In the world of programming, data structures play an important role in managing and manipulating data effectively. One such dynamic and widely used data structure in Java is the ArrayList. This article explores the intricacies of Java ArrayList, from its fundamentals to its practical applications.
What is an ArrayList in Java?
An ArrayList is a part of the Java Collections Framework, which extends the capabilities of arrays. Unlike arrays, ArrayLists can dynamically grow in size, making them highly flexible. They belong to the java.util
package and provide numerous methods for data manipulation.
Benefits of Using ArrayList
ArrayLists offer several advantages:
- Dynamic Sizing: They can expand or shrink as needed.
- Versatility: Storing objects of any type is possible.
- Easy Retrieval: Elements can be accessed using their index.
- Powerful Methods: Methods like
add
,remove
, andsort
simplify operations.
Declaring and Initializing an ArrayList
To create an ArrayList, you need to declare it and then initialize it. Here’s a basic example:
import java.util.ArrayList;
ArrayList<String> names = new ArrayList<>();
This creates an ArrayList named names
to store strings.
Adding Elements to an ArrayList
You can add elements to an ArrayList using the add
method. For instance:
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Removing Elements from an ArrayList
Removing elements is as straightforward as adding them. The remove
method helps with that.
names.remove("Bob");
Accessing Elements in an ArrayList
To retrieve elements, you can use their index.
String firstPerson = names.get(0); // Retrieves "Alice"
Exploring the Dynamics of ArrayList Size and Capacity in Java
When working with an ArrayList in Java, it's essential to understand the distinction between its size and capacity. The size corresponds to the current count of elements that the ArrayList holds. Contrarily, the capacity refers to the maximum number of elements that the ArrayList can contain without need for resizing.
Now, let's delve into what 'resizing' actually means. When we add more elements to the ArrayList and exceed its current capacity, the ArrayList automatically increases its capacity. This process is known as 'resizing'. Resizing can affect the memory footprint of your Java program, as an ArrayList with a larger capacity will naturally consume more memory.
To illustrate this concept, suppose we have an ArrayList with a current capacity of 10. If we try to add an 11th element, the ArrayList will automatically resize itself to accommodate the new addition. This operation is different from other processes such as 'sorting' or 'filtering' where the size or arrangement of elements are modified, but the capacity remains the same.
Understanding the difference between the 'size' and 'capacity' of an ArrayList, and mastering the workings of 'resizing', are key to optimizing your Java programs. It's this fine distinction that can lead to more efficient use of memory resources. Hence, make sure to keep this in mind when you're dealing with ArrayLists in Java.
Iterating Through an ArrayList
Iterating through an ArrayList is essential for various operations. The enhanced for-loop simplifies this task.
for (String name : names) {
System.out.println(name);
}
Sorting an ArrayList
To sort the elements in an ArrayList, you can use the Collections.sort
method.
import java.util.Collections;
Collections.sort(names);
Common Operations with ArrayList
Other common operations include checking if an ArrayList is empty, clearing all elements, determining its size, and ensuring it is imported into the code.
boolean isEmpty = names.isEmpty();
names.clear();
int listSize = names.size();
ArrayList vs. Arrays
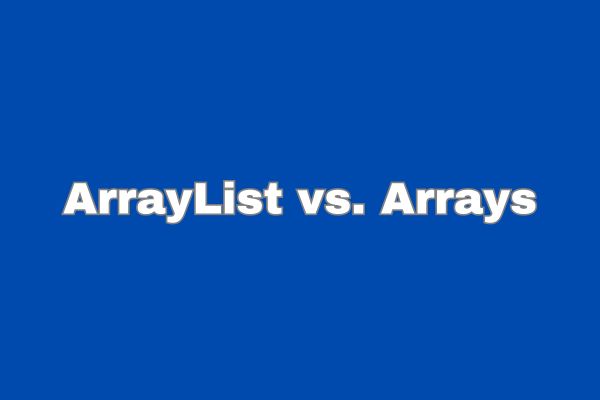
When it comes to programming, one of the most significant factors to keep in mind is data structure, and among the most commonly used ones are arrays and ArrayLists. Arrays are undoubtedly essential and provide a straightforward approach to storing similar types of data, but their utilization may be inadequate in certain situations, hence the introduction of ArrayLists.
ArrayLists are a more advanced data structure that offer unravelled flexibility and capabilities beyond the traditional arrays. Their benefits are diverse, including speed optimization, enhancement of code readability, and robust functionality that makes handling data a breeze.
For instance, one main advantage of ArrayLists is their dynamic quality. Array needs to know its size at the initialization stage, while ArrayLists are perceived as more flexible where their size can be varied – you can increase or decrease the size as you wish. Likewise, adding, removing or locating an item from ArrayList is more efficient than performing the same operations in arrays.
That alteration capability, however, comes with a minor setback relative to the memory usage: ArrayLists consume more memory compared to arrays. To better understand this, envision having an ArrayList filled with 5 data items. In an ArrayList, the overhead per element is typically larger than in an array, because each element in an ArrayList is an object that requires additional memory beyond the data it holds. As a result, if we consider storing 5 integers, an ArrayList could consume almost twice as much memory as an array would. Although ArrayLists are an efficient choice in multiple scenarios, considering the memory usage aspects could lead us to prefer traditional arrays in memory-constrained applications.
Now, how do you declare and initialize an ArrayList? Here's a basic example:
ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple");fruits.add("Banana");
In the code mentioned above, we're creating an ArrayList named 'fruits'. To add elements to it (like "Apple" and "Banana"), we used the 'add' method.
All in all, understanding when to use an ArrayList over a traditional array is key to efficient and effective programming. It relies on a number of factors, primarily on the specific needs of your program, such as the importance of memory usage, speed, ease of use, and flexibility. More often though, the dynamic nature of ArrayLists makes it more favorable to programmers.
Best Practices for Using ArrayList
To make the most of ArrayLists, follow these best practices:
- Specify the data type.
- Use the diamond operator for type inference.
- Consider capacity when adding elements in bulk.
- Use enhanced for-loops for iteration.
Understanding and Utilizing Java ArrayLists
Here, we delve into the effective use of Java's ArrayList. Simply put, ArrayList is a dynamic array-like data structure in the Java Collection Framework. However, as we continue, we will use uncomplicated language, doing our best to avoid complex computer science jargon.
Let's initially understand, declare, and initialize an ArrayList. Using the methods ‘add’, ‘remove’, ‘get’, and ‘set’, we add elements, eliminate them, retrieve them, and alter them, respectively. While it seems straightforward, let’s illustrate these operations with a couple of practical examples for better comprehension.
Imagine you’re a school teacher, you can use an ArrayList to manage your students' grades. Initially, you declare an ArrayList and then add grades as they are scored. In case a grade has to be updated, you can change it directly. Now, what if a student leaves or a new one joins? The 'remove' or 'add' method can be manipulated. Additionally, using 'get', you can retrieve any stored grade. This simple, flexible, and dynamic storage system is the QColorArrayList.
To further clarify, let's compare an ArrayList to its equivalents, LinkedList and Vector in the Java Collection Framework. You'll notice similar functionalities but different implementation ways, focusing on how they are best used effectively.
Expanding on ArrayList usage, consider a warehouse inventory management scenario. The elements of the ArrayList could be the items in the warehouse. Add new items with 'add', remove sold ones with 'remove', or update item details with 'set'. The potential uses of ArrayLists are numerous, demonstrating its significant because of its flexibility.
However, programmers need to be wary of common pitfalls when using ArrayLists. For instance, working with threads or understanding how ArrayLists' dynamism affects performance. We will investigate these issues to help you avoid complications.
Our goal is not to overwhelm you with advanced topics, but to give you insight into more intricate concerns as and when you're prepared. ArrayList, just one tool in the Java Collection Framework, offers significant benefits, especially when used carefully and correctly.
ArrayLists offer a flexible tool in Java programming, particularly when dealing with dynamic data structures like stacks or queues. They provide the advantage of storing data without the need to predefine an array size, which makes it an essential part of Java programming. To illustrate, one might use ArrayLists in implementing a dynamic stack, a common data structure in computer science that operates on a "Last In, First Out" principle. For another practical example, ArrayLists can be used in creating a queue system in a Java application, wherein elements are added and removed from one end. By incorporating these specific examples, the function and applicability of ArrayLists in Java programming becomes much clearer.
Conclusion
In the world of Java programming, the ArrayList is a valuable tool that simplifies data management. With dynamic sizing, powerful methods, and extensive use cases, it’s a data structure that every Java developer should be well-versed in.
FAQs
- What is the difference between ArrayList and arrays in Java?
ArrayLists are dynamic, can store objects of any type, and provide extensive methods for data manipulation, whereas arrays have a fixed size and are limited in functionality. - How do I add elements to an ArrayList?
You can use theadd
method to add elements to an ArrayList. - Can I sort an ArrayList in Java?
Yes, you can use theCollections.sort
method to sort the elements in an ArrayList. - What are some best practices for using ArrayList in Java?
It’s advisable to specify the data type, use the diamond operator, consider capacity when adding elements in bulk, and use enhanced for-loops for iteration. - What are some common use cases for ArrayList in Java?
ArrayLists are used in various scenarios, such as managing lists of user information, maintaining inventory items, and more.