Introduction
In web development, CSS (Cascading Style Sheets) plays a vital role in styling and formatting web pages. Selectors are the backbone of CSS, as they allow developers to target specific elements on a web page and apply styles. CSS selector specificity is the concept that determines the priority and hierarchy of selectors when multiple selectors target the same element. In this article, we will explore the ins and outs of CSS selector specificity, its importance in web development, and how to effectively utilize selectors to style web pages.
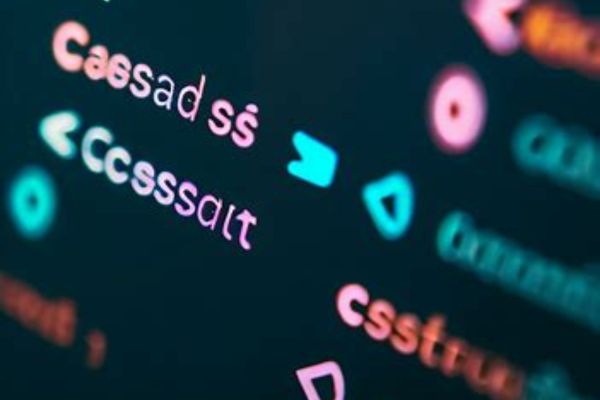
1. Understanding CSS Selectors
CSS selectors are patterns used to select and target specific HTML elements for styling. Selectors can be based on element names, IDs, classes, attributes, relationships between elements, and more. By combining different selectors, developers can precisely target specific elements and apply styles accordingly.
<!-- Example of an element selector -->
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
/* Styling elements using element selectors */
h1 {
color: blue;
}
p {
font-size: 16px;
}
2. Specificity Rules
CSS selector specificity determines which styles are applied to an element when conflicting rules exist. Specificity is calculated based on the types of selectors used. Generally, the more specific a selector, the higher its specificity. Specificity rules can be summarized as follows:
- Inline styles have the highest specificity.
- ID selectors have a higher specificity than class selectors and element selectors.
- Class selectors have a higher specificity than element selectors.
- The universal selector (*) has no specificity.
- Combinations of selectors and pseudo-classes increase specificity.
3. Inline Styles
Inline styles are CSS styles applied directly to an HTML element using the style
attribute. They have the highest specificity, meaning that inline styles will always override any other external or internal styles applied to the same element. While inline styles can be useful for quick styling fixes, they are generally not recommended for extensive styling due to their low maintainability.
<!-- Example of inline styles -->
<p style="color: red;">This paragraph has inline styles.</p>
4. ID Selectors
ID selectors are denoted by the #
symbol followed by an ID name. These are unique identifiers assigned to specific elements in the HTML markup. ID selectors have a higher specificity than class selectors and element selectors. It is best practice to use ID selectors sparingly and only for unique elements on a page to maintain a modular and reusable codebase.
<!-- Example of an ID selector -->
<div id="my-element">This element has an ID.</div>
/* Styling an element using an ID selector */
#my-element {
background-color: #f0f0f0;
padding: 10px;
}
5. Class Selectors
Class selectors target elements based on their assigned class names. Its names can be shared among multiple elements, allowing developers to apply the same styles to multiple elements. Class selectors have a higher specificity than element selectors but a lower specificity than ID selectors. They are commonly used for styling groups of related elements.
<!-- Example of a class selector -->
<div class="my-class">This element has a class.</div>
/* Styling elements using class selectors */
.my-class {
font-weight: bold;
}
6. Element Selectors
Element selectors target elements based on their HTML tag names. They have the lowest specificity compared to ID selectors and class selectors. Element selectors apply styles to all elements of the specified type on a web page. While useful for general styling, they should be used judiciously to prevent unintended style conflicts.
/* Styling elements using element selectors */
p {
color: green;
}
7. Combining Selectors
Developers can combine multiple selectors to create more specific targeting. This can be achieved by adding classes or IDs to elements, nesting selectors, or utilizing sibling and descendant selectors. Combining selectors increases specificity, allowing developers to fine-tune the styling of individual elements or groups of elements.
<!-- Example of a combination of selectors -->
<ul>
<li class="item">Item 1</li>
<li class="item">Item 2</li>
<li class="item active">Item 3</li>
</ul>
/* Styling elements using a combination of selectors */
.item {
color: gray;
}
.item.active {
color: blue;
font-weight: bold;
}
8. Pseudo-Classes and Pseudo-Elements
Pseudo-classes and pseudo-elements provide additional ways to select and style elements based on various states or specific parts of an element. It targets elements in specific conditions, such as :hover
for mouse hover effects or :first-child
for selecting the first child element of a parent. Pseudo-elements target specific parts of an element, such as ::before
and ::after
for adding content before or after an element’s content.
/* Styling elements using pseudo-classes */
a:hover {
color: red;
}
/* Styling elements using pseudo-elements */
p::before {
content: "Before";
}
p::after {
content: "After";
}
9. The !important
Rule
The !important
rule is a declaration that can be added to a CSS property to override any other styles applied to the same element. While it can be a powerful tool for specific cases, it should be used sparingly and with caution. Overusing the !important
rule can lead to code maintenance issues and make it challenging to troubleshoot styling problems.
/* Example of using the !important rule */
p {
color: green !important;
}
10. Inheritance and Specificity
CSS styles can be inherited from parent elements to their child elements. Inheritance allows developers to apply styles to parent elements and have those styles automatically propagate to their child elements. However, when conflicting styles exist between inherited styles and styles applied directly to an element, specificity rules come into play to determine the final styling.
<!-- Example of inheritance -->
<div class="parent">
<p>This paragraph inherits styles from its parent.</p>
</div>
/* Styling parent and inherited child elements */
.parent {
font-weight: bold;
}
.parent p {
color: blue;
}
11. Best Practices
for Using Selectors
To utilize CSS selectors effectively, consider the following best practices:
- Use classes for reusable styles and IDs for unique elements.
- Avoid inline styles except for small, isolated changes.
- Keep selectors as specific as necessary to avoid style conflicts.
- Use descriptive class names to enhance code readability and maintainability.
- Combine selectors intelligently to target elements precisely.
- Use comments in CSS code to provide context and explanations.
12. Advanced Selector Techniques
CSS provides advanced selector techniques to target elements based on complex conditions and relationships. Some of these techniques include attribute selectors, sibling selectors, child selectors, adjacent selectors, and more. Understanding and utilizing these advanced techniques can significantly enhance the flexibility and precision of CSS styling.
/* Example of an attribute selector */
input[type="text"] {
border: 1px solid gray;
}
/* Example of a sibling selector */
h2 + p {
margin-top: 20px;
}
/* Example of a child selector */
div > p {
color: red;
}
/* Example of an adjacent selector */
h3 ~ p {
font-weight: bold;
}
13. Common Mistakes to Avoid
When working with CSS selectors, there are several common mistakes that developers should be aware of and avoid. These include:
- Overusing the
!important
rule. - Relying solely on inline styles.
- Using overly generic or ambiguous selectors.
- Neglecting code organization and maintainability.
- Ignoring specificity conflicts and unintended style overrides.
14. A Deep Dive into Testing and Debugging CSS Selectors
If you've ever designed a website, you know that CSS selectors are the magic behind the scenes. Being able to identify and resolve issues within your CSS selectors can transform your web design. Today we're focusing on practical methods and tools for testing and debugging these selectors. It's not just about knowing the tools exist, but how you can leverage them effectively.
Common Pitfalls and How to Avoid Them
Even skilled designers sometimes encounter problems when testing and debugging CSS Selectors. Understand these common pitfalls to avoid unnecessary frustration. We'll walk you through each issue, why it happens, and how to solve it.
When Things Go Wrong: Case Examples
Nothing teaches better than real-world examples. That's why we're giving you an inside look at instances when incorrect use of CSS Selectors led to a troubled web design. We'll delve into how the problems were identified and the testing and debugging methods used to rectify the situation.
The Evolution of Testing and Debugging
As the web continues to evolve, so too does the complexity of testing and debugging CSS selectors. You'll need different or additional approaches as more intricate conditions are applied. Whether you're using advanced selector techniques or maintaining a site's design over time, we'll discuss how your approach to testing and debugging must adapt.
The Importance of Ongoing Testing and Debugging
Creating styles is just the beginning. Even after a site's design is live, your work isn't over. Testing and debugging is a continuous task that ensures your site remains functional and attractive. Whether it's for initial design or ongoing maintenance, having an understanding of testing and debugging CSS selectors will keep your website running smoothly.
You're here to learn, and our aim is to make sure you leave with a stronger understanding of this topic. We're simplifying where needed, adding detail where necessary. Regardless of your experience level, we hope you find value in our deep dive into testing and debugging CSS selectors.
Inspecting and debugging the CSS selectors is vital to guarantee that styles are accurately and consistently applied. Utilizing browser developer tools enables developers to verify selector targeting, evaluate computed styles, and pinpoint any conflicts or issues. Regular inspection and debugging have the potential to save considerable time, effort, and reduce the likelihood of run-time styling errors.
Inspecting and debugging CSS selectors isn't just about checking the applied styles. It's essential to understand the concept of CSS specificity and how it impacts the debugging process. When a style isn't applied as expected or faces conflicts, it might be due to the specificity rules not being correctly implemented. Therefore, it's important to conduct regular checks of the specificity to ensure correct application and avoid potential issues.
The use of browser developer tools should be explored further. These tools are not just helpful in selector targeting, but they can also evaluate computed styles, find conflicts, and troubleshoot any issues. It's crucial to learn how to effectively use these tools in everyday development.
Testing shouldn't just be limited to CSS selectors. Inline styles, ID selectors, class selectors, and element selectors also require regular testing to ensure they're functioning as intended. In addition, discussing how to check and test pseudo-classes, pseudo-elements, and the "!important" rule is vital for comprehensive CSS debugging.
It's not uncommon for beginners to make mistakes during the testing and debugging process. These can be avoided by being aware of common pitfalls and practicing regularly. Some universal errors involve incorrect usage or understanding of the CSS inheritance. It's important to understand how parent elements can affect the child elements and how to debug inherited styles.
In conclusion, a detailed walkthrough of testing and debugging CSS selectors, along with an exploration of tools and practices, can drastically improve the quality of the webpage. It not only helps developers ensure smooth functioning but also enhances their knowledge of CSS, promoting better design and development practices.
15. Conclusion
CSS selector specificity is a fundamental concept in web development that determines how styles are applied to elements. Understanding and effectively utilizing selectors can significantly enhance the precision and maintainability of CSS styling. By following best practices, avoiding common mistakes, and leveraging advanced selector techniques, developers can create visually appealing and well-structured web pages.
FAQs
Q1: What is the importance of CSS selector specificity in web development?
CSS selector specificity is crucial in determining which styles are applied to elements when conflicting rules exist. It ensures that styles are accurately targeted and helps maintain code modularity and reusability.
Q2: Can inline styles override other styles applied to the same element?
Yes, inline styles have the highest specificity and will always override any other external or internal styles applied to the same element.
Q3: How can I target specific parts of an element using CSS?
You can use pseudo-classes and pseudo-elements to target specific parts of an element. Pseudo-classes target elements in specific conditions, while pseudo-elements target specific parts of an element’s content.
Q4: Are there any best practices for using CSS selectors?
Yes, some best practices include using classes for reusable styles, avoiding inline styles, keeping selectors as specific as necessary, using descriptive class names, and combining selectors intelligently.
Q5: How can I test and debug CSS selectors?
You can use browser developer tools to inspect and verify selector targeting, check computed styles, and identify any conflicts or issues. Regular testing and debugging can help ensure correct and consistent styling.
How To Handle CSS Precedence When Using React And CSS Classes?