Tailwind CSS vs Material-UI- In the ever-evolving realm of front-end development, the choice of a framework can significantly impact the way you build and style your web applications. Two prominent contenders in this space are Tailwind CSS and Material-UI. Each brings its unique approach to the table. In this article, we will delve into the depths of these frameworks, providing you with code examples in the form of tables to help you make an informed decision for your next project.
Introduction
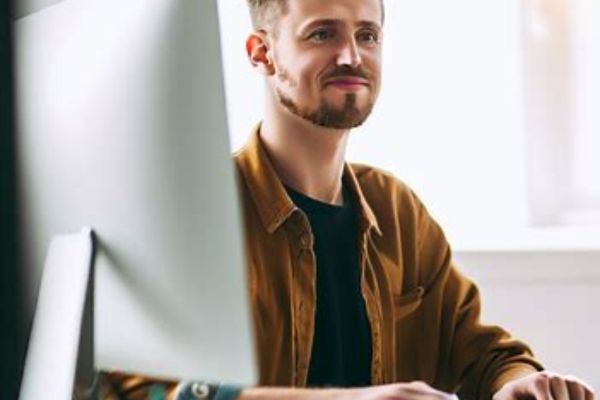
Frontend frameworks play a pivotal role in shaping the visual and interactive aspects of web applications. Tailwind CSS and Material-UI are two influential frameworks, each offering distinct advantages. Let’s explore these frameworks in-depth and compare their capabilities.
Getting Started
To set up Tailwind CSS, you’ll need to install it via npm and configure your project’s stylesheet. In contrast, Material-UI requires installing its core components into your React application using npm or yarn.
Tailwind CSS | Material-UI |
---|---|
npm install tailwindcss | npm install @mui/material @emotion/react @emotion/styled |
Configure tailwind.config.js | Import components and start using in React app |
Styling Elements
Tailwind CSS leverages utility classes to apply styles directly to HTML elements.
<button class="bg-blue-500 text-white p-2 rounded">Click me</button>
Material-UI employs the makeStyles
function to define styles for components.
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles((theme) => ({
button: {
background: 'blue',
color: 'white',
padding: theme.spacing(2),
borderRadius: theme.shape.borderRadius,
},
}));
// Inside component
const classes = useStyles();
// ...
<Button className={classes.button}>Click me</Button>
Creating Layouts
Tailwind CSS facilitates responsive layouts with its grid system.
<div class="grid grid-cols-2 gap-4">
<div>Left Column</div>
<div>Right Column</div>
</div>
Material-UI provides the Grid component for creating layouts.
import Grid from '@mui/material/Grid';
// Inside component
<Grid container spacing={4}>
<Grid item xs={6}>Left Column</Grid>
<Grid item xs={6}>Right Column</Grid>
</Grid>
Customization
Customizing colors and fonts in Tailwind CSS can be achieved through configuration.
// tailwind.config.js
module.exports = {
theme: {
extend: {
colors: {
primary: '#3490dc',
},
fontFamily: {
custom: ['CustomFont', 'sans'],
},
},
},
variants: {},
plugins: [],
};
Material-UI’s ThemeProvider enables comprehensive theming and customization.
import { createTheme, ThemeProvider } from '@mui/material/styles';
const theme = createTheme({
palette: {
primary: {
main: '#3490dc',
},
},
typography: {
fontFamily: 'CustomFont, sans-serif',
},
});
// Wrap app with ThemeProvider
<ThemeProvider theme={theme}>
{/* Your app components */}
</ThemeProvider>
Component Examples
Tailwind CSS offers button styling through utility classes.
<button class="bg-blue-500 text-white p-2 rounded">Click me</button>
Material-UI provides a Button component for consistent button design.
import Button from '@mui/material/Button';
// Inside component
<Button variant="contained" color="primary">Click me</Button>
Form Elements
Tailwind CSS styles form inputs using utility classes.
<input class="border p-2 rounded" type="text" placeholder="Enter your name">
Material-UI’s TextField component is designed for form inputs.
import TextField from '@mui/material/TextField';
// Inside component
<TextField label="Name" variant="outlined" />
Navigation
Creating navigation bars in Tailwind CSS involves styling HTML elements.
<nav class="bg-gray-800 text-white p-4">
<a href="#" class="text-white">Home</a>
<a href="#" class="text-white ml-4">About</a>
</nav>
Material-UI’s AppBar component streamlines navigation bar creation.
import AppBar from '@mui/material/AppBar';
import Toolbar from '@mui/material/Toolbar';
import Typography from '@mui/material/Typography';
// Inside component
<AppBar position="static">
<Toolbar>
<Typography variant="h6">Home</Typography>
<Typography variant="h6" sx={{ marginLeft:
'auto' }}>About</Typography>
</Toolbar>
</AppBar>
Animations and Transitions
Tailwind CSS provides utility classes for adding animations.
<div class="animate-pulse">Content pulsates</div>
Material-UI integrates with CSS transitions and animations.
import { CSSTransition } from 'react-transition-group';
// Inside component
<CSSTransition in={true} appear={true} timeout={1000} classNames="fade">
<div>Content fades in</div>
</CSSTransition>
// CSS
.fade-enter {
opacity: 0;
}
.fade-enter-active {
opacity: 1;
transition: opacity 1s;
}
Integration with JavaScript
Tailwind CSS can be enhanced with JavaScript libraries like Alpine.js.
<div x-data="{ open: false }">
<button @click="open = !open">Toggle</button>
<div x-show="open">Content toggles</div>
</div>
Material-UI seamlessly integrates with React events.
import React, { useState } from 'react';
// Inside component
const [open, setOpen] = useState(false);
<button onClick={() => setOpen(!open)}>Toggle</button>
<div style={{ display: open ? 'block' : 'none' }}>Content toggles</div>
Performance Considerations
Tailwind CSS generates only the CSS classes you use, leading to smaller file sizes.
Material-UI allows tree-shaking, but the entire library needs to be bundled, impacting the bundle size.
Community and Documentation
Tailwind CSS boasts an active community and thorough documentation.
Material-UI offers extensive documentation and is backed by a vibrant community.
Use Cases and Projects
Tailwind CSS is ideal for projects that require quick prototyping and iteration.
Material-UI excels in projects that demand a polished and consistent UI.
Pros and Cons
Tailwind CSS | Material-UI |
---|---|
Rapid development with utility classes | Polished design with pre-styled components |
Smaller CSS file sizes | Comprehensive theming and customization |
Limited visual consistency | Larger bundle size |
Ideal for prototyping | Best suited for sophisticated UI |
Choosing the Right Framework
Consider your project’s requirements, team expertise, and performance needs.
If You Need… | Choose… |
---|---|
Speedy prototyping | Tailwind CSS |
Refined UI design | Material-UI |
Small CSS files | Tailwind CSS |
Comprehensive theming | Material-UI |
Component consistency | Material-UI |
Conclusion
Tailwind CSS and Material-UI are both powerful frontend frameworks, each catering to specific needs. Tailwind CSS provides rapid development with utility classes, while Material-UI offers a refined UI through pre-styled components. By analyzing your project’s requirements and considering the strengths of each framework, you’ll be equipped to make an informed decision for your front-end endeavors.
FAQs
- Can I use Tailwind CSS and Material-UI together?
Yes, it’s possible to use them together, but it might lead to complex styles and potential conflicts. - Which framework has better community support?
Both frameworks have active communities and extensive documentation, ensuring strong support. - Do these frameworks work well with other JavaScript libraries?
Yes, both Tailwind CSS and Material-UI can be integrated with JavaScript libraries like React. - Can I customize the design of components in Tailwind CSS?
While Tailwind CSS offers customization, Material-UI provides more extensive theming capabilities. - Which framework is more suitable for large-scale applications?
Material-UI’s theming, component consistency, and customization options make it a strong choice for large projects.