PowerShell Parsing HTML:- Are you a tech enthusiast, programmer, or IT professional looking to extract valuable information from websites? PowerShell, a versatile scripting language developed by Microsoft, can be your ultimate solution. In this article, we’ll dive into the world of PowerShell and explore how it can simplify the process of parsing HTML, making web data extraction a breeze.
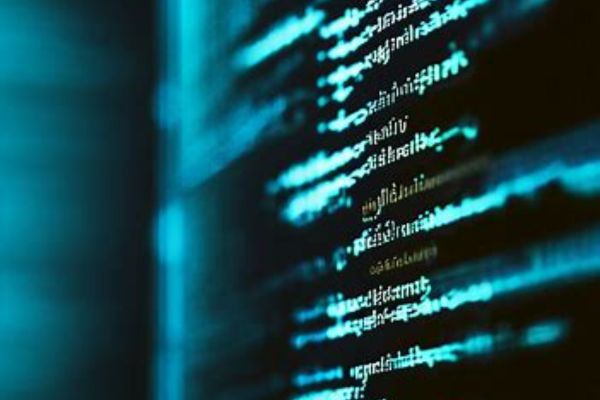
1. Introduction to PowerShell and HTML Parsing
PowerShell, primarily known for automating tasks on Windows systems, can also be an invaluable tool for web data extraction. HTML parsing is the process of extracting specific information from web pages’ HTML code, enabling you to gather data for analysis, reporting, or integration into other applications.
2. Advantages of Using PowerShell for HTML Parsing
PowerShell offers several advantages when it comes to HTML parsing. Firstly, it’s native to Windows, meaning you don’t need to install any third-party libraries. Secondly, it integrates seamlessly with other Microsoft technologies, making it a natural choice for Windows-focused projects. Lastly, PowerShell’s scripting capabilities provide the flexibility required to handle varying HTML structures across different websites.
3. Setting Up Your PowerShell Environment
Before diving into HTML parsing, ensure you have PowerShell installed on your system. Open a PowerShell console or terminal to start executing scripts. You can check your PowerShell version by typing Get-Host | Select-Object Version
.
4. Understanding HTML Structure
To effectively parse HTML, it’s crucial to understand the structure of the web page. HTML is composed of elements, each enclosed in tags. These tags provide information about the content’s meaning and how it should be displayed.
5. Using PowerShell’s Invoke-WebRequest Cmdlet
PowerShell’s Invoke-WebRequest
cmdlet is your gateway to fetching web content. It sends an HTTP request to a specified URL and retrieves the HTML content. You can then access and manipulate this content using various techniques.
# Fetch HTML content from a website
$url = "https://example.com"
$response = Invoke-WebRequest -Uri $url
# Access the HTML content
$htmlContent = $response.Content
6. Extracting Data with CSS Selectors
CSS selectors are powerful tools for targeting specific HTML elements. With PowerShell, you can leverage CSS selectors to extract the exact data you need. For instance, (.class)
selects elements by class, while #(id)
selects elements by ID.
# Extract data using CSS selectors
$title = $htmlContent | Select-String -Pattern '<h1 class="title">(.+)</h1>' |
ForEach-Object { $_.Matches.Groups[1].Value }
7. Navigating HTML Elements
HTML documents have a hierarchical structure, and PowerShell lets you navigate through this hierarchy effortlessly. By understanding parent-child relationships, you can accurately locate and extract your desired information.
# Traverse the HTML hierarchy
$element = $response.ParsedHtml.getElementById("myElement")
8. Handling Dynamic Web Pages
Some websites load content dynamically through JavaScript. PowerShell can handle such scenarios by simulating a browser’s behavior. Wait for a moment after loading a page to ensure its dynamic content has rendered.
# Simulate browser behavior for dynamic pages
$ie = New-Object -ComObject "InternetExplorer.Application"
$ie.Navigate($url)
# Wait for the page to load
while ($ie.Busy -eq $true) {
Start-Sleep -Milliseconds 100
}
9. Dealing with Forms and User Input
Interacting with forms on web pages requires sending POST or GET requests. PowerShell allows you to mimic user input by sending data to forms and retrieving the response, making it possible to automate tasks involving form submissions.
# Fill out and submit a form
$form = $response.Forms[0]
$form.Fields["username"] = "myUsername"
$form.Fields["password"] = "myPassword"
$response = $form.Submit()
10. Error Handling for Robust Scripts
Robust scripts handle errors gracefully. PowerShell enables you to implement error-handling mechanisms, such as try
and catch
, to ensure your script doesn’t break unexpectedly.
# Implement error handling
try {
# Your code here
}
catch {
Write-Host "An error occurred: $_"
}
11. Automating Web Data Extraction
With PowerShell, you can automate the entire web data extraction process. Schedule your scripts to run at specific intervals, ensuring you always have the latest data at your fingertips.
# Schedule script to run daily
$trigger = New-ScheduledTaskTrigger -Daily -At 3am
$action = New-ScheduledTaskAction -Execute "powershell.exe" -Argument "C:\Path\to\your\script.ps1"
Register-ScheduledTask -Trigger $trigger -Action $action -TaskName "WebDataExtraction"
12. Combining PowerShell with Other Tools
PowerShell’s versatility shines when combined with other tools. Integrate it with data analysis tools, databases, or reporting systems to create comprehensive solutions.
# Integrate with Excel for data analysis
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open("C:\Path\to\your\workbook.xlsx")
$worksheet = $workbook.Sheets.Item(1)
$worksheet.Cells.Item(1, 1).Value = "Data extracted from web"
$workbook.Save()
$excel.Quit()
13. Best Practices for Efficient HTML Parsing
- Target specific elements rather than parsing the entire page.
- Regularly update your parsing scripts to accommodate website changes.
- Keep your code modular and well-documented for easy maintenance.
14. Security Considerations
Be cautious when parsing HTML from untrusted sources, as it can lead to security vulnerabilities. Sanitize and validate incoming data to prevent potential attacks.
15. Conclusion
PowerShell’s HTML parsing capabilities empower you to extract valuable information from websites efficiently. Whether you’re a developer, sysadmin, or data analyst, mastering PowerShell’s web data extraction techniques opens up a world of possibilities.
FAQs
Q1: Is PowerShell only for Windows systems?
A1: Yes, PowerShell is primarily designed for Windows systems, but there’s an open-source version called PowerShell Core that works on various platforms.
Q2: Can I use PowerShell to interact with APIs?
A2: Absolutely! PowerShell can make API calls, retrieve data, and perform various operations just like other programming languages.
Q3: Does HTML parsing with PowerShell require coding knowledge?
A3: While coding knowledge is beneficial, PowerShell’s syntax is relatively straightforward, making it accessible to beginners.
Q4: Are there any limitations to HTML parsing using PowerShell?
A4: PowerShell’s HTML parsing capabilities are robust, but it might struggle with highly complex websites with intricate structures.
Q5: Where can I learn more about advanced PowerShell scripting?
A5: You can find extensive resources, tutorials, and communities online dedicated to PowerShell scripting and automation.