Introduction
In the world of computer programming, efficient data input is essential for creating interactive applications. Java, being one of the most popular programming languages, offers various ways to handle user input from keyboards. This article will delve into the different methods and techniques in Java for handling keyboard input. Whether you are a beginner or an experienced developer, understanding these concepts is crucial to creating robust and user-friendly applications.
1. Basic Keyboard Input
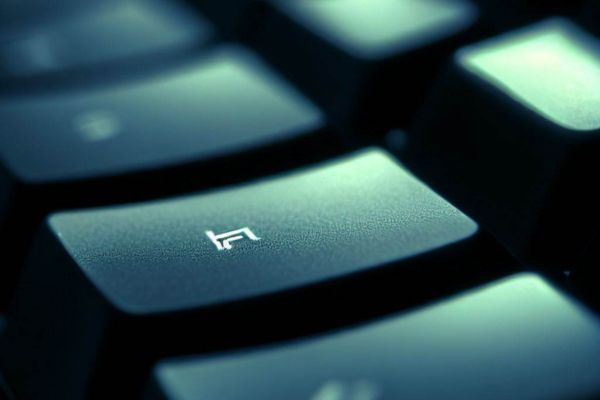
To get started with keyboard input in Java, you need to use the java.util.Scanner
class. This class provides convenient methods to read different types of data from the keyboard, such as integers, floats, and strings. The following code snippet demonstrates how to read a line of text from the user:
import java.util.Scanner;
public class KeyboardInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "! Nice to meet you.");
}
}
2. Reading Numeric Input
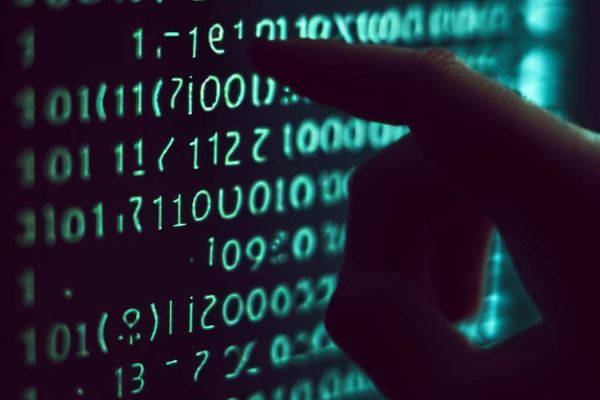
Java allows you to read numeric input from the keyboard using various methods of the Scanner
class. For instance, to read an integer, you can use nextInt()
:
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = scanner.nextInt();
System.out.println("You are " + age + " years old.");
Similarly, you can use nextFloat()
to read floating-point numbers.
3. Input Validation
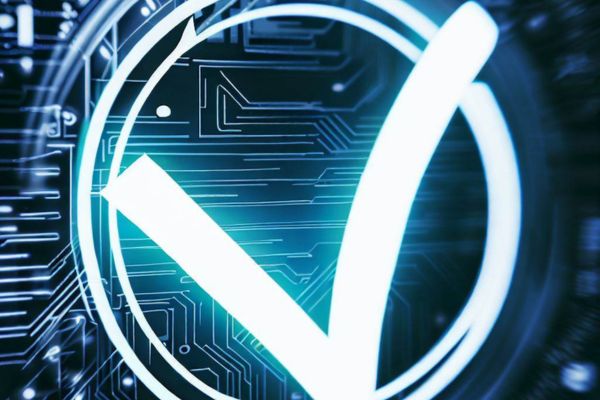
When dealing with user input, it’s crucial to validate the data to ensure it matches the expected format. Java provides various techniques to handle input validation. One common approach is using loops to prompt the user until valid input is provided:
Scanner scanner = new Scanner(System.in);
int age = 0;
while (age <= 0) {
System.out.print("Enter your age (must be a positive number): ");
age = scanner.nextInt();
}
System.out.println("Valid age: " + age);
4. Handling Keyboard Events
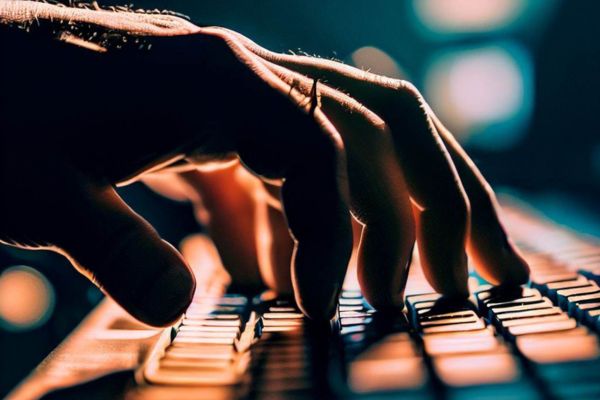
Apart from console-based input, Java allows you to handle keyboard events in graphical applications. Swing, the standard Java GUI library, provides functionality to handle key events. You can use the KeyListener
interface to capture and respond to keyboard events:
import javax.swing.*;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class KeyboardEventExample extends JFrame implements KeyListener {
public KeyboardEventExample() {
addKeyListener(this);
setFocusable(true);
setFocusTraversalKeysEnabled(false);
}
@Override
public void keyTyped(KeyEvent e) {
// Code to handle key-typed events
}
@Override
public void keyPressed(KeyEvent e) {
// Code to handle key-pressed events
}
@Override
public void keyReleased(KeyEvent e) {
// Code to handle key-released events
}
}
5. Dealing with Special Keys
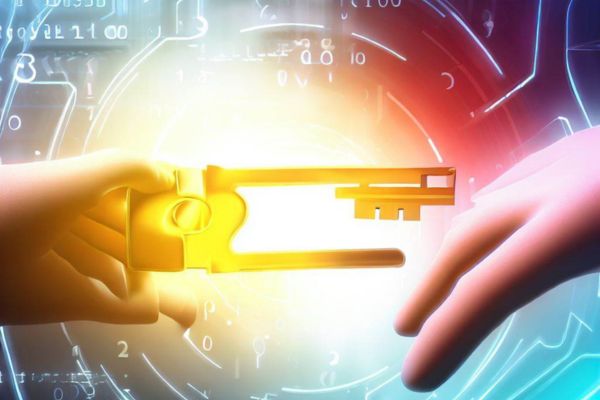
In Java, special keys such as arrow keys, function keys, and modifier keys can be handled using their corresponding key codes. The KeyEvent
class defines constants for these key codes. For instance, to detect the Enter key press, you can use KeyEvent.VK_ENTER
:
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_ENTER) {
// Code to handle Enter key press
}
}
6. Advanced Input Handling
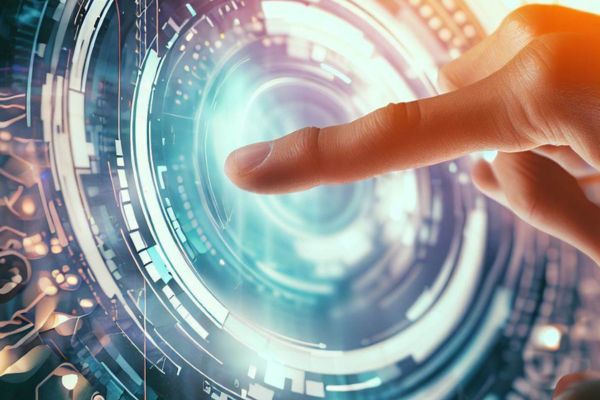
For more complex input scenarios, Java provides additional libraries and APIs. For instance, if you need to implement input with real-time feedback, you can explore using the java.awt.Robot
class to simulate keypresses programmatically. Additionally, the java.awt.event.KeyEvent
class offers more extensive functionality to handle various keyboard-related events.
Conclusion
In conclusion, understanding how to handle input from keyboards is essential in Java programming. The Scanner
class enables you to read user input effortlessly, while Swing and KeyListener
help you capture keyboard events in graphical applications. By incorporating input validation, you can ensure the reliability of your applications and enhance the user experience.
FAQs
1. How do I clear the keyboard buffer in Java?
To clear the keyboard buffer in Java, you can use the Scanner
class’s nextLine()
method after reading the desired input. This will consume any remaining input in the buffer.
2. Can I detect multiple keypresses simultaneously?
Yes, Java allows you to detect multiple keypresses simultaneously using a combination of key codes and event handling. You can track the state of different keys and implement custom logic accordingly.
3. What is the difference between keyPressed()
and keyTyped()
methods?
The keyPressed()
method is called when a key is physically pressed down, while the keyTyped()
method is called when the Unicode character represented by the key is output.
4. How can I handle keyboard input in Android app development?
In Android app development, you can use the EditText
widget to capture user input from the on-screen keyboard. You can then process this input using Java’s regular input handling techniques.
5. Are there any alternative libraries for handling keyboard input in Java?
Yes, besides the standard Java libraries, you can explore third-party libraries like JInput and LWJGL that provide more advanced input handling capabilities for games and multimedia applications.