When we talk about programming, we often focus on the creation and design of software. However, an equally important aspect is dealing with the unexpected issues that pop up while a program is running. These issues, known as runtime errors, can disrupt a smoothly running application and present challenges for developers. This article aims to shed light on what runtime errors are, how they can be debugged effectively, and strategies to prevent them from occurring in the first place.
Understanding Runtime Errors
What Is a Runtime Error in Programming?
A runtime error is a problem that occurs when a program is running. Unlike syntax errors, which are detected when the code is being written and are prevented from running, runtime errors sneak past the development phase and rear their heads during execution. Essentially, it means something went wrong while the program was active, causing it to stop unexpectedly or behave in unintended ways.
Runtime errors can be caused by various issues, such as attempting to divide a number by zero, failing to allocate enough memory for the program’s operations, or trying to access an array element that does not exist. These are issues that weren’t caught during coding because, in many cases, the program’s logic seemed correct at the time of writing.
For example, if a program is designed to calculate the average of a list of numbers but doesn’t account for the possibility that the list might be empty, attempting to run this calculation with an empty list will cause a division by zero error. This is a classic runtime error.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static double calculateAverage(List<Integer> numbers) {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return sum / numbers.size();
}
public static void main(String[] args) {
List<Integer> emptyList = new ArrayList<>();
double average = calculateAverage(emptyList);
System.out.println("Average of empty list: " + average);
}
}
Output = ERROR!
Exception in thread "main" java.lang.ArithmeticException: / by zero
at Main.calculateAverage(Main.java:10)
at Main.main(Main.java:15)
To handle runtime errors, programmers often use error-handling strategies like “try-catch” blocks. In such a structure, the “try” section contains code that might cause an error, and the “catch” section defines what to do if an error occurs. This way, instead of crashing, the program can perhaps display a helpful message to the user or attempt an alternative operation.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static double calculateAverage(List<Integer> numbers) {
try {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return sum / numbers.size();
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
return 0; // Default value or handle it as needed
}
}
public static void main(String[] args) {
List<Integer> emptyList = new ArrayList<>();
double average = calculateAverage(emptyList);
System.out.println("Average of empty list: " + average);
}
}
Output = ERROR!
Error: / by zero
Average of empty list: 0.0
Understanding and managing runtime errors are crucial for creating reliable and robust software. They remind us that foreseeing and planning for unexpected situations is a significant part of programming. By anticipating potential runtime errors and implementing preventive measures, developers can enhance the user experience and maintain the integrity of their programs.
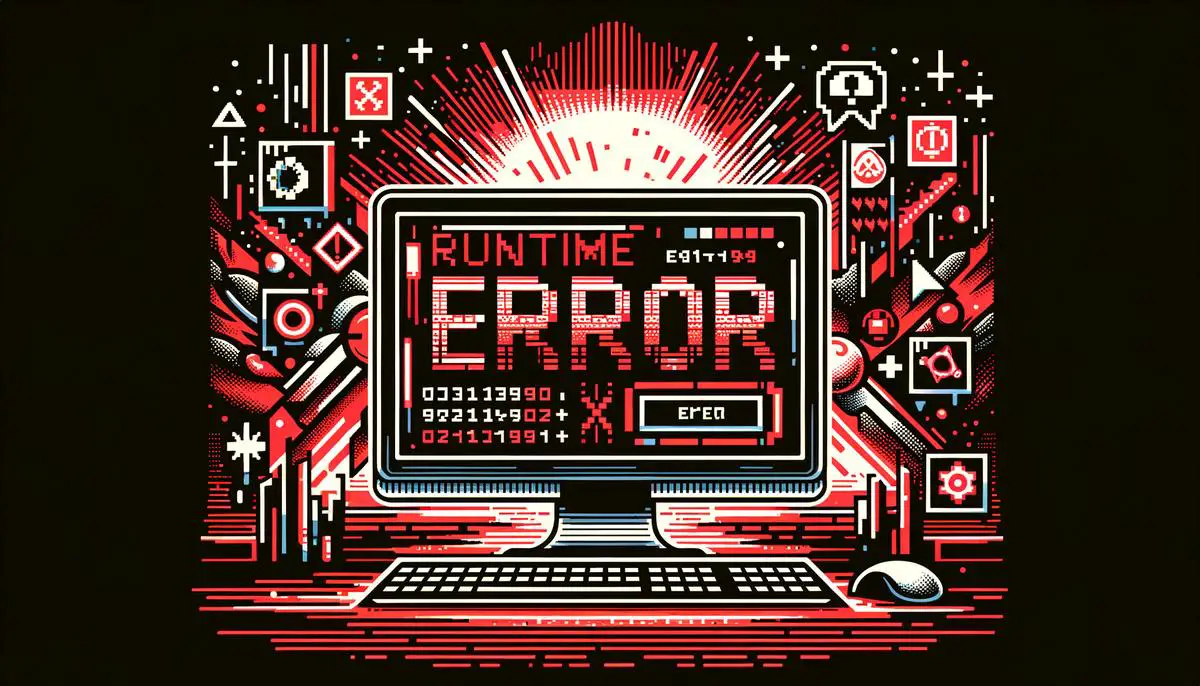
Debugging Techniques
Efficient Debugging of Runtime Errors
To adeptly navigate the landscape of runtime errors, programmers lean on a series of tried-and-true debugging steps. Acting as digital detectives, developers follow these phases to isolate and rectify elusive issues that only surface during execution. Embark on this journey of debugging with clarity and precision, embracing tools and techniques fashioned to turn confusion into code perfection.
1. Leverage Integrated Development Environments (IDEs): Modern IDEs are not just code editors; they are powerful allies in the quest to conquer runtime errors. Utilize their built-in debugging features, which highlight troublesome lines and offer real-time insights into variable states and memory use. Running your code within an IDE often points directly to the nefarious line causing your runtime woes.
2. Implement Logging Strategically: Insert logging statements at critical points in your application to track the flow of execution and variable states. This breadcrumbs approach allows you to trace back from the error to the original flaw in logic or data handling. Be methodical: start with broad sections and narrow down, focusing logs around suspected areas of failure.
3. Utilize Breakpoints: Breakpoints are a debugger’s best friend. By strategically placing these pause-points in your code, you force the execution to halt, enabling an examination of intermediate states of your application. Scrutinize variables and their transformations at each juncture to pinpoint where reality diverges from expectation.
4. Conduct Step-by-Step Execution: Once breakpoints bring your application to a pause, use step-over and step-into functionalities. This meticulous walkthrough of your code’s execution path illuminates hidden issues and clarifies the sequence of operations, revealing where and why failures occur.
5. Compare Expected versus Actual Output: Keep close tabs on the Output window of your IDE or debugging tool. Frequently, runtime errors throw specific exception messages that clue you into the nature of the problem, whether it be an out-of-bounds error or a null reference exception.
6. Enlist the Help of Peer Review: Two heads (or more) are better than one, especially when untangling complex runtime errors. A fresh pair of eyes might catch oversights or flawed assumptions that went unnoticed. Don’t underestimate the power of collaborative debugging.
7. Consult Documentation and Resources: Sometimes, runtime errors stem from misunderstandings or misuse of language features or third-party libraries. Dive into the official documentation or explore communities like Stack Overflow for insights and solutions from those who’ve wrestled with similar issues.
8. Test Rigorously with Different Data Sets: Certain runtime errors surface under specific conditions or with particular inputs. Broaden your testing scope with varied and boundary-pushing datasets to unearth these hidden bugs.
By incorporating these steps into your debugging routine, tearing down the barriers erected by runtime errors becomes less daunting. Remember, mastering the art of debugging is a recurrent learning process, equipping you over time to swiftly and efficiently resolve issues, ensuring your code runs smoothly and reliably. So, arm yourself with these strategies and face runtime errors with renewed confidence and skill.
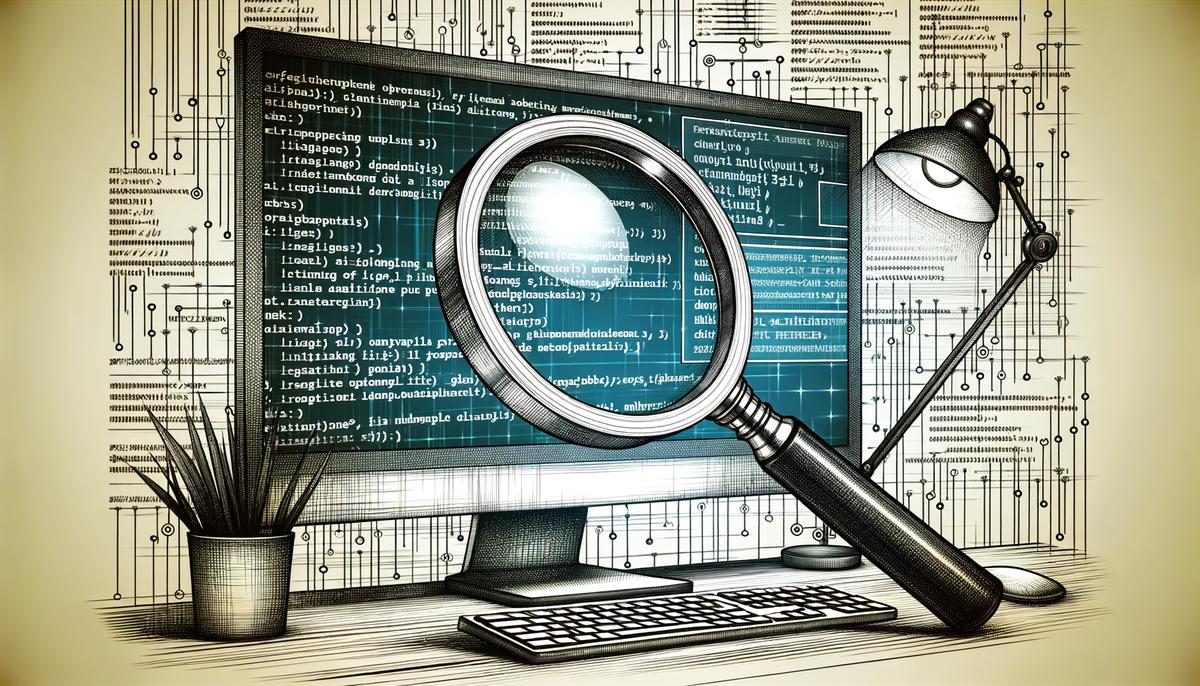
Preventive Strategies
Regular Code Review Sessions
Schedule regular code review sessions, where peers meticulously go through the written code. This allows for the identification of potential runtime errors which might not be evident to the original coder. Encourage a culture where feedback is seen as an opportunity for improvement rather than criticism.
Adopt Static Code Analysis Tools
Static code analysis tools help in scrutinizing the code without executing it. They efficiently detect problems that could lead to runtime errors, such as uninitialized variables, type mismatches, and more. Integrating these tools into the development process can catch issues early on.
Practice Defensive Programming
Defensive programming involves writing code that anticipates and safeguards against possible errors. For example, always check that variables have valid values before using them in operations. Consider worst-case scenarios and include checks or validations to handle those cases effectively.
Use Thorough and Diverse Testing Strategies
Don’t rely solely on one type of testing. Combine various methods such as unit tests, integration tests, and system tests to cover different aspects of the software. This comprehensive approach ensures that different components work individually and together as expected.
Implement Error Logging Strategically
While not a prevention method, effective error logging tracks when and where errors occur, providing valuable insights for correcting them. Ensure logs are detailed enough to identify the error context but not overwhelming to the point of being unusable.
Adopt Code Linters
Code linters are tools that automatically check for style violations, potentially dangerous constructs, and syntactic discrepancies in code. They can guide programmers towards best practices and away from patterns prone to errors.
Understand the Domain Well
A deep understanding of the problem domain enables developers to foresee and mitigate common runtime issues specific to the domain. Tailor error prevention strategies to the nuances of the application’s environment.
Stay Updated on Best Practices
Programming languages and technologies evolve, bringing new best practices and paradigms for error prevention. Stay informed on the latest developments in the programming community to adopt more effective strategies over time.
By knitting these strategies into the fabric of the development process, teams can significantly reduce the occurrence of runtime errors, leading to more robust and reliable applications.
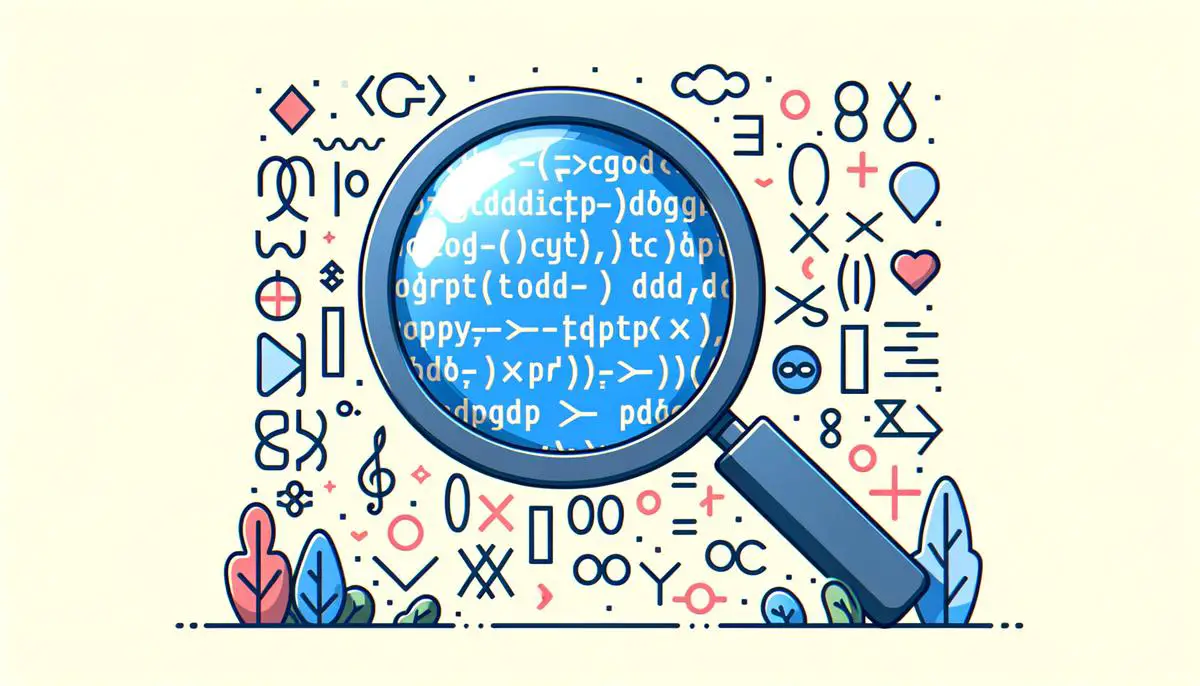
Mastering the art of handling runtime errors is not just about fixing what’s broken; it’s about fostering an environment where such errors are less likely to occur. By embracing error prevention strategies and honing debugging skills, developers can create more reliable and robust applications. This commitment to quality not only improves the user experience but also enhances the developer’s ability to tackle challenges head-on with confidence. Ultimately, understanding and managing runtime errors is a crucial step towards achieving excellence in programming.