Understanding Function Parameters and Arguments
Function parameters are names listed in the function definition. They serve as placeholders for the values that will be passed when invoking the function. Meanwhile, arguments are the actual values supplied to the function when it’s called. Understanding the difference is essential for writing clear and effective code.
Consider a basic example:
function greet(name) {
console.log('Hello ' + name);
}
greet('Alice'); // Outputs: Hello Alice
Here, name
is a parameter, and 'Alice'
is the argument.
Default Parameters
Default parameters in JavaScript allow you to set default values for parameters. If no value or undefined
is passed for a parameter, it will take on the default value specified.
function greet(name = 'Guest') {
console.log('Hello ' + name);
}
greet(); // Outputs: Hello Guest
greet('Alice'); // Outputs: Hello Alice
This feature reduces the need for additional checks within the function.
Rest Parameters
Rest parameters allow a function to accept an indefinite number of arguments as an array. This is indicated by three dots (...
) before the parameter name.
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3)); // Outputs: 6
console.log(sum(1, 2, 3, 4, 5)); // Outputs: 15
Rest parameters provide flexibility without needing to explicitly list all the parameters.
Arguments Object
In non-arrow functions, the arguments
object is available. It’s an array-like object containing all the arguments passed to the function.
function showArguments() {
console.log(arguments);
}
showArguments(1, 'test', true); // Outputs: { '0': 1, '1': 'test', '2': true }
Using arguments
, you can access all the parameters passed without formally defining them.
Parameter Destructuring
ES6 brought parameter destructuring, allowing you to unpack values from arrays or properties from objects directly into variables.
function greet({name, age}) {
console.log(`Hello, ${name}. You are ${age} years old.`);
}
const person = {name: 'John', age: 25};
greet(person); // Outputs: Hello, John. You are 25 years old.
You can also destructure arrays:
function display([firstName, lastName]) {
console.log('Hello ' + firstName + ' ' + lastName);
}
greet(['John', 'Doe']); // Outputs: Hello John Doe
Handling parameters correctly ensures functions are both powerful and flexible. They are essential for tasks like function overloading, effective type-checking, and maintaining clean code. Using clear and understandable parameter names enhances the readability and maintainability of the code.
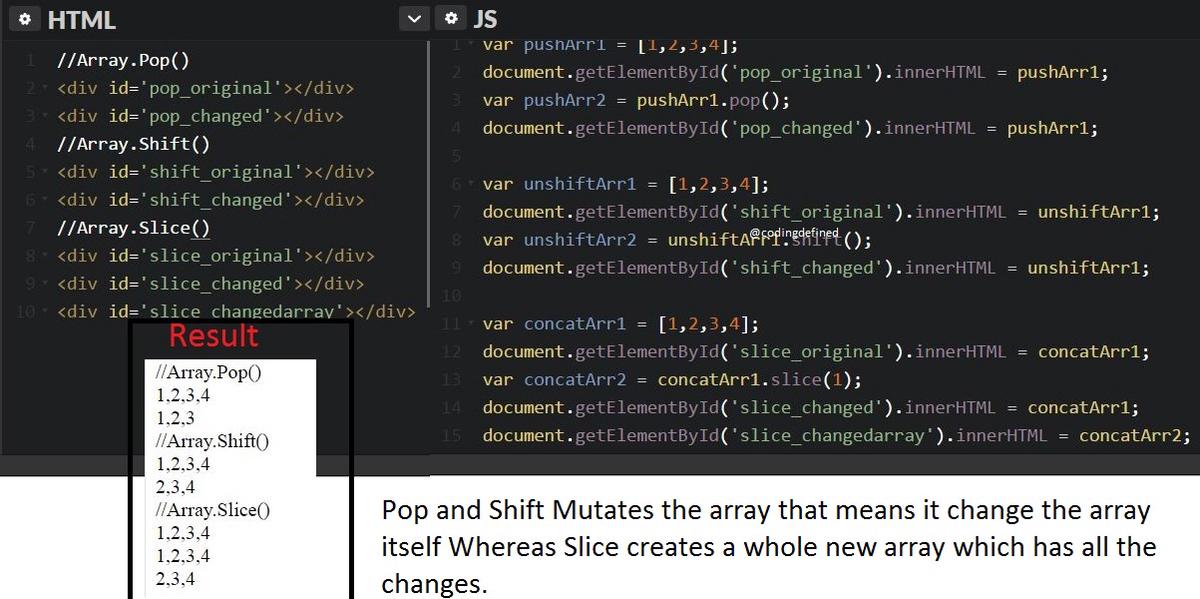
Default Parameters
In ES6, the concept of default parameters was introduced, allowing developers to set default values for function parameters. This ensures that parameters will always have a value, even if the function is invoked without arguments.
Defining default parameters is straightforward. You simply assign a default value to a parameter within the function definition. If no value or undefined
is passed to the parameter when the function is called, the default value will be used.
Consider this example:
function greet(name = 'Guest') {
console.log(`Hello, ${name}`);
}
greet(); // Outputs: Hello, Guest
greet('Alice'); // Outputs: Hello, Alice
In the greet
function, the name
parameter defaults to 'Guest'
if no argument is provided. When invoking greet()
without any arguments, it prints “Hello, Guest.” However, when an argument like 'Alice'
is supplied, it prints “Hello, Alice.”
Default parameters are particularly useful in scenarios where the function may often be called without certain arguments or where you’d like to provide a fallback value.
Imagine you’re creating a function to calculate the area of a rectangle. If not given, you want the function to assume it’s a square with equal sides. You can use default parameters to achieve this:
function calculateArea(length, width = length) {
return length * width;
}
console.log(calculateArea(5)); // Outputs: 25 (uses length for both width and length)
console.log(calculateArea(5, 3)); // Outputs: 15
Here, the width
parameter defaults to the value of length
if no second argument is provided. As a result, calling calculateArea(5)
will calculate the area of a 5×5 square.
Another practical use case for default parameters is in configuration functions where you might have a lot of optional settings. Default parameters can make configuration smoother by automatically filling in missing settings with sensible defaults.
function createUserProfile(username, role = 'Guest', isActive = true) {
return {
username,
role,
isActive
};
}
const user1 = createUserProfile('Alice');
const user2 = createUserProfile('Bob', 'Admin', false);
console.log(user1); // Outputs: { username: 'Alice', role: 'Guest', isActive: true }
console.log(user2); // Outputs: { username: 'Bob', role: 'Admin', isActive: false }
In this example, role
defaults to 'Guest'
and isActive
defaults to true
. This allows the function to be versatile and the code to remain clean without flooding it with conditional checks.
When utilizing default parameters, it’s important to note that they only apply if the argument passed is undefined
. If an argument is explicitly passed as null
or other falsy values like 0
or an empty string, the default parameter value will not take effect.
function logValue(value = 'Default Value') {
console.log(value);
}
logValue(undefined); // Outputs: Default Value
logValue(null); // Outputs: null
logValue(''); // Outputs:
logValue(0); // Outputs: 0
In the logValue
function, the default value ‘Default Value’ is applied only when the argument is undefined
. Other values, including falsy ones like null
, an empty string, or 0
, will override the default.
Rest Parameters and the Arguments Object
Rest parameters allow you to represent an indefinite number of arguments as an array. This syntax uses three dots (...
) before the parameter name and provides a flexible way to work with functions that require a variable number of arguments. They are especially useful when you don’t know how many arguments will be passed to the function or when processing any number of parameters the same way.
Consider this example:
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3)); // Outputs: 6
console.log(sum(1, 2, 3, 4, 5)); // Outputs: 15
Here, the sum
function takes any number of number arguments, sums them up, and returns the total. The rest parameter ...numbers
gathers the arguments into an array, making it simple to use array methods like reduce
.
Rest parameters can also be combined with other named parameters, but they must be the last parameter:
function combineStrings(separator, ...strings) {
return strings.join(separator);
}
console.log(combineStrings('-', 'one', 'two', 'three')); // Outputs: one-two-three
In this function, separator
is a standard parameter, and ...strings
is the rest parameter. The function joins the provided strings using the specified separator.
The arguments
object is another way to handle multiple function arguments. It is available in non-arrow functions and contains an array-like collection of all the arguments passed to the function, regardless of how the parameters are defined. The arguments
object is not as flexible and lacks many array methods directly, making it less convenient compared to rest parameters.
Here’s an example using the arguments
object:
function showArguments() {
for (let i = 0; i < arguments.length; i++) {
console.log(arguments[i]);
}
}
showArguments(1, 'test', true); // Outputs 1, 'test', true
While the arguments
object can be useful, it cannot be utilized with arrow functions and is not as clear or structured as rest parameters for modern JavaScript coding practices.
Comparison and Use Cases
Both rest parameters and the arguments
object allow functions to handle multiple inputs flexibly, but they have distinct differences and specific use cases:
- Rest Parameters are preferred in modern JavaScript because they are array instances, providing access to array methods like
map
,filter
, andreduce
. They also improve code readability and maintainability.
function add(...numbers) {
return numbers.filter(Number.isFinite).map(num => num * 2);
}
console.log(add(1, 2, 'a', 4)); // Outputs: [2, 4, 8]
- The
arguments
Object is useful for backward compatibility with older codebases or when refactoring legacy code where changing parameter definitions might be impractical.
function legacyLog() {
console.log(`Arguments length: ${arguments.length}`);
Array.prototype.forEach.call(arguments, (arg, index) => {
console.log(`Argument ${index}: ${arg}`);
});
}
legacyLog(5, 'b', {}, null); // Logs length and each argument.
When to Use Each
- Use Rest Parameters when writing or refactoring modern JavaScript, especially if you need to leverage array operations and maintain clear, concise function definitions.
- Use the
arguments
Object in cases where you deal with non-arrow functions and need access to all invoked parameters, particularly in maintaining older codebases or for some specific edge cases where direct use ofarguments
is already established.
Embracing rest parameters in new development while reserving the arguments
object for legacy support helps you strike a balance between modern coding practices and maintaining compatibility with older code. Thus, utilizing these tools appropriately can greatly enhance function flexibility and robustness in your JavaScript projects.
Destructuring Parameters
Destructuring parameters, introduced in ES6, bring convenience to JavaScript functions by allowing you to unpack values from arrays or objects directly within the function signature. This promotes cleaner, more readable code and reduces the need to manually extract properties or array elements within the function body.
In destructuring, you can specify which parts of an object or array you want to extract as parameters. This is especially useful when dealing with intricate objects or when you want to directly access deep properties without intermediate variables.
Consider an example using objects:
function printUserDetails({name, age, location}) {
console.log(`Name: ${name}, Age: ${age}, Location: ${location}`);
}
const user = {name: 'Alice', age: 30, location: 'New York'};
printUserDetails(user); // Outputs: Name: Alice, Age: 30, Location: New York
In this example, the printUserDetails
function directly extracts the name
, age
, and location
properties from the user
object. This makes the function signature more informative and avoids repetitive code that manually assigns these values within the function.
Destructuring can also be applied to arrays:
function displayCoordinates([x, y]) {
console.log(`X: ${x}, Y: ${y}`);
}
const coordinates = [10, 20];<br>displayCoordinates(coordinates); // Outputs: X: 10, Y: 20
Here, displayCoordinates
takes an array as an argument and immediately extracts its first two elements as x
and y
. This technique is clean and efficient, avoiding the need for index-based access within the function.
When destructuring, you can also provide default values to ensure that parameters are initialized correctly even if the corresponding parts of the object or array are undefined
:
function greetUser({name = 'Guest', age = 18} = {}) {
console.log(`Hello, ${name}. You are ${age} years old.`);
}
greetUser(); // Outputs: Hello, Guest. You are 18 years old.
greetUser({name: 'Bob'}); // Outputs: Hello, Bob. You are 18 years old.
In the greetUser
function, default values are provided for both name
and age
. The function itself defaults to an empty object, ensuring it won’t break if called without any arguments.
Destructuring is also valuable when working with deeply nested objects. Instead of manually extracting each nested property, you can do it all at once in the function signature:
function displayVehicleDetails({model, engine: {type, horsepower}}) {
console.log(`Model: ${model}, Engine Type: ${type}, Horsepower: ${horsepower}`);
}
const vehicle = {model: 'Tesla Model S', engine: {type: 'Electric', horsepower: 762}};
displayVehicleDetails(vehicle); // Outputs: Model: Tesla Model S, Engine Type: Electric, Horsepower: 762
In this example, the displayVehicleDetails
function directly extracts the model
of the vehicle as well as the type
and horsepower
from the nested engine
object. This approach greatly simplifies the function logic and enhances readability.
Destructuring is not just limited to parameters of the main function but can be nested within other parameter structures. Here’s an example:
function processOrder({customer: {name, contact}, items}) {
console.log(`Customer: ${name}, Contact: ${contact}, Items: ${items.length}`);
}
const order = {
customer: {name: 'Alice', contact: '555-1234'},
items: ['item1', 'item2', 'item3']
};
processOrder(order); // Outputs: Customer: Alice, Contact: 555-1234, Items: 3
In processOrder
, the function signature destructures the customer
object and items
array from the order
object, providing straightforward access to nested properties.
In summary, destructuring parameters bring clarity, reduce redundancy, and enhance the expressiveness of function definitions in JavaScript. By skillfully applying object and array destructuring within function signatures, you can write more concise, readable, and maintainable code.
Best Practices for Handling Function Parameters
One essential aspect of working with JavaScript function parameters is adhering to certain best practices to ensure your code remains clean, flexible, and reusable. Properly managing function parameters improves code readability and reduces the likelihood of errors. Here are some guidelines:
Always validate your parameters. Ensuring that your function receives the correct type and number of parameters helps prevent unexpected behavior and runtime errors. JavaScript, being dynamically typed, doesn’t enforce argument types or counts, so it’s up to the developer to ensure that functions are called with the appropriate values.
For instance, if a function expects a number, you should check the type and provide meaningful feedback if the validation fails:
function add(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error('Both parameters must be numbers');
}
return a + b;
}try {
console.log(add(2, 3)); // Outputs: 5
console.log(add(‘2’, 3)); // Throws Error
} catch (error) {
console.error(error.message);
}Use default parameters to handle undefined arguments. This can prevent errors that occur when a function receives fewer arguments than required. Default parameters ensure that every parameter has a valid value.
function greet(name = 'Guest') {
console.log(`Hello, ${name}`);
}greet(); // Outputs: Hello, Guest
greet(‘Alice’); // Outputs: Hello, AliceWhen dealing with multiple parameters, especially those that are optional, consider using an object to pass parameters. This approach improves readability by clearly specifying which values correspond to which parameters. It also allows for more flexibility in the function calls, as the order of parameters in the object does not matter.
function configureUser({ username, role = 'Guest', isActive = true }) {
console.log(`Username: ${username}, Role: ${role}, Active: ${isActive}`);
}configureUser({ username: ‘Alice’ }); // Outputs: Username: Alice, Role: Guest, Active: true
configureUser({ username: ‘Bob’, role: ‘Admin’, isActive: false }); // Outputs: Username: Bob, Role: Admin, Active: falseTo maintain clean and manageable code, especially in large projects, functions should ideally accept no more than three parameters. If more data needs to be passed, refactor the function to accept an options object. This practice keeps your function signatures clean and makes your code self-documenting.
function addToCart({ itemName, quantity = 1, price }) {
console.log(`Adding ${quantity} of ${itemName} to cart at $${price} each.`);
}addToCart({ itemName: ‘Laptop’, price: 999 }); // Outputs: Adding 1 of Laptop to cart at $999 each.
addToCart({ itemName: ‘Mouse’, quantity: 2, price: 25 }); // Outputs: Adding 2 of Mouse to cart at $25 each.Leverage destructuring for setting default values. This can make your function definitions more readable and ensure that defaults are applied consistently.
function registerUser({ username, password, email = '' }) {
if (!username || !password) {
throw new Error('Username and password are required');
}
console.log(`Registering user ${username} with email ${email}`);
}registerUser({ username: ‘Alice’, password: ‘securepass’ }); // Outputs: Registering user Alice with email
registerUser({ username: ‘Bob’, password: ‘securepass’, email: ‘bob@example.com’ }); // Outputs: Registering user Bob with email bob@example.comKeep your parameters and functions SRP (Single Responsibility Principle) compliant, meaning each function should perform a single task or responsibility. This reduces complexity and makes functions easier to test and debug.
In summary, effective parameter handling in JavaScript involves error checking, leveraging default parameters, using objects for multiple or optional parameters, and ensuring the readability and maintainability of your code. By following these best practices, you create flexible and reusable functions, leading to cleaner and more reliable code.
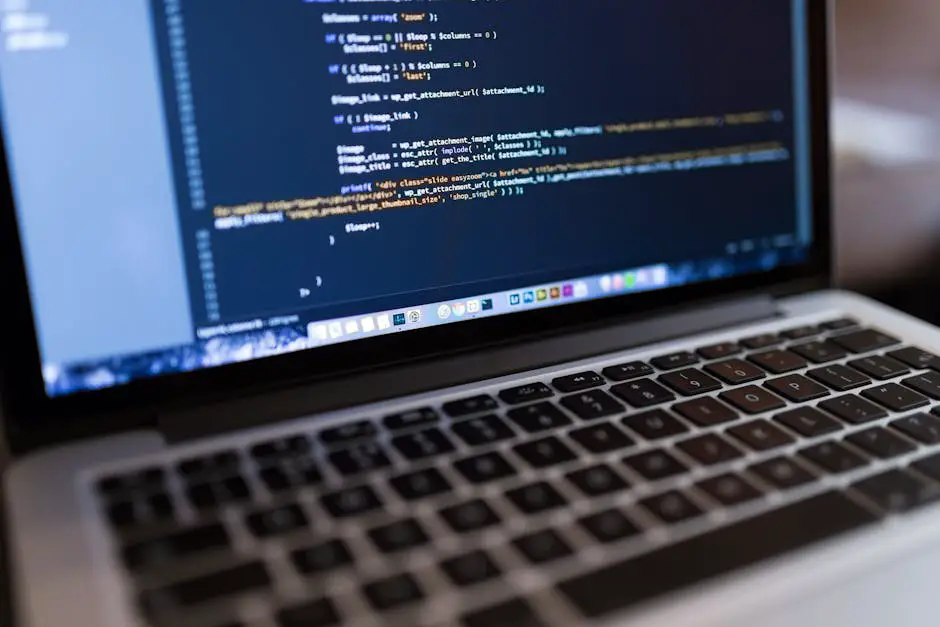
Understanding and effectively using function parameters and arguments is fundamental to writing clear and versatile JavaScript code. By mastering default parameters, rest parameters, the arguments
object, and destructuring, you can create more robust and maintainable functions.
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.