Java projects offer a practical way to enhance your programming skills. Working on these projects can provide valuable hands-on experience with core Java concepts and techniques.
1. To-Do List Application

A to-do list application is a practical starting point for novice Java developers. This project involves creating a user interface that lets users add, delete, and update tasks. You’ll gain experience with arrays or database integration, user input handling, and GUI development using Swing or JavaFX.
Start by implementing a basic task list that supports adding tasks. Store tasks in an array or a simple linked list. As you progress, integrate features like deleting and updating tasks.
- Add date functionalities to set task deadlines or reminders using Java’s Date and Calendar classes.
- Consider color-coding tasks based on priority levels for a more intuitive interface.
- Use file I/O operations to save the task list for persistent storage.
- Advanced developers can integrate a database using JDBC to connect to SQL.
To further challenge your skills, integrate notifications for task reminders using a background timer or scheduler. This will teach you threading and concurrency in Java.
2. Quiz Application

Developing a quiz application allows you to explore Java programming concepts. Start with a fixed set of questions and build a basic interface to display these questions and collect user responses. Use data structures to store questions and answers, and design a GUI using Swing or JavaFX.
Implement features such as:
- Randomizing questions
- Tracking scores
- Displaying results
This will give you experience with Java’s randomization features and event handling.
Consider adding multiplayer support to deepen your understanding of Java’s concurrency and multi-threading capabilities. Each user instance can run on a separate thread, allowing you to manage multiple quiz sessions simultaneously.
Integrate a feature where users can add their own questions. This could involve creating a form for input or building a simple database to store user-generated content. Use JDBC for database integration.
Enhance the user interface with progress bars or a countdown timer for each question. This will require utilizing Java’s Timer and TimerTask classes.
“Finally, deploy your application on a web server or as a standalone desktop application to demonstrate your competence in Java’s core and advanced features.”
3. Time Zone Converter

A time zone converter application strengthens your understanding of GUI development with Swing and API integration. Design a user interface with input fields for the current time and time zone selections. Incorporate a button to trigger the conversion process.
Leverage APIs such as the World Time API or Google Time Zone API for accurate time conversions. This will give you experience with Java’s networking capabilities, including making HTTP requests and parsing JSON or XML responses.
Use Java’s java.time
package for handling and manipulating date and time across different time zones. Implement multiple time zone conversions within the same session and add functionality to save recent conversions.
Enhance the user experience with:
- Error handling
- Validations
- Auto-complete feature for time zone selection
Implement timezone conversion logging using File I/O operations or a lightweight database like SQLite through JDBC.
For an additional challenge, add a feature that accounts for daylight saving changes, demonstrating your ability to implement more complex business logic.
4. Memory Game

Creating a memory game pushes your Java skills into game development and graphics handling. Design a graphical interface where users flip cards to match pairs within a grid.
Set up the GUI using Java’s Swing library with a grid layout. Use JPanel
for the grid and JButton
for each card. Implement action listeners to flip cards and reveal images.
Incorporate a timer to track gameplay duration using javax.swing.Timer
. Implement a scoring system that rewards successful matches and penalizes failed attempts.
Add features like:
- Highlighting matched pairs
- Disabling successfully paired cards
- Including a leaderboard to track high scores or fastest completion times
Enhance the game with sound effects using javax.sound.sampled
for card flips or matches. Polish the look and feel by customizing GUI components with different fonts, colors, and layouts.
5. Tic-Tac-Toe Game

Develop a tic-tac-toe game starting with a console-based version and progressing to a GUI version. Create a 3×3 board using a two-dimensional array to store player moves and check for winning conditions.
Implement a loop to alternate between player turns, using conditionals to check for valid moves and determine game outcomes. Write methods to check rows, columns, and diagonals for three identical symbols.
Transition to a GUI-based application using Swing or JavaFX. Create a visual board representation using buttons within a grid layout. Use action listeners to handle button clicks and update the game state.
Add a status bar to provide feedback to players. Incorporate features like:
- Highlighting the winning line
- Implementing an AI opponent using algorithms like minimax
Include a restart button to reset the game board for a new round. This project solidifies your understanding of Java’s core concepts and provides practice in managing game logic, user interactions, and GUI components.
Did you know? The first computer program to play Tic-Tac-Toe was written in 1952 by A.S. Douglas as part of his Ph.D. thesis at the University of Cambridge.
6. Contact Book

A contact book application is a practical project that enhances understanding of object-oriented programming, file handling, and data structures like ArrayLists. It involves implementing functionalities to add, delete, update, and search for contacts.
Start by designing the Contact class:
public class Contact {
private String name;
private String phone;
private String email;
private String address;
// Constructor, getters, and setters
}
Create the ContactBook class to manage contacts:
import java.util.ArrayList;
public class ContactBook {
private ArrayList contacts;
public ContactBook() {
this.contacts = new ArrayList<>();
}
public void addContact(Contact contact) {
contacts.add(contact);
}
public void deleteContact(String name) {
// Iterate through the list to find and remove the contact
}
public void updateContact(String name, Contact updatedContact) {
// Find the contact and update its details
}
public Contact searchContact(String name) {
// Return the contact that matches the search criteria
}
// Additional helper methods
}
Implement methods for adding, deleting, updating, and searching contacts. Use file handling to ensure contacts are retained across sessions:
import java.io.*;
import java.util.stream.Collectors;
public class ContactBook {
// Existing code
public void saveContactsToFile(String filename) throws IOException {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(filename))) {
for (Contact contact : contacts) {
writer.write(contact.toString());
writer.newLine();
}
}
}
public void loadContactsFromFile(String filename) throws IOException {
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
contacts = reader.lines()
.map(line -> parseContact(line))
.collect(Collectors.toCollection(ArrayList::new));
}
}
private Contact parseContact(String line) {
// Parse contact details from a line and return a Contact object
}
}
Create a simple text-based or graphical user interface to interact with the contact book. Consider adding sorting and filtering options to enhance functionality.
This project offers a comprehensive exploration of OOP principles, data management, user interactivity, and file handling, making it a valuable addition to a beginner’s portfolio.
7. Number Guessing Game

A number guessing game is a good project for beginner Java developers to understand control flow, loops, and conditional logic. The system generates a random number, and the player guesses what it is within a specified range.
Here’s a basic implementation:
import java.util.Random;
import java.util.Scanner;
public class NumberGuessingGame {
public static void main(String[] args) {
Random random = new Random();
int numberToGuess = random.nextInt(100) + 1;
int numberOfTries = 0;
Scanner scanner = new Scanner(System.in);
int guess;
boolean win = false;
while (!win) {
System.out.println("Guess a number between 1 and 100:");
guess = scanner.nextInt();
numberOfTries++;
if (guess == numberToGuess) {
win = true;
} else if (guess < numberToGuess) {
System.out.println("Too low. Try again.");
} else {
System.out.println("Too high. Try again.");
}
}
System.out.println("You win! The number was " + numberToGuess);
System.out.println("It took you " + numberOfTries + " tries.");
}
}
To add more challenge, implement a limit on the number of guesses:
public class NumberGuessingGame {
public static void main(String[] args) {
Random random = new Random();
int numberToGuess = random.nextInt(100) + 1;
int numberOfTries = 0;
int maxAttempts = 10;
Scanner scanner = new Scanner(System.in);
int guess;
boolean win = false;
while (numberOfTries < maxAttempts && !win) {
System.out.println("Guess a number between 1 and 100, attempt " +
(numberOfTries + 1) + " of " + maxAttempts + ":");
guess = scanner.nextInt();
numberOfTries++;
if (guess == numberToGuess) {
win = true;
break;
} else if (guess < numberToGuess) {
System.out.println("Too low. Try again.");
} else {
System.out.println("Too high. Try again.");
}
}
if (win) {
System.out.println("You win! The number was " + numberToGuess);
System.out.println("It took you " + numberOfTries + " tries.");
} else {
System.out.println("You've used all " + maxAttempts + " attempts. The number was " + numberToGuess);
}
}
}
Consider adding features like:
- Replay options
- A GUI
- A high score tracker
This project combines basic programming concepts with an interactive application, reinforcing understanding of Java features like control flow, loops, and conditions. It’s an excellent way to practice problem-solving skills and logical thinking1.
“Games like this are not just fun, but they’re excellent tools for learning programming concepts and logic.”
8. Currency Converter

A currency converter application is a practical project for beginners in Java. It covers fundamental concepts like handling user input, performing calculations, and integrating external APIs. The goal is to create a program that converts amounts from one currency to another, based on either predefined exchange rates or real-time data.
Basic Setup
Design a simple user interface using Java Swing or JavaFX. Include input fields for the amount, dropdown menus for selecting currencies, and a conversion button.
Handling User Input
Capture and validate user inputs. Ensure the user enters a valid numerical value and selects both currencies before conversion.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class CurrencyConverter extends JFrame {
private JTextField amountField;
private JComboBox fromCurrency;
private JComboBox toCurrency;
private JLabel resultLabel;
public CurrencyConverter() {
// GUI setup
setTitle("Currency Converter");
setSize(300, 200);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(null);
// UI components
amountField = new JTextField();
fromCurrency = new JComboBox<>(new String[]{"USD", "EUR", "GBP"});
toCurrency = new JComboBox<>(new String[]{"USD", "EUR", "GBP"});
JButton convertButton = new JButton("Convert");
resultLabel = new JLabel("Result: ");
// Positioning
amountField.setBounds(50, 30, 100, 25);
fromCurrency.setBounds(160, 30, 70, 25);
toCurrency.setBounds(160, 60, 70, 25);
convertButton.setBounds(100, 100, 90, 25);
resultLabel.setBounds(50, 130, 200, 25);
// Adding components
add(amountField);
add(fromCurrency);
add(toCurrency);
add(convertButton);
add(resultLabel);
// Button action
convertButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
convertCurrency();
}
});
}
private void convertCurrency() {
try {
double amount = Double.parseDouble(amountField.getText());
String from = (String) fromCurrency.getSelectedItem();
String to = (String) toCurrency.getSelectedItem();
if (from.equals(to)) {
resultLabel.setText("Result: " + amount);
} else {
double rate = getConversionRate(from, to);
double result = amount * rate;
resultLabel.setText("Result: " + result);
}
} catch (NumberFormatException ex) {
resultLabel.setText("Invalid input");
}
}
private double getConversionRate(String from, String to) {
// Dummy predefined rates
if (from.equals("USD") && to.equals("EUR")) return 0.85;
else if (from.equals("USD") && to.equals("GBP")) return 0.75;
// Other conversions as needed
return 1.0; // fallback
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new CurrencyConverter().setVisible(true));
}
}
Mathematical Operations
Perform the actual currency conversion by multiplying the input amount by the conversion rate.
Predefined Exchange Rates
Start with predefined rates for a controlled learning environment.
Real-Time Exchange Rates
For a more advanced version, integrate an external API to fetch up-to-date conversion rates. Use Java’s HttpURLConnection
or libraries like HttpClient
to send HTTP requests to a currency converter service.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class CurrencyConverter {
// Existing code...
private double getConversionRate(String from, String to) {
double rate = 1.0; // Default rate
try {
String apiUrl = "http://api.exchangeratesapi.io/v1/latest?access_key=YOUR_API_KEY&symbols=" + to + "&base=" + from;
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder content = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
conn.disconnect();
JSONObject json = new JSONObject(content.toString());
rate = json.getJSONObject("rates").getDouble(to);
} catch (Exception e) {
e.printStackTrace();
}
return rate;
}
// Additional logic and main method...
}
Error Handling
Implement error handling for scenarios where the API is unreachable or returns an error. Display appropriate messages to inform the user of any issues.
User-Friendly Extensions
Consider adding features such as:
- Historical rate charts
- Input suggestions
- A favorites system for commonly converted currencies
9. Text Editor

Creating a text editor application is a useful project for beginner Java developers to improve their understanding of GUI development using Swing. This project involves implementing a user-friendly interface with basic text editing functionalities.
Basic Setup
Set up the main JFrame for your application with a JTextArea as the main editing area.
import javax.swing.*;
public class TextEditor extends JFrame {
private JTextArea textArea;
public TextEditor() {
setTitle("Simple Text Editor");
setSize(500, 500);
setDefaultCloseOperation(EXIT_ON_CLOSE);
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane);
createMenuBar();
}
private void createMenuBar() {
// Menu bar setup goes here
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new TextEditor().setVisible(true));
}
}
Adding Functionalities
Build a menu bar with items like File, Edit, and Format. Include options for changing text size and color, and toggling bold, italic, or underlined text.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.text.*;
public class TextEditor extends JFrame {
private JTextArea textArea;
public TextEditor() {
setTitle("Simple Text Editor");
setSize(500, 500);
setDefaultCloseOperation(EXIT_ON_CLOSE);
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane);
createMenuBar();
}
private void createMenuBar() {
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem saveItem = new JMenuItem("Save");
fileMenu.add(saveItem);
JMenu editMenu = new JMenu("Edit");
JMenuItem cutItem = new JMenuItem("Cut");
editMenu.add(cutItem);
JMenu formatMenu = new JMenu("Format");
JMenuItem boldItem = new JMenuItem("Bold");
JMenuItem italicItem = new JMenuItem("Italic");
JMenuItem underlineItem = new JMenuItem("Underline");
JMenuItem colorItem = new JMenuItem("Text Color");
formatMenu.add(boldItem);
formatMenu.add(italicItem);
formatMenu.add(underlineItem);
formatMenu.add(colorItem);
menuBar.add(fileMenu);
menuBar.add(editMenu);
menuBar.add(formatMenu);
setJMenuBar(menuBar);
saveItem.addActionListener(e -> saveFile());
cutItem.addActionListener(e -> textArea.cut());
boldItem.addActionListener(e -> textArea.setFont(
textArea.getFont().deriveFont(Font.BOLD)));
italicItem.addActionListener(e -> textArea.setFont(
textArea.getFont().deriveFont(Font.ITALIC)));
underlineItem.addActionListener(e -> textArea.setFont(
textArea.getFont().deriveFont(Font.PLAIN)));
colorItem.addActionListener(e -> {
Color color = JColorChooser.showDialog(this, "Choose Text Color", Color.BLACK);
textArea.setForeground(color);
});
}
private void saveFile() {
JFileChooser fileChooser = new JFileChooser();
int option = fileChooser.showSaveDialog(this);
if (option == JFileChooser.APPROVE_OPTION) {
try {
File file = fileChooser.getSelectedFile();
FileWriter writer = new FileWriter(file);
writer.write(textArea.getText());
writer.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new TextEditor().setVisible(true));
}
}
Key Features
- Text Manipulation: Implement event listeners for the menu items to handle text formatting operations.
- File Saving and Loading: Integrate file saving and loading functionalities using
JFileChooser
,FileWriter
, andFileReader
classes. - Enhancing User Experience: Add options for changing font sizes and styles using dialogs or combo boxes.
This project provides hands-on experience with GUI development, event handling, text manipulation, and file I/O operations, resulting in a useful application for your portfolio. It’s an excellent way to practice real-world programming skills while creating a tool that you might actually use in your daily life.
10. Library Management System

Creating a library management system involves database connectivity and user interface design. This project manages book inventories, member registrations, and tracks borrowing and returning of books. It uses Java’s JDBC and Swing or JavaFX libraries.
Setup and Database Design
Design a database to store information about books, members, and transactions. Use MySQL or SQLite. Create tables for books, members, and transactions. Use JDBC for database connectivity.
CREATE TABLE Books (
bookID INT PRIMARY KEY,
title VARCHAR(100),
author VARCHAR(100),
genre VARCHAR(50),
quantity INT
);
CREATE TABLE Members (
memberID INT PRIMARY KEY,
name VARCHAR(100),
contact VARCHAR(100)
);
CREATE TABLE Transactions (
transactionID INT PRIMARY KEY,
bookID INT,
memberID INT,
borrowDate DATE,
returnDate DATE,
FOREIGN KEY (bookID) REFERENCES Books(bookID),
FOREIGN KEY (memberID) REFERENCES Members(memberID)
);
Database Connectivity with JDBC
Write Java classes to handle database operations. Develop methods for connecting to the database, adding new books and members, borrowing books, and returning them. Use prepared statements to prevent SQL injection.
import java.sql.*;
public class Database {
private Connection connect() {
String url = "jdbc:sqlite:library.db";
Connection conn = null;
try {
conn = DriverManager.getConnection(url);
} catch (SQLException e) {
System.out.println(e.getMessage());
}
return conn;
}
public void addBook(int bookID, String title, String author, String genre, int quantity) {
String sql = "INSERT INTO Books(bookID, title, author, genre, quantity) VALUES(?,?,?,?,?)";
try (Connection conn = this.connect();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, bookID);
pstmt.setString(2, title);
pstmt.setString(3, author);
pstmt.setString(4, genre);
pstmt.setInt(5, quantity);
pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
// Methods for adding members, borrowing and returning books, etc.
}
User Interface Design
Design the user interface using Swing or JavaFX. Create forms for adding new books and members, and for borrowing and returning books. Use JTable
to display lists of books and members.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LibraryManagementSystem extends JFrame {
private Database db;
private JTextField titleField;
private JTextField authorField;
private JTextField genreField;
private JTextField quantityField;
public LibraryManagementSystem() {
db = new Database();
setTitle("Library Management System");
setSize(600, 400);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(null);
JLabel titleLabel = new JLabel("Title:");
titleLabel.setBounds(50, 50, 100, 25);
add(titleLabel);
titleField = new JTextField();
titleField.setBounds(150, 50, 200, 25);
add(titleField);
JLabel authorLabel = new JLabel("Author:");
authorLabel.setBounds(50, 100, 100, 25);
add(authorLabel);
authorField = new JTextField();
authorField.setBounds(150, 100, 200, 25);
add(authorField);
JLabel genreLabel = new JLabel("Genre:");
genreLabel.setBounds(50, 150, 100, 25);
add(genreLabel);
genreField = new JTextField();
genreField.setBounds(150, 150, 200, 25);
add(genreField);
JLabel quantityLabel = new JLabel("Quantity:");
quantityLabel.setBounds(50, 200, 100, 25);
add(quantityLabel);
quantityField = new JTextField();
quantityField.setBounds(150, 200, 200, 25);
add(quantityField);
JButton addButton = new JButton("Add Book");
addButton.setBounds(150, 250, 150, 25);
add(addButton);
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int bookID = new java.util.Random().nextInt(1000); // Generate a random book ID
String title = titleField.getText();
String author = authorField.getText();
String genre = genreField.getText();
int quantity = Integer.parseInt(quantityField.getText());
db.addBook(bookID, title, author, genre, quantity);
JOptionPane.showMessageDialog(null, "Book Added Successfully");
}
});
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new LibraryManagementSystem().setVisible(true));
}
}
Borrowing and Returning Books
Implement functionality for borrowing and returning books. Add checks to ensure a member does not borrow more books than allowed and that books are available before borrowing. Update the database accordingly.
public void borrowBook(int memberID, int bookID) {
String sql = "INSERT INTO Transactions(memberID, bookID, borrowDate) VALUES(?,?,CURRENT_DATE)";
String updateBookSql = "UPDATE Books SET quantity = quantity - 1 WHERE bookID = ? AND quantity > 0";
try (Connection conn = this.connect();
PreparedStatement pstmt = conn.prepareStatement(sql);
PreparedStatement updateBookStmt = conn.prepareStatement(updateBookSql)) {
conn.setAutoCommit(false); // transaction block
pstmt.setInt(1, memberID);
pstmt.setInt(2, bookID);
pstmt.executeUpdate();
updateBookStmt.setInt(1, bookID);
int rows = updateBookStmt.executeUpdate();
if (rows == 0) throw new SQLException("Book not available");
conn.commit();
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
public void returnBook(int memberID, int bookID) {
String sql = "UPDATE Transactions SET returnDate = CURRENT_DATE WHERE memberID = ? AND bookID = ?";
String updateBookSql = "UPDATE Books SET quantity = quantity + 1 WHERE bookID = ?";
try (Connection conn = this.connect();
PreparedStatement pstmt = conn.prepareStatement(sql);
PreparedStatement updateBookStmt = conn.prepareStatement(updateBookSql)) {
pstmt.setInt(1, memberID);
pstmt.setInt(2, bookID);
pstmt.executeUpdate();
updateBookStmt.setInt(1, bookID);
updateBookStmt.executeUpdate();
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
Enhancing the System
Consider adding features to handle overdue books and notifications. Use Java Mail API to send email reminders for overdue books. Include a search functionality for books and members.
This project provides experience with database connectivity, GUI design, and application logic in a practical context. Here are some key benefits:
- Real-world application: Simulates a system used in actual libraries
- Database management: Teaches proper handling of data storage and retrieval
- User interface design: Provides practice in creating intuitive and functional GUIs
- Error handling: Implements checks and balances to maintain data integrity
- Scalability: Can be expanded with additional features like fine calculation or book reservations
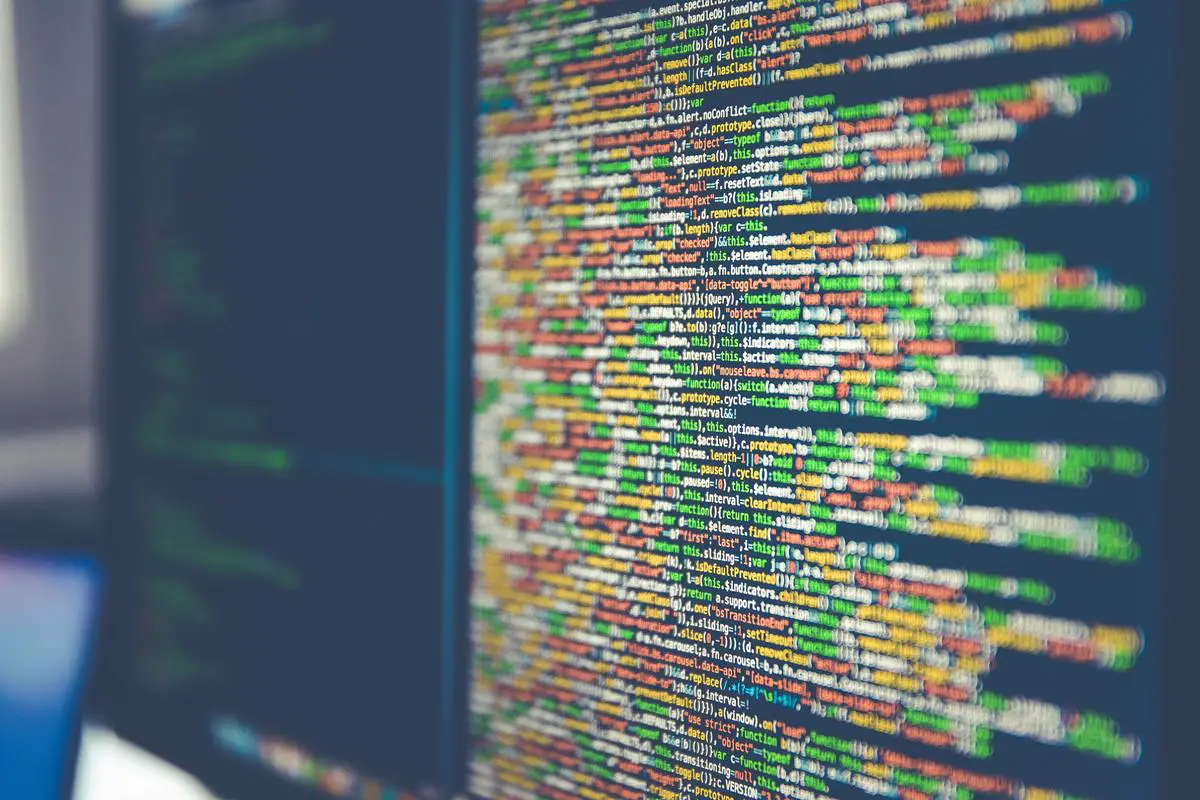
Photo by markusspiske on Unsplash
Each project mentioned provides a stepping stone towards mastering Java. By engaging with these applications, you improve your coding abilities and create tangible examples of your work that can be showcased in a portfolio. Remember, the journey to becoming a proficient Java developer is a continuous learning process, and these projects serve as excellent milestones along the way.
Spring MVC Ajax JSON Response Example: A Professional Guide
Understanding JSON Parsing with JavaScript
Java Common Interview Questions
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.