Java is one of the most popular programming languages worldwide, and thousands of people prepare for Java interviews daily. Why not? Java offers vast career options for you! We are providing some common interview questions in Java to help you succeed in your interviews and secure a bright future.
Basic Java Questions
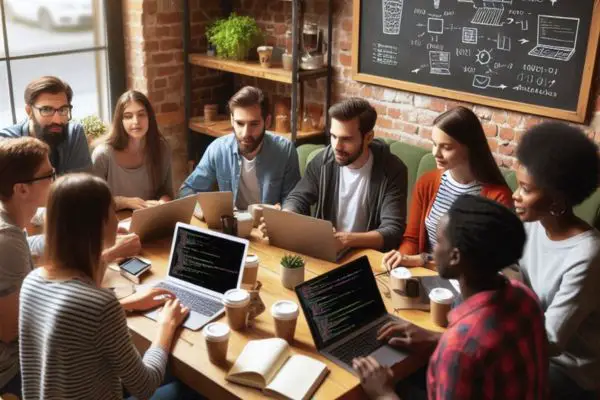
What is Java?
Java is a high-level, object-oriented programming language developed by Sun Microsystems and released in 1995. Designed to have as few implementation dependencies as possible, it follows the principle of “write once, run anywhere” (WORA), meaning that compiled Java code can run on any platform that supports Java without the need for recompilation.
Features of Java
Some of the key features of Java that make it powerful.
Object-Oriented: Java is based on the concept of objects, which can contain data (fields) and methods (functions). This approach helps in organizing and managing complex software projects.
Platform-Independent: Java code is compiled into bytecode, It allows it to run on any device that has a Java Virtual Machine (JVM) installed. This makes Java highly portable across different operating systems and hardware architectures.
Simple and Secure: Java is designed to be easy to learn and use, with a syntax similar to C++. It also incorporates various security features to prevent common programming errors and protect against malicious code.
Robust and Reliable: Java emphasizes early checking for possible errors, with features such as strong memory management, exception handling, and automatic garbage collection.
Multithreaded: Java supports multithreading, allowing concurrent execution of two or more threads (lightweight processes), which can improve the performance of applications.
Rich Standard Library: Java provides a vast standard library (Java API) that includes classes and methods for various tasks, such as data structures, networking, input/output operations, and graphical user interface (GUI) development.
Community and Ecosystem: Java has a large, active community of developers and a rich ecosystem of frameworks, libraries, and tools that enhance its capabilities and make development faster and easier.
Differences between Java and other programming languages
Java has several distinctive features that set it apart from other programming languages. Here are some key differences:
Platform Independence:
- Java: Compiled into bytecode, which can run on any device with a Java Virtual Machine (JVM), ensuring cross-platform compatibility.
- Other Languages: Many are compiled directly into machine code specific to an operating system or processor, requiring recompilation for different platforms.
Object-Oriented:
- Java: Purely object-oriented, meaning almost everything is an object. It strictly adheres to principles like inheritance, encapsulation, and polymorphism.
- Other Languages: Some languages like C++ are object-oriented but allow procedural programming as well. Others like C are purely procedural.
Memory Management:
- Java: Automatic memory management through garbage collection, which helps prevent memory leaks and simplifies development.
- Other Languages: Languages like C and C++ require manual memory management, which can lead to memory leaks and pointer errors if not handled carefully.
Security:
- Java: Designed with a strong emphasis on security, with built-in features like the Java Security Manager and bytecode verification.
- Other Languages: Security features vary widely. For instance, C and C++ lack many of the built-in security features found in Java.
Standard Library:
- Java: Comes with a comprehensive standard library (Java API) that supports a wide range of functionalities, including data structures, networking, and GUI development.
- Other Languages: While many have extensive libraries (like Python’s standard library or .NET framework for C#), the breadth and integration of Java’s standard library are notable.
Concurrency:
- Java: Provides built-in support for multithreading, making it easier to write concurrent applications.
- Other Languages: Concurrency support varies. For example, C++11 and later versions have improved concurrency features, but older versions required more effort. Python supports concurrency, but its Global Interpreter Lock (GIL) can be a limitation.
Syntax and Ease of Learning:
- Java: Has a syntax similar to C++, making it familiar to those who know C/C++ but designed to be simpler and more readable.
- Other Languages: Syntax varies widely. For instance, Python is known for its readability and simplicity, while languages like Perl are often criticized for syntactic complexity.
Performance:
- Java: Generally slower than languages like C++ because of the overhead of the JVM and garbage collection. However, Just-In-Time (JIT) compilation and other optimizations help improve performance.
- Other Languages: C and C++ are known for high performance due to direct compilation to machine code. Scripting languages like Python are typically slower due to interpretation at runtime.
Community and Ecosystem:
- Java: Benefits from a large, active community and a robust ecosystem, including many frameworks and tools (e.g., Spring, Hibernate).
- Other Languages: The size and activity of the community can vary. For example, Python also has a large and active community, while some niche languages may have smaller communities.
Use Cases:
- Java: Widely used for enterprise applications, Android app development, web applications, and large systems.
- Other Languages: Use cases vary. For instance, Python is popular in data science and web development, while C is often used in system programming and embedded systems.
Object-Oriented Programming Concepts
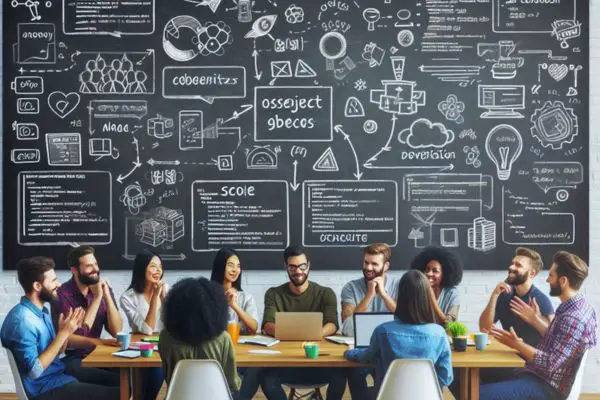
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of “objects,” which are instances of classes. OOP focuses on using objects to design and build applications. It allows for a modular programming approach and aims to make software more flexible, reusable, and easier to maintain.
Principles of OOP
- Inheritance: A mechanism where a new class inherits the properties and behavior of an existing class, promoting code reuse.
- Polymorphism: The ability of different classes to respond to the same method call in different ways, typically through method overriding and method overloading..
- Abstraction: Hiding the complex implementation details and exposing only the necessary parts, usually through abstract classes and interfaces.
- Encapsulation: Combining data and the methods that work on that data into a single unit, and restricting access to some of the object’s components.
Inheritance in Java
Inheritance is one of the core concepts of Object-Oriented Programming (OOP) in Java. It allows a class to inherit properties and behaviors (fields and methods) from another class. The class that is inherited from is called the superclass (or parent class), and the class that inherits from it is called the subclass (or child class).
Key Points of Inheritance:
- Code Reusability: Inheritance allows for the reuse of code, as methods and fields defined in a superclass are automatically available to any subclass.
- Method Overriding: Subclasses can provide specific implementations of methods that are already defined in their superclass.
- Polymorphism: Inheritance supports polymorphism, which allows methods to do different things based on the object it is acting upon, even when using a reference of the superclass type.
Syntax
To create a subclass in Java, you use the extends
keyword. Here’s a basic example:
// Superclass
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
// Subclass
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
In the example above, Dog
is a subclass of Animal
. It inherits the eat()
method from the Animal
class, and it also defines its own method bark()
.
Using Inherited Methods
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // Inherited method
myDog.bark(); // Subclass-specific method
}
}
When myDog.eat()
is called, it uses the eat()
method from the Animal
class. When myDog.bark()
is called, it uses the bark()
method from the Dog
class.
Method Overriding
A subclass can override a method from its superclass to provide a specific implementation:
class Dog extends Animal {
@Override
void eat() {
System.out.println("The dog eats bones.");
}
}
In this case, when myDog.eat()
is called, it will output “The dog eats bones.”
Super Keyword
The super
keyword is used to refer to the immediate parent class object. It is often used to access superclass methods or constructors.
class Animal {
Animal() {
System.out.println("Animal constructor called");
}
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
Dog() {
super(); // Calls the constructor of the superclass
System.out.println("Dog constructor called");
}
@Override
void eat() {
super.eat(); // Calls the superclass method
System.out.println("The dog eats bones.");
}
}
When creating a Dog
object, the output will be:
Animal constructor called
Dog constructor called
And when calling myDog.eat()
, the output will be:
This animal eats food.
The dog eats bones.
Inheritance Hierarchies
Classes can inherit from other classes in a hierarchy. However, Java does not support multiple inheritance (a class cannot inherit from more than one class). Java supports single inheritance, but it allows multiple inheritance through interfaces.
Example of an Inheritance Hierarchy:
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Mammal extends Animal {
void walk() {
System.out.println("This mammal walks.");
}
}
class Dog extends Mammal {
void bark() {
System.out.println("The dog barks.");
}
}
In this hierarchy, Dog
inherits from Mammal
, and Mammal
inherits from Animal
.
Polymorphism in Java
Polymorphism in Java means that objects of different types can be treated as instances of a shared superclass. There are two main types:
- Compile-time Polymorphism (Method Overloading): This occurs when there are multiple methods with the same name but different parameters in the same class. The correct method is chosen at compile time based on the arguments used to call the method.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In the example above, add
method is overloaded with different parameter types (int
and double
).
- Runtime Polymorphism (Method Overriding): This occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The method that gets executed is determined at runtime based on the object that is invoking it.
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
public void sound() {
System.out.println("Dog barks");
}
}
Here, Dog
overrides the sound
method of Animal
.
Polymorphism allows for flexibility in designing and using Java classes, promoting code reusability and abstraction.
Abstraction and Encapsulation
Abstraction hides the complicated parts and shows only the important features of an object. Encapsulation, on the other hand, is about bundling the data and methods operating on the data into a single unit or class, and restricting the access to some of the object’s components.
Java Basics
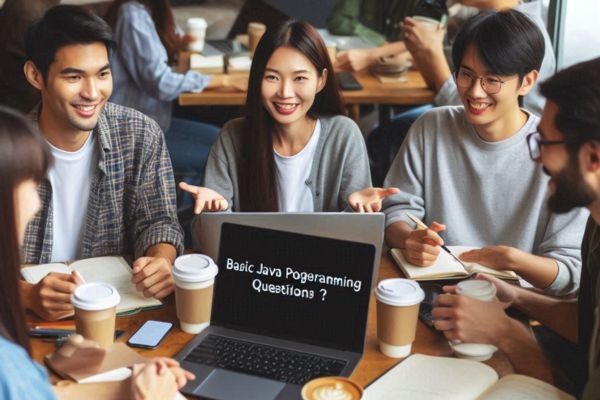
Java Data Types
Java supports various data types, including:
- Primitive Types: int, byte, short, long, float, double, boolean, char.
- Reference Types: Objects, Arrays.
Variables in Java
Variables are containers that store data values. In Java, you need to declare the type of variable before using it.
Operators in Java
Java provides various operators to perform operations on variables and values, such as:
Arithmetic Operators: +, -, *, /, %.
Relational Operators: ==, !=, >, <, >=, <=.
Logical Operators: &&, ||, !.
Control Statements
Control statements manage the flow of execution in a program by determining the sequence in which code statements are executed. These include:
Conditional Statements: if, else if, else, switch.
Looping Statements: for, while, do-while.
Java Arrays
Arrays in Java store multiple values in one variable, rather than using separate variables for each value.
Advanced Java Concepts
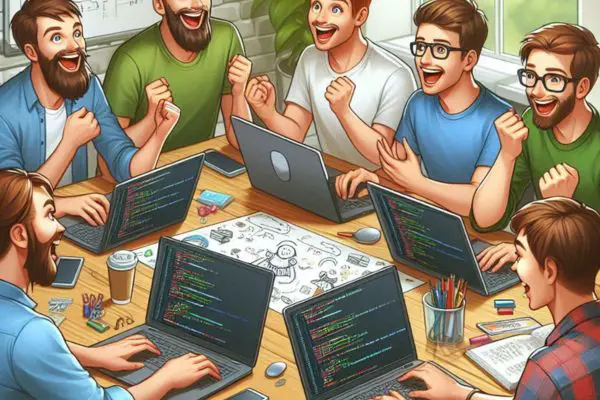
Exception Handling
Exception handling in Java is a robust mechanism for managing runtime errors, ensuring that the application functions smoothly. Key keywords include try, catch, throw, throws, and finally.
Multithreading
Multithreading is a Java feature that facilitates the simultaneous execution of multiple threads. It helps in performing multiple operations simultaneously, enhancing performance.
Collections Framework
The Java Collections Framework offers a set of interfaces and classes to store and manage groups of data as a single unit. Examples include ArrayList, HashSet, and HashMap.
Generics in Java
Generics allow types (classes and interfaces) to be used as parameters when defining classes, interfaces, and methods. This allows for stronger type checks at compile time and eliminates the need for type casting.
Java 8 Features
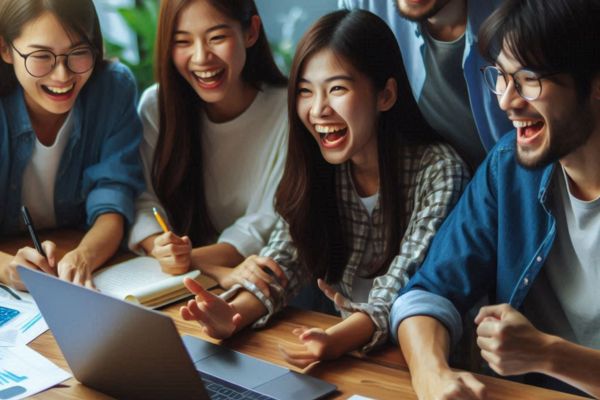
Lambda Expressions
Lambda expressions, introduced in Java 8, offer a clear and concise way to represent a single-method interface using an expression. They enable you to treat functionality as a method argument or pass a block of code around.
Stream API
The Stream API allows for processing sequences of elements in a declarative manner, enabling operations like filter, map, and reduce on collections.
Functional Interfaces
In Java, a functional interface is defined as an interface with only a single abstract method. They enable lambda expressions to be used in Java.
Default Methods
Default methods in interfaces allow you to add new functionalities to interfaces without breaking the classes that implement these interfaces.
Optional Class
The Optional class provides a container that may or may not hold a non-null value. It is used to represent optional values instead of null.
Java Programming and Coding Questions
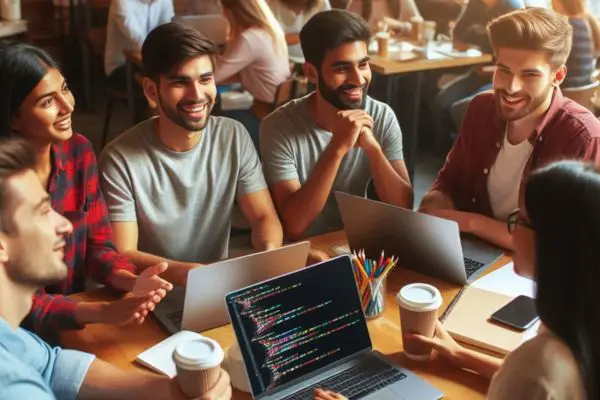
Common Coding Problems
Prepare to solve coding problems such as:
Palindrome Check: Write a method to check if a given string is a palindrome.
Fibonacci Series: Generate a Fibonacci series up to a given number.
Sorting Algorithms: Implement sorting algorithms like Quick Sort, Merge Sort, etc.
How to Approach Coding Questions
Understand the problem, plan your solution, write the code, and then test it thoroughly. Always consider edge cases and optimize your code for better performance.
Examples of Coding Questions
Write a program to reverse a string.
Implement a stack using arrays.
Find the largest element in an array.
Java Frameworks and Libraries
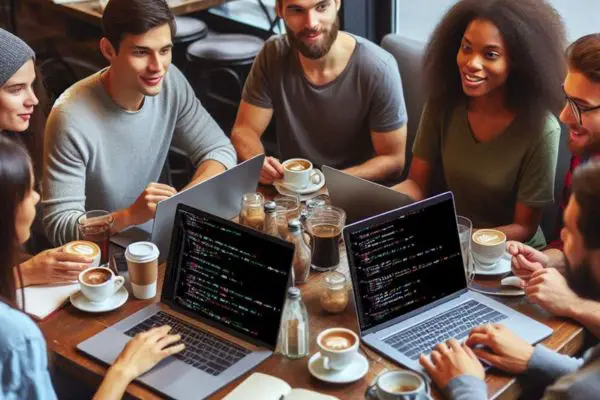
Introduction to Java Frameworks
Java frameworks provide pre-written code to help you build applications more efficiently. They often come with tools and libraries that streamline development.
Spring Framework
The Spring Framework is a powerful, feature-rich framework for building enterprise-level applications. It supports dependency injection, transaction management, and more.
Hibernate
Hibernate is an object-relational mapping (ORM) framework that makes database interactions easier in Java applications.
Popular Java Libraries
Some popular Java libraries include Apache Commons, Google Guava, and Jackson for JSON processing.
Java Development Tools
Integrated Development Environments (IDEs)
Popular Java IDEs include Eclipse, IntelliJ IDEA, and NetBeans, offering features like code completion, debugging, and more.
Build Tools
Maven and Gradle are widely used build tools in Java that help manage project dependencies and automate the build process.
Version Control Systems
Git is the most popular version control system, used for tracking changes in source code during software development.
Database Connectivity
JDBC Overview
Java Database Connectivity (JDBC) is an API that enables connecting to a database and executing queries.
Connecting to a Database
Using JDBC, you can connect to a database using a connection string and perform CRUD (Create, Read, Update, Delete) operations.
CRUD Operations
CRUD operations are the basic functions of persistent storage. In Java, these can be implemented using SQL commands through JDBC.
Web Technologies in Java
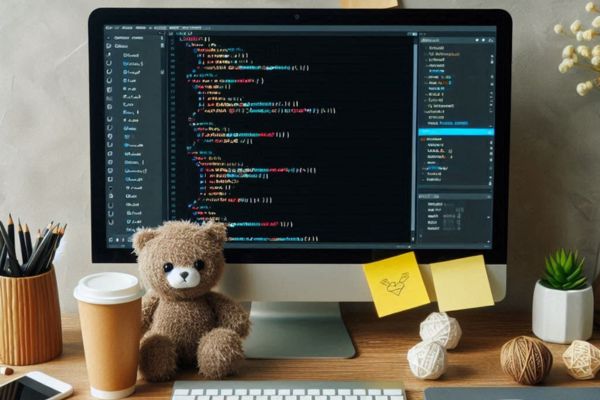
Servlets
Servlets are Java programs that run on a web server, handling client requests and generating dynamic web content.
JSP (JavaServer Pages)
JSP is a technology that allows software developers to create dynamically generated web pages using HTML, XML, or other document types.
Java Web Frameworks
Popular Java web frameworks include Spring MVC, JSF (JavaServer Faces), and Struts, which help in building web applications efficiently.
Best Practices in Java Programming
Code Quality
Maintain high code quality by following coding standards, writing clean and readable code, and conducting code reviews.
Testing
Implement thorough testing, including unit tests, integration tests, and system tests, to ensure your code works correctly.
Performance Optimization
Optimize performance by profiling your code, identifying bottlenecks, and using efficient algorithms and data structures.
Common Mistakes in Java Programming
Pitfalls to Avoid
Avoid common mistakes such as neglecting exception handling, writing inefficient code, and ignoring code readability.
Debugging Tips
Use debugging tools provided by your IDE, add meaningful log statements, and systematically isolate and fix issues.
Mock Interview Tips
How to Prepare for a Java Interview
Review core Java concepts, practice coding problems, and familiarize yourself with the company’s tech stack and interview format.
Tips for Cracking the Interview
Stay calm, explain your thought process, write clean code, and don’t be afraid to ask clarifying questions.
Sample Interview Questions
- What is the difference between abstract classes and interfaces?
- Explain the significance of the ‘this’ keyword in Java.
- How does garbage collection work in Java?
Conclusion
Preparing for a Java interview can seem daunting, but with the right approach and thorough preparation, you can excel. Focus on understanding core concepts, practicing coding problems, and familiarizing yourself with advanced topics and frameworks. Good luck!
FAQs
What is the difference between JDK, JRE, and JVM?
The JDK (Java Development Kit) includes tools for developing Java applications, the JRE (Java Runtime Environment) provides the libraries and JVM required to run Java applications, and the JVM (Java Virtual Machine) executes Java bytecode on any machine.
How is Java platform-independent?
Java is platform-independent because it uses the JVM to convert bytecode into machine-specific code, allowing the same Java program to run on different platforms without modification.
What is the role of the main method in Java?
The main method is the entry point of any Java application. It is the method that gets called when a Java program starts.