Java, a versatile programming language, offers robust tools for developers. Yet, the journey isn’t always smooth. In this article, we’ll dive deeper into the world of Java exceptions, exploring not only theoretical concepts but also providing hands-on code examples for a clearer understanding.
Understanding Java exceptions is crucial for any developer. Exceptions are errors that occur during the execution of a program and can disrupt its normal flow. They can be caused by various factors, such as invalid input, resource unavailability, or programming mistakes.
To resolve a Java exception, the first step is to identify the type of exception that occurred. Java provides a wide range of exception classes, each designed to handle specific types of errors. By understanding the exception hierarchy and knowing which exceptions to catch, developers can effectively handle errors and prevent program crashes.
Once the exception type is identified, the next step is to analyze the exception message and stack trace. The exception message provides valuable information about the error, while the stack trace shows the sequence of method calls that led to the exception. By carefully examining these details, developers can pinpoint the root cause of the exception and take appropriate actions to resolve it.
In the upcoming sections, we’ll explore common types of Java exceptions and their causes, along with practical examples of how to handle them. By unraveling the mystery of Java exceptions, developers can become more proficient in troubleshooting and resolving errors, ultimately improving the reliability and stability of their Java applications. So let’s dive in and unravel the mystery of Java exceptions together.
I. Introduction
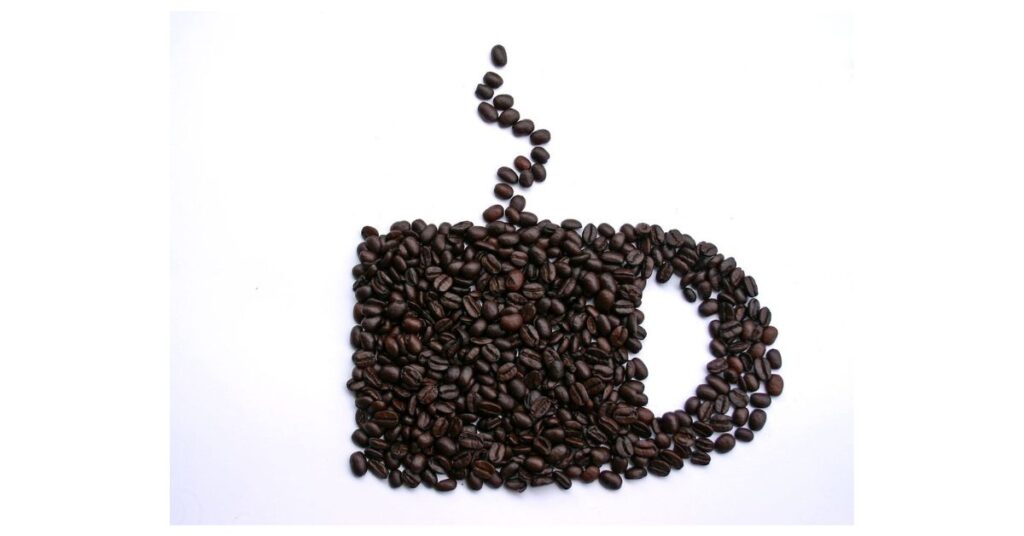
Java exceptions are an integral part of programming, but they can often be puzzling and challenging to handle. Resolving Java exceptions is crucial for maintaining stable and reliable code. In this section, we will delve into the mystery of Java exceptions and explore effective strategies for resolving them.
To begin our journey, we will combine theoretical concepts with practical code examples. By understanding the underlying principles of Java exceptions, we can better grasp how to tackle them when they arise. We will explore different types of exceptions, such as checked and unchecked exceptions, and learn how to handle them appropriately.
Additionally, we will discuss common techniques for debugging and troubleshooting exceptions. This includes using exception stack traces to identify the root cause of the issue and employing try-catch blocks to gracefully handle exceptions in our code.
Throughout this section, we will emphasize the importance of proactive exception handling and best practices for writing robust and resilient code. By the end of our exploration, you will have a clearer understanding of how to unravel the mystery of Java exceptions and confidently resolve them in your own programming endeavors. So, let’s dive in and demystify the world of Java exceptions together.
II. Understanding Java Exceptions
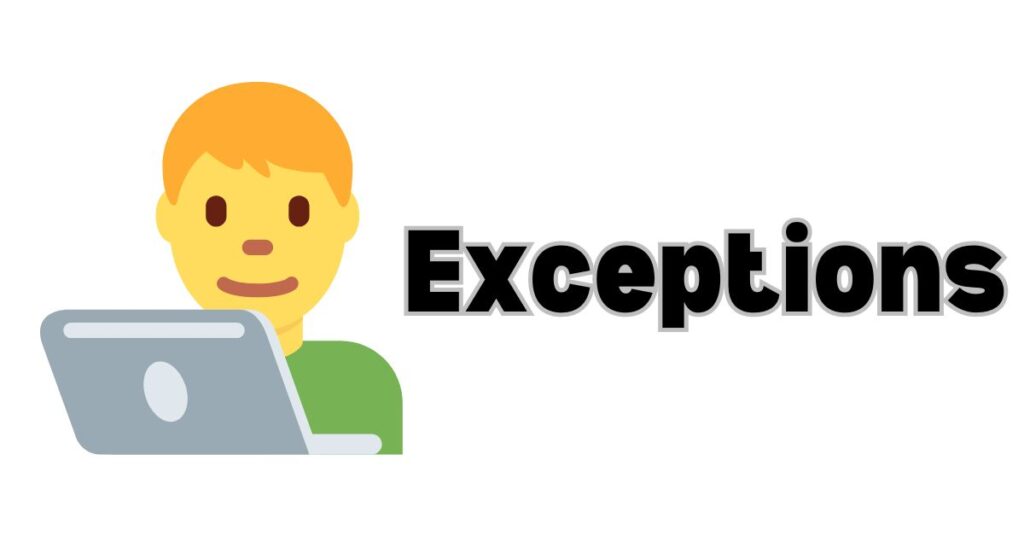
Definition of Java Exceptions
An exception in Java is a crucial event that can disrupt the regular execution of a program. When an exception occurs, it interrupts the normal flow of the program and can lead to unexpected behavior or errors. Understanding Java exceptions is essential for developers to effectively handle and resolve them.
Java exceptions are objects that represent exceptional conditions or errors that occur during the execution of a program. These exceptions can be caused by various factors, such as invalid input, resource unavailability, or programming errors. By throwing and catching exceptions, developers can handle these exceptional conditions and ensure that their programs continue to run smoothly.
One of the key concepts in understanding Java exceptions is the try-catch block. Within a try block, developers can write the code that may potentially throw an exception. If an exception occurs, it is caught by the catch block, which contains the code to handle the exception. This allows developers to gracefully handle errors and prevent their programs from crashing.
By understanding the different types of exceptions in Java, developers can anticipate potential issues and implement appropriate error-handling mechanisms. Java provides a hierarchy of exception classes, with the base class being the Exception class. This hierarchy allows for more specific exception handling based on the type of error encountered.
Consider this code snippet:
try {
// Code that may throw an exception
} catch (Exception e) {
// Handle the exception
System.out.println("An exception occurred: " + e.getMessage());
}
Here, we encapsulate the potentially problematic code within a try
block. If an exception occurs, the catch
block is executed to handle it gracefully.
Different Types of Exceptions
Java exceptions fall into two categories: checked and unchecked. A checked exception must be handled explicitly, while an unchecked exception can be left unhandled. For example:
// Checked Exception
try {
FileInputStream file = new FileInputStream("example.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
// Unchecked Exception
int result = 10 / 0; // ArithmeticException
III. Common Java Exception Messages
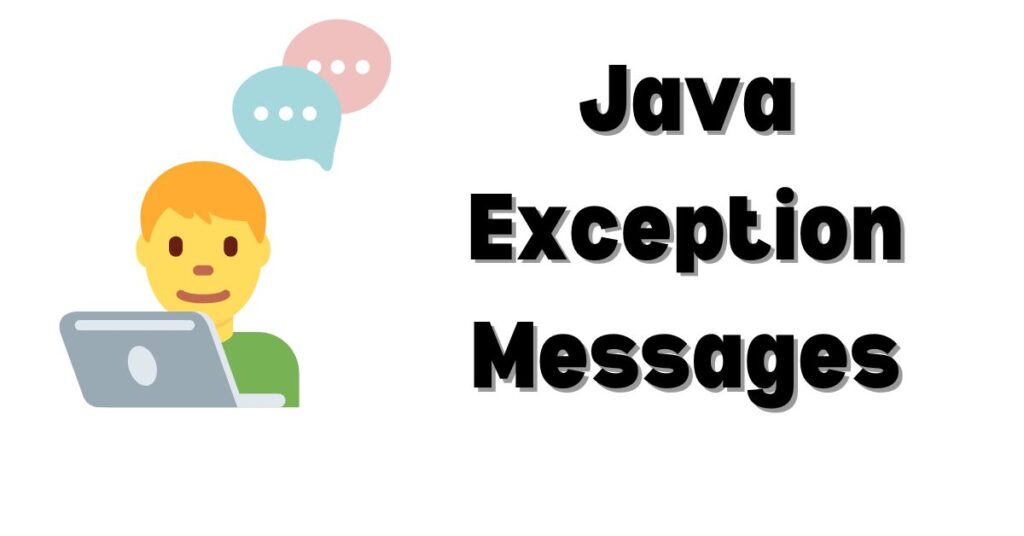
Understanding error messages is crucial for effective debugging. Let’s decipher a common error message:
NullPointerException: Attempt to invoke a method on a null object reference.
This message indicates an attempt to use a method on an object that is currently null
. Recognizing these messages accelerates the troubleshooting process.
IV. Identifying the Cause
Strategies to Identify the Root Cause
When an exception occurs, identifying its origin is vital. Employ logging or print statements strategically:
try {
// Code that may throw an exception
} catch (Exception e) {
// Log the exception details
logger.error("Exception occurred: " + e.getMessage());
// Print stack trace for detailed analysis
e.printStackTrace();
}
Importance of Debugging in Resolving Exceptions
Debugging tools like breakpoints and watches are indispensable. Set breakpoints at critical points to inspect variables and trace the flow:
public class Example {
public static void main(String[] args) {
int result = divideNumbers(10, 0);
System.out.println("Result: " + result);
}
private static int divideNumbers(int numerator, int denominator) {
// Set a breakpoint on the next line
return numerator / denominator;
}
}
V. Handling Exceptions Effectively
Implementing Try-Catch Blocks
A fundamental approach is using try-catch blocks. This example demonstrates handling a specific exception:
try {
int result = divideNumbers(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero: " + e.getMessage());
}
Using the ‘Throws’ Clause for Exception Handling
When a method can’t handle an exception internally, it can be declared using the ‘throws’ clause:
public void readFile(String filename) throws FileNotFoundException {
FileInputStream file = new FileInputStream(filename);
// Further code for reading the file
}
VI. Best Practices for Exception Handling
Writing Clean and Efficient Code
Preventative measures are paramount. Check for null values before invoking methods:
String value = // some computation or input;
if (value != null) {
// Safely invoke methods on 'value'
value.length();
}
Utilizing Specific Exception Handling for Different Scenarios
Tailor your approach based on the type of exception. Here, we handle different exceptions differently:
try {
// Code that may throw various exceptions
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage());
} catch (Exception e) {
System.out.println("An unexpected exception occurred: " + e.getMessage());
}
VII. Exception Handling Tools and Techniques
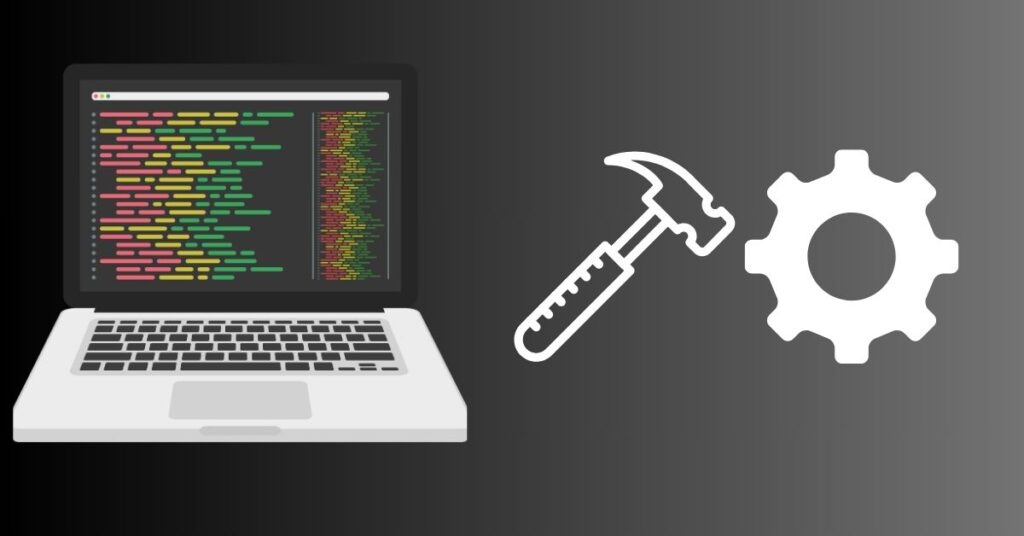
Overview of Tools Available for Exception Tracking
Tools like Log4j and Sentry provide valuable insights into exceptions. Configure Log4j in your project for detailed logs:
import org.apache.log4j.Logger;
public class Example {
private static final Logger logger = Logger.getLogger(Example.class);
public static void main(String[] args) {
try {
// Code that may throw an exception
} catch (Exception e) {
logger.error("Exception occurred: " + e.getMessage());
}
}
}
Advanced Techniques for Handling Complex Exceptions
For complex scenarios, consider using aspect-oriented programming (AOP) with frameworks like AspectJ. This allows you to separate exception-handling concerns from regular code:
public aspect ExceptionAspect {
pointcut handleExceptions():
execution(* com.example..*(..)) && (throws(Exception));
after() throwing(Exception e): handleExceptions() {
// Custom exception handling logic
System.out.println("Exception handled: " + e.getMessage());
}
}
VIII. Real-world Examples
Practical Examples of Resolving Java Exceptions
Let’s dive into practical examples:
Example 1: Handling File Not Found Exception
try {
FileInputStream file = new FileInputStream("example.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
Example 2: Division with Exception Handling
try {
int result = divideNumbers(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero: " + e.getMessage());
}
private static int divideNumbers(int numerator, int denominator) {
return numerator / denominator;
}
IX. Proactive Exception Prevention
Coding Practices to Minimize the Occurrence of Exceptions
Prevention is the best strategy. Validate inputs and anticipate potential issues:
public class StringUtils {
public static int safeStringLength(String value) {
return value == null ? 0 : value.length();
}
}
Writing Robust and Error-Resistant Code
Robust code anticipates issues and handles them gracefully:
public class MathUtils {
public static int safeDivision(int numerator, int denominator) {
return denominator != 0 ? numerator / denominator : 0;
}
}
X. Staying Updated with Java Exception Handling
The Importance of Keeping Up with Java Updates
Regular updates bring enhancements. Stay informed about the latest Java releases:
// Check your current Java version
System.out.println("Java Version: " + System.getProperty("java.version"));
New Features and Improvements in Exception Handling
Explore new features. In Java 17, a new method isEmpty()
is introduced for Optional:
Optional<String> optionalValue = // some optional value;
if (optionalValue.isEmpty()) {
System.out.println("Optional is empty");
} else {
System.out.println("Value: " + optionalValue.get());
}
XI. Troubleshooting Common Issues
Addressing Common Pitfalls in Exception Resolution
Common pitfalls include neglecting null checks. Address them:
String value = // some computation or input;
if (value != null) {
// Safely invoke methods on 'value'
value.length();
}
Tips for Efficient Troubleshooting
Efficiency is key. Utilize logging for detailed insights:
try {
// Code that may throw an exception
} catch (Exception e) {
logger.error("Exception occurred: " + e.getMessage());
e.printStackTrace();
}
XII. Community Support and Resources
Leveraging Online Forums and Communities for Assistance
Online communities are invaluable. Platforms like Stack Overflow offer a wealth of knowledge:
// When in doubt, seek community assistance
// Stack Overflow: https://stackoverflow.com/
Recommended Resources for Learning More about Java Exceptions
Expand your knowledge with quality resources:
// Books
// - "Effective Java" by Joshua Bloch
// - "Java Concurrency in Practice" by Brian Goetz
// Online Courses
// - Coursera: "Java Programming and Software Engineering Fundamentals"
// - Udemy: "Complete Java Masterclass"
// Tutorials
// - Baeldung (https://www.baeldung.com/): In-depth Java tutorials
XIV. Conclusion
In conclusion, resolving Java exceptions demands a combination of theoretical understanding and hands-on experience. By delving into code examples, identifying best practices, and leveraging community support, you empower yourself to navigate the complex landscape of exception resolution.
XV. FAQs
- Can all Java exceptions be prevented?
- While not all exceptions can be prevented, adopting best coding practices significantly reduces the likelihood of encountering them.
- What role does debugging play in exception resolution?
- Debugging is crucial for identifying the root cause of exceptions, enabling developers to address issues at their source.
- How often should I update my Java version for better exception handling?
- Regular updates are recommended to leverage the latest features and improvements in Java exception handling.
- Are there tools specifically designed for tracking and resolving Java exceptions?
- Yes, there are several tools tailored for efficient tracking and resolution of Java exceptions.
- Can community support really help in resolving complex exceptions?
- Absolutely. Community support provides diverse perspectives and shared experiences, often leading to innovative solutions.
Java 5 vs Java 6 vs Java 7 vs Java 8: Detailed Comparison