As we continue to expand on the digital landscape, the significance of building resilient and robust applications cannot be understated. Among the many facets of such applications, the utility of databases is key to managing, retrieving, and manipulating data. Java, being one of the most commonly used programming languages, offers varied and efficient ways to interact with databases via JDBC (Java Database Connectivity). From understanding the intricacies of JDBC, grasping connection procedures, and mastering error handling techniques, this discourse provides a comprehensive insight into connecting to a database in Java.
Understanding JDBC
Exploring JDBC and Its Vital Role in Java
The tech world today is a digital arena where Java stakes a claim. The Java Database Connectivity (JDBC) holds a fundamental pivot in this milieu. As a software application programmer interface (API), JDBC is designed to work within the Java environment, facilitating communication and interaction between Java and wide-ranging databases. It executes this ingenious feat across all platforms, forging a significant innovation that makes data-handling tasks easier for developers.
One principal trait of JDBC is its standardization. JDBC maintains a neutral platform which allows it to function with any database, provided a suitable driver exists. This universal appeal fosters database independency, offering seamless transitions and flexibility. With this impressive capability, there is absolutely no need to code the application to a particular database.
In action, JDBC works by utilizing drivers, small software packages that provide a mechanism to connect from Java to databases. These drivers communicate with the database servers using the specific server’s language. Four types of JDBC drivers are currently available, each with different methods of making a connection:
The JDBC-ODBC Bridge Driver acts as a bridge to communicate with database servers using the ODBC driver. The Native-API Driver converts JDBC commands into database-specific calls. The Network Protocol Driver, on the other hand, makes use of middleware to send calls from the client to the server. Lastly, the Thin Driver is ideal for client-side use with no assistance from software on the server-side.
However, putting JDBC into operation is not a daunting task. By employing the proper syntax in coding, as is the nature with Java, anyone can reap the multi-faceted benefits of JDBC. Once the appropriate driver is loaded, a connection is made using the DriverManager.getConnection() method. Following this, a SQL statement object is created, using which SQL queries are dispatched to the respective databases. The delightfully direct ResultSet.execute() method then retrieves the result.
In a closer perspective, understanding JDBC balloons into an exciting exploration of how its elements brilliantly intermingle towards managing data in Java. It offers the allure of seamless communication between Java applications and any database, a tech marvel indeed. As developers or tech enthusiasts, understanding JDBC and its workings in Java prompts the exciting possibility of expansive development, unhindered by the limitations of database dependency. Remember to always select and utilize the appropriate JDBC driver depending on the specifics of your application to achieve optimal results – putting the technology’s full capabilities to its best use. It’s never been a better time to be a Java developer with the artful mechanics of JDBC at your fingertips. Enjoy the journey!
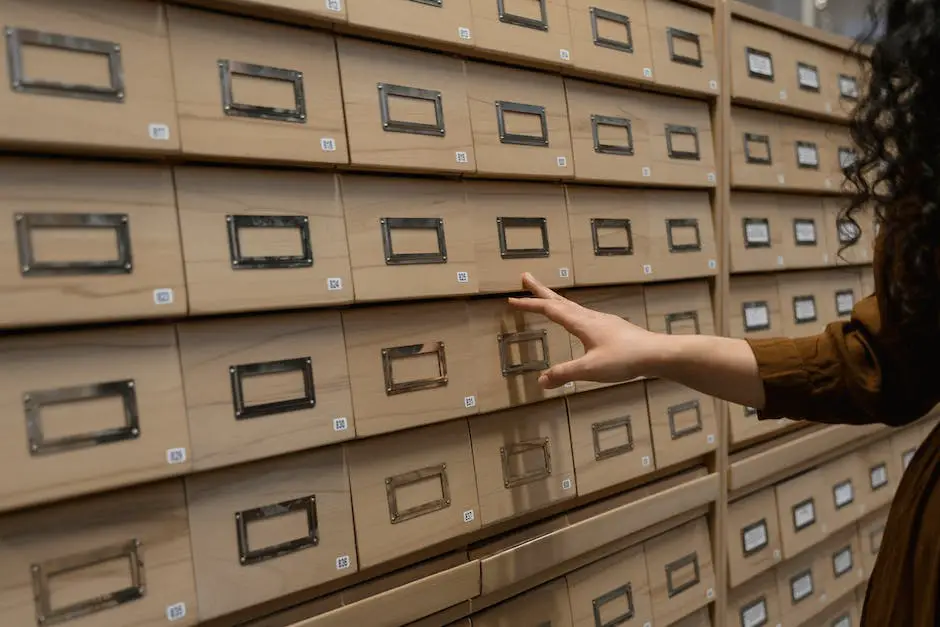
Implementing Connection Procedure
Delving Deeper: Establishing a Database Connection in Java Using JDBC
In the ever-evolving realm of technology, efficiency reigns supreme. The sense of urgency is felt more than ever in the way developers interact with and manipulate databases to achieve desired objectives promptly. For Java programmers, the Java Database Connectivity (JDBC) API becomes the ultimate tool of choice. It masterfully simplifies the pivotal task of connecting a database with a Java application. Let’s decipher the steps involved in this process.
Setting the Stage: JDBC API And Suitable Drivers
Before embarking on the journey, ensure that you’re armed with a JDBC API, and an appropriate JDBC driver. The API acts like a bridge, enabling Java applications to dialogue with the database, and the JDBC driver makes this dialogue possible.
Linking to a Database: Code Construction
Let’s now explore how to make our JDBC and database introduce themselves:
-
Load the JDBC Driver: Initiate the connection by loading the JDBC driver via the static method
Class.forName()
.Class.forName("com.mysql.jdbc.Driver");
Here,
com.mysql.jdbc.Driver
is the driver class specific for MySQL database. Remember, the driver class varies based on the database. -
Connect to the Database: You interact with the database through the
java.sql.Connection
interface. To establish a connection, use theDriverManager.getConnection()
method.Connection con = DriverManager.getConnection(url, userName, password);
A few notes on the parameters:
- The
url
is the database URL. For a MySQL database, it typically takes this form:
jdbc:mysql://hostname/databaseName
- The
- The
userName
andpassword
are your credentials for the database. Configure SQL Statements: Following a successful connection, you’re now ready to execute
CREATE
,INSERT
,UPDATE
,DELETE
, andSELECT
SQL commands via thejava.sql.Statement
interface. You can create an instance by calling thecreateStatement
method on the connection object.Statement stmt = con.createStatement();
You can then execute SQL statements using the
executeQuery()
orexecuteUpdate()
methods.-
Close the Connection: Always remember to conclude by closing the connection. This prevents unnecessary memory leaks and related issues.
con.close();
Given that this process heavily interacts with external resources, it is susceptible to unforeseen errors. It’s crucial to implement proper exception handling within your code to manage this. Ensure every SQL operation is wrapped within a try-catch
block, specifically looking out for SQLException
instances.
This excursion through database connectivity in Java provides the next-level proficiency and command developers need. Leveraging the JDBC API and drivers, Java can converse fluently with any database, harnessing data to solve complex problems and deliver stunning results. Consequently, professionals poised at the intersection of Java and JDBC are set for remarkable data management feats and significant computational efficiency.
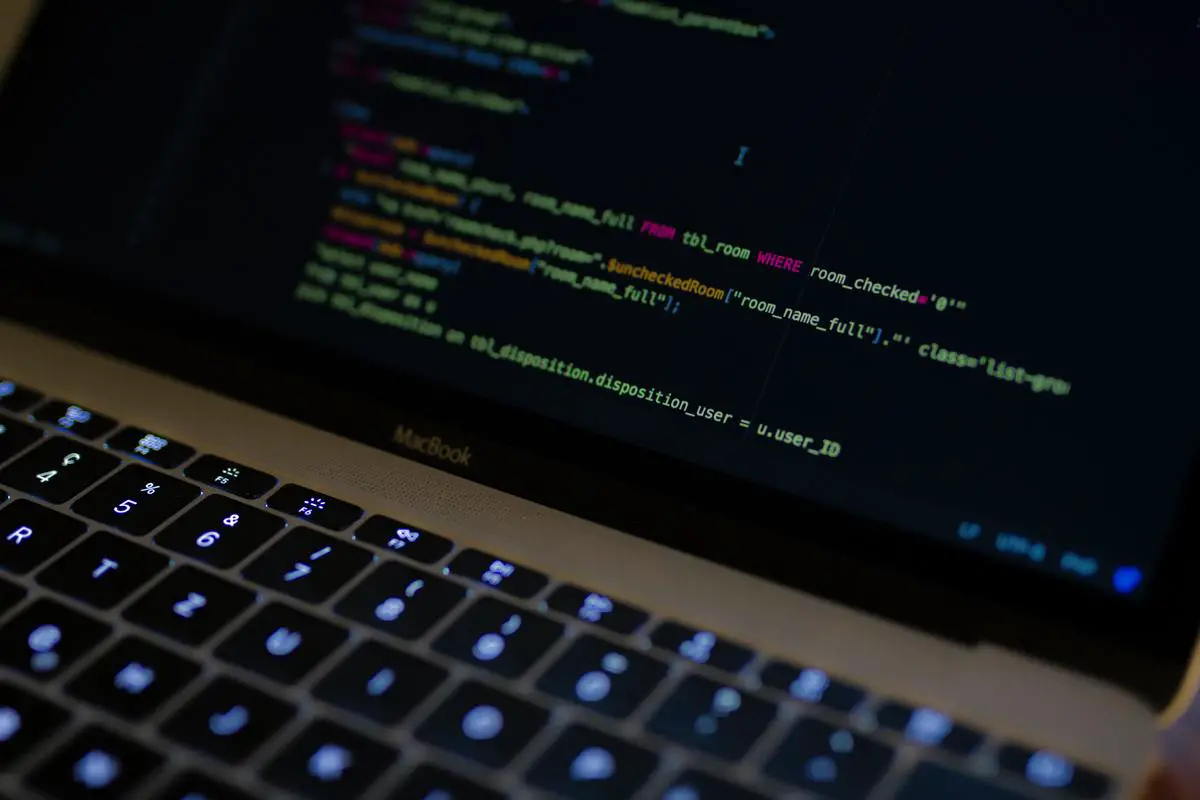
Photo by casparrubin on Unsplash
Error Handling
Moving beyond the basics of Java Database Connectivity (JDBC) and database interaction, error handling stands as the major pivot. Though the JDBC framework does an excellent job in standardizing the way Java interacts with databases, it throws up certain errors and exceptions during connectivity. Here, we unfold the best ways to tackle such issues.
Firstly, let’s distinguish between Errors and Exceptions. Errors are serious issues that applications can’t recover from, often highlighting problems within the Java Virtual Machine (JVM), while Exceptions denote conditions that can be handled or averted by the programmer.
In JDBC, the SQLException class manages the database access errors. Best practice encourages you to anticipate potential issues like transactional errors, connection failures or SQL related anomalies, and handle them in your program code.
Yet how, exactly? The first step upon any SQLException is to call the getSQLState method. It returns a five-char alphanumeric code, providing insights about the exact nature of the error. A set of standard SQLState values make communication with the database management system more intuitive even in case of any connectivity issues.
For transparency and specific error logging, deploy the getErrorCode method. It returns vendor-specific values, thereby highlighting the precise error that has originated from the database.
To get a descriptive error message outlining what went wrong, bring the getMessage method into play. This could be particularly useful when analyzing SQLException.
Error tracking gets far productive with getNextException method. This conveys chained exceptions for situations where one exception triggers another. Such traceability is critical when debugging complex database operations.
Handle nested exceptions using getCause and initCause from the Throwable class. It unravels the underlying cause of the SQLException. For more nuanced visibility into the stack trace, use printStackTrace. This method prints the SQLException, its backtrace, and the chain of exceptions, lends more power to the debugging process.
To summarize: anticipate, capture, and handle SQLExceptions skillfully. JDBC serves as a robust interfacing standard, but command over error handling ensures your Java applications run smoothly, despite hiccups in database connectivity. Ensure that your code is proactive, not just reactive. It is crucial to understand that mastering error management in JDBC is less about circumventing issues, but more about continuously improving the connectivity, thereby elevating the performance and stability of your Java applications. Programming is, after all, not just about building—it’s about troubleshooting and improving.
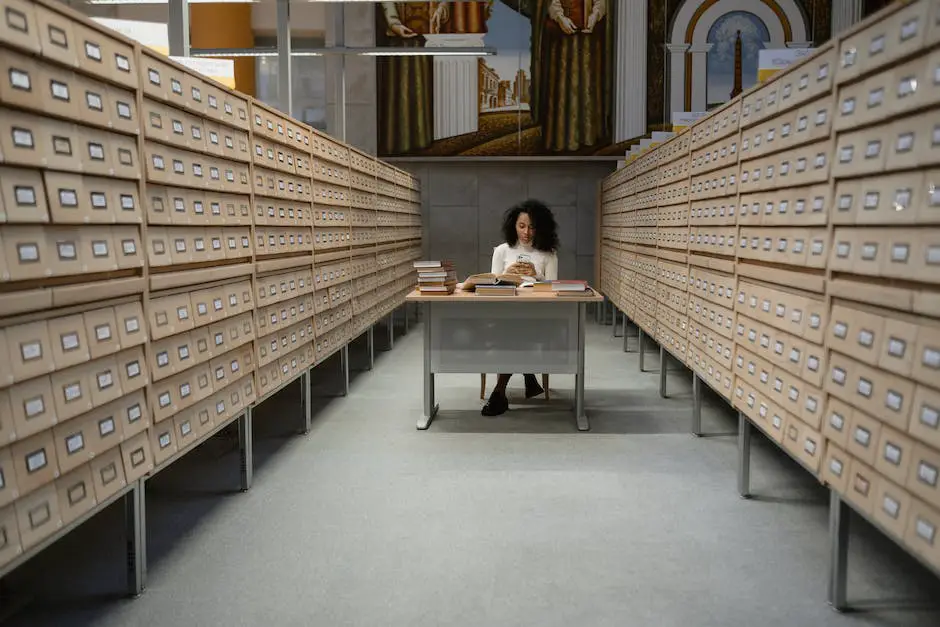
Developing a solid understanding of Java Database Connectivity (JDBC) and practicing the procedures of implementing connections with a keen focus on error handling can greatly enhance your ability to create powerful and error-resilient applications. When you consider the various methods to query and update data in a database, handling exceptions correctly becomes paramount. These skills, when learned and applied effectively, serve as a quintessential part of your journey to becoming a proficient Java programmer and handling database connectivity with finesse. May your journey be filled with understanding the codes, connecting the dots, and unveiling the intricacies of Java database interaction!
Visual Studio Code for Java: Boost Your Coding Efficiency