Java is a versatile and widely used programming language, which often requires developers to compare integer values for different applications. Whether you’re an experienced Java developer or just getting started with the language, it’s important to understand the intricacies of integer comparison. Let’s dive deeper into the world of comparing integers in Java and explore best practices, common mistakes to avoid, and advanced techniques.
I. Introduction
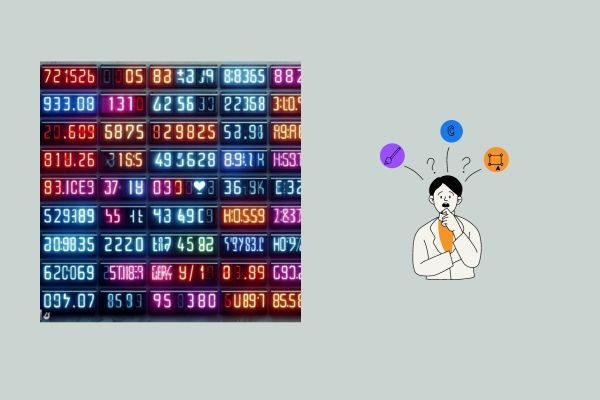
A. Brief Overview of Java Programming Language
Java is known for its platform independence and object-oriented approach. It provides a robust environment for developing diverse applications. Understanding how to compare integer values is fundamental for effective Java programming.
B. Importance of Comparing Integer Values in Java
Integer comparison is a common function in programming, and in Java, it is essential for decision-making, sorting, and various algorithm implementations. Mastering the art of comparing integers ensures efficient and error-free code.
II. Basic Comparison Operators
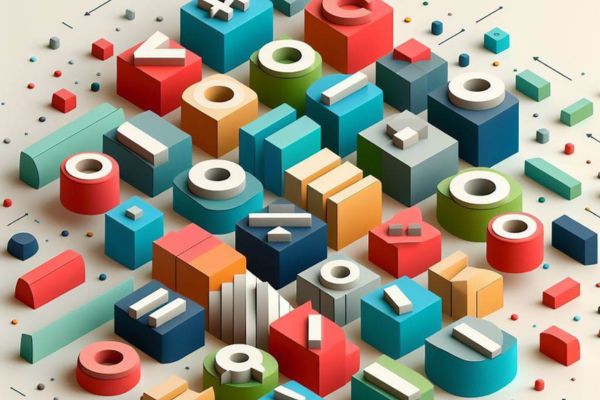
A. Explanation of Equality (==) Operator
In Java, the equality (==) operator checks if two integer values are identical. It’s a straightforward way to compare integers for equality.
int a = 5;
int b = 5;
boolean areEqual = (a == b); // true
B. Overview of the Inequality (!=) Operator
The inequality (!=) operator, on the other hand, evaluates to true if the integers being compared are not equal. It’s the negation of the equality operator.
int x = 10;
int y = 20;
boolean notEqual = (x != y); // true
C. Demonstrating the Less Than (<) and Greater Than (>) Operators
For numerical comparisons, the less than (<) and greater than (>) operators come into play. They help establish the relative order of integer values.
int num1 = 15;
int num2 = 10;
boolean isGreaterThan = (num1 > num2); // true
III. Object-Oriented Comparison
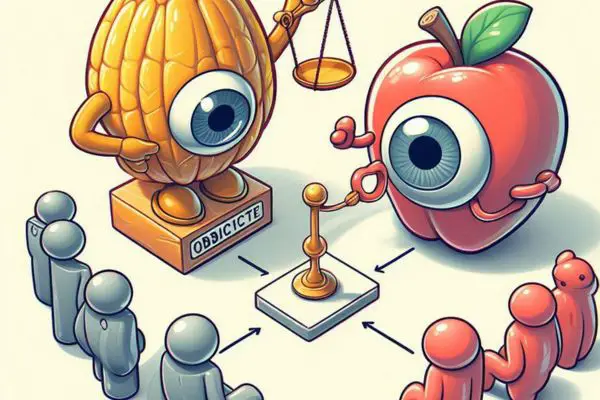
A. Introduction to Wrapper Classes
In Java, integers are primitive data types, but they can also be represented as objects using wrapper classes like Integer. Understanding wrapper classes is crucial for object-oriented comparisons.
Integer intObj1 = new Integer(5);
Integer intObj2 = new Integer(5);
boolean objectEquality = intObj1.equals(intObj2); // true
B. Using compareTo() Method for Integer Comparison
The compareTo() method, available in wrapper classes, facilitates object-based comparison. It returns a negative, zero, or positive integer depending on whether the current object is less than, equal to, or greater than the specified object.
Integer numA = 15;
Integer numB = 10;
int result = numA.compareTo(numB); // result is positive
C. Exploring equals() Method for Object-Based Comparison
The equals() method, inherited from the Object class, can be overridden to provide a custom implementation for comparing integer objects. This method is particularly useful when dealing with objects rather than primitive data types.
class CustomInteger {
private int value;
@Override
public boolean equals(Object obj) {
// Custom implementation for object comparison
}
}
IV. Best Practices for Integer Comparison
A. Dealing with Null Values
When comparing integers that might be null, it’s essential to handle null values gracefully to prevent NullPointerExceptions.
Integer nullableInt1 = null;
Integer nullableInt2 = 10;
boolean nullComparison = (nullableInt1 != null && nullableInt1.equals(nullableInt2)); // false
B. Handling Edge Cases and Exceptions
Consideration of edge cases, such as Integer.MIN_VALUE and Integer.MAX_VALUE, is crucial to avoid unexpected behavior during comparisons.
int minValue = Integer.MIN_VALUE;
int maxValue = Integer.MAX_VALUE;
boolean edgeCaseComparison = (minValue < maxValue); // true
C. Considering Performance Implications
Choosing the right approach to integer comparison can impact performance. Consider the context and choose the most efficient method for your specific use case.
// Consider the performance of equality check versus compareTo() in large datasets
V. Common Mistakes to Avoid
A. Pitfalls with Floating-Point Comparison
Avoid using floating-point numbers for exact equality checks due to precision issues. Stick to integer values for accurate comparisons.
double floatingPointNum = 1.1;
boolean floatingPointComparison = (floatingPointNum == 1.1); // may not always be true
B. Importance of Parentheses in Complex Comparisons
When dealing with complex expressions involving multiple operators, always use parentheses to ensure the intended order of evaluation.
int p = 5, q = 10, r = 15;
boolean complexComparison = ((p < q) && (q < r)); // true
C. Understanding the Pitfalls of Using == for Object Comparison
Using the == operator for object comparison can lead to unexpected results. Object comparison should be done using the equals() method.
Integer objInt1 = new Integer(5);
Integer objInt2 = new Integer(5);
boolean objectComparison = (objInt1 == objInt2); // false
VI. Integer Comparison in Collections
A. Sorting Integers in Collections
Sorting integers in collections requires an understanding of sorting algorithms and their impact on performance.
List<Integer> integerList = Arrays.asList(5, 3, 8, 1, 7);
Collections.sort(integerList);
B. Custom Comparators for Complex Sorting
For custom sorting criteria, implementing a Comparator allows developers to define specific rules for ordering integers.
List<Integer> customSortList = Arrays.asList(5, 3, 8, 1, 7);
Collections.sort(customSortList, (num1, num2) -> num2 - num1);
C. Tips for Optimizing Collection-Based Comparisons
Optimizing comparisons within collections involves considering the size of the dataset and selecting the most efficient algorithms.
// Choose the right sorting algorithm based on the size of the collection
VII. Advanced Techniques
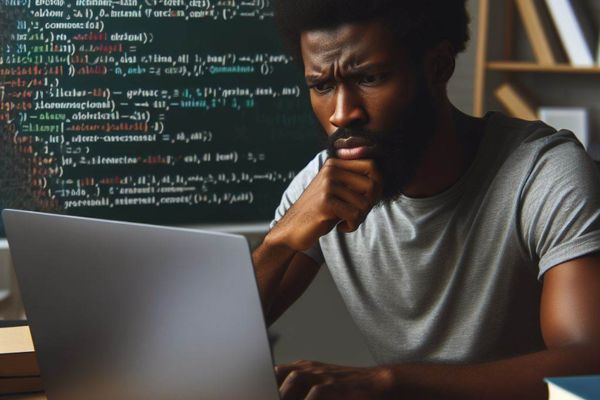
A. Bitwise Comparison for Efficiency
Bitwise comparison is a low-level technique that can significantly improve the efficiency of integer comparisons.
int bitwiseNum1 = 5;
int bitwiseNum2 = 3;
int bitwiseResult = bitwiseNum1 & bitwiseNum2; // bitwise AND operation
B. Exploring the Use of Comparator Interface
The Comparator interface provides a way to implement custom comparison logic, offering flexibility in sorting and ordering.
List<Integer> customComparatorList = Arrays.asList(5, 3, 8, 1, 7);
Collections.sort(customComparatorList, Comparator.reverseOrder());
C. Leveraging Third-Party Libraries for Specialized Comparisons
Third-party libraries like Apache Commons Lang provide utilities for specialized integer comparisons, saving development time and effort.
// Example of using Apache Commons Lang for specialized comparisons
import org.apache.commons.lang3.builder.CompareToBuilder;
public class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Student other) {
return new CompareToBuilder()
.append(this.name, other.name)
.append(this.age, other.age)
.toComparison();
}
public static void main(String[] args) {
Student student1 = new Student("Alice", 20);
Student student2 = new Student("Bob", 22);
Student student3 = new Student("Alice", 22);
// Using compareTo method for specialized comparison
int result1 = student1.compareTo(student2);
int result2 = student1.compareTo(student3);
System.out.println("Comparison Result 1: " + result1);
System.out.println("Comparison Result 2: " + result2);
if (result1 < 0) {
System.out.println(student1.getName() + " comes before " + student2.getName());
} else if (result1 > 0) {
System.out.println(student1.getName() + " comes after " + student2.getName());
} else {
System.out.println(student1.getName() + " and " + student2.getName() + " are equal");
}
if (result2 < 0) {
System.out.println(student1.getName() + " comes before " + student3.getName());
} else if (result2 > 0) {
System.out.println(student1.getName() + " comes after " + student3.getName());
} else {
System.out.println(student1.getName() + " and " + student3.getName() + " are equal");
}
}
public String getName() {
return name;
}
}
VIII. Real-World Examples
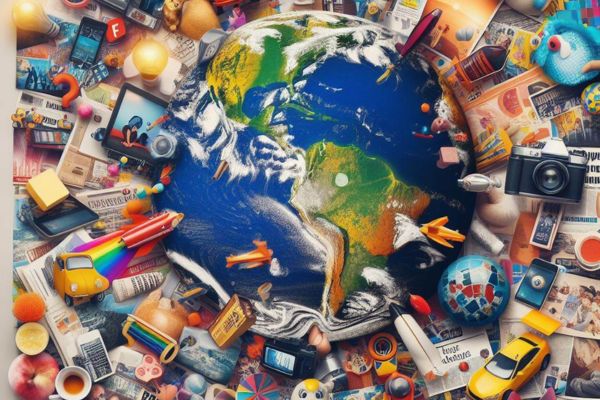
A. Practical Scenarios for Integer Comparison
Explore real-world scenarios where integer comparison plays a pivotal role, such as sorting algorithms, searching, and data analysis.
// Implementing integer comparison in a real-world scenario
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Employee implements Comparable<Employee> {
private String name;
private int yearsOfExperience;
public Employee(String name, int yearsOfExperience) {
this.name = name;
this.yearsOfExperience = yearsOfExperience;
}
@Override
public int compareTo(Employee other) {
// Compare employees based on years of experience
return Integer.compare(this.yearsOfExperience, other.yearsOfExperience);
}
public static void main(String[] args) {
// Create a list of employees
List<Employee> employees = new ArrayList<>();
employees.add(new Employee("Alice", 5));
employees.add(new Employee("Bob", 8));
employees.add(new Employee("Charlie", 3));
// Sort the list of employees based on years of experience
Collections.sort(employees);
// Display the sorted list
System.out.println("Sorted list of employees based on years of experience:");
for (Employee employee : employees) {
System.out.println(employee.getName() + " - " + employee.getYearsOfExperience() + " years of experience");
}
}
public String getName() {
return name;
}
public int getYearsOfExperience() {
return yearsOfExperience;
}
}
B. Code Snippets for Better Understanding
Providing practical code snippets enhances understanding and helps developers implement effective integer comparison in their projects.
// Code snippets for various integer comparison scenarios
1. Simple Integer Comparison:
public class IntegerComparison {
public static void main(String[] args) {
int num1 = 10;
int num2 = 20;
// Compare two integers
if (num1 < num2) {
System.out.println(num1 + " is less than " + num2);
} else if (num1 > num2) {
System.out.println(num1 + " is greater than " + num2);
} else {
System.out.println(num1 + " is equal to " + num2);
}
}
}
2. Sorting Integer Array:
import java.util.Arrays;
public class IntegerArraySorting {
public static void main(String[] args) {
int[] numbers = {30, 10, 50, 20, 40};
// Sort the array in ascending order
Arrays.sort(numbers);
// Print the sorted array
System.out.println("Sorted Array: " + Arrays.toString(numbers));
}
}
3. Integer Comparison in a List:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class IntegerListComparison {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
numbers.add(40);
// Sort the list in ascending order
Collections.sort(numbers);
// Print the sorted list
System.out.println("Sorted List: " + numbers);
}
}
4. Integer Comparison using Objects:
import java.util.Comparator;
public class ObjectIntegerComparison {
public static void main(String[] args) {
// Custom class for objects with an integer field
class CustomObject {
private int value;
public CustomObject(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
// Comparator for comparing objects based on integer field
Comparator<CustomObject> comparator = Comparator.comparingInt(CustomObject::getValue);
CustomObject obj1 = new CustomObject(30);
CustomObject obj2 = new CustomObject(10);
// Compare objects using the comparator
int result = comparator.compare(obj1, obj2);
// Print the comparison result
System.out.println("Comparison Result: " + result);
}
}
C. Handling Large Datasets Efficiently
Efficiency becomes crucial when dealing with large datasets. Learn techniques to optimize integer comparisons for improved performance.
// Strategies for handling large datasets in integer comparison
IX. Java 8 and Beyond
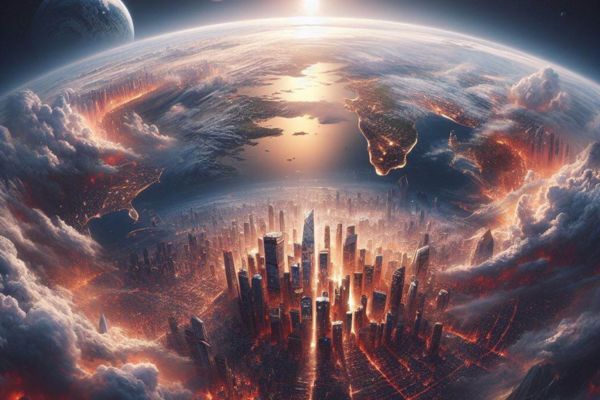
A. Overview of the Comparator.comparing() Method
Java 8 introduced the Comparator.comparing() method, simplifying the process of creating comparators for objects with natural ordering.
List<String> stringList = Arrays.asList("Apple", "Banana", "Orange", "Grapes");
stringList.sort(Comparator.comparing(String::length));
B. Stream API for Streamlined Comparisons
The Stream API in Java 8 offers a concise and expressive way to perform operations on sequences of elements, including comparisons.
List<Integer> streamList = Arrays.asList(5, 3, 8, 1, 7);
int sum = streamList.stream().mapToInt(Integer::intValue).sum();
C. Lambdas and Functional Programming for Elegant Solutions
Utilize lambdas and functional programming paradigms to create concise and elegant solutions for integer comparison.
List<Integer> lambdaList = Arrays.asList(5, 3, 8, 1, 7);
lambdaList.forEach(num -> System.out.print(num + " "));
X. Conclusion
X. Conclusion
A. Recap of Key Points
Summarize the key takeaways, emphasizing the importance of choosing the right method for integer comparison based on the specific use case.
B. Emphasizing the Importance of Proper Integer Comparison in Java
Reiterate the significance of proper integer comparison in writing robust and efficient Java code.