Welcome to the comprehensive discussion on the Java Util Package. It’s a salient feature of the Java classes that serves an integral role in any coding project. This package is a priceless treasure trove for any Java developer, as it simplifies numerous programming tasks and allows developers to focus on the logic and design of their code. Whether you’re a budding programmer or an experienced developer seeking to refine your understanding, the following exploration promises to be a voyage of insight into the mechanics and practical application of this crucial Java component. Be prepared to delve into the components of Java Util Package, its utilization in real-world scenarios, a wild ride through its advanced features, and a conclusion with useful tips and practices. This journey promises not only to improve your practical coding skills but also to expand your understanding of how versatile coding tools like the Java Util Package can elevate your coding efficiency to a whole new level.
Visual Studio Code for Java: Boost Your Coding Efficiency
Input Keyboard in Java: A Comprehensive Guide
Understanding the Java Util Package
The realm of programming is ever-evolving, ever-expanding, and at its core, nestled in its intricate web are various languages and tools. Among these, the Java programming language stands prominent and in many ways, unparalleled. But what makes Java truly efficient and incredibly powerful is its extensive collection of pre-packaged libraries, the notable one being the Java util package.
Adept enthusiasts might already be familiar with the Java util package or java.util but for the uninitiated, it’s like a massive toolbox providing an arsenal of tools, often simplifying complex coding scenarios. Primarily, java.util provides classes and interfaces to handle a range of functionalities related to system design and data manipulation. Imagine a scenario where you are creating a game and you need a way to interact with the time and date. Or perhaps you’re working on a task manager application and need a structure to hold and organize “to-do” items. This is where Java util package steps in with classes like Calendar, Date, Timezone, and ArrayList among others.
Diving deeper, the java.util package can be primarily divided into several sections. The Collection Framework consists of an array of classes, like ArrayList and LinkedList, built to store, retrieve, manipulate, and communicate aggregate data. Easy storage and speedy access to data makes these particular utility classes worth exploring.
Then there are classes more oriented towards events and event handling, such as Timer and TimerTask, which are quintessential for scheduling tasks to run at specific times. In fact, TimerTasks can be used to run a specific thread at regular intervals, a useful tool for tasks such as updating a time display.
Additional classes in the java.util package are excellent for date manipulation, such as the Calendar and Date classes. These classes simplify working with dates and times, which are naturally complex due to time zones, daylight saving time transitions, and more.
Random class serves a real purpose in a wide spectrum of computer applications, from gaming to statistical sampling to cryptography. It streams pseudorandom numbers, which look random but are generated deterministically from a “seed” value.
There are also “wrapper classes” like Integer or Boolean in the java.util package. These are particularly interesting because they “wrap” the primitive data types into an object of that class.
In essence, the Java util package is a treasure trove that caters to an extensive variety of programming scenarios. It’s truly the heart of Java – meeting challenges, enhancing efficiency, and elevating programming capabilities on about any Java project you’re building. It doesn’t matter if you’re fine-tuning a complex company-wide software or just building a simple game, mastering the java.util package can really fuel your Java programming skills.
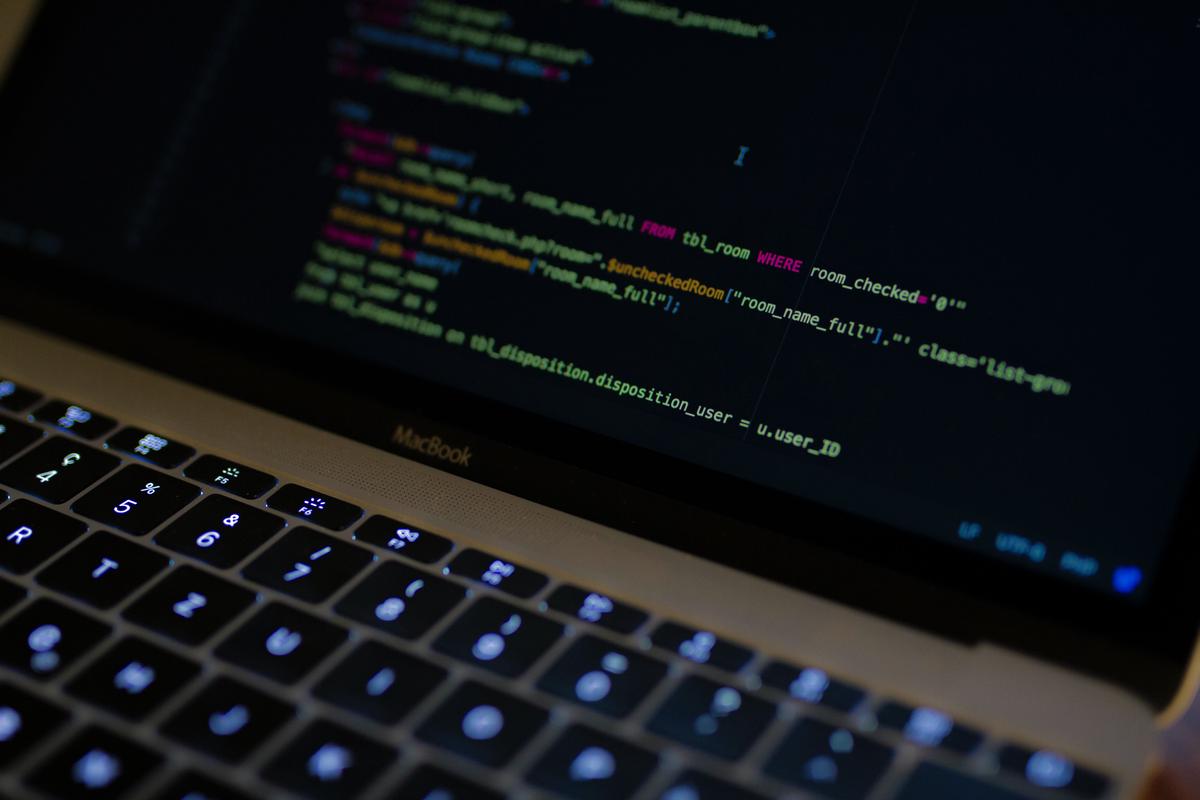
Photo by casparrubin on Unsplash
Components of Java Util Package
The Java util package is incredibly versatile and is a necessity for programmers who wish to improve their Java coding capabilities. An advanced understanding of it is paramount for achieving efficiency and productivity, two things any tech-savvy individual values. Let’s delve into other vital elements contained within the java.util package, which were not covered earlier.
The package is home to various legacy classes such as Dictionary, Hashtable, Stack, and Properties. Although the Collections Framework – with classes like ArrayList, and LinkedList – has mostly superseded these, their understanding is essential for compatibility with older codes. Hashtable, for instance, can store key-value pairs in a map. Stack, is an extended vector class following the ‘Last-In-First-Out’ principle. Properties class can maintain lists of values where the key is a string, and the associated value is also a string, often utilized in ‘key=value’ format.
Let’s now explore the functions related to arrays. We have Arrays class, which provides methods to manipulate arrays, be it sorting, searching, or comparing. The Comparator interface, on the other hand, empowers developers to order objects in a particular sequence – increasing or decreasing, or any order as per the application’s need.
The java.util package also caters automating time-linked operations. The Timer and TimerTask classes, while briefly touched on before, play a pivotal role in scheduling tasks. A Timer object holds instances of TimerTask to be executed after a specified time interval. Programmers could make a PC remind them of a meeting at a set time, for instance – the possibilities are endless.
There is also the java.util.logging package – it’s a logging framework by Java itself, allowing coders to log messages for diagnostic purposes. It can record exceptions, errors, debug information, and also other application events.
Last but not least, the Random and Math classes provide additional functionalities for mathematical and statistical operations. They cater to generating random numbers, trigonometric functions, logarithmic calculations, rounding off numbers, etc.
The java.util package is an expansive toolbox every Java programmer must be well-versed with. From handling collections of data, manipulating dates, automating tasks, to performing complex mathematical operations and logging – there’s much to explore and harness for streamlined, efficient coding. It isn’t merely a set of random utilities, but a structured assortment of classes and interfaces that has become a backbone for Java programmers across the globe. Mastering it would undoubtedly translate into a professional proficiency inevitable for contemporary software programming and development.
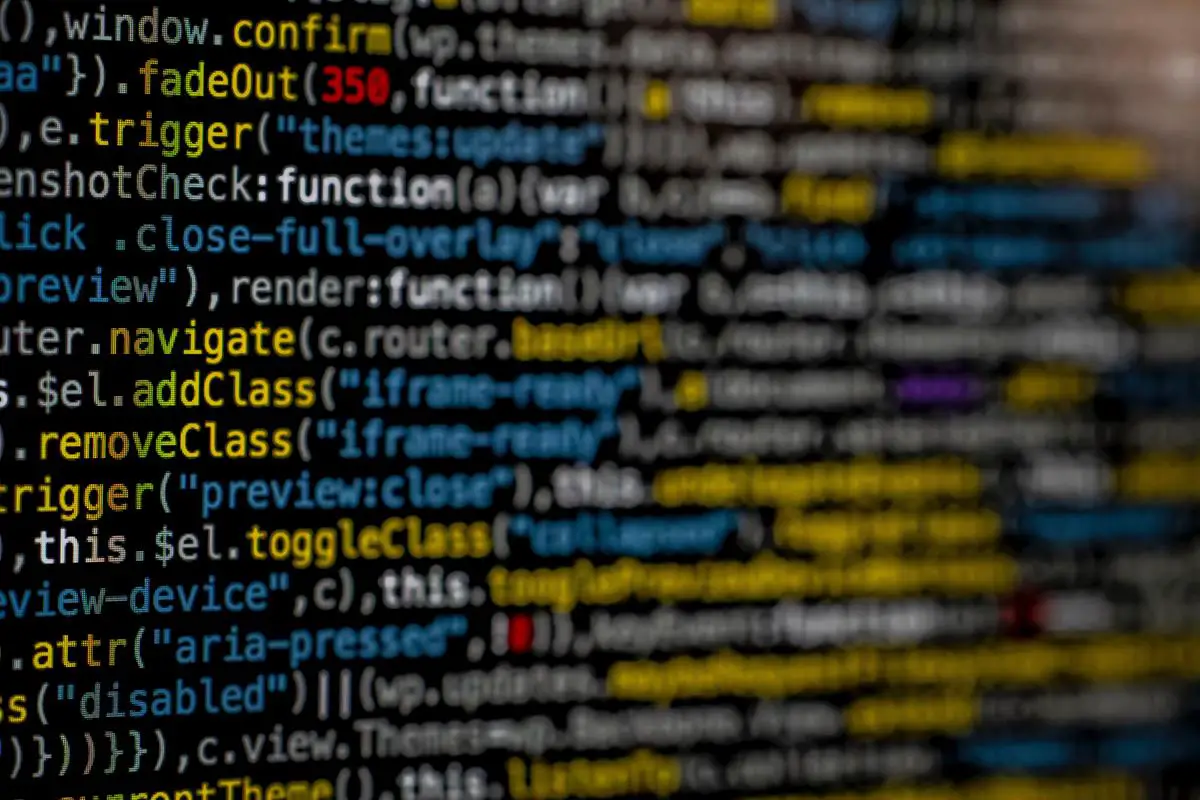
Photo by markusspiske on Unsplash
Utilizing Java Util in Real-life Scenarios
Implementing Java Util in Coding Scenarios: Methods Unboxed
Digging deep into the Java util package reveals an expansive toolkit ready to streamline your coding process. These tools aren’t just for show, as you’ll soon discover. They’ve got serious muscle that can supercharge your Java programming skills when applied practically.
Let’s dissect further.
Harnessing Power of Legacy Classes
These underrated classes from the olden days – Dictionary, Hashtable, Stack, and Properties – bring some serious heat to the table. Take Hashtable and Stack; they’re perfect for data manipulation scenarios, whether you need to structure data using key-value mapping or leverage lifo (last-in, first-out) principle in a unique way.
Properties class? That’s your savior when it comes to setting up configuration files. Swapping configurations, managing system properties or even bundling language-specific resources? There’s a class for that. So before overlooking these vintage gems, remember – they were built to deliver.
Arrays and Comparator: Speeding Up Operations
The Arrays class, in particular, proves itself a terrific tool when working with any array-based data. The secret lies in its methods that make it a breeze to format, sort, or search arrays. Want to know if two arrays are the same? There’s a method for you: Arrays.equals().
Comparing arrays lexicographically, copying arrays, filling array elements, transforming the entire list into a string – the list of methods is dynamic in the Arrays class. Conversely, the Comparator interface is an ally when creating custom ordering of objects, taking your coding efficiency to another level.
Balancing Utility with Timer and TimerTask
Should you find your coding scenarios need precise timing or performing a task at a regular interval – no problem! Use the Timer class to schedule tasks for one-time execution, or repeated execution at a fixed interval. You can easily designate tasks as threads, using TimerTask for multithreading your codes. Management of code timing has never been so efficient.
Making Use of Logging
Ever find yourself deep into a debugging session and yearning for a comprehensive log file? Java.util’s logging package is a game changer. Logging messages improve traceability and error detection. It allows for detailed tracking of the system’s operation, making it easier for the developer to maintain the system and diagnose issues before they grow from molehills into mountains.
Maximizing Mathematical and Statistical Operations
Java.util package also brings statistical and mathematical operations to the forefront. Harness the power of Random and Math classes to generate random numbers or execute advanced mathematical operations. Need to calculate the max of two numbers, absolute value or a trigonometric sine or a logarithm? The Math class gives you these functions.
Unleashing the power of the Java.util package is a smart move in your coding toolbox. It lets you automate complex processes, master your coding environment and create a skeleton for some genuinely engaging programming scenarios. So dive headfirst into Java.util. With this package, the fun has only just begun.
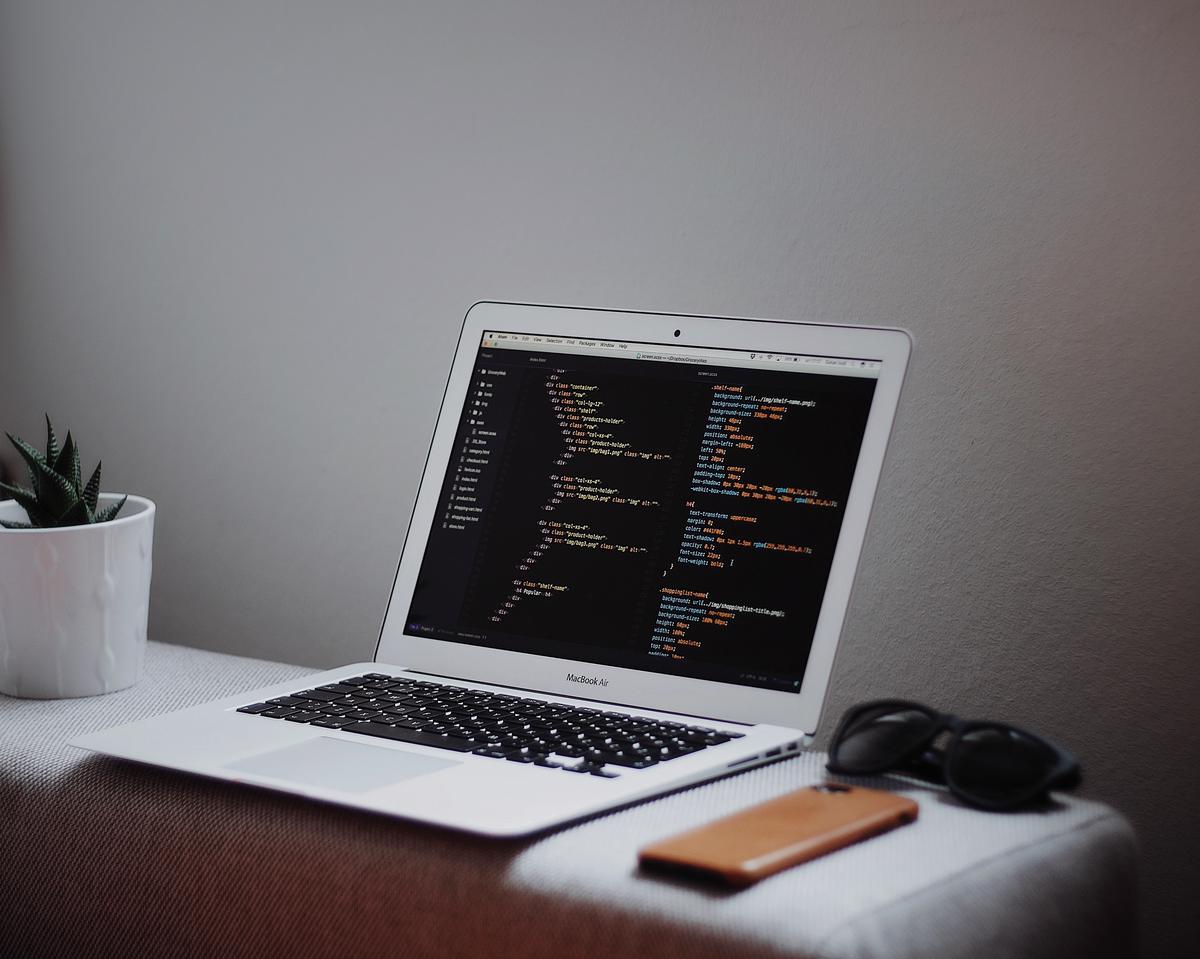
Photo by goran_ivos on Unsplash
Exploring Advanced Features of Java Util
Starting from the basics, the additional advanced features of the Java util package offer an extensive degree of functionality that allows for efficient and optimized coding experience. Building on the already established functionalities explored so far, we will delve into more specific and sophisticated features that the java.util package boasts of, which can help developers work more smartly and efficiently.
Delving into the more advanced aspects, we should first touch on the java.util.concurrent
package that holds various utilities aiding in writing reliable and scalable concurrent code. With classes like ConcurrentHashMap
, CountDownLatch
, Semaphore
, and CyclicBarrier
, concurrent processing and thread safe collections become a breeze, enhancing multi-threading capabilities.
Next, we shift our focus to the java.util.function
package. This offers functional interfaces like Consumer
, Function
, Predicate
and Supplier
which serve as a cornerstone for lambda expressions and method references ushering in enormous flexibility and reducing boilerplate code.
Moving beyond arrays to tackle more complex data, we find the java.util.stream
package. Introduced in Java 8, it provides a powerful abstraction for working with sequences of data in a declarative way. Stream API allows huge volumes of data to be processed efficiently and in parallel, opening doors to performant data analytics solutions.
Understanding the importance of internationalization, we find various classes in the java.util
package like Locale
, Currency
and ResourceBundle
which aid in writing applications keeping the global audience in mind. With features to manipulate calendars and time zones, format dates and currency for different locales, it eliminates manual handling of these complexities.
Lastly, the java.util.zip
package provides classes for reading and writing the standard ZIP and GZIP file formats, thus offering a lower-level access to data with enhanced compression capabilities. This helps in reducing the storage cost and transmission time of large chunks of data.
In conclusion, the java.util package is not just a collection of utility classes and interfaces, it is an ever-evolving toolkit providing vast and essential utilities that support everyday programming tasks. Understanding and unlocking its potential can add strength to a developer’s coding prowess, paving the way for streamlined processes and more effective systems. Whether one is developing a simple application or working on complex distributed systems, the Java util package, with its advanced features, proves itself as an indispensable tool in a developer’s kit.
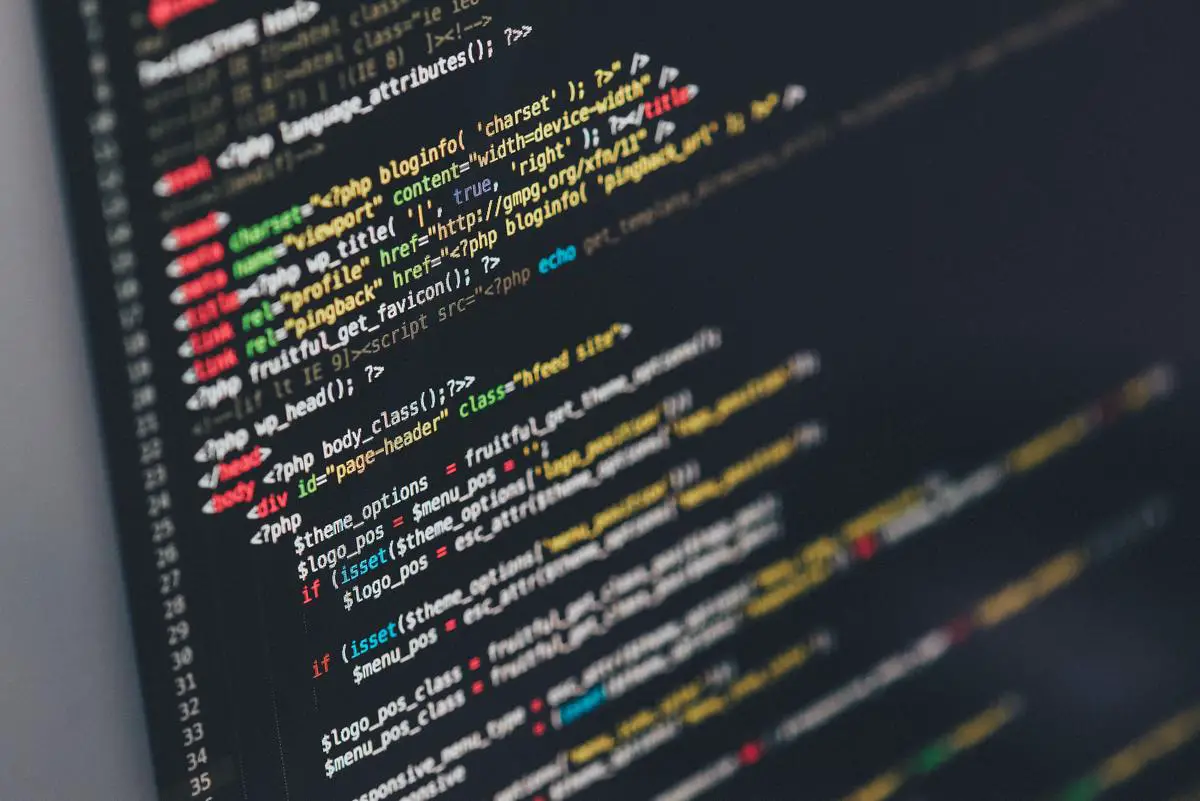
Photo by ilyapavlov on Unsplash
Tips and Best Practices for Using Java Util
Now that we’ve covered the many facets of the Java util package, let’s delve into some key tips and best practices for using this versatile tool. Remember, the path to Java mastery lies in understanding its intrinsic concepts and nomenclature, and the java.util package is an integral part of that journey.
- Embrace object-oriented programming: As you work with internal classes like Dictionary, Hashtable, and others, remember these are objects. Utilize encapsulation, abstraction, inheritance, and polymorphism to maximize their utility.
- Master the art of sorting and searching: The Arrays class and the Comparator interface are your ace tools for managing arrays. Practice creating custom object ordering using Comparator, and embrace the use of Arrays class methods.
- Use Timer and TimerTask for efficient time management: Java’s Timer and TimerTask provide superior task scheduling with utmost precision. They are particularly crucial in multi-threading environments.
- Log wisely: The java.util.logging package offers a comprehensive logging system for tracking activities and troubleshooting errors. Customize your logging practices per your needs – a well-maintained log can be the lifesaver when crunching fine-grained bugs.
- Utilize Random and Math classes: For mathematical and statistical operations, always leverage the Random and Math classes. They are precise, efficient, and multi-functional.
- Leverage concurrency utilities: Java.util.concurrent package offers high-level utilities that promote multi-threading, enhancing your programs’ performance, and strengthens the aspect of parallel processing.
- Implement functional interfaces: Expedite your code by utilizing lambda expressions and method references provided by java.util.function. This feature significantly aids in creating flexible and efficient chain-like code structures.
- Exploit the capabilities of Streams: Processing sequential and parallel data execution becomes a cinch with java.util.stream. It ensures optimal CPU utilization and maintains code readability.
- Think global, code local: Always consider internationalization in your projects. Use java.util’s locale handling, time zones, and currency classes to create more versatile and globally aware applications.
- Manage compressed data: The java.util.zip provides utilities to efficiently work with ZIP and GZIP file formats, which is indispensable in this data-conscious era.
Comprehending and employing these key insights will help you proficiently maneuver the Java util package, facilitating coded solutions that are streamlined, readable, and performance-intensive. The efficient use of this package corners the upkeep of automation, thus nurturing your potential rise as a pro Java developer on the relentless tech forefront. All set, Java geeks! Happy coding!
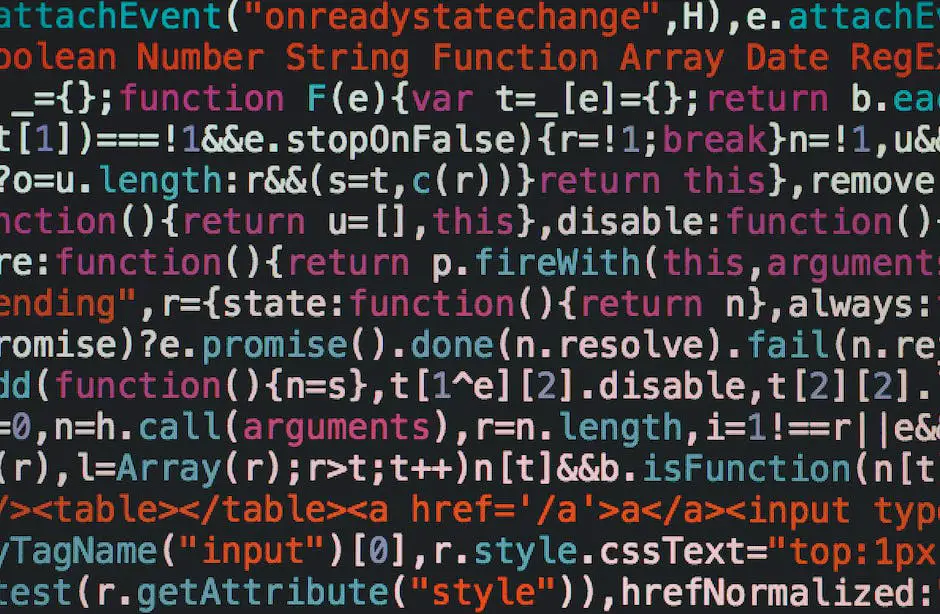
The profundity and breadth of the Java Util Package cannot be overstressed. As its components interlock seamlessly, like gears in a sophisticated clockwork mechanism, efficient solutions are crafted in the world of programming. Programming doesn’t just about using tools to solve problems; it’s about using them right and mastering them. We hope this foray into the Java Util Package has solidified your understanding of its various components and offered you workable, practical ways to use them in your projects. More than that, it’s our aspiration that the in-depth discussion on the advanced features has opened your eyes to the true potential of Java programming. We leave you with these useful guidelines and practices as you venture forth to apply this newfound knowledge and make your code a testament to the elegance of Java’s problem-solving capabilities.