Using toLocaleString() for Currency Formatting
The toLocaleString()
method is a built-in JavaScript tool that’s perfect for converting numbers into neatly formatted currency strings. This method allows customization using locales and options parameters, making it a versatile choice.
const number = 123456.789;
const formattedNumber = number.toLocaleString('en-US', {
style: 'currency',
currency: 'USD',
});
console.log(formattedNumber); // Output: $123,456.79
In this example, the locale 'en-US'
designates the United States, and the style
is set to 'currency'
to indicate currency formatting. The currency
option is defined as 'USD'
, representing U.S. Dollars.
To adjust the number of decimal places, use minimumFractionDigits
and maximumFractionDigits
:
const number = 123456.789;
const formattedNumber = number.toLocaleString('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2,
maximumFractionDigits: 2,
});
console.log(formattedNumber); // Output: $123,456.79
This approach ensures the number is formatted with exactly two decimal places.
For different locales, simply change the locale string. Here’s how you can switch it to German formatting:
const number = 123456.789;
const formattedNumber = number.toLocaleString('de-DE', {
style: 'currency',
currency: 'EUR',
});
console.log(formattedNumber); // Output: 123.456,79 €
Here, 'de-DE'
denotes the German locale, and 'EUR'
represents Euros.
The flexibility of toLocaleString()
extends beyond locales and basic currency formatting. It includes intricate adjustments, making your web content more accessible and accurate across global audiences.
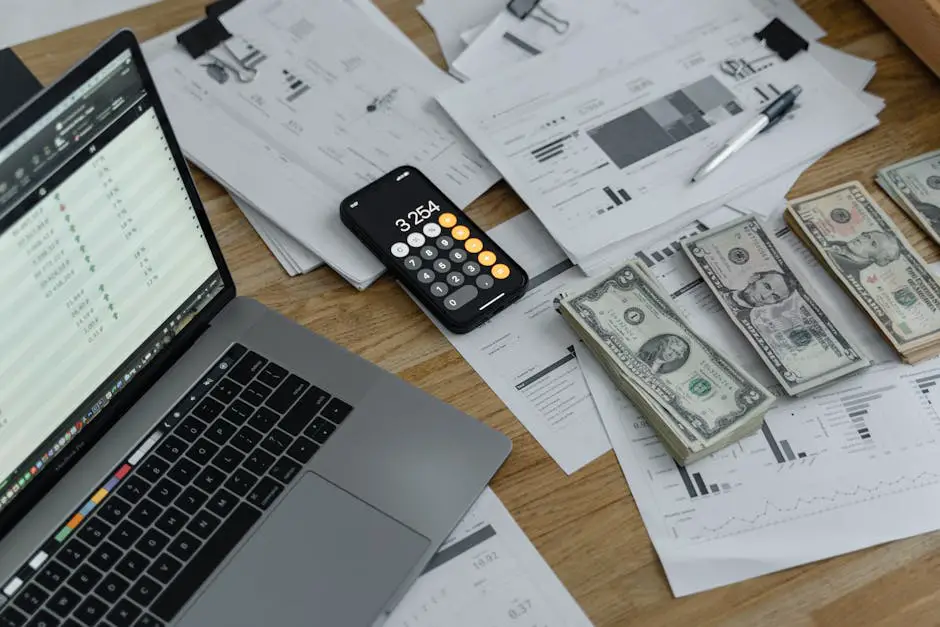
Intl.NumberFormat Constructor
The Intl.NumberFormat
constructor is another powerful tool within the ECMAScript Internationalization API, designed to handle number formatting with comprehensive locale and style options. This constructor enables you to format numbers seamlessly into various styles such as currency, percentage, and more, ensuring global compatibility and readability.
Here’s a basic example of how to use the Intl.NumberFormat
constructor for formatting numbers as currency.
const number = 123456.789;
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD'
});
const formattedNumber = formatter.format(number);
console.log(formattedNumber); // Output: $123,456.79
In this instance, 'en-US'
specifies the locale for the United States, and the style
is set to 'currency'
. The currency
option indicates the currency type, in this case, U.S. Dollars ('USD'
).
To customize the number of decimal places displayed, you can specify the minimumFractionDigits
and maximumFractionDigits
options:
const number = 123456.789;
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2,
maximumFractionDigits: 2
});
const formattedNumber = formatter.format(number);
console.log(formattedNumber); // Output: $123,456.79
The two options above ensure consistent precision by forcing the formatted output to show exactly two decimal places.
For those working with different currency locales, the Intl.NumberFormat
constructor allows easy localization adjustments. Here’s how to format the same number for the German locale using Euros:
const number = 123456.789;
const formatter = new Intl.NumberFormat('de-DE', {
style: 'currency',
currency: 'EUR'
});
const formattedNumber = formatter.format(number);
console.log(formattedNumber); // Output: 123.456,79 €
In this example, 'de-DE'
is the locale string for Germany, and 'EUR'
specifies the Euro currency. The constructor handles all the intricacies of locale-specific formatting, offering a hassle-free solution.
Intl.NumberFormat
isn’t limited to just currency. You can format numbers into percentages and other units as well. Here’s how to format a number as a percentage:
const number = 0.89;
const formatter = new Intl.NumberFormat('en-US', {
style: 'percent',
minimumFractionDigits: 2,
maximumFractionDigits: 2
});
const formattedNumber = formatter.format(number);
console.log(formattedNumber); // Output: 89.00%
In the above code, the style
is set to 'percent'
, which converts the fraction 0.89 into a percentage, formatted to two decimal places.
If you need to format numbers with units such as liters, you can utilize the unit
and unitDisplay
options:
const number = 45;
const formatter = new Intl.NumberFormat('en-US', {
style: 'unit',
unit: 'liter',
unitDisplay: 'long'
});
const formattedNumber = formatter.format(number);
console.log(formattedNumber); // Output: 45 liters
Here, style
is set to 'unit'
, and unit
specifies the type of unit, which is 'liter'
in this case. unitDisplay
controls how the unit is displayed, with options such as 'long'
, 'short'
, or 'narrow'
.
By leveraging Intl.NumberFormat
, you gain precise and locale-aware control over number formatting, making your applications more adaptable and user-friendly across diverse regions.
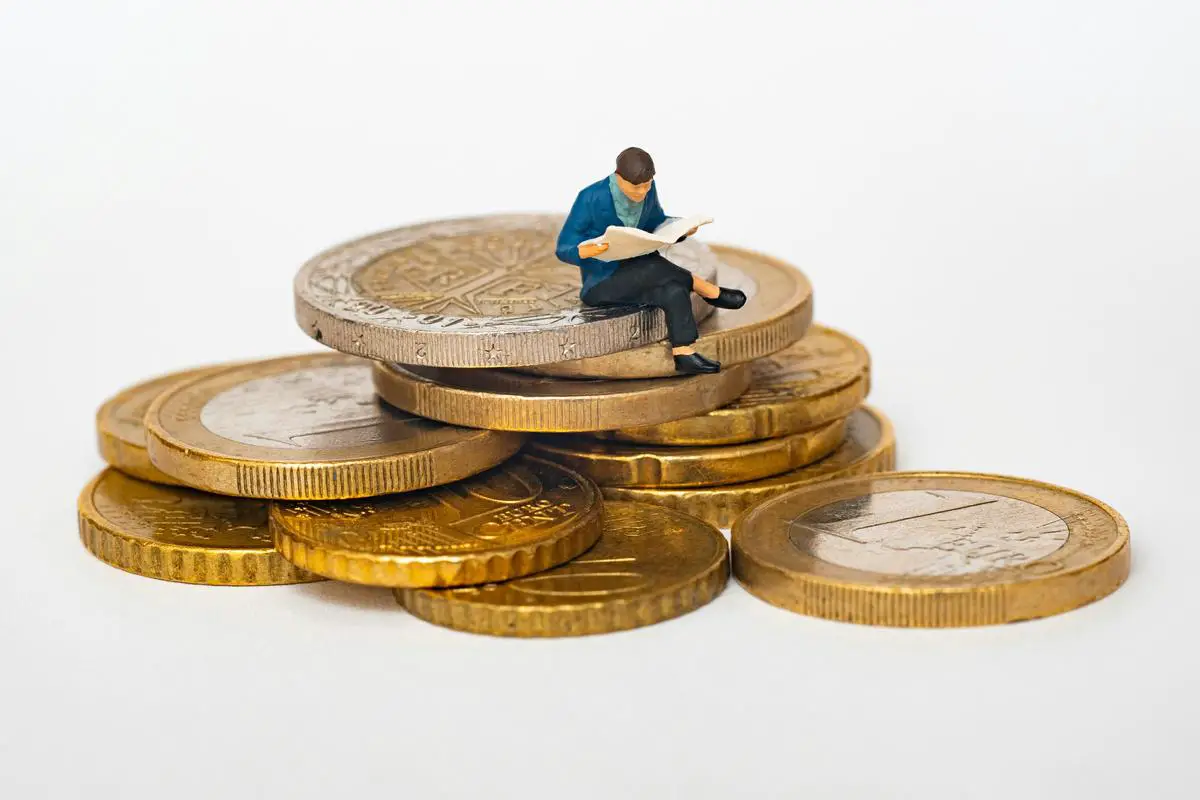
Photo by mathieustern on Unsplash
Handling Large Numbers with Intl.NumberFormat
By employing the Intl.NumberFormat
, you can accurately format very large numbers by utilizing strings or BigInt values, thus ensuring precision and accuracy without compromising critical data. JavaScript, for large integers, suffers from precision loss especially when they exceed the Number.MAX_SAFE_INTEGER
, but with Intl.NumberFormat
, this issue is proficiently handled.
Consider the case where you are formatting a substantial monetary value. Here’s how to do it using a string representation to avoid precision loss:
const largeNumberString = "987654321987654321";
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD'
});
const formattedNumber = formatter.format(largeNumberString);
console.log(formattedNumber); // Output: $987,654,321,987,654,321.00
In this code snippet, we used largeNumberString
to represent an exceptionally large number, and Intl.NumberFormat
handled it seamlessly, displaying the formatted currency string with complete accuracy.
Alternatively, when working with really large integers, JavaScript’s BigInt
can be leveraged to retain precision:
const largeNumberBigInt = 987654321987654321n;
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD'
});
const formattedNumber = formatter.format(largeNumberBigInt);
console.log(formattedNumber); // Output: $987,654,321,987,654,321.00
Here, the number 987654321987654321
is represented as a BigInt (noted by the ‘n’ suffix), and Intl.NumberFormat
accurately formats it without losing precision.
Another compelling example can be the formatting of scientific notations, which encompasses extraordinarily large figures:
const largeScientificNumber = "1e+110";
const formatter = new Intl.NumberFormat('en-US');
const formattedNumber = formatter.format(largeScientificNumber);
console.log(formattedNumber); // Output: 1e+110
With Intl.NumberFormat
, even numbers represented in scientific notation preserve their integrity and are displayed as intended.
When implementing Intl.NumberFormat
for handling large numbers, ensure to exploit the full flexibility provided by the options
attribute. This enhances the accuracy of the formatted output:
const largeNumber = "123456789123456789123456789";
const formatter = new Intl.NumberFormat('en-US', {
notation: 'compact',
compactDisplay: 'short'
});
const formattedNumber = formatter.format(largeNumber);
console.log(formattedNumber); // Output: 123T
In this example, the notation
is set to compact
, and compactDisplay
set to short
, resulting in an abbreviated form suited for presenting large numbers concisely.
To summarize, Intl.NumberFormat
provides a strong mechanism for handling massive numerals efficiently and precisely, catering to a broad range of formatting needs.
Custom Currency Formatting Using Regular Expressions
Regular expressions offer a highly customizable approach to currency formatting, providing control over thousands separators and decimal points. This method is particularly beneficial when built-in methods don’t meet specific formatting requirements.
Using regular expressions, you can enhance your formatting to suit diverse and unique standards. Here’s an example where we format a number with thousands separators and attach a currency symbol:
const formatCurrency = (number, symbol = '$') => {
// Convert the number to a string and add thousands separators
const formattedNumber = number.toString().replace(/B(?=(d{3})+(?!d))/g, ',');
// Return the formatted number with the currency symbol
return `${symbol} ${formattedNumber}`;
};
const number = 123456.789;
console.log(formatCurrency(number)); // Output: $123,456.789
In this example, the formatCurrency
function takes a number and an optional currency symbol. The regular expression B(?=(d{3})+(?!d))
identifies positions in the string where a comma should be inserted as a thousands separator. This customizes the formatting to include commas, making the number readable and adding the specified currency symbol.
To customize decimal places, you can modify the function to precisely control the number of digits:
const formatCurrency = (number, symbol = '$', decimals = 2) => {
const fixedNumber = number.toFixed(decimals);
const formattedNumber = fixedNumber.replace(/B(?=(d{3})+(?!d))/g, ',');
return `${symbol} ${formattedNumber}`;
};
const number = 123456.789;
console.log(formatCurrency(number, '$', 2)); // Output: $123,456.79
Here, toFixed(decimals)
is used to control the number of decimal places. This ensures the formatted number has exactly the specified digits following the decimal point, enhancing the customization further.
For international currency formatting, adapt the function to handle various symbols and rules, such as for European formatting:
const formatCurrency = (number, symbol = '€', decimals = 2) => {
const fixedNumber = number.toFixed(decimals).replace('.', ',');
const formattedNumber = fixedNumber.replace(/B(?=(d{3})+(?!d))/g, '.');
return `${symbol} ${formattedNumber}`;
};
const number = 123456.789;
console.log(formatCurrency(number, '€', 2)); // Output: €123.456,79
In this example, decimal points are replaced by commas, and thousands separators are replaced by periods, following European standards. This further illustrates the flexibility of using regular expressions for custom currency formatting.
By leveraging regular expressions, you can create tailored formatting solutions that address unique and specific requirements beyond the capabilities of built-in methods. This approach ensures your application’s numeric data presentation is optimized for various contexts, enhancing both usability and clarity.
Writio: Your AI content writer for top notch articles. This article was crafted by Writio.
- ECMAScript 2021 Internationalization API Specification. Ecma International. 2021.
- MDN Web Docs. Number.prototype.toLocaleString(). Mozilla. 2023.
- MDN Web Docs. Intl.NumberFormat. Mozilla. 2023.
- Haverbeke M. Eloquent JavaScript: A Modern Introduction to Programming. 3rd ed. No Starch Press; 2018.