Java’s ‘static final’ keyword plays a pivotal role in establishing the foundation of many class designs, serving as a bedrock for the creation of constants and the assurance of immutable data structures. It is a beacon of stability in a mutable world, imbuing class variables with essential properties that govern their behavior and access. Through this essay, we will dissect the semantics of ‘static final’, unraveling its conceptual depth and the syntactical governance it imposes on Java class members. Our journey will traverse through the landscapes of static membership and finality, exploring their collaborative effect in crafting secure, thread-safe code that stands resilient against the unpredictable flow of data modification.
The Semantics of Static Final in Java
Unveiling the Significance of ‘static final’ in Java
In the intricate tapestry of Java programming, the ‘static final’ combination plays a pivotal role in crafting efficient and secure code. A foundation of understanding in object-oriented languages, like Java, prompts one to appreciate the ramifications of this combination on the very fabric of software development.
The term ‘static’ in Java parlance refers to a class level variable or method. Distinguished from instance variables that necessitate an object for access, static elements belong to the class itself, not to any particular instance. This implies that a single copy of a static variable is shared across all instances of the class, conserving memory and ensuring consistency of the value.
The other term, ‘final’, imbues an immutable character to the variables. When a variable is declared as final, its value, once assigned, is etched in stone, preventing further modification. This is not just a syntactical decree; it reflects the commitment of the variable to remain unchanged throughout its lifecycle. Hence, a final variable can be safely read without the synchronization that is typically required in a multithreaded context, thus avoiding potential concurrency issues.
When combined, ‘static final’ attributes to a variable both class-wide reach and immutability. Such variables are often used to define constants that represent fixed values, such as: public static final double PI = 3.14159;
. Here, PI is a constant that holds a value which is a fundamental aspect of the circle across any instance or usage within the program.
The immutable nature of ‘static final’ variables is not merely a matter of maintaining constancy; it also influences the performance of an application. Since these variables are resolved at compile-time, the Java compiler can inline the values where they are used, leading to an optimized set of bytecode instructions that are devoid of unnecessary overhead.
Moreover, the static final pattern finds particular utility in defining configuration or convention that centralizes control. If any such values need changing, it can be adjusted in a singular location, reducing the potential for errors that could arise from updating multiple spots throughout the application.
Conclusively, ‘static final’ is not an arcane combination of keywords but a profound construct that embodies principles of efficiency, security, and maintainability in Java programming. Understanding its application is crucial to developing robust code that stands the test of usage and time.
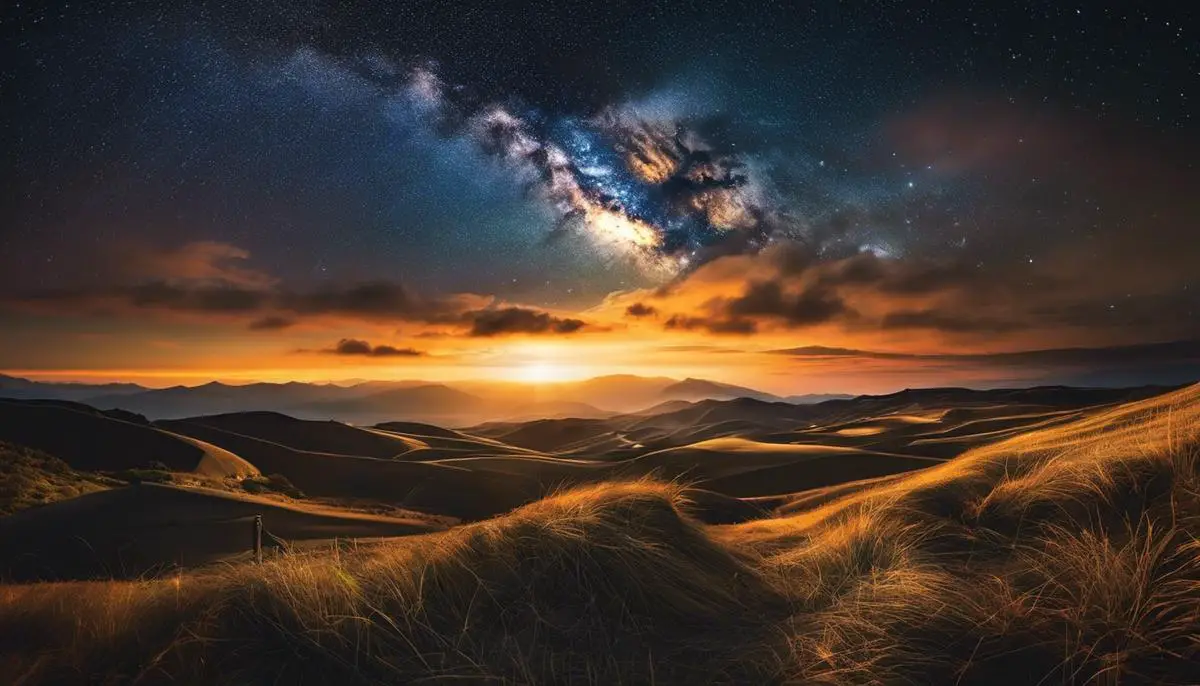
Practical Applications of Static Final
In the realm of Java programming, the utilization of ‘static final’ variables extends beyond fundamental principles, permeating diverse areas of software development. These variables, often referred to as constants, are not merely placeholders for fixed values; they serve critical functions in real-world applications, shaping the robustness and clarity of codebases.
Let us delve into the practical scenarios that evidentially underscore the utility of ‘static final’ variables in Java. A prime example exists within the world of application development where the ‘static final’ paradigm is pivotal in the construal of shared configuration properties. Software of moderate to substantial complexity frequently necessitates a myriad of configuration settings that, while constant throughout execution, are universally accessible across various components. ‘Static final’ variables admirably address these requirements by providing a single source of truth, mitigating the potential for error stemming from duplicated literals throughout the codebase.
public class AppConfig {
// Example of using static final for configuration settings
public static final String DATABASE_URL = "jdbc:mysql://localhost:3306/mydatabase";
public static final String FILE_PATH = "/path/to/files";
}
In addition to configuration settings, ‘static final’ variables play an instrumental role in defining natural constants and standard values, which are immutable by their intrinsic nature. For instance, mathematical constants such as π (pi) or scientific constants like the speed of light are universally recognized, unvarying quantities that are embedded in a multitude of calculations. Representing these constants with ‘static final’ variables ensures they are accurately conveyed and persistently reliable within the application, safeguarding the integrity of the computations they influence.
public class MathConstants {
// Example of using static final for mathematical constants
public static final double PI = 3.14159;
public static final double SPEED_OF_LIGHT = 299792458; // in meters per second
}
Furthermore, in the development of APIs and libraries, ‘static final’ variables are paramount in denoting versioning information. It is customary for shared libraries to entrench their version number within a ‘static final’ string, affording consumers of the API facile access to this critical data. By leveraging this convention, software systems can programmatically react to different library versions, thereby enhancing compatibility and providing finer-grained control over dependencies.
Another noteworthy application of ‘static final’ variables is in the software pattern known as Enums (enumerations), wherein a set of predefined values gains definition. Intended to represent a specific set of possible values (such as days of the week or directions), Enums utilize ‘static final’ variables to internalize these designated values. Their enforcement of type safety regarding these fixed sets precludes the admittance of invalid or unintended data, an assurance that bolsters the robustness of the code.
Moreover, ‘static final’ variables find extensive use in defining error messages and codes within a system. Standardized error handling is indispensable to modern software, and the clear articulation of such messages and codes through constants is vital for consistent interpretation. Subsequently, maintenance and debugging processes are streamlined, contributing to the overall maintainability of the application.
In conclusion, the implementation of ‘static final’ variables is integral to the architecture of enduring, effective Java software. By serving as the foundation for configurational parameters, natural and scientific constants, API version details, predefined value sets in Enums, and standardized error messages, these constants are far more than passive, immutable data. They embody the cardinality of consistent, reliable, and communicable elements across sprawling codebases, championing a methodology that emphasizes integrity, durability, and clarity in Java programming endeavors.
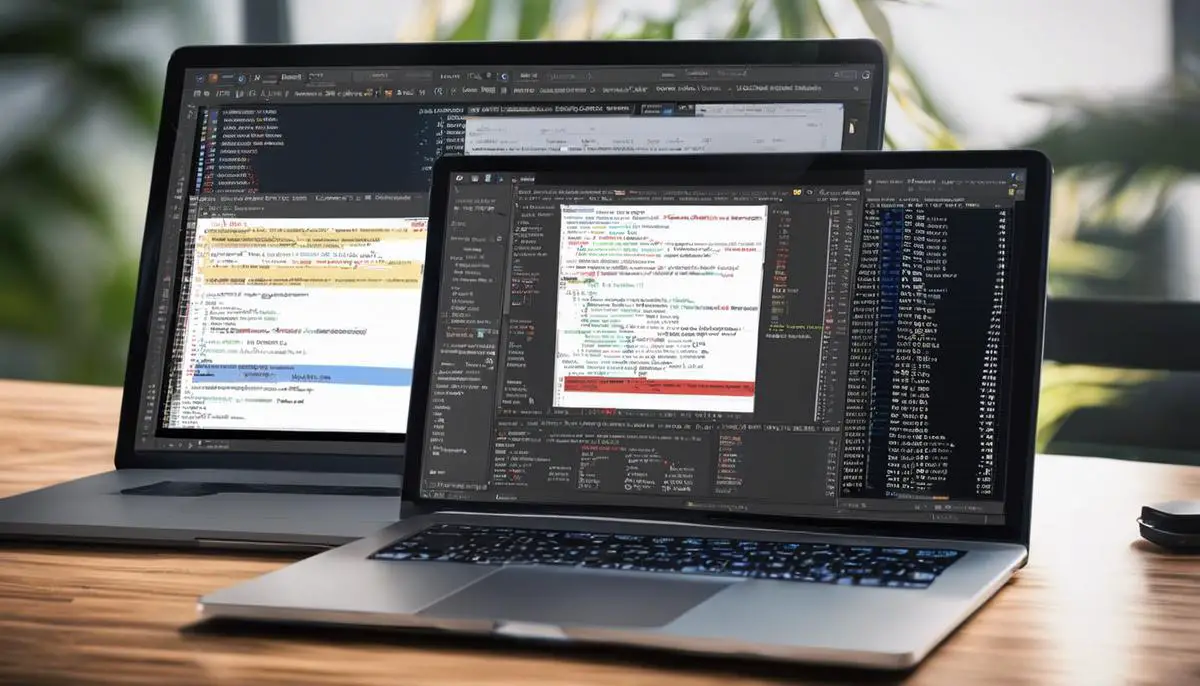
Comparative Analysis with Other Modifiers
Distinct from individual instance fields which maintain separate and unique states across a multiplicity of objects, ‘static final’ fields exemplify the essences of both invariability and singularity at the class level. An instance field, despite its utility, undergoes instantiation with each object; it embodies a mutable state subjective to each entity. The prevalence of ‘static’ modality provides a solution to the void of a single source of truth accessible across all instances, mitigating the undue instantiation of duplicates.
When referring to configurational settings, if the landscape demands an immutable yet universally accessible value, ‘static final’ emerges as the sine qua non. Consider database connection strings or file paths—the usage of ‘static final’ not only uniformly distributes this information to all classes and objects but also shelters it from inadvertent alteration. Such stability becomes a linchpin for applications where consistent reference is paramount.
Furthermore, the lexicon of natural and scientific enumerations, constants such as Planck’s constant (h), or the mathematical π (pi) are resilient to change — their values transcendent across time and space. Embedding such constants within a ‘static final’ verse serves a testament to their steadfastness and proffers an accessible, unalterable reference point for any operation demanding precision and consistency.
In the realm of Application Programming Interfaces, or APIs, where contract adherence is critical, API versioning underlines another servant of ‘static final’ usage. Establishing and clearly stating the API version through ‘static final’ fields enforces conformity and clarity amidst the evolution of software services, ensuring coherency and backward compatibility.
Enumerations, or Enums, exploit the ‘static final’ paradigm to impose a systematic and type-safe means of defining sets of constants. In these cases, the ‘static’ infuses these sets with global reach, whilst ‘final’ instills immutability, collectively guaranteeing dependability and integrity to these finite lists of permissible values.
Error codes and messages—vital cogs in the diagnostic machinery—gain succinctness and security through ‘static final’ decree. Their role surpasses mere information conveyance to enact as a cornerstone of effective debugging and user communication, where any slip in consistency can unravel into a maelic mire.
In summary, ‘static final’ variables plant their flags in the landscape of Java programming; they form the bedrock upon which robustness and maintenance rest. Through their rightful application, Java software turns into a fortress with battlements of constancy and a universally accessible armory, fostering an environment where scalability, security, and reliability burgeon.
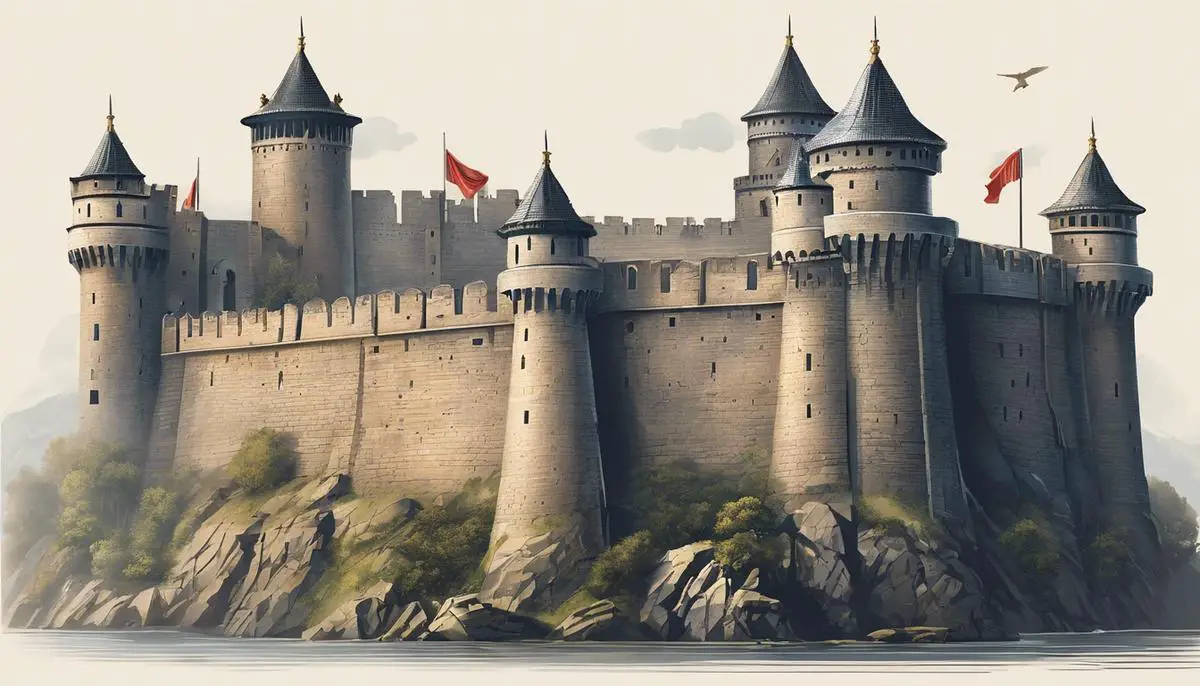
Best Practices and Design Considerations
Upon delving into the subtleties of the ‘static final’ keyword in Java, we must highlight additional nuances that drive the development of cohesive and high-quality software systems. The proper employment of ‘static final’ extends to several critical areas beyond defining constants and shared configuration parameters, which warrants a deeper examination.
Best practices suggest that ‘static final’ fields should encapsulate intrinsic qualities that are inherently bound to the class they reside in. A quintessential example is the mathematical constant π (pi) in a geometry library. Such use of ‘static final’ underscores the universal truth encapsulated by these variables, relevant to all instances of the class, and indeed, beyond it.
For the conservation of resources, especially memory allocation, when multiple objects of a class are instantiated, ‘static final’ variables ensure that a single copy exists, reducing redundancy. This principle underscores not only an efficient use of resources but also mitigates potential discrepancies, as multiple instances of the variable cannot exist with divergent values.
Further, adherence to naming conventions for ‘static final’ variables is paramount. Convention dictates all-uppercase letters with underscores to separate the words, aiding in the immediate recognition of such constants within the codebase, fostering readability and understandability among developers.
One must exercise prudence in initializing ‘static final’ variables. Since they are assigned once and are bound for the lifetime of the application, initialization must occur either inline or within a static block. Failure to correctly initialize a ‘static final’ variable can result in compilation errors that prevent the application from running.
In encapsulating values that should not change once assigned, ‘static final’ fields contribute to the security of an application. Through prohibiting the alteration of these fields post-initialization, the integrity of the values is maintained, which is especially crucial for security-sensitive applications where, for instance, cryptographic key values are stored.
Furthermore, documentation is an indispensable complement to the use of ‘static final’ fields. Clear and comprehensive commentary on the purpose and intended use of each constant enhances its utility and safeguards against cases of misuse or misunderstanding, thereby preserving the robustness of the code.
It is a given that ‘static final’ variables should, by their immutable nature, be considered only when the value they encapsulate is a definitive invariant of the class—unchanging across all possible states and configurations.
Conclusively, adherence to established coding principles when using ‘static final’ variables streamlines the development process, reduces the likelihood of errors, bolsters performance, and enhances the modularity and readability of Java codebases. These variables act as a precision tool within the symbiotic tapestry of object-oriented programming—a tool that, when applied with judicious insight and well-defined intent, significantly elevates the caliber of Java applications.
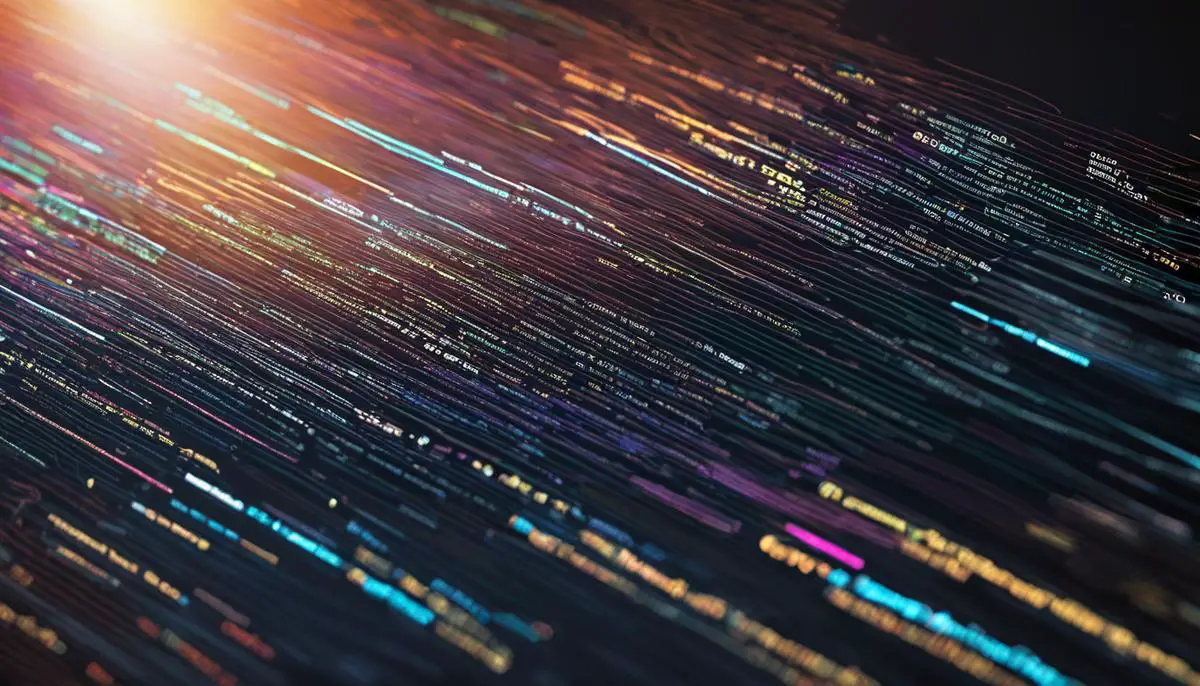
The journey through the world of ‘static final’ unearths the crucial role it plays in the orchestration of Java applications. It equips developers with a toolkit for building immutable references, enabling a synergy between memory efficiency and code integrity. Arming oneself with the knowledge of how ‘static final’ operates, juxtaposed with a strategic approach grounded in best practices and design principles, one can harness its full potential. It is the conscientious application of these principles that will elevate our practices from mere coding to the artistry of software craftsmanship, ensuring that the immutable constants we define stand the test of time, as enduring pillars within the fluid and evolving world of Java development.