JSON (JavaScript Object Notation) is a lightweight data-interchange format that has become a popular choice for data exchange between systems. Java is one of the most widely used programming languages, and with the release of Java 8, it has become much easier to work with JSON data. In this article, we will explore how to convert JSON data to Java objects in Java 8.
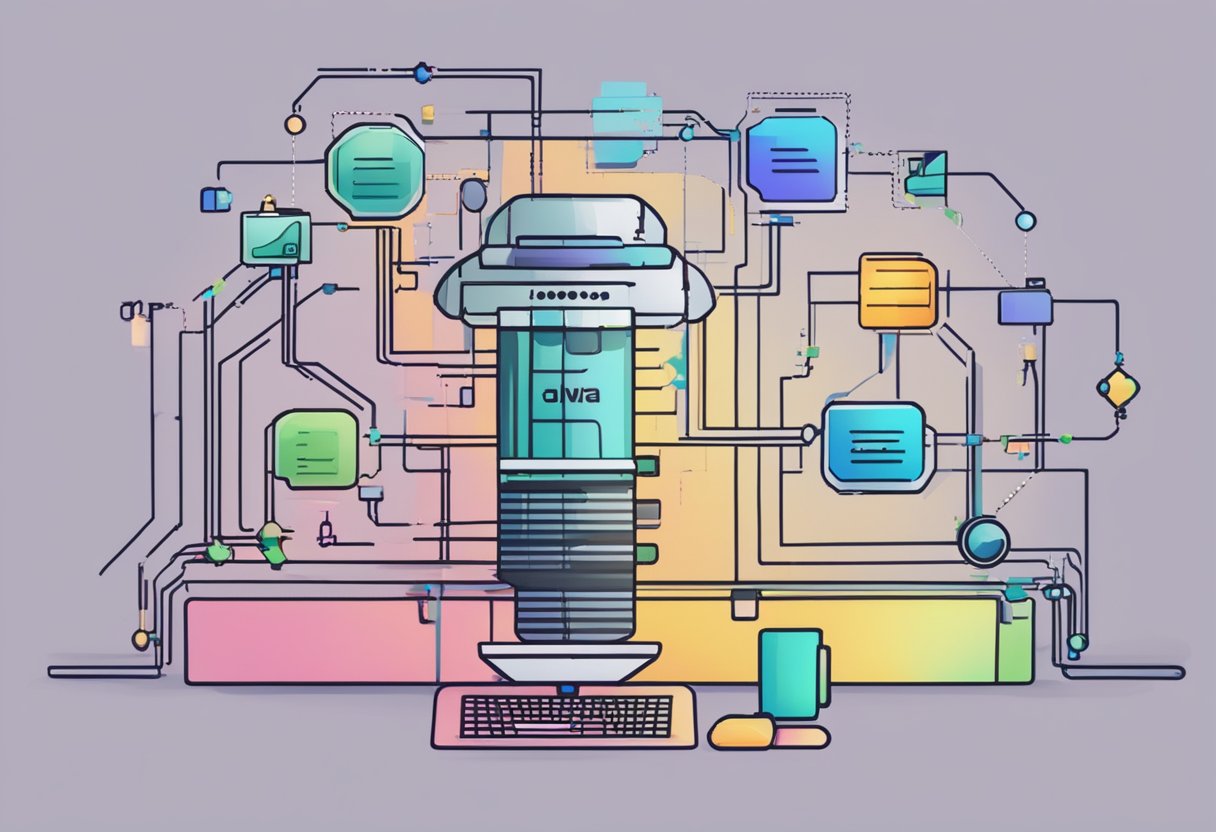
To understand how to convert JSON data to Java objects, it is important to first understand what JSON is. JSON is a text-based format that is used to represent data objects. It is similar to XML but is much simpler and easier to read. JSON data is made up of key-value pairs, where the key is a string and the value can be a string, number, boolean, null, array, or another JSON object.
With the release of Java 8, working with JSON data has become much easier. Java 8 introduced a new package called java.util.stream that provides a functional programming style of working with collections. This package includes a new class called Collectors that provides a set of predefined collectors for common operations such as grouping, filtering, and mapping. These collectors can be used to convert JSON data to Java objects in a concise and readable way.
Key Takeaways
- JSON is a lightweight data-interchange format that is used to represent data objects.
- Java 8 introduced a new package called java.util.stream that provides a functional programming style of working with collections, making it easier to work with JSON data.
- The Collectors class in Java 8 provides a set of predefined collectors that can be used to convert JSON data to Java objects in a concise and readable way.
Understanding JSON
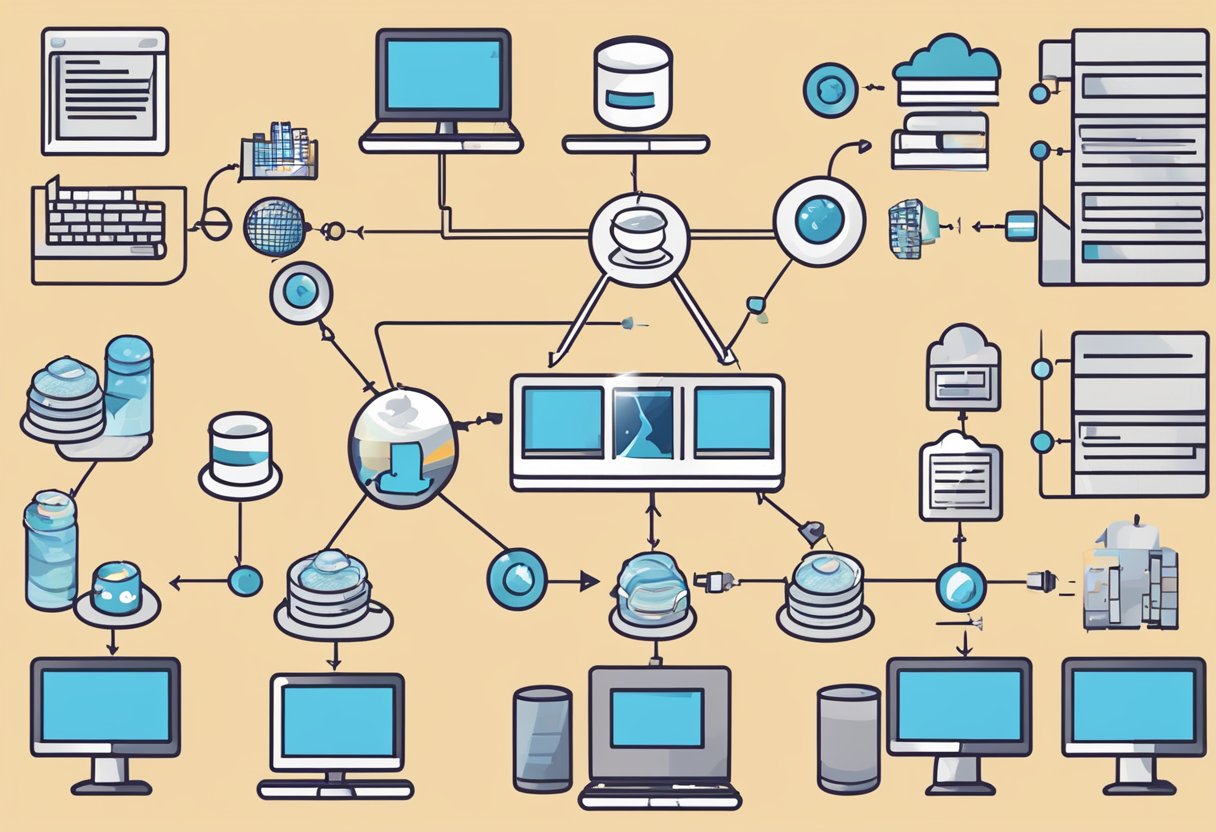
In order to understand how to convert JSON to Java objects, we first need to understand what JSON is.
JSON stands for JavaScript Object Notation and is a lightweight data-interchange format. It is easy to read and write and is language-independent. This means that JSON can be used with any programming language.
JSON data is represented as key-value pairs, where the key is a string and the value can be a string, number, boolean, null, array, or another JSON object. Here is an example of JSON data:
{
"name": "John Doe",
"age": 30,
"isMarried": false,
"hobbies": ["reading", "hiking", "traveling"],
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345"
}
}
In this example, the key-value pairs represent information about a person, including their name, age, marital status, hobbies, and address. The hobbies value is an array, while the address value is another JSON object.
JSON is commonly used for client-server communication, as it is lightweight and easy to parse. It is also used for storing and exchanging data between applications.
Now that we have a basic understanding of JSON, we can move on to how to convert JSON data to Java objects.
Java 8 and JSON
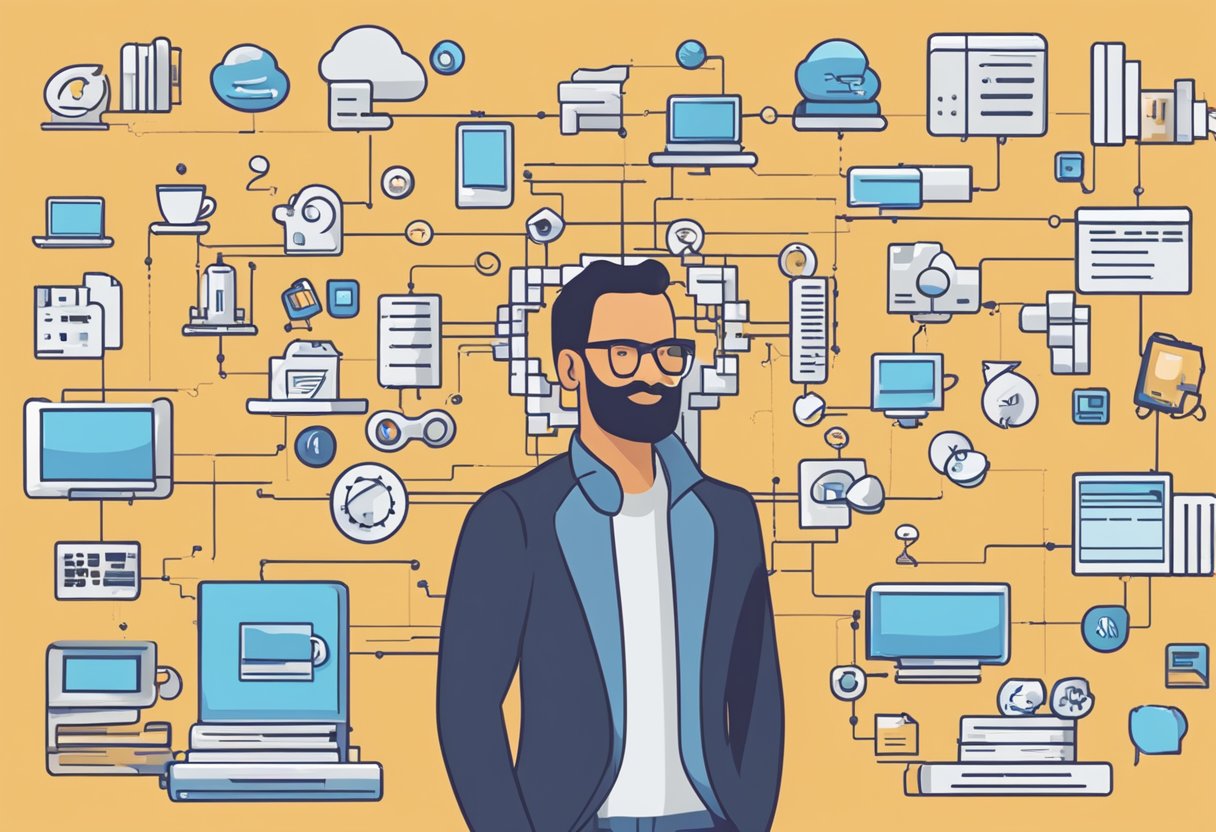
In Java 8, working with JSON data has become even easier thanks to the introduction of new features and libraries. Here are some key points to keep in mind when using Java 8 with JSON:
- Stream API: Java 8’s Stream API can be used to process JSON data efficiently. By converting a JSON string into a stream of objects, we can perform operations such as filtering, mapping, and reducing on the data.
- Jackson: Jackson is a popular Java library for working with JSON data. With its support for Java 8 features such as lambdas and streams, Jackson makes it easy to convert JSON data to Java objects and vice versa.
- Gson: Gson is another popular Java library for working with JSON data. While it doesn’t have the same level of Java 8 support as Jackson, it’s still a powerful library that can handle complex JSON structures with ease.
- Java 8 Date/Time API: Java 8’s Date/Time API can be used to parse and format dates and times in JSON data. With its support for ISO-8601 formats and time zones, the Date/Time API makes it easy to work with date and time data in JSON.
- Functional Interfaces: Java 8’s functional interfaces can be used to simplify the processing of JSON data. By defining custom functional interfaces, we can create reusable code that can be used across different parts of our application.
Overall, Java 8 provides a powerful set of tools for working with JSON data. With its support for lambdas, streams, and functional interfaces, Java 8 makes it easy to process and manipulate JSON data in a concise and efficient manner.
Converting JSON to Java Object in Java 8
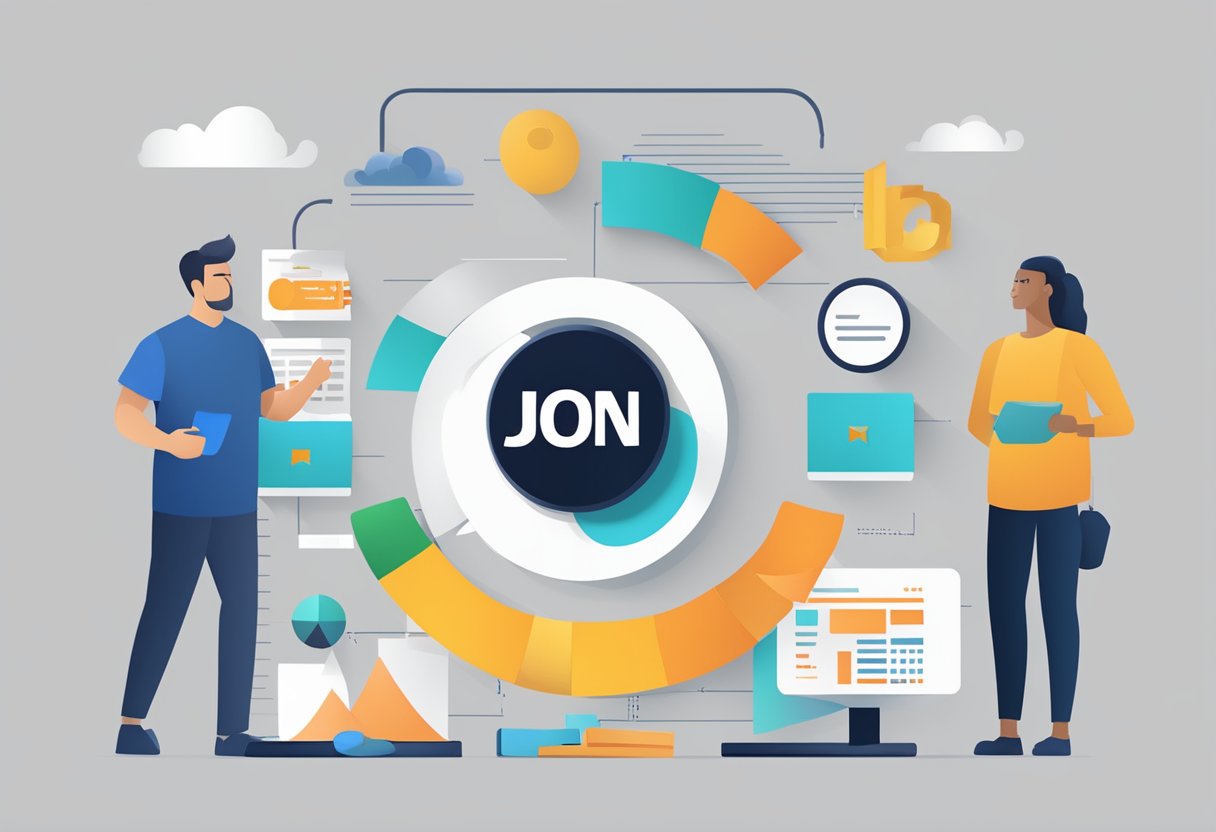
In this section, we will discuss how to convert JSON to Java Object using Java 8. We will cover the following sub-sections: Importing Libraries, Creating Java Classes, and Parsing JSON.
Importing Libraries
Before we can start converting JSON to Java Object, we need to import the necessary libraries. In Java 8, we can use the Jackson library to convert JSON to Java Object. To import the Jackson library, we need to add the following dependency to our pom.xml file:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.5</version>
</dependency>
Creating Java Classes
To convert JSON to Java Object, we need to create Java classes that represent the JSON data. We can use the Jackson library to automatically generate Java classes from JSON data. To do this, we need to create a Java class with the same structure as the JSON data and annotate it with the @JsonProperty annotation. For example:
public class Person {
@JsonProperty("name")
private String name;
@JsonProperty("age")
private int age;
// getters and setters
}
Parsing JSON
Once we have our Java classes, we can use the ObjectMapper class from the Jackson library to convert JSON to Java Object. To do this, we can use the readValue() method and pass in the JSON data and the class we want to convert it to. For example:
String json = "{ \"name\": \"John\", \"age\": 30 }";
ObjectMapper objectMapper = new ObjectMapper();
Person person = objectMapper.readValue(json, Person.class);
In the code above, we first create a String that contains the JSON data. We then create an ObjectMapper object and use the readValue() method to convert the JSON data to a Person object.
In conclusion, converting JSON to Java Object in Java 8 is a simple process that can be accomplished using the Jackson library. We need to import the necessary libraries, create Java classes that represent the JSON data, and use the ObjectMapper class to parse the JSON data.
Common Challenges and Solutions
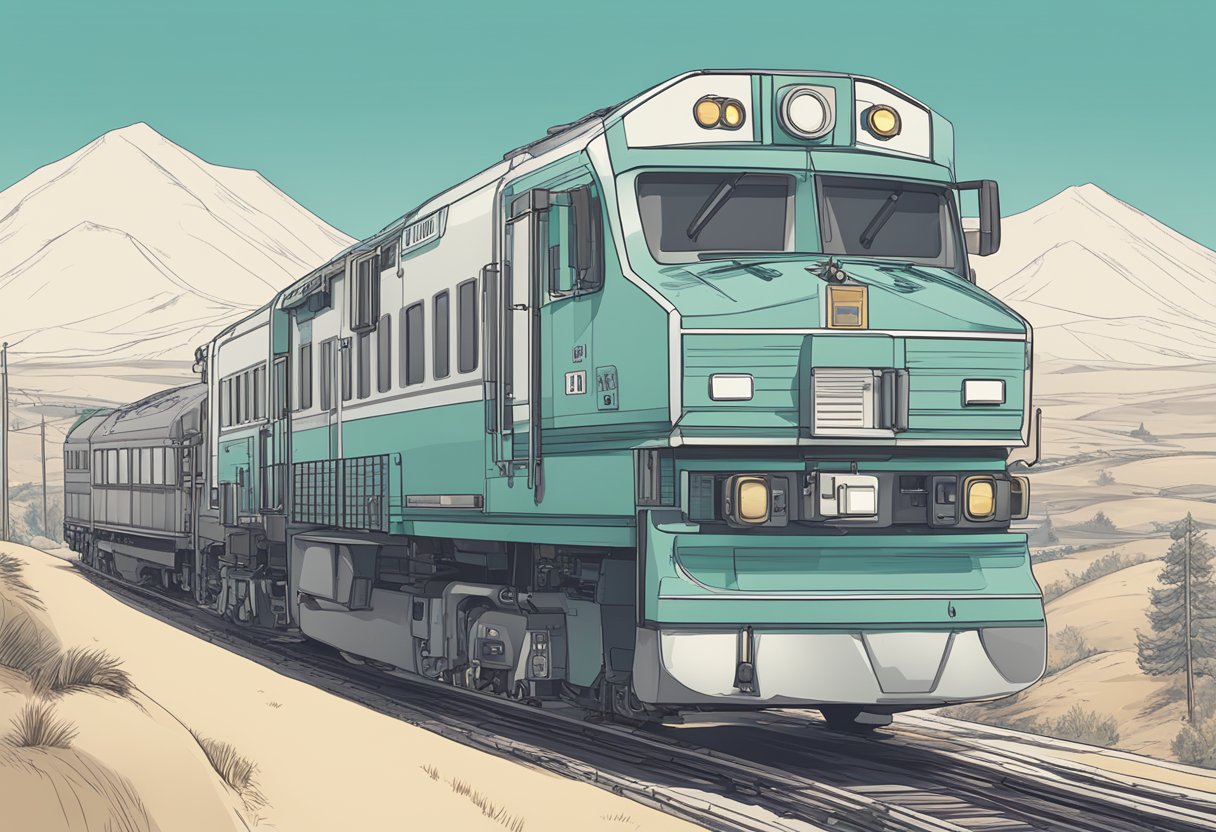
When working with JSON data in Java, there are some common challenges that developers may encounter. In this section, we will discuss some of these challenges and provide solutions for them.
Handling Nested JSON
One of the challenges when dealing with JSON data is handling nested objects. When parsing a JSON file, it is common to come across nested objects, which can be difficult to handle. To handle nested JSON objects, we can use a library like Gson or Jackson, which provide methods to convert JSON data to Java objects.
Gson provides a method called fromJson()
which can be used to convert a JSON string to a Java object. Jackson provides a similar method called readValue()
which can be used to convert a JSON string to a Java object. Both of these libraries also provide methods to handle nested objects.
To handle nested objects, we can create Java classes that correspond to the JSON structure. We can then use the library to convert the JSON data to Java objects, which can be easily manipulated.
Dealing with Large JSON Files
Another challenge when working with JSON data is dealing with large JSON files. When parsing a large JSON file, the application may run out of memory or take a long time to process the data. To deal with large JSON files, we can use a streaming API like Jackson.
Jackson provides a streaming API that allows us to process JSON data in a streaming fashion. Instead of loading the entire JSON file into memory, we can read the data in chunks and process it one piece at a time. This can help to reduce memory usage and improve performance.
To use the Jackson streaming API, we can create a JsonParser
object and use its next()
method to read the next JSON token. We can then process the token and continue reading the file until we reach the end.
In conclusion, handling nested JSON and dealing with large JSON files are common challenges when working with JSON data in Java. By using libraries like Gson and Jackson, and techniques like creating Java classes and using streaming APIs, we can overcome these challenges and process JSON data efficiently.
Best Practices for JSON to Java Object Conversion
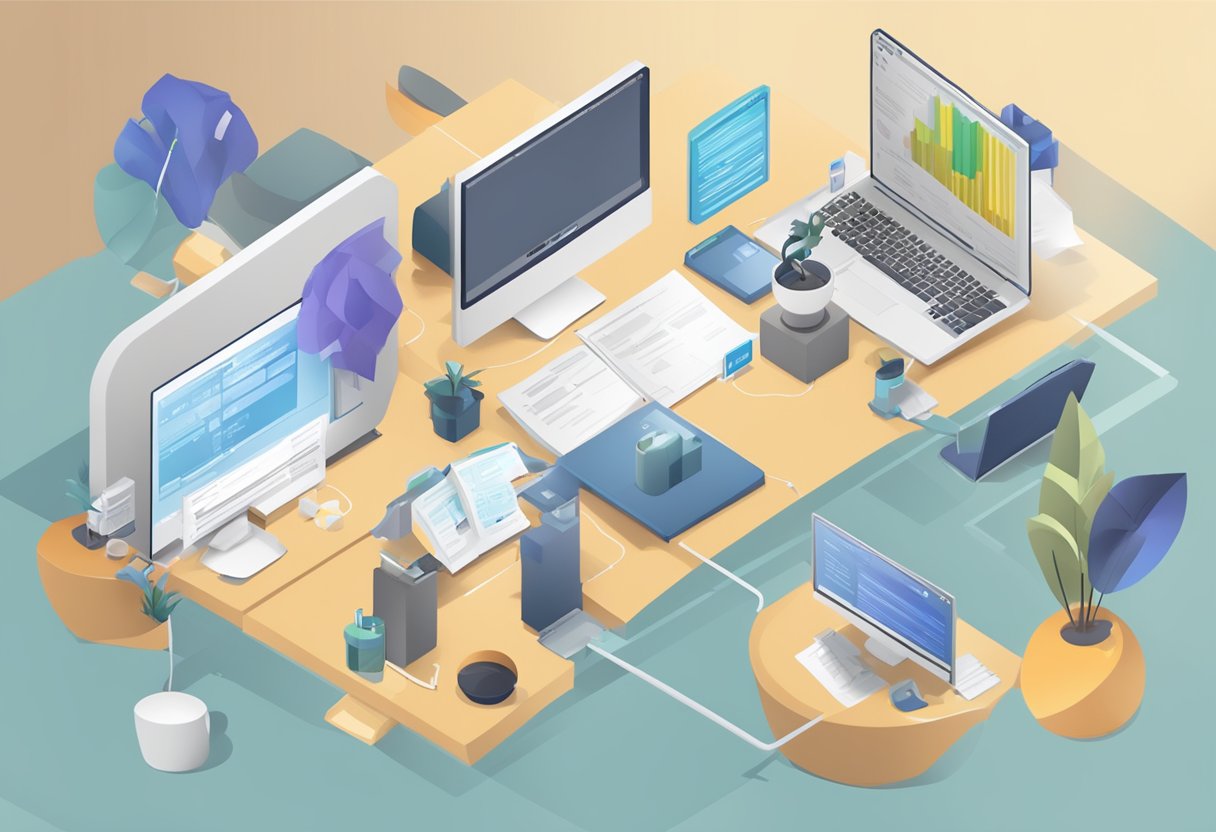
When converting JSON to Java objects, there are several best practices that we should follow to ensure that our code is efficient and maintainable. Here are some tips that we can keep in mind:
Use a JSON Library
Using a JSON library like Jackson, Gson, or Json-io can make the conversion process much easier and more efficient. These libraries provide methods for converting JSON data to Java objects and vice versa, and they handle many of the details of the conversion process for us. Additionally, they often offer features like data validation and error handling that can help us catch and handle errors more effectively.
Use Java Classes
When converting JSON data to Java objects, it’s important to use Java classes to represent the data. This allows us to take advantage of Java’s object-oriented programming features, which can make our code more organized and easier to maintain. Additionally, using Java classes can help us catch errors earlier in the development process by providing compile-time type checking.
Use Annotations
Many JSON libraries support annotations that can be used to customize the conversion process. For example, we can use annotations to specify the names of JSON fields that should be mapped to specific Java class properties, or to indicate that certain properties should be ignored during the conversion process. Using annotations can make our code more concise and easier to read, and can help us avoid errors that might occur if we had to manually specify all of the conversion details.
Handle Exceptions
When converting JSON data to Java objects, it’s important to handle exceptions that might occur during the process. For example, if the JSON data contains an unexpected value or if there is a problem with the conversion process, an exception might be thrown. By handling exceptions gracefully, we can ensure that our code doesn’t crash or behave unexpectedly, and we can provide more meaningful error messages to our users.
By following these best practices, we can ensure that our JSON to Java object conversion code is efficient, maintainable, and error-free.
Frequently Asked Questions
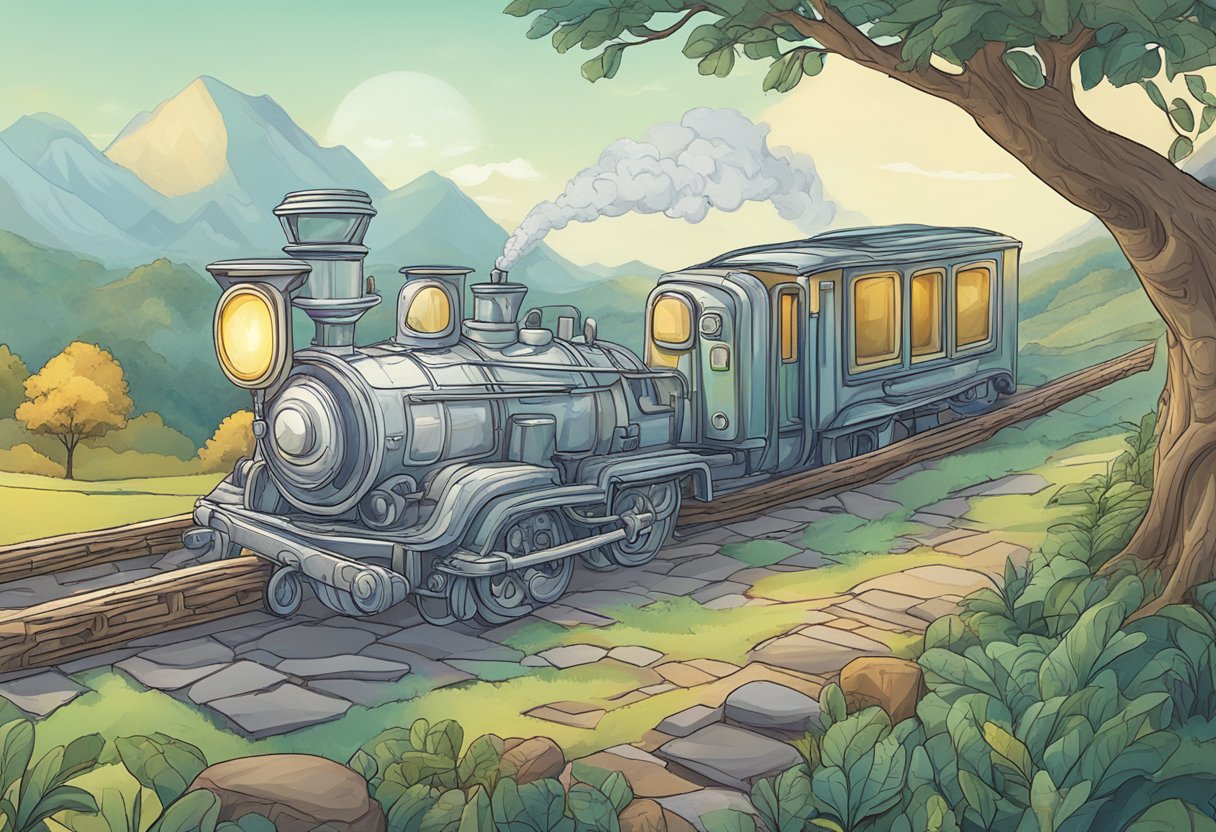
How to convert JSON to Java object in Spring Boot?
To convert JSON to Java object in Spring Boot, we can use the Jackson library. First, we need to add the dependency in the pom.xml file. Then, we can use the ObjectMapper
class to convert the JSON string to the Java object. Here is an example:
ObjectMapper objectMapper = new ObjectMapper();
MyClass myClass = objectMapper.readValue(jsonString, MyClass.class);
Convert JSON file to Java object
To convert a JSON file to a Java object, we can use the Jackson library. First, we need to read the content of the file and store it in a string. Then, we can use the ObjectMapper
class to convert the JSON string to the Java object. Here is an example:
File file = new File("path/to/json/file");
String jsonString = FileUtils.readFileToString(file, StandardCharsets.UTF_8);
ObjectMapper objectMapper = new ObjectMapper();
MyClass myClass = objectMapper.readValue(jsonString, MyClass.class);
Convert JSON to Java object dynamically
To convert JSON to Java object dynamically, we can use the Gson library. First, we need to create a JsonElement
object from the JSON string using the JsonParser
class. Then, we can use the Gson
class to convert the JsonElement
to the Java object. Here is an example:
String jsonString = "{\"name\":\"John\",\"age\":30}";
JsonParser parser = new JsonParser();
JsonElement jsonElement = parser.parse(jsonString);
Gson gson = new Gson();
MyClass myClass = gson.fromJson(jsonElement, MyClass.class);
How to create JSON object in Java 8?
To create a JSON object in Java 8, we can use the built-in JsonObject
class from the javax.json
package. Here is an example:
JsonObject jsonObject = Json.createObjectBuilder()
.add("name", "John")
.add("age", 30)
.build();
How to convert JSON string to JSON object in Java 8?
To convert a JSON string to a JSON object in Java 8, we can use the built-in JsonReader
class from the javax.json
package. Here is an example:
String jsonString = "{\"name\":\"John\",\"age\":30}";
JsonReader reader = Json.createReader(new StringReader(jsonString));
JsonObject jsonObject = reader.readObject();
How to iterate JSON object in Java 8?
To iterate a JSON object in Java 8, we can use the JsonArray
class from the javax.json
package. Here is an example:
JsonObject jsonObject = Json.createObjectBuilder()
.add("name", "John")
.add("age", 30)
.build();
JsonArray jsonArray = Json.createArrayBuilder().add(jsonObject).build();
for (JsonObject object : jsonArray.getValuesAs(JsonObject.class)) {
String name = object.getString("name");
int age = object.getInt("age");
System.out.println(name + " " + age);
}