In the continually evolving world of programming, Java remains a consistently relevant and widely used language. The concept of Object-Oriented Programming (OOP) is crucial within Java, with understanding the function and importance of classes, objects, and methods as the primary foundational knowledge. An integral part of this proficiency is mastering the Java Collection Framework, which encompasses the add() method among the interfaces of List, Set, and Queue. This proficiency is no small task; however, through engaging with practical implementation, examples, and studying the structure of these facets, one can become adept with the add() method in diverse scenarios.
Understanding Object-Oriented Programming in Java
The Role and Importance of Object-Oriented Programming in Java
If you’re even peripherally involved in the world of programming, chances are high you’ve crossed paths with the term ‘Object-Oriented Programming’ or more simply, OOP. This invaluable approach reigns supreme across diverse programming landscapes, most notably in the Java realm. So, what’s all the buzz about? Let’s delve deeper.
Object-Oriented Programming, OOP in short, is a programming paradigm centered around the concept of ‘objects’. These objects are instances of classes, formed by data fields and procedures together called methods. What makes OOP stand out is that it models programming structures much aligned with real-world entities, each object being a unique entity with specific attributes and behavior.
Three critical elements rally under the OOP banner: encapsulation, inheritance, and polymorphism. Encapsulation safeguards data from unintended access and manipulation, allowing data and associated methods to operate as an independent ‘object’. Inheritance permits new classes to inherit properties of existing classes, ushering in code reusability and efficiency. Polymorphism facilitates the same function to handle different data types and objects, thus improving code simplicity and robustness.
Now, the million-dollar question arises – why is OOP crucial for Java? To put it simply, Java and OOP share more than a casual relationship. Agreed, Java incorporates other paradigms, too, but OOP is part and parcel of Java’s genetic makeup. Here’s why.
Firstly, by focusing on objects instead of procedures, OOP facilitates the creation of more manageable and readable codes. It propels a modular approach, enabling programmers to work on individual objects separately, rendering Java development more effective and bug-resistant.
Another prime reason lies in the way OOP anchors Java’s fundamental code reusability. Class inheritance empowers Java developers to recycle existing classes and codes, resulting in quicker, efficient, and less prone-to error-programming.
Lastly, consider the benefits of encapsulation and polymorphism in Java. Encapsulation ensures tighter control over class elements, letting programmers construct adequately protected classes, and thus robust software. Polymorphism, meanwhile, maximizes code flexibility and interface versatility.
In conclusion, the synergy of Java and OOP turns out to be more than just a marriage of convenience. Object-Oriented Programming equips Java with the muscle to tackle complex projects, simplify coding, and optimize software development effectiveness. Understanding and mastering OOP, therefore, key essentials to unlocking the power of Java in all its glory. So, in this complex and shifting terrain of technology, embracing OOP in Java becomes not merely an option, but a competitive necessity.
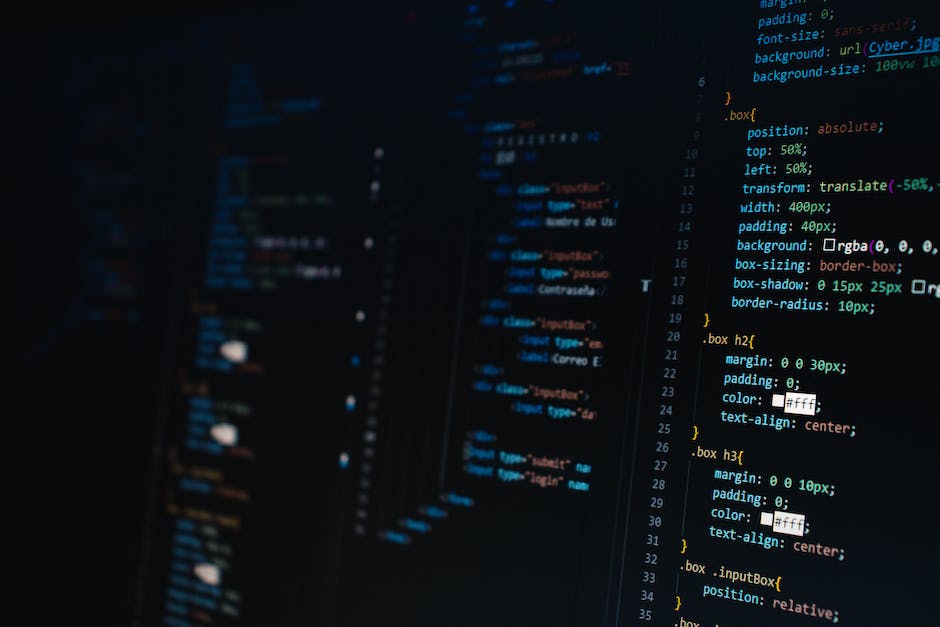
Exploration of Java Collection Framework
The indispensable Java Collection Framework: How the add() method comes into play
The Java Collection Framework (JCF) is another fundamental aspect of Java programming that builds upon the fundamental Object-Oriented Programming concepts. The JCF provides an architectural structure that offers a standardized system to manipulate data groups. This framework is servers as an ensemble of classes and interfaces that are implemented with OOP principles, serving as performance-enhancing tools for more efficient Java development.
Comprehending the structure and the purpose of the JCF is essential in achieving smooth, streamlined Java programming. It’s built like a hierarchy, beginning with the Collection interface at the top, which is then further branched out into Set, List, and Queue interfaces. The Map interface, another important aspect, isn’t a true ‘child’ of the Collection interface but is undeniably an integral part of the framework. With its unique ability to map unique keys to specific values, the Map interface serves as a valuable component of the JCF.
Now, what about the add() method?
The add() method is a built-in function from the Collection interface that performs the shared operation of adding elements to collections. The versatility of the add() method can be seen in its straightforward usage across the different types of collections, such as List, Set, and Queue, which simplifies data manipulation for the developer.
This is how it applies to a Set: the Set interface is designed to hold unique elements, and the Set relies on the add() method to add these distinct items. This means that when you try to use the add() method to insert a duplicate item into a Set, the method simply refuses to comply, thus upholding the Set’s uniqueness principle.
On the other hand, in a List, where element order and duplicates are allowed, using the add() method enables not just the addition of elements, but also allows for insertion at specific indexes.
Lastly, in a Queue, the add() method inserts elements at the tail of the Queue, assisting in maintaining the first-in, first-out (FIFO) rule of the Queue.
In summary, the JCF built on OOP concepts, coupled with the handy add() method, makes complex data handling a smooth sailing task. They serve as an exemplar of how technology can solve problems and automate tasks, making them indispensable tools in the Java developer’s arsenal in this ever-evolving technological space.
As technology continues to advance and innovate, the Java Collection Framework and the add() method stand as robust and reliable tools for Java developers. Mastering their usage is crucial for effective and efficient Java development, providing a streamlined approach to tackle the challenges presented by our ever-growing digital world.
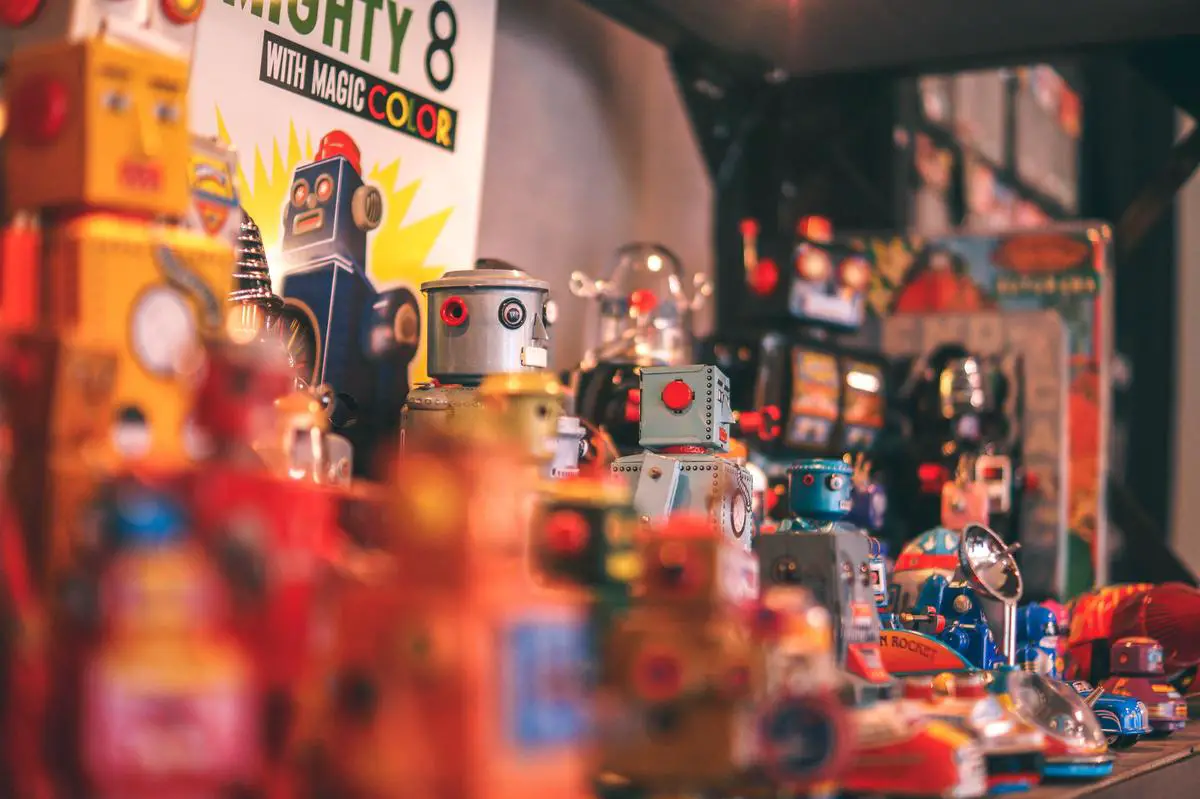
Implementation and Examples of the add() Method
Now, let’s delve a bit deeper into how the add()
method is implemented in Java. It’s a commonly used method in Java Collections Framework (JCF), and its primary function is to append elements to a collection. However, it’s essential to know that the behavior of this method varies depending on the type of collection being used.
Interaction with a Set in Java, for example, is unique. Since Set, as a rule, doesn’t allow duplicates, triggering the add()
method would yield two possible outcomes. If the Set doesn’t already contain the specified element, the add()
method would integrate that element successfully and return true. However, if the Set already comprises the element, the method would simply discard the operation and return false. In coding terms, this process would look something like this:
Set<String> techSet = new HashSet<>();
techSet.add("AI"); // Returns true
techSet.add("AI"); // Returns false
In the case of a List interface, the add()
method works differently. It not only allows duplicates but also provides the flexibility to place them at specific indices. When the add()
method is utilized without specifying an index, it automatically appends the element to the list’s end.
Conversely, when an index is stated, the method inserts the new element at that designated position shifting subsequent elements, if any, one place to the right. The method then returns a void since this operation manifests no ambiguity. The code illustration below demonstrates these functionalities:
List<String> techList = new ArrayList<>();
techList.add("AI"); // Adds "AI" at the end of the list
techList.add(0, 'AR'); // Inserts "AR" at index 0
Usage of the add()
method in Queue relies on its inherent first-in, first-out (FIFO) property. The add()
function here would append the new element at the queue’s tail and then return true. If the requested operation can’t be accomplished due to capacity restrictions, it throws an IllegalStateException. For instance:
Queue<String> techQueue = new LinkedList<>();
techQueue.add("AI"); // Adds "AI" at the tail of the queue
Efficient use of the add()
method, however, warrants a cautionary understanding of its pitfalls. In the case of a fixed-size List, attempts to add an element beyond the entity’s capacity, even at a specified index, would throw an UnsupportedOperationException. Therefore, proficiency in handling these exceptional cases is crucial when deploying the add()
method in real-world applications.
Drawing a conclusion from this, the add()
method indeed embodies a functional utility in manipulating collections in Java. Mastering its use while acknowledging its limitations is key to gaining a tactical edge in Java programming. Whether your collection is a Set, a List, or a Queue, the add()
method stands as an invaluable tool in your arsenal, offering tailored solutions for different data management requirements.
Finally, like many nuances and electives in Java and Object-oriented programming, the exercise of the add()
method in your projects will depend upon your requirement. Although seemingly straightforward, remember that every method, including add()
, is a step towards a more refined project in this continually evolving technological grandeur.
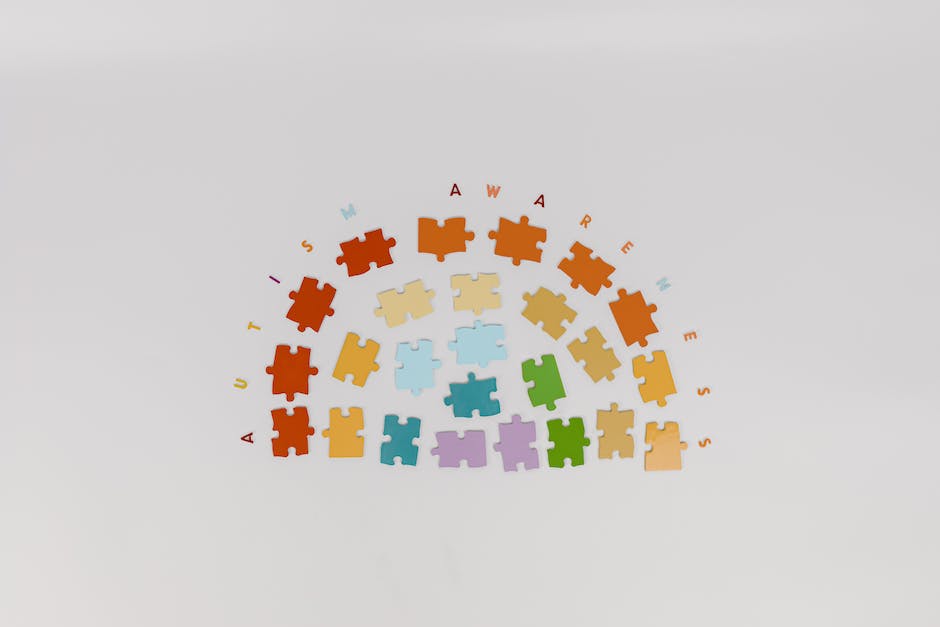
Mastering the add() method’s use in the Java Collection Framework might initially seem like a daunting task. However, with a clear understanding of Object-Oriented Programming and the function of classes, objects, and methods, any proficient Java programmer can harness its full potential. The value of comprehending and being able to utilize the Set add() method in Java cannot be overstated. As with any programming concept, continued practice, exploration, and application in various contexts will reinforce this tool’s functionality and versatility—enabling you to be an accomplished Java developer who adeptly navigates the Object-Oriented Programming world.
Mastering Java: How to Add Method