specific a selector is, the higher its specificity value. For example, an ID selector has a higher specificity value than a class selector, which has a higher specificity value than an element selector.
Specificity can also be affected by the use of pseudo-classes, pseudo-elements, and inline styles. For example, a selector with a pseudo-class has a higher specificity value than a selector without a pseudo-class.
How Specificity Affects Overriding
Understanding CSS specificity is important when it comes to overriding styles. When two or more rules apply to the same element, the rule with the highest specificity value will be applied.
For example, suppose we have two rules that apply to a paragraph element: one rule sets the font size to 16 pixels and the other rule sets the font size to 18 pixels. If the first rule uses a class selector and the second rule uses an ID selector, the second rule will take precedence because it has a higher specificity value.
To override a style, we can increase the specificity of our selector. We can do this by using more specific selectors or by using the !important keyword. However, it is generally not recommended to use !important because it can make it difficult to override styles in the future.
In summary, understanding CSS specificity is important when it comes to overriding styles. By using more specific selectors or by increasing the specificity of our selectors, we can override styles and ensure that our styles are applied correctly.
Using JavaScript to Override Styles
CSS styles can be overridden using JavaScript. In this section, we will explore how to use JavaScript to override styles.
Accessing CSS Properties with JavaScript
Before we can override a CSS style, we need to access the CSS property we want to modify. We can do this using JavaScript DOM manipulation.
To access a CSS property using JavaScript, we can use the style
property of an element. For example, to access the color
property of an element with the id myElement
, we can use the following JavaScript code:
var element = document.getElementById("myElement");
var color = element.style.color;
This code will retrieve the value of the color
property of the myElement
element.
Overriding Styles with JavaScript
Once we have accessed the CSS property we want to modify, we can override it using JavaScript. There are several ways to do this, depending on the specific use case.
Changing a Single Property
To change a single CSS property using JavaScript, we can simply assign a new value to the style
property of the element. For example, to change the color
property of an element with the id myElement
to red, we can use the following JavaScript code:
var element = document.getElementById("myElement");
element.style.color = "red";
This code will change the color
property of the myElement
element to red.
Changing Multiple Properties
To change multiple CSS properties using JavaScript, we can use the cssText
property of the element. The cssText
property allows us to set the entire style attribute of an element using a string of CSS rules. For example, to change the color
and background-color
properties of an element with the id myElement
, we can use the following JavaScript code:
var element = document.getElementById("myElement");
element.style.cssText = "color: red; background-color: blue;";
This code will change the color
property of the myElement
element to red and the background-color
property to blue.
In summary, we can use JavaScript to access and override CSS styles. By using DOM manipulation, we can access CSS properties and modify them as needed. Depending on the specific use case, we can change a single property or multiple properties using JavaScript.
Best Practices for Overriding CSS Styles
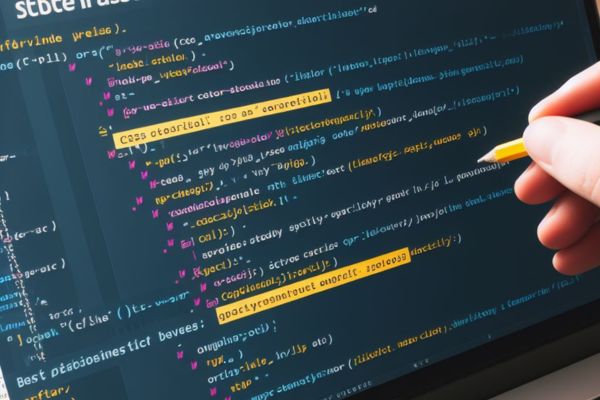
When it comes to overriding CSS styles, it’s important to follow best practices to ensure that your code is clean and easy to maintain. Here are some tips to keep in mind:
1. Use Specificity to Your Advantage
CSS specificity determines which style rule takes precedence when there are multiple rules that apply to the same element. To override a style rule, you can use a more specific selector. For example, if you want to override a style applied to all p
elements, you can use a more specific selector like #content p
.
2. Avoid Using !important
While using !important
can be a quick way to override a style rule, it can also make your code harder to maintain. Instead, try to use specificity to your advantage as mentioned above.
3. Use External Stylesheets
Using external stylesheets can make it easier to manage your styles and keep your HTML clean. You can override styles by adding a new rule to your external stylesheet with a more specific selector.
4. Avoid Inline Styles
Inline styles can be difficult to manage and override. Instead, try to use external stylesheets and selectors with higher specificity.
5. Comment Your Code
Commenting your code can make it easier to understand and maintain. When overriding styles, it can be helpful to include a comment explaining why you are overriding a particular style rule.
By following these best practices, you can ensure that your code is clean, easy to maintain, and follows industry standards.
Common Mistakes to Avoid
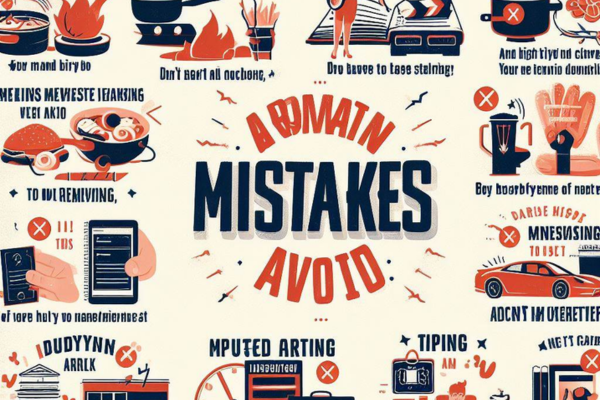
When it comes to CSS, there are a few common mistakes that many developers make when trying to override styles. Here are some of these mistakes and how to avoid them:
1. Using !important Too Much
Using !important
to override a style is a quick and easy way to get the job done, but it should be used sparingly. When used too much, it can make your code difficult to maintain and debug. Instead of using !important
, try to use more specific selectors or increase the specificity of your existing selectors.
2. Not Understanding CSS Specificity
CSS specificity determines which styles are applied to an element when multiple styles are defined. It’s important to understand how specificity works so that you can write effective selectors. A good rule of thumb is to use the least amount of specificity necessary to achieve your desired result.
3. Not Using Cascading Order
The order in which your styles are defined can affect which styles are applied to an element. Styles defined later in your code will override styles defined earlier. Understanding the cascading order of styles is important when trying to override existing styles.
4. Not Using a CSS Reset
Different browsers have different default styles for elements, which can lead to inconsistencies in how your website looks across different browsers. Using a CSS reset can help to normalize these default styles and make your page look consistent across all browsers.
By avoiding these common mistakes, you can write more effective CSS code and have an easier time overriding existing styles.
Frequently Asked Questions
How can I override CSS styles in React?
In React, you can override CSS styles by using inline styles or by creating a separate CSS file and importing it into your component. Inline styles take precedence over external styles, so you can use them to override specific properties. However, keep in mind that inline styles can make your code harder to read and maintain. Alternatively, you can use CSS modules or styled components to create component-specific styles that won’t affect other components.
What’s the best way to override inline styles in CSS?
Inline styles can be difficult to override because they have the highest specificity. One way to override them is to use the !important
keyword, but this should be used sparingly since it can cause unexpected behavior. Another option is to use a more specific selector, such as an ID or a combination of classes.
How do I override CSS styles using !important?
The !important
keyword can be used to override any other styles applied to an element. However, it should be used sparingly since it can cause unexpected behavior and make your code harder to maintain. If possible, try to use more specific selectors or inline styles to override styles instead.
What’s the syntax for overriding a CSS class style?
To override a CSS class style, you can create a new rule with the same selector and a higher specificity. For example, if you have a class .button
with a style background-color: blue
, you can override it with .my-button.button { background-color: red; }
. This will only affect elements with both the .my-button
and .button
classes.
How do I override styles in SCSS?
In SCSS, you can use the !default
flag to set default values for variables. Then, you can override these variables in your component-specific styles. For example, you can define $primary-color: blue !default;
in your global styles, and then override it with $primary-color: red;
in your component-specific styles.
Can I override a child element’s CSS style from a parent element?
Yes, you can override a child element’s CSS style from a parent element by using a more specific selector. For example, if you have a child element with a class .button
and a parent element with a class .container
, you can override the child element’s style with .container .button { background-color: red; }
. This will only affect .button
elements that are inside a .container
element.
How to Change Font Color in HTML: Guide
Basic CSS Interview Questions with Answers
CSS Cheat Sheet: A Comprehensive Guide
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.