If you are working with Next.js and come across the error “NextRouter was not mounted”, this could be a frustrating issue that disrupts your development workflow. This error commonly arises from problems with how the useRouter
hook or routing system is used in your application. In this article, we’ll explore what this error means, why it happens, and how to fix it.
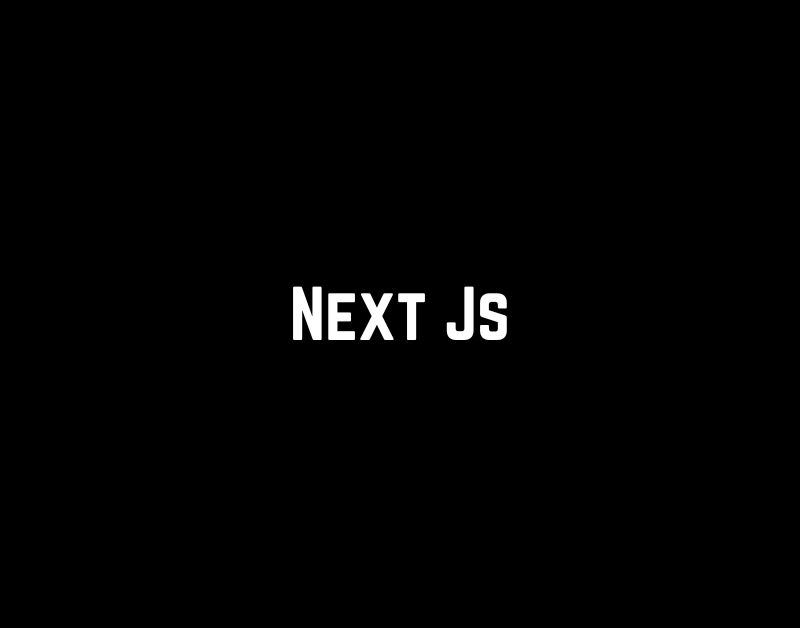
What is the useRouter
Hook?
Next.js offers a built-in router to handle navigation between pages. The useRouter
hook is a utility provided by Next.js to access router information in functional components. It allows you to get the current route’s pathname, query parameters, and provides methods to programmatically navigate between pages.
import { useRouter } from 'next/router';
const MyComponent = () => {
const router = useRouter();
return <div>Current route: {router.pathname}</div>;
};
Common Usage of useRouter
The useRouter
hook is typically used to:
- Access query parameters.
- Programmatically navigate between routes using
router.push()
. - Retrieve information like the current path or locale.
JavaScript Function Parameters
Default Parameters in JavaScript: A Comprehensive Guide
Why Does the “NextRouter Was Not Mounted” Error Occur?
This error occurs when the Next.js routing context has not been properly initialized before the useRouter
hook is called. Below are some common scenarios where this might happen:
1. Using useRouter
Outside a Component
One of the most common mistakes is using the useRouter
hook outside of a React component. The useRouter
hook is specifically designed to be used inside React functional components, and calling it elsewhere (e.g., in a helper function) will result in the error.
// Incorrect usage:
const router = useRouter(); // ❌ This is outside the component!
const myHelperFunction = () => {
console.log(router.pathname);
};
To fix this, ensure that the hook is only used inside a functional component.
// Correct usage:
const MyComponent = () => {
const router = useRouter();
const myHelperFunction = () => {
console.log(router.pathname);
};
return <div>Current route: {router.pathname}</div>;
};
2. Server-Side Rendering Context
Next.js uses both server-side and client-side rendering. The useRouter
hook is only available in client-side rendering because it deals with browser-specific functionality like navigating between routes.
If you mistakenly use useRouter
in server-side code like getServerSideProps
or getStaticProps
, this error can occur.
// Incorrect: Using `useRouter` in server-side code
export async function getServerSideProps(context) {
const router = useRouter(); // ❌ This is server-side code!
// ... server-side logic
}
To resolve this, make sure that useRouter
is used in a client-side component and not in server-side functions.
3. Using Custom _app.js
or _document.js
Incorrectly
If you have customized your Next.js app by modifying the _app.js
or _document.js
files, ensure that the internal router context is properly initialized.
In _app.js
, Next.js automatically wraps the application with its own <RouterProvider>
context. If you accidentally break this context (e.g., by overriding it incorrectly), you might see the “NextRouter was not mounted” error.
// Correct usage in `_app.js`
import '../styles/globals.css';
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
How to Fix “NextRouter was not Mounted”
Let’s walk through some steps to fix the error based on different scenarios.
1. Ensure the Hook is Used Inside a Component
Make sure that the useRouter
hook is only used within a React component. If you are calling it outside of a component, move the logic inside.
import { useRouter } from 'next/router';
const MyComponent = () => {
const router = useRouter();
const navigate = () => {
router.push('/about');
};
return <button onClick={navigate}>Go to About</button>;
};
2. Check Server-Side Functions
Ensure that useRouter
is not being used in getStaticProps
or getServerSideProps
, as these are server-side functions and don’t have access to the client-side router.
// Correct usage: Do not use `useRouter` in server-side functions
export async function getServerSideProps() {
// Server-side logic here
return { props: {} };
}
3. Verify Your Custom _app.js
If you have customized the _app.js
file, ensure you aren’t accidentally breaking the router context. You don’t need to manually handle the router provider in most cases, as Next.js takes care of this.
4. Conditional Router Usage
If your component is rendered on both the server and client-side, you might want to ensure that the router is only accessed after the component mounts on the client.
import { useRouter } from 'next/router';
import { useEffect, useState } from 'react';
const MyComponent = () => {
const router = useRouter();
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null;
}
return <div>Current route: {router.pathname}</div>;
};
Conclusion
The “NextRouter was not mounted” error in Next.js occurs when the useRouter
hook is used incorrectly or outside of the proper context. By ensuring that you are using the hook inside client-side functional components and avoiding its use in server-side functions, you can resolve this issue.
Understanding the distinction between server-side and client-side behavior in Next.js is essential to avoid this and other routing-related errors. Keep these best practices in mind when working with Next.js routing to ensure a smoother development experience.
Def Javascript: Understanding Its Role in Modern Web Development