In today’s interconnected digital landscape, the seamless integration of web services is a cornerstone of software engineering. RESTful services, standing as a beacon of simplicity and effectiveness, have become an integral part of that domain. This essay embarks on a journey to unravel the core principles that underpin RESTful services, demonstrating their elegant stateless interactions and standardized protocol usage that thrive in the Java ecosystem. As we navigate through the popular libraries and tools fashioning RESTful clients in Java, from Apache HttpClient to the finesse of Spring Boot, we’ll illuminate the path to mastery for both novices and seasoned developers alike.
Understanding RESTful Services
In the world of software architecture, RESTful services have become an indispensable framework for building scalable web applications. REST, or Representational State Transfer, is a set of guidelines that, when followed, enables the creation of services that work efficiently on the web. So, what exactly makes RESTful services so essential for modern application development, and how do they function? Let’s dissect this framework to understand its core principles and operations.
At their most fundamental level, RESTful services are designed to be stateless and resource-based. This means that each service interaction is executed as if it were a discrete transaction, independent of any previous interaction. Utilizing HTTP protocols, RESTful services communicate through a straightforward, yet potent set of operations known as CRUD – Create, Read, Update, Delete. This simplification leads to services that are not just easy to understand but also easy to integrate across various platforms and languages.
The backbone of RESTful services lies in its use of resources. In REST terms, a resource is anything that’s important enough to be referenced as a standalone entity. Typically, resources are accessed via URIs (Uniform Resource Identifiers). Imagine each resource as a unique web page, and its URI is its web address. When a client wants to interact with a resource, it sends an HTTP request – employing methods like GET to retrieve a resource, POST to create one, PUT to update it, or DELETE to remove it.
Handling these HTTP requests is where RESTful services shine. When a server receives a request, it interprets the HTTP method and the URI to determine what action is being requested on which resource. The beauty of REST is that it relies on the existing infrastructure of the web, meaning it leverages what’s already in place rather than requiring specialized systems to operate.
Additionally, RESTful services put a heavy emphasis on the format of the output. JSON (JavaScript Object Notation) has become the de facto standard due to its lightweight nature and compatibility with web technologies, although XML and other formats can also be utilized. The choice of format is crucial because it defines how different systems digest and process the data they exchange.
One of the greatest advantages of RESTful services is their statelessness. Since each request is an independent operation, RESTful APIs can scale up to meet high demand without concern for the sessions other services might require. This aspect is essential for cloud computing and services that might experience unpredictable loads.
In essence, RESTful services epitomize the philosophy of using simple, universal standards to build powerful, interoperable web applications. Their adherence to stateless operations and system-agnostic communication ensures that they can be seamlessly adopted, maintained, and scaled, allowing for smooth, effective exchange of data across a myriad of systems. In an era where flexibility and efficiency in software development are paramount, the relevance of RESTful services is indisputable. Armed with an understanding of how they work, developers and tech enthusiasts alike can architect web-based solutions that are both robust and elegantly streamlined.
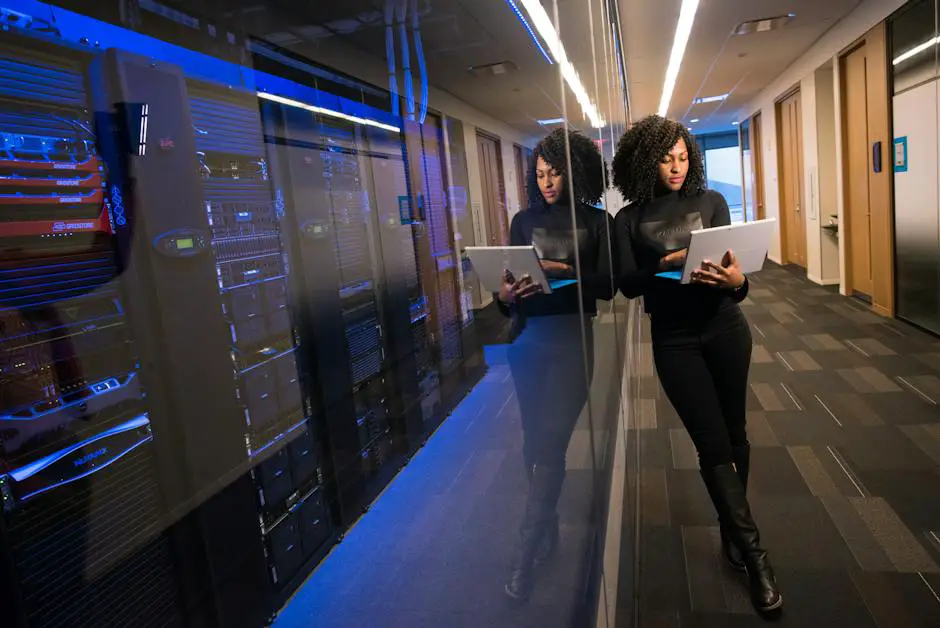
Popular RESTful Client Libraries for Java
Java Libraries Setting the Standard for Building RESTful Clients
When it comes to building RESTful clients in Java, a few libraries have established themselves as indispensable tools in any developer’s arsenal. They streamline RESTful service integration and provide a much-needed abstraction layer to handle complex HTTP communications, ultimately facilitating the development process and enabling more robust client implementations.
OkHttp: A Critical Tool for Efficient HTTP Communications
In the realm of HTTP client libraries, OkHttp is a front-runner, offering features that ensure HTTP requests are sent and responses are handled with minimal fuss. Its ability to maintain a connection pool for repeat requests to the same host eliminates the overhead of creating a new connection each time, which is a boon for performance. Also, OkHttp’s interface for crafting requests and reading responses is clean and intuitive, which aligns with a no-nonsense approach to REST client development.
Retrofit: A Retrofit to Developer Productivity
Retrofit has gained popularity for its declarative style and its focus on converting HTTP API into a Java interface. It builds on the capabilities of OkHttp by adding a layer of abstraction that allows HTTP requests and responses to be represented as Java methods and objects, respectively. Retrofit works well with converters like Gson or Jackson to seamlessly handle serialization and deserialization of data. Interceptors to log or modify requests and responses make it phenomenally flexible, accustoming it to scenarios where precise tuning of HTTP interactions is paramount.
Jersey: A Champion of JAX-RS Compliance
For Java developers, Jersey remains the go-to JAX-RS API implementation for RESTful services. It shines with features that align with standardized Java EE technologies, providing a comprehensive framework that eases development of REST clients and servers alike. Jersey’s model is robust and follows the orthodoxy of enterprise Java, making it an ideal choice for those entrenched in the Java ecosystem.
RestTemplate: Spring’s Answer to Simplicity
Spring Framework’s RestTemplate has traditionally been favored in the Spring community for RESTful client creation. Its methods for HTTP operations encapsulate tedious boilerplate code, freeing developers to focus on the business logic. It offers error handling and integrates effortlessly with Spring’s wider ecosystem, which is invaluable for applications dependent on Spring components. However, worth noting is that the newer Spring WebFlux’s WebClient is recommended over RestTemplate for fresh developments as it embraces reactive programming principles and offers non-blocking I/O operations for improved performance.
WebClient: The Reactive Pacesetter
Spring’s WebClient is the modern alternative to RestTemplate, designed to handle asynchronous requests and catering to reactive applications. It is a part of Spring WebFlux, and it’s built to operate in event-driven environments where scalability and efficiency are pivotal. Its reactive nature makes it adept at handling concurrent requests with fewer resources, making it the library of choice for developers eyeing the cutting edge of RESTful client technology.
Selecting the appropriate Java library for building a RESTful client decisively depends on specific project requirements, existing technology stack, performance demands, and developer familiarity. However, the aforementioned libraries stand out due to their widespread adoption, continued support, and reputation for facilitating the creation of effective, high-quality RESTful clients. Whether aligning with traditional enterprise patterns or embracing asynchronous paradigms, there’s a library tailored to the needs of Java developers committed to RESTful client construction.
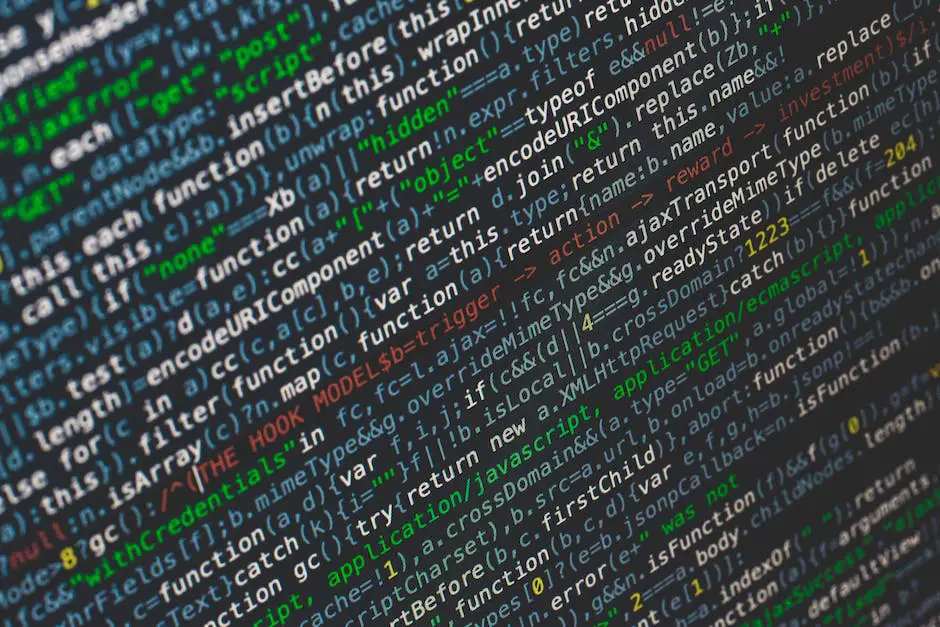
Creating a RESTful Client with Java and Spring Boot
Moving from the comprehensive overview of RESTful services and the potential Java libraries available for their implementation, it’s critical to pivot into how one can actually put these conceptions into practice specifically using Java and Spring Boot. To create a RESTful client in this robust framework, harness the strengths of Spring’s RestTemplate or WebClient, both of which offer a polished experience for HTTP interactions in Java applications.
First of all, one must understand when to use RestTemplate versus WebClient. RestTemplate, for many years, has been the go-to synchronously executing client, excellent for common API consumption whilst Thread blocking. On the other hand, WebClient, introduced in Spring 5, supports asynchronous operations and fits especially well with non-blocking and reactive programming models. For applications that demand scalability, real-time processing, and efficient resource usage, WebClient is the top choice.
Here’s a step-by-step guide to implement a RESTful client using RestTemplate in a Spring Boot application:
- Add Spring Boot Starter Web dependency: Ensure to include ‘spring-boot-starter-web’ in the build configuration to access RestTemplate.
- Create a Bean for RestTemplate: This allows for dependency injection and the ability to customize configurations, such as timeouts and message converters.
<pre><code>@Configuration public class AppConfig { @Bean public RestTemplate restTemplate() { return new RestTemplate(); } }</code></pre>
- Inject RestTemplate into your service: This allows for reuse and management by Spring’s container.
<pre><code>@Service public class ApiService { @Autowired private RestTemplate restTemplate; // ...methods utilizing restTemplate }</code></pre>
- Use RestTemplate’s methods for CRUD operations: getForObject, postForObject, put, and delete are direct mappings to HTTP verbs, suitable for interaction with most RESTful APIs.
Moving on to WebClient, it’s designed to be used with Spring’s reactive stack which supports asynchronous I/O. Here’s how to use WebClient:
- Add WebClient dependency: ‘spring-boot-starter-webflux’ is required for reactive web programming support and WebClient.
- Configure WebClient Bean: Like RestTemplate, WebClient is also auto-configurable and can be customized in a configuration class.
<pre><code>@Bean public WebClient.Builder webClientBuilder() { return WebClient.builder(); }</code></pre>
- Inject WebClient.Builder and build an instance: This enables creating a WebClient instance with various configurations as per requirements.
<pre><code>@Service public class ReactiveApiService { private final WebClient webClient; public ReactiveApiService(WebClient.Builder webClientBuilder) { this.webClient = webClientBuilder .baseUrl("http://api.example.com") .build(); } // ...reactive methods using webClient }</code></pre>
- Perform operations: WebClient provides several methods, such as get, post, put, and delete, along with a fluent API to construct the requests and handle responses in an asynchronous manner.
When selecting between RestTemplate and WebClient, consider the application’s exact requirements and the overall architecture. For blocking IO or traditional servlet stacks, RestTemplate remains a suitable choice. For non-blocking, reactive systems, embrace WebClient to leverage its full potential.
Pragmatically speaking, whichever tool adopted, the key is to ensure proper error handling, connection pooling, and implementing timeouts, thus ushering applications into an era of resilience and efficiency. The judicious selection of a RESTful client is indispensable for scaling software systems and simplifying their intercommunications while promoting modularity and improving performance.
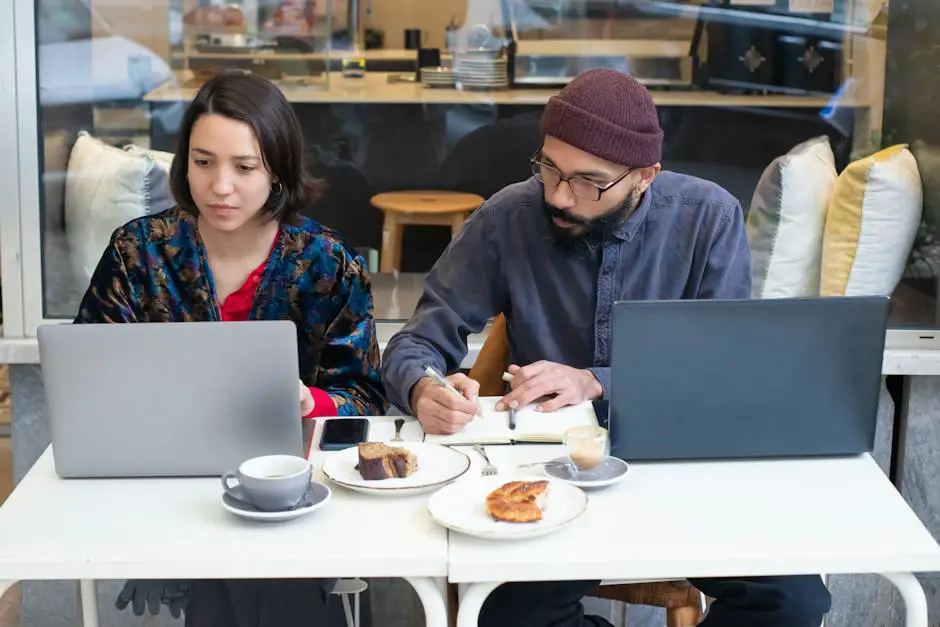
Authentication and Security in RESTful Clients
Enhancing Authentication and Security in RESTful Java Clients
In the realm of RESTful Java clients, addressing authentication and security is pivotal to protecting resources from unauthorized access and ensuring data integrity. Here’s how tech enthusiasts tackle these concerns efficiently.
OAuth 2.0 for Delegated Authorization:
OAuth 2.0 is the industry standard for secure delegated access, allowing applications to obtain limited access to user accounts on an HTTP service. Java clients can leverage OAuth libraries like ScribeJava for implementing OAuth authorization flows, which grant tokens to be used for API requests, without exposing user credentials directly.
JWT for Compact Token-based Authentication:
JSON Web Tokens (JWT) offer a self-contained way for securely transmitting information between parties. When a user authenticates, a JWT is issued, which can be verified and trusted because it is digitally signed. Using libraries like JJWT in Java clients assists in creating and parsing JWTs, thus streamlining authentication and authorization processes in a lightweight manner.
HTTPS for Secure Communication:
Always ensure communication with RESTful services is over HTTPS to prevent man-in-the-middle attacks. Java clients should make use of SSL/TLS protocols to encrypt data in transit. Libraries such as OkHttp and WebClient inherently support HTTPS and are optimized for such secure transmissions.
Client Certificates for Mutual Authentication:
For an additional layer of security, mutual TLS (mTLS) can be used where both the client and the server authenticate each other with certificates. Java clients can be configured to present client certificates to RESTful services that mandate certificate-based authentication, thus enhancing trust and security.
Rate Limiting and Throttling:
Institute rate limiting on the client side to avoid overwhelming RESTful services with too many requests, which could trigger security breaches. With proper error handling tied into rate limiting, Java clients can more gracefully handle request rejections due to exceeding limits, ensuring they respect server constraints and contribute to overall system stability.
CSRF Protection for State-Changing Operations:
For Java web applications, Cross-Site Request Forgery (CSRF) tokens can safeguard against unauthorized state-changing operations initiated by malicious actors. Modern Java web frameworks often have built-in CSRF protection mechanisms that can be easily configured for RESTful clients.
Input Validation to Mitigate Security Risks:
All user input should be validated to protect against injection attacks. Using a combination of server-side validation and Java client-side input sanitization, the system can prevent most common injection vulnerabilities, such as SQL injection and cross-site scripting (XSS).
Harden Headers to Reduce Attack Surface:
Security headers like Content Security Policy (CSP), X-Content-Type-Options, and X-XSS-Protection serve as the first defense against several classes of attacks. Configuring these headers properly in responses from Java clients can make a substantial difference in the security posture of an application.
Avoid Client-side Storage of Sensitive Data:
Java clients should minimize storing sensitive information, such as tokens and passwords, on the client side. If storage is necessary, use secure mechanisms such as encrypted preferences or key stores, and clear such information as soon as it is no longer needed.
Security is an ever-evolving field, and adhering to best practices for authentication and secure communication is paramount. By implementing the above strategies in RESTful Java clients, developers can significantly bolster the security of their systems and protect their users’ data. With a focus on solid authentication mechanisms and secure transmission protocols, Java clients for RESTful services can maintain a strong defense against potential threats.
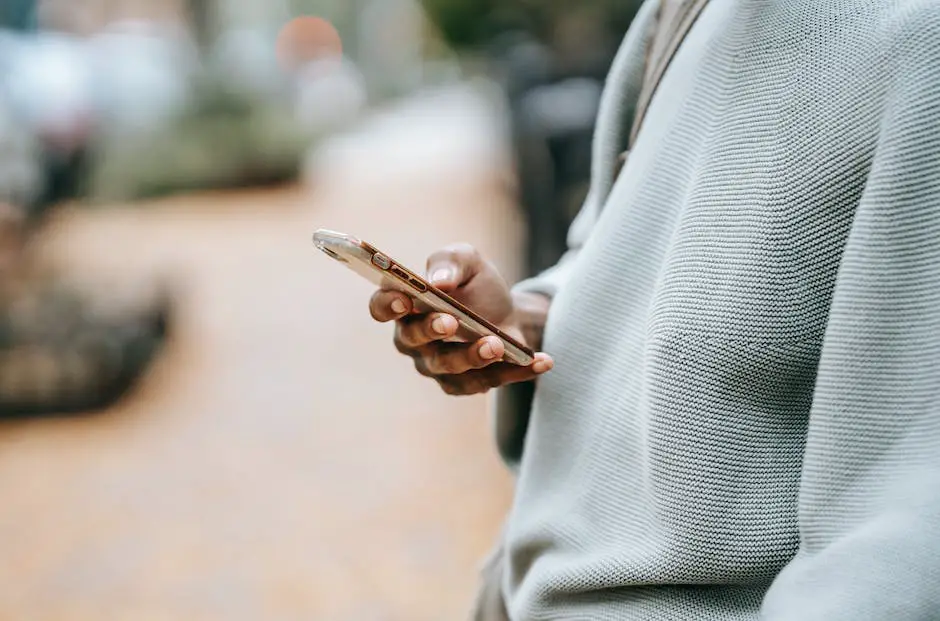
Unit Testing RESTful Clients in Java
Leveraging Mocking Frameworks for Effective Java REST Client Unit Testing
In the realm of Java REST client development, unit tests are the backbone of a solid, reliable, and maintainable codebase. An often-overlooked aspect of this is the strategy for mocking external dependencies, which ensures that unit tests are truly isolated, examining components in a microcosm without real-world data interaction.
Mockserver for Simulating APIs
Mockserver is an indispensable tool for simulating RESTful APIs. It allows developers to create a mock server that behaves like the end system. Java REST clients can then interact with this controlled environment where responses are predictable and tunable for various test scenarios. It’s an excellent way to validate that the client handles expected and unexpected responses robustly.
WireMock: Realistic HTTP Server Stubbing
WireMock stands out for its ability to mimic the behavior of complex APIs with little setup overhead. Its fluent Java API enables the creation of stubs for HTTP requests, giving testers full control over responses. It goes a step further with support for proxying, which records real service behavior that can be useful in aggressive testing of Java REST clients against potential real-world anomalies.
JUnit 5 with Mockito for Dependency Mocking
Unit testing in Java would be nearly impossible to envision without mentioning JUnit 5 – the current standard for writing repeatable tests. When coupled with Mockito, it becomes a powerhouse for mocking dependencies. It allows testers to simulate service-layer responses, ensuring that REST client logic can be tested in isolation from services it relies on. Mockito’s ArgumentCaptor feature is particularly useful for capturing parameters passed to mocked methods, asserting that the REST client acts upon received data correctly.
Utilizing Testcontainers for Integration Testing
While slightly outside the realm of conventional unit testing, it’s important to mention Testcontainers – a powerful library that supports Java integration tests with real services running in Docker containers. With Testcontainers, one can spin up a real database or any other service your Java REST client may interact with online, carry out tests, and then dispose of it cleanly. This is integration testing with the simplicity of unit tests.
AssertJ for Fluent Assertion Syntax
AssertJ provides a rich set of fluent assertions, which improve readability and make test code more intuitive. When dealing with complex responses from a RESTful service, it helps in clearly stating what the Java REST client is expected to produce, from JSON payloads to HTTP status assertions, making tests easier to understand and maintain.
Continuous Integration Pipelines for Automation
Lastly, to ensure unit tests run as expected after each code change, integrating them into a CI/CD pipeline is vital. Tools such as Jenkins or GitHub Actions automatically execute test suites upon code commits, allowing developers to detect and fix issues promptly. Not only do they automate the testing process, but they also ensure tests are run in a clean, standardized environment every time.
By embedding these strategies into the development workflow, Java RESTful clients can be unit tested with high effectiveness, leading to applications that are robust, secure, and maintainable. Ultimately, it’s about building confidence in the code that powers today’s interconnected software ecosystem.
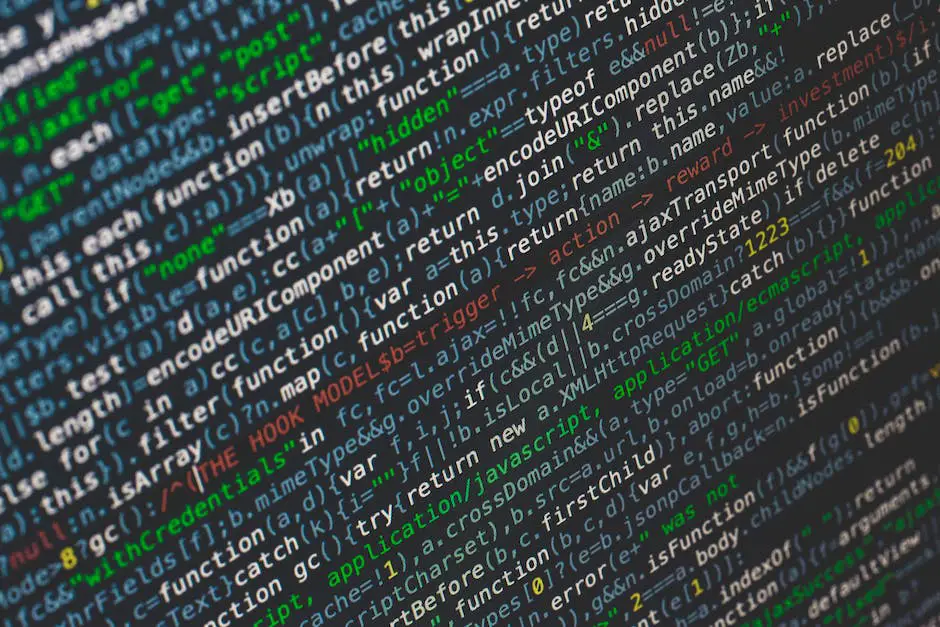
Performance Optimization of Java RESTful Clients
Maximizing Java RESTful Clients: From Connection Management to Performance Tuning
In the high-stakes world of software development, performance can make or break the user experience. When building Java RESTful clients, every millisecond counts. So how does one squeeze out every ounce of performance from these clients? Let’s dive in.
Connection management is a critical aspect often overlooked. Utilizing a shared connection pool across client instances significantly reduces latency. Apache HttpClient provides robust connection pooling capabilities, essential for high-load environments. It’s imperative to properly configure the pool size and the connection timeout to match your specific use case and avoid bottlenecks.
Caching strategies come into play when aiming to reduce network calls. Implementing an HTTP cache reduces the need to fetch unchanged resources, thereby conserving bandwidth and speeding up response time. EHCache or Caffeine can be integrated with HttpClient to cache responses based on headers like Cache-Control, paving the way for an efficient RESTful client.
Compression is another lever to pull when optimizing performance. Clients can leverage GZip or Deflate compression to reduce the size of request and response bodies. Make sure to enable compression in the client configuration, and watch data transfer size plummet – a straightforward tweak with substantial impact.
When looking at serialization and deserialization, faster libraries can lead to performance improvements. Consider libraries like Jackson or Gson for JSON processing, with a focus on their abilities to handle large and complex data structures. Furthermore, be meticulous in choosing serialization formats; binary ones like Protocol Buffers can offer remarkable speed and efficiency gains over textual formats like JSON or XML.
Batching and asynchronous requests harness the power of modern CPUs more effectively. Rather than executing synchronous operations that waste valuable CPU cycles waiting for responses, batching sends multiple requests in a single operation, and asynchronous processing takes advantage of callback mechanisms to handle responses when ready, maintaining high throughput.
Performance tuning also involves setting appropriate memory allocation for the JVM. Garbage collection can have a pronounced impact on performance. Hence, fine-tuning garbage collection strategies, such as selecting the right collector or setting heap size, can be pivotal in getting the most out of your RESTful clients.
Profiling and monitoring are key in identifying bottlenecks. Tools like JProfiler, Java Mission Control, or Application Performance Management (APM) services, such as New Relic or Dynatrace, provide critical insights into the client’s performance at runtime. By continuously monitoring, one can preemptively catch issues that could degrade performance.
Lastly, reactive programming can be a game changer for RESTful clients dealing with high-load, latency-sensitive workloads. Libraries like Project Reactor, integrated with WebClient, allow for event-driven, non-blocking interaction patterns that can lead to more scalable services by optimizing resource usage.
Incorporating these strategies can significantly enhance a Java RESTful client’s performance. However, remain vigilant; not all optimizations are universally beneficial. They should be considered in the context of specific application requirements. Measure, then optimize, and continuously test – the trifecta for high-performance RESTful clients.
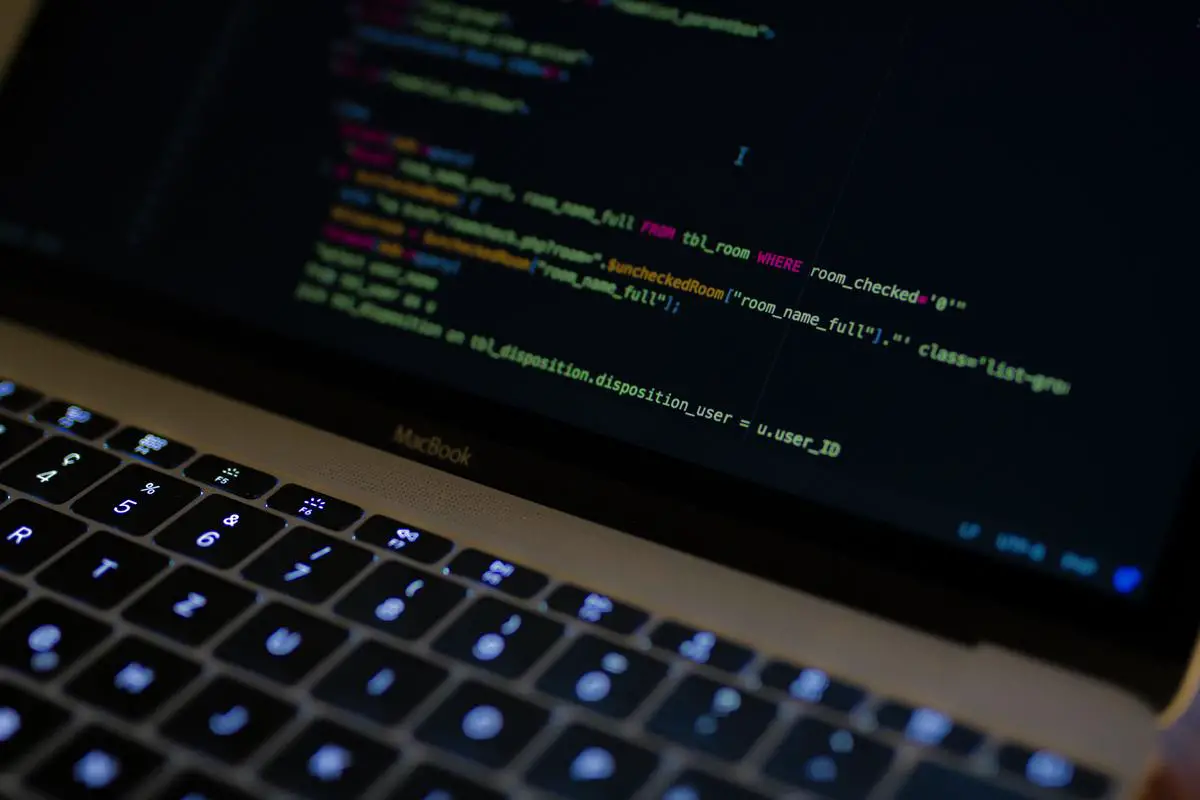
Photo by casparrubin on Unsplash
Embarking on the development of RESTful clients in Java is a venture that marries the robustness of Java with the flexibility of RESTful services, creating an environment ripe for innovation and growth. Through this examination of tools, security practices, testing frameworks, and performance optimizations, we have charted a course that enables developers to forge efficient and secure RESTful clients. Armed with this knowledge, you stand ready to craft solutions that not only meet the demand for functionality but also uphold the standards for quality and security in the dynamic landscape of software development.
Understanding JSON Parsing with JavaScript