Center aligning in HTML is a common task when it comes to web development. It is used to position text, images, and other elements in the center of the page or a container. Center aligning an element can be done in several ways, and it depends on the type of element and the layout of the web page.
We can do using <center> tag in html like this:-
<center>
hello world!
<p>hello world!</p>
</center>
HTML5 does not support this tag.
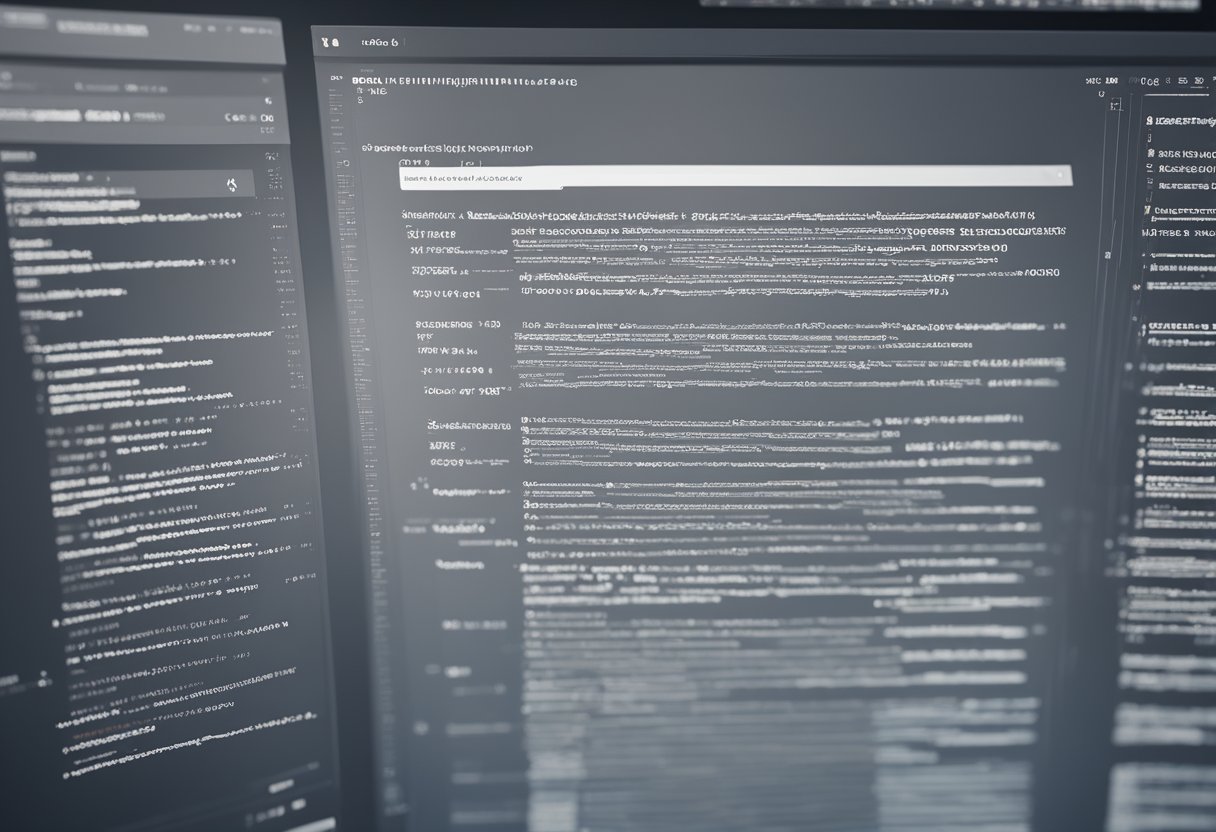
One of the most common ways to center align an element in HTML is by using the text-align
property. This property can be applied to the container element that holds the element you want to center align. For example, to center align a paragraph element, you can apply the text-align
property to the parent div element. Another way to center align elements is by using the margin
property. This property can be used to center align block-level elements by setting the left and right margins to auto
.
Understanding HTML Alignment

The Role of HTML in Web Design
HTML (Hypertext Markup Language) is the standard markup language used to create web pages. It is the foundation of every web page, and it is responsible for the structure and content of a web page. HTML provides a variety of tags that web developers can use to control the layout and presentation of a web page, including text alignment.
Historical Methods of Alignment
In the early days of the web, aligning text was a challenge. Web developers often used tables to align text, which was not an ideal solution. However, with the introduction of CSS (Cascading Style Sheets), web developers can now use CSS to align text more efficiently.
The text-align
property is used to align text in HTML. Text alignment can be set to left, right, center, or justified. The text-align
property can be applied to block-level elements, such as div
, h1
, p
, and table
.
The following table shows the different values that can be used with the text-align
property:
Value | Description |
---|---|
left | Aligns text to the left |
right | Aligns text to the right |
center | Centers text |
justify | Justifies text (creates even spacing) |
To center align in HTML, set the text-align
property to center
. For example, to center align a heading, use the following code:
<h1 style="text-align: center;">Center Aligned Heading</h1>
In conclusion, HTML provides a variety of tags and properties that web developers can use to control the layout and presentation of a web page. The text-align
property is used to align text in HTML, and it can be set to left, right, center, or justified. To center align in HTML, set the text-align
property to center
.
CSS for Center Alignment

Center alignment is a common practice in web design to give a neat and organized look to the website. There are different ways to center-align elements in HTML, but the most common method is by using CSS. In this section, we will discuss two methods of using CSS to center-align elements in HTML.
Inline CSS for Quick Tweaks
Inline CSS is a quick and easy way to center-align elements in HTML. It is useful when you need to make a quick tweak to the alignment of an element without affecting the rest of the page’s styling. To center-align an element using inline CSS, add the style attribute to the HTML tag and set the text-align property to center. For example:
<p style="text-align: center;">This paragraph is center-aligned.</p>
This method is useful when you need to make quick tweaks to the alignment of an element. However, it is not recommended for larger projects as it can make the code hard to maintain.
External CSS for Consistent Styling
External CSS is a better method for center-aligning elements in HTML when you need consistent styling across the website. To center-align an element using external CSS, create a CSS file and add the following code:
.center {
text-align: center;
}
Then, link the CSS file to the HTML document using the link tag:
<head>
<link rel="stylesheet" href="style.css">
</head>
Now, you can use the class name “center” in the HTML tag to center-align the element:
<p class="center">This paragraph is center-aligned.</p>
This method is more efficient and recommended for larger projects as it makes the code easier to maintain and update.
In conclusion, center alignment is an important aspect of web design that can be achieved using CSS. Inline CSS is useful for quick tweaks, while external CSS is recommended for larger projects. By using these methods, web designers can achieve consistent and organized alignment across their website.
Centering Elements in HTML

Centering elements in HTML can be achieved through various methods depending on the type of element. In this section, we will explore how to center text, block elements, inline elements, and images in HTML.
Centering Text Elements
To center text elements such as headings, paragraphs, and spans, you can use the CSS property text-align
. Set the property value to center
to horizontally center the text. For example:
<h1 style="text-align: center;">Centered Heading</h1>
<p style="text-align: center;">Centered Paragraph</p>
<span style="text-align: center;">Centered Span</span>
Centering Block Elements
Block elements such as divs, sections, and articles can be centered using the CSS margin
property. Set the left and right margins to auto
and set the width of the element. This will center the element horizontally. For example:
<div style="width: 50%; margin: 0 auto;">Centered Div</div>
<section style="width: 75%; margin: 0 auto;">Centered Section</section>
<article style="width: 90%; margin: 0 auto;">Centered Article</article>
Centering Inline Elements
Inline elements such as links, spans, and images can be centered by wrapping them in a block-level element and centering that element. For example:
<div style="text-align: center;">
<a href="#">Centered Link</a>
<span>Centered Span</span>
<img src="image.jpg" alt="Centered Image">
</div>
Centering Images
Images can be centered by setting the left and right margins to auto
and setting the display property to block
. This will center the image horizontally. For example:
<img src="image.jpg" alt="Centered Image" style="display: block; margin: 0 auto;">
In conclusion, centering elements in HTML can be achieved through various methods depending on the type of element. By using the appropriate CSS properties, you can easily center text, block elements, inline elements, and images in your HTML documents.
Advanced Centering Techniques
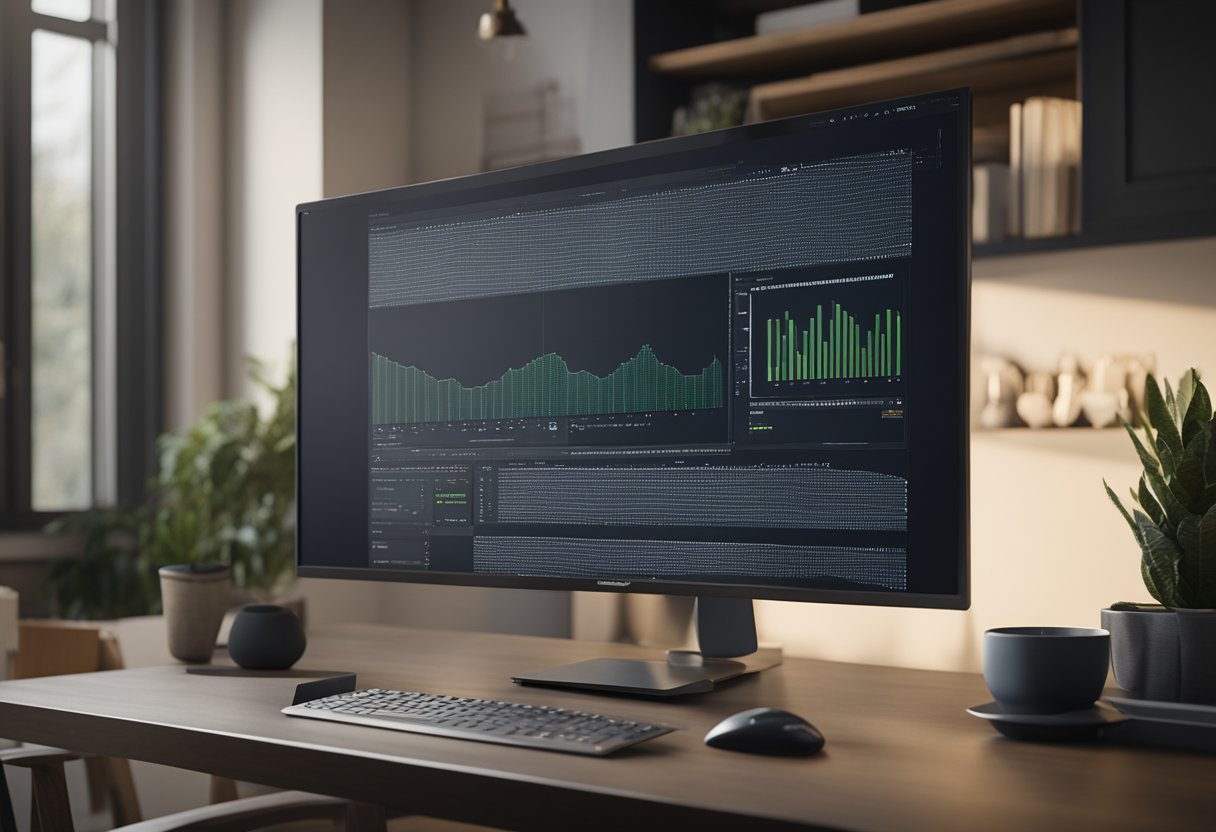
Sometimes the basic centering techniques in HTML are not enough to achieve the desired result. In such cases, advanced centering techniques can be used to achieve the desired result.
Flexbox for Responsive Design
Flexbox is a powerful layout tool that can be used to create responsive designs. It is a one-dimensional layout model that can be used to align items both horizontally and vertically. Flexbox is especially useful for creating responsive designs because it allows you to easily change the layout of your page based on the size of the screen.
To use Flexbox, you need to first define a container element and then add child elements to it. You can then use the justify-content
and align-items
properties to align the child elements both horizontally and vertically. For example, to center align a child element horizontally and vertically, you can use the following code:
.container {
display: flex;
justify-content: center;
align-items: center;
}
Grid Layout for Complex Structures
Grid Layout is a two-dimensional layout model that can be used to create complex structures. It allows you to create a grid of rows and columns and then place items within that grid. Grid Layout is especially useful for creating complex layouts that require a lot of precision.
To use Grid Layout, you need to first define a container element and then add child elements to it. You can then use the grid-template-columns
and grid-template-rows
properties to define the grid structure. You can then use the grid-column
and grid-row
properties to place items within the grid. For example, to center align a child element horizontally and vertically, you can use the following code:
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(3, 1fr);
}
.item {
grid-column: 2 / 3;
grid-row: 2 / 3;
}
By using advanced centering techniques like Flexbox and Grid Layout, you can create complex layouts that are both responsive and precise. These techniques can be used to achieve the desired result when the basic centering techniques in HTML are not enough.
What is the Difference Between CSS Grid and Flexbox?
Common Centering Challenges

Centering elements in HTML can be a challenge, especially when dealing with different browsers and screen sizes. Here are some common challenges and how to handle them.
Handling Different Browsers
Different browsers may interpret centering code differently, which can lead to inconsistencies in the appearance of centered elements. One way to handle this is to use browser-specific CSS code. For example, to center an element horizontally in Internet Explorer, use the following code:
.element {
display: flex;
justify-content: center;
}
To center an element vertically in Internet Explorer, use the following code:
.element {
display: flex;
align-items: center;
}
Adapting to Various Screen Sizes
Another challenge when centering elements is ensuring that they are centered regardless of the screen size. One way to handle this is to use media queries. For example, to center an element horizontally on screens smaller than 768 pixels, use the following code:
@media screen and (max-width: 768px) {
.element {
display: flex;
justify-content: center;
}
}
To center an element vertically on screens smaller than 768 pixels, use the following code:
@media screen and (max-width: 768px) {
.element {
display: flex;
align-items: center;
}
}
By using these techniques, you can ensure that your centered elements look consistent across different browsers and screen sizes.
More Ways To Center Content Using CSS
Best Practices for Center Alignment

When it comes to center alignment in HTML, there are some best practices that should be followed to ensure that the website is readable and accessible. Here are some of the best practices for center alignment in HTML:
Maintaining Readability
While center alignment can be visually appealing, it can also make the content harder to read if not used properly. Therefore, it is important to use center alignment sparingly and only when it is necessary. For example, center alignment can be used for headings, but not for body text. Additionally, it is important to ensure that there is enough white space around the centered content to make it stand out and easy to read.
Ensuring Accessibility
It is essential to ensure that the website is accessible to all users, including those with disabilities. When using center alignment, it is important to make sure that the content is still accessible to users who rely on assistive technologies such as screen readers. One way to do this is to use CSS to center the content instead of the HTML center tag, which is deprecated in HTML5 and can cause accessibility issues.
Another way to ensure accessibility is to use descriptive text for images and other visual elements that are centered. This will allow users who cannot see the image to understand its purpose and context. Additionally, it is important to use clear and concise language in the centered content to make it easy to understand for all users.
By following these best practices, developers can create visually appealing and accessible websites that are easy to read and navigate.
Tools and Resources

Editors and IDEs for HTML and CSS
When it comes to writing HTML and CSS, there are a variety of editors and integrated development environments (IDEs) available to choose from. Some popular options include:
- Visual Studio Code: a free, open-source code editor with a variety of extensions available for HTML and CSS development.
- Sublime Text: a lightweight code editor with a variety of features for HTML and CSS development.
- Atom: a free, open-source code editor with a variety of packages available for HTML and CSS development.
These editors and IDEs can help developers write cleaner, more organized code, as well as provide helpful features like syntax highlighting and auto-completion.
Online Communities and Support
In addition to tools for writing HTML and CSS, there are also a variety of online communities and support resources available for developers looking to learn how to center align text in HTML. Some popular options include:
- Stack Overflow: a question and answer site where developers can ask and answer questions related to HTML and CSS development.
- W3Schools: a website with a variety of tutorials and resources for learning HTML and CSS.
- MDN Web Docs: a website with a variety of documentation and resources for HTML and CSS development.
These online resources can be a valuable tool for developers looking to learn more about center aligning text in HTML, as well as for troubleshooting issues and getting help from other developers.
Frequently Asked Questions

What is the CSS property to horizontally center text within an element?
The CSS property used to horizontally center text within an element is text-align
. This property can be set to center
to center-align the text within the element. For example, to center-align the text within a div
element, you can add the following CSS rule:
div {
text-align: center;
}
How can I center an image within a div using HTML?
To center an image within a div
element using HTML, you can add the align
attribute to the img
tag and set it to middle
. For example:
<div>
<img src="path/to/image.jpg" align="middle">
</div>
Alternatively, you can use CSS to center the image within the div
element:
div {
display: flex;
justify-content: center;
align-items: center;
}
What methods are available to vertically align content in CSS?
There are several methods available to vertically align content in CSS, including:
- Using
vertical-align
property: This property can be used to vertically align inline or table-cell elements. However, it does not work with block-level elements. - Using
line-height
property: This property can be used to vertically center text within an element. - Using
display: flex
: This property can be used to vertically center content within a container element.
How do I horizontally center a block-level element like a div?
To horizontally center a block-level element like a div
, you can use the margin
property and set its value to auto
. For example:
div {
width: 50%;
margin: 0 auto;
}
This will center the div
element horizontally within its parent container.
What is the proper way to center a form on a webpage using HTML and CSS?
To center a form on a webpage using HTML and CSS, you can wrap the form in a div
element and use the text-align
property to center-align the div
. For example:
<div style="text-align: center;">
<form>
<!-- Form fields go here -->
</form>
</div>
Alternatively, you can use CSS to center the form within the div
element:
div {
display: flex;
justify-content: center;
align-items: center;
}
form {
width: 50%;
}
Which CSS property can be used to center headings in an HTML document?
The CSS property used to center headings in an HTML document is text-align
. You can set the text-align
property to center
to center-align the heading. For example:
h1 {
text-align: center;
}
This will center-align the h1
heading within its parent container.