As a versatile and powerful programming language, JavaScript offers a myriad of functions and features that help create complex, interactive web applications. An important yet often overlooked part of this toolkit is indexing. Indexing in JavaScript involves accessing specific elements within data structures, such as arrays and strings, based on their position or ‘index’. Having a firm comprehension of indexing is crucial to efficiently navigate, manipulate, and extract information from these data structures. In this article, we will traverse a journey beginning from the basic understanding of JavaScript and its indexing, diving deeper into various indexing methods, exploring practical applications and examples, and finally touching upon advanced indexing techniques.
Understanding the basics of Javascript and Indexing
Understanding Basics of Javascript: A High-level, Dynamic Programming Language
Javascript is a high-level, interpreted programming language that enables interactive web pages. It’s widely utilized in web development to add dynamic behavior to static HTML pages. Javascript was first introduced in 1995 as a way to make websites more engaging and user-friendly. Today, it is an essential part of the web, supporting every modern browser.
Javascript is also considered a multi-paradigm language, which means it supports multiple programming paradigms – procedural, object-oriented, and functional programming. It is characterized by its dynamic typing, prototype-based object-orientation, and first-class functions.
Javascript Syntax and Structure
The syntax of Javascript, like any programming language, describes the rules for creating a valid program or statement. Javascript syntax is heavily influenced by C and Java languages. The code is case-sensitive and made of Unicode characters. A Javascript program is typically composed of statements, variables, operators, comments, loops, control structures, functions, and objects, among other elements.
Javascript is single-threaded, which means it processes one operation at a time in a single sequence or thread of execution. To avoid blocking (or waiting for one operation to complete before moving to the next), Javascript utilizes asynchronous programming through callbacks and promises.
Deep Dive into Indexing: From General Programming to Javascript Specifics
Indexing is a common concept in programming, related to how you access and manipulate data, especially within arrays and objects. An index refers to a specific position within an array or an object. It’s a way to reference or locate specific elements in these data structures.
Within Javascript, arrays are zero-indexed, meaning that the first element in an array is at position 0, the second is at position 1, and so forth. Thus, if you have an array of five items, the index of the last item will be 4, not 5. There are various methods to manipulate arrays in Javascript using these indices, including push
(adds to the end), pop
(removes from the end), shift
(removes from the front), and unshift
(adds to the front).
Indexing isn’t just for arrays; Javascript objects access their properties using “keys,” similar to indexes in arrays. Each key points to a property (or value) of the object.
Why Indexing Matters in Javascript
Indexing plays a vital part in Javascript, facilitating efficient manipulation of data. This includes the adding, removing, or updating of elements within an array or properties within an object. The control it offers over the data flow in your application can significantly enhance your program’s overall performance.
Mistakes made while indexing could cause errors or unpredicted behaviors. For example, references to an undefined array index would return an undefined
in Javascript, which can cause unexpected outcomes in your code.
By comprehending the intricacies of Javascript and its indexing principles, you can improve the readability of your code, its efficiency and robustness, making complex web applications easier to construct and maintain.
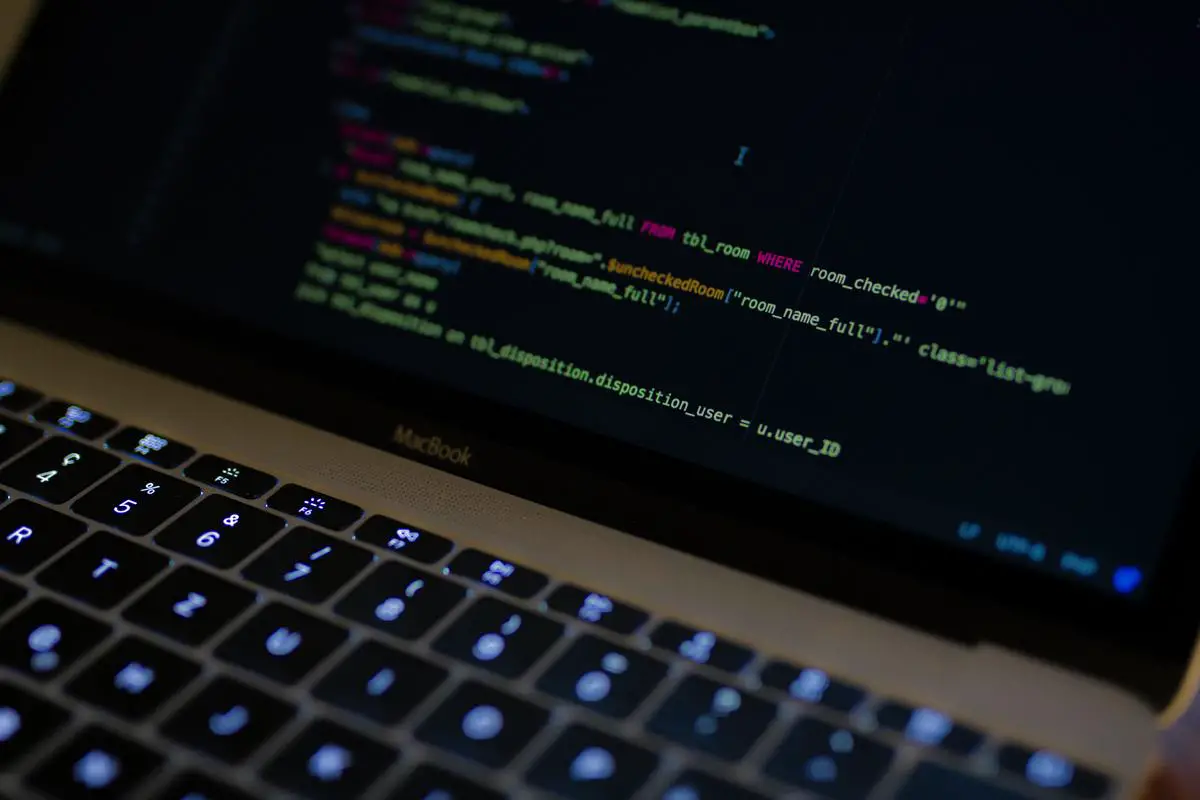
Photo by casparrubin on Unsplash
Detailed Understanding of JavaScript Indexing
Diving Deeper into JavaScript Indexing
JavaScript, a dynamic programming language, supports indexing as a method to access or modify specific elements within arrays or strings. Each position within a string or array has a corresponding index value, starting from zero. For instance, in the string “Coding,” ‘C’ has an index of 0, and ‘O’ has an index of 1.
Array Indexing in JavaScript
Arrays, ordered lists of items, allow storing multiple values in a single variable. In JavaScript, arrays utilize square brackets ([]). To access an array item, refer to an index number. Suppose we have an array named ‘technologies’ that includes ‘JavaScript’, ‘Python’, ‘Ruby’. To access ‘Python’, use technologies[1]. This method shows array indexing, with JavaScript as the 0 index and Python as index 1.
String Indexing in JavaScript
Like array, string indexes start at zero. Each character corresponds to an index position. In the string “JavaScript”, the character “J” is the 0 index and “a” is index 1.
JavaScript Indexing Properties and Methods
JavaScript provides properties and methods for efficient handling of array and string indexing. The ‘length’ property returns the number of characters in a string or array’s elements. For a string “Coding”, Coding.length would return 6.
The ‘indexOf()’ method applies to arrays and strings to return a value’s first index. For an array [‘Apple’, ‘Banana’, ‘Mango’] Mango’s index is 2 returned through ‘indexOf(Mango)’.
Handling Indexing Errors in JavaScript
What happens when you call an undefined index in JavaScript? The language returns ‘undefined’. No errors are thrown. For instance, with an example array [‘Apple’, ‘Banana’, ‘Mango’], calling array[3] returns ‘undefined’, as no element corresponds to the 3rd index.
JavaScript, however, offers methods to handle out-of-bounds indexing. The ‘splice()’ function adds or removes elements. If negative, the index starts from the array’s end. If greater than or equal to the array’s length, it starts from the array’s end.
Understanding Indexing in JavaScript
To maximize JavaScript performance, a clear grasp of efficient indexing becomes essential. When dealing with multidimensional arrays or nested objects, one must exercise caution as complex indexing can hamstring performance, especially in large datasets.
Fortunately, JavaScript provides a host of unique properties and methods designed to streamline indexing tasks, including ‘length’, ‘indexOf()’, and ‘splice()’. A strong command over such elements enables hassle-free data manipulation in arrays or strings.
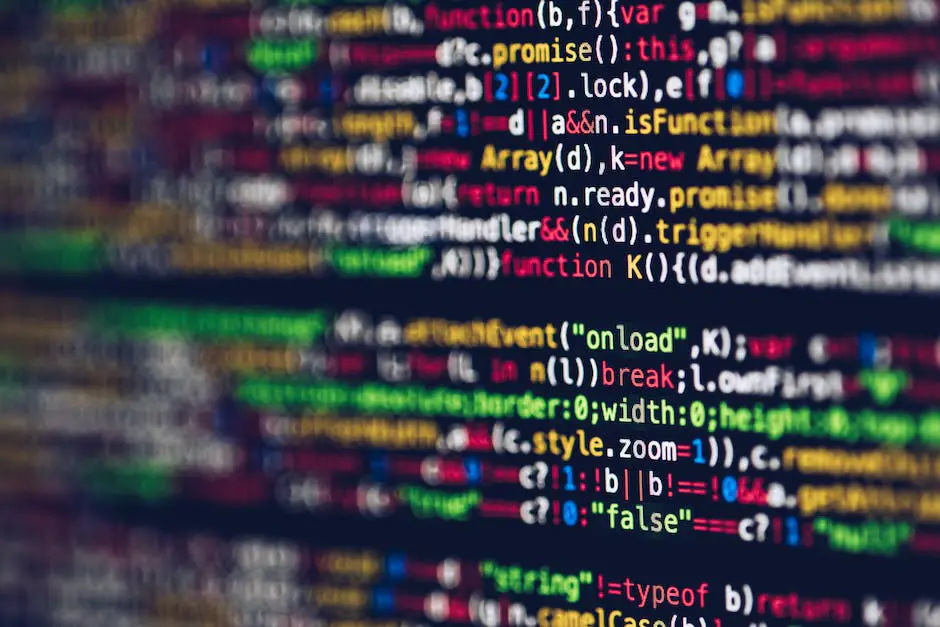
Practical Applications and Examples of JavaScript Indexing
Exploring the Importance of Indexing in JavaScript
In JavaScript, indexing is a method that allows for precise reference to an element in an array or object. Each data element is distinguished by an index, either numerical or string-based, which serves as a unique identifier and gateway to accessing and manipulating the said item. Beyond simplifying the coding process, proficient use of JavaScript indexing bolsters the speed and efficiency of the code execution.
Examples of JavaScript Indexing
Typically, you will find JavaScript indexing being used in array manipulations. For instance, suppose you have an array of elements:
<code>var fruits = ["Apple", "Banana", "Mango", "Orange", "Peach"];</code>
You can use the index to access a specific element in the array. Indexes in JavaScript start from zero, meaning the first element has an index of 0, the second an index of 1, and so forth. If you wanted to access the third element “Mango”, you would write:
<code>var thirdFruit = fruits[2];</code>
The number 2 is the index that represents the position of “Mango” in the array.
JavaScript Indexing in Iteration
JavaScript Indexing is also commonly used in iterative processes, such as loops. For example, you can use a for loop to iterate through the array and display all the fruits:
<code>for(var i = 0; i < fruits.length; i++) {<br>console.log(fruits[i]);<br>}</code>
In this snippet, i
stands for index. In each iteration, you use the index i
to access each element in the array.
Indexing issues to avoid
When working with JavaScript indexing, it’s crucial to avoid off-by-one errors, which occur when you try to access an index that is either one digit higher or one digit lower than it should be. Recall that JavaScript indexing starts at 0. Trying to access an element at an index that does not exist in the array would return undefined
.
For instance, if you try to access the sixth fruit from our fruits array:
<code>var nonExistentFruit = fruits[5];</code>
It would result in undefined
being stored in nonExistentFruit
, since our array only contains five elements, and the index starts at 0.
Diving into JavaScript: Understanding String Indexing
In the realm of JavaScript programming, the power of indexing is not confined merely to arrays. It extends to being able to access a specific character within a string. For instance:
<code>var hello = "Hello, World!";</code>
This shows that you could access a particular character just as you would fetch an element from an array:
<code>var letter = hello[4];</code>
Here, letter
represents the “o”.
Such application of JavaScript Indexing is significant when it comes to manipulating data structures and strings, making it a vital concept for practical coding.
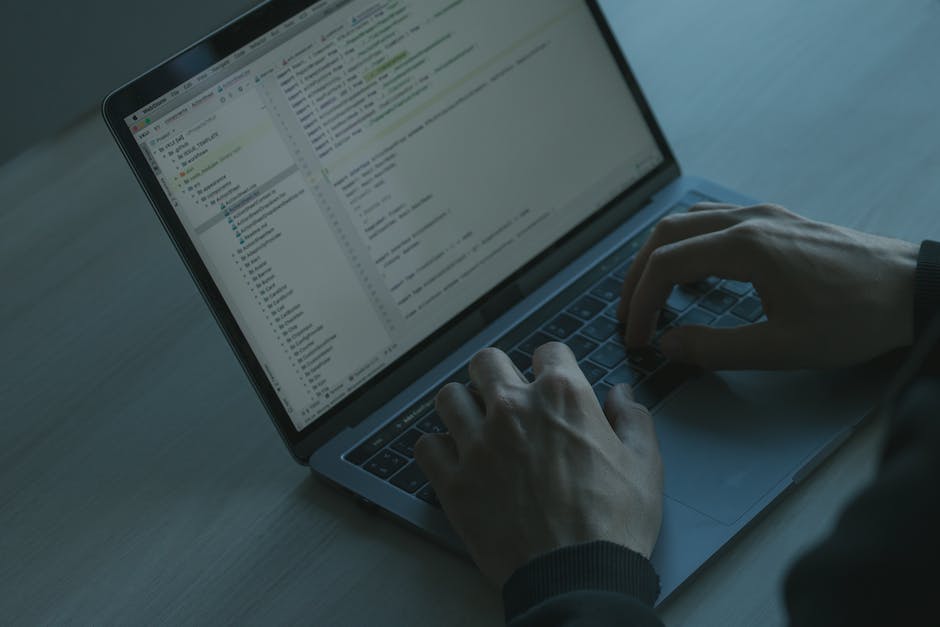
Advanced Indexing Techniques in Javascript
Elaborating on JavaScript Indexing: A Deep-Dive into Basics
Moving ahead, it is important to note that indexing in JavaScript is a fundamental technique that aids in accessing and altering array data structures. Essentially, an index in JavaScript is a numeric key allowing access to a specific value within an array. It’s more or less similar to the concept of unique identifiers. With standard JavaScript arrays commencing from zero, the index of the first element is 0, the second is at 1 and it continues in this fashion. This not only helps in efficient data retrieval but also enhances the capability to operate on selected parts of the array.
Multi-Dimensional Arrays
Moving onto more advanced concepts, multi-dimensional arrays are arrays that contain other arrays as their elements. This creation of “array of arrays” essentially formulates a grid-like or matrix structure. Think of multi-dimensional arrays as greatly beneficial when trying to represent complex structures like graphs or grids. JavaScript does not directly support multi-dimensional arrays, however, you can create them by using arrays of arrays.
For instance, to access the first element in the second array stored within a ‘master’ array, you would use syntax like masterArray[1][0].
Associative Arrays
JavaScript also introduces the concept of associative arrays. Rather than using numeric indexes to access elements, associative arrays use strings as indexes. This makes them similar to dictionaries, where specific keys are mapped to specific values.
It’s important to note that true associative arrays aren’t natively supported in JavaScript. However, JavaScript developers have adopted the use of JavaScript objects as a workaround. JavaScript objects consist of key-value pairs, where the keys act as textual indexes, enabling a similar functionality to associative arrays.
Complex Data Structures
JavaScript enables developers to build more complex data structures like stacks, queues, linked lists, trees, and graphs. These structures can offer specific benefits in particular situations. For example, stacks are used under-the-hood in JavaScript for function call stacks, while queues can be beneficial in handling asynchronous tasks.
Impacts of JavaScript Indexing on Performance
Finally, understanding and correctly using JavaScript indexing methods and data structures can greatly impact the code’s efficiency and performance. Standard array indexing is generally very fast. However, associative array-like structures with string keys instead of numeric indexes can be slightly slower due to the time required for string comparison and hashing.
Moreover, the use of complex data structures can speed up or slow down code depending on how well they’re suited to the particular task. For example, a well-implemented search algorithm on a binary tree might find data much faster than a simple linear search on an array.
Understanding and Performance of JavaScript Indexing
Understanding and using these advanced indexing techniques is a crucial skill for any JavaScript developer looking to write efficient and high-performing code.
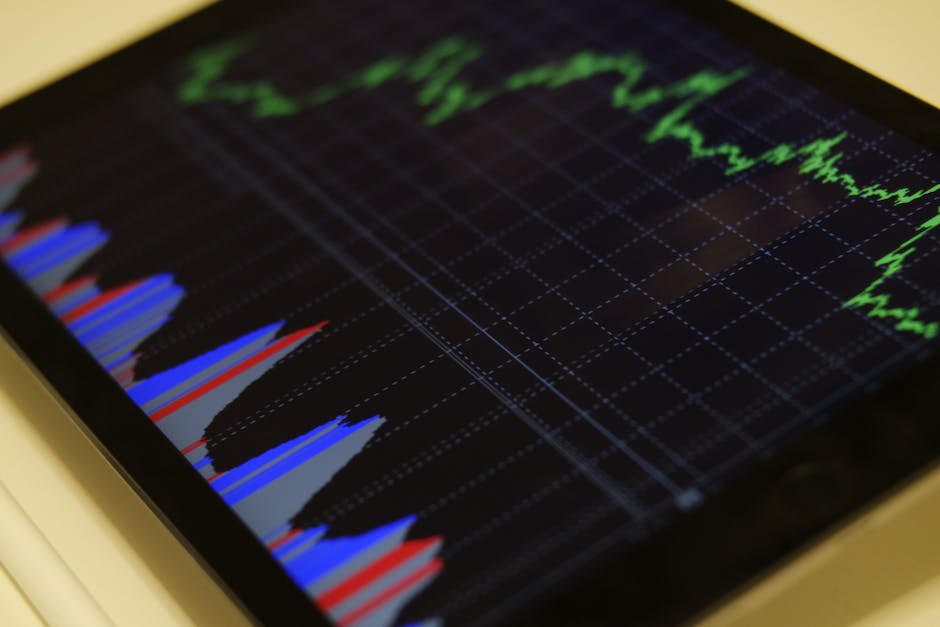
By enhancing your knowledge on JavaScript Indexing, you not only grow as a more informed, knowledgeable, and versatile JavaScript developer, but you also gain a powerful tool to solve complex programming problems with ease.
Whether it’s the basics of array or string indexing, understanding properties and methods, or utilizing advanced techniques like multidimensional arrays and associative arrays, a mastery of indexing provides a significant edge in developing efficient and optimized code. The real-world examples and potential applications of these concepts solidify them in practical use. Remember, the journey of learning and mastery in any programming concept, including JavaScript indexing, is a step-by-step process. Be patient, stay curious, and keep exploring, and the seemingly complex world of JavaScript indexing will unfold itself to you.