When designing a website, you may want to display a limited amount of text initially, with an option to reveal more content. This functionality can be useful when you want to avoid clutter on your page while still giving users the option to see more details. A simple, lightweight way to achieve this is through CSS alone, without the need for JavaScript.
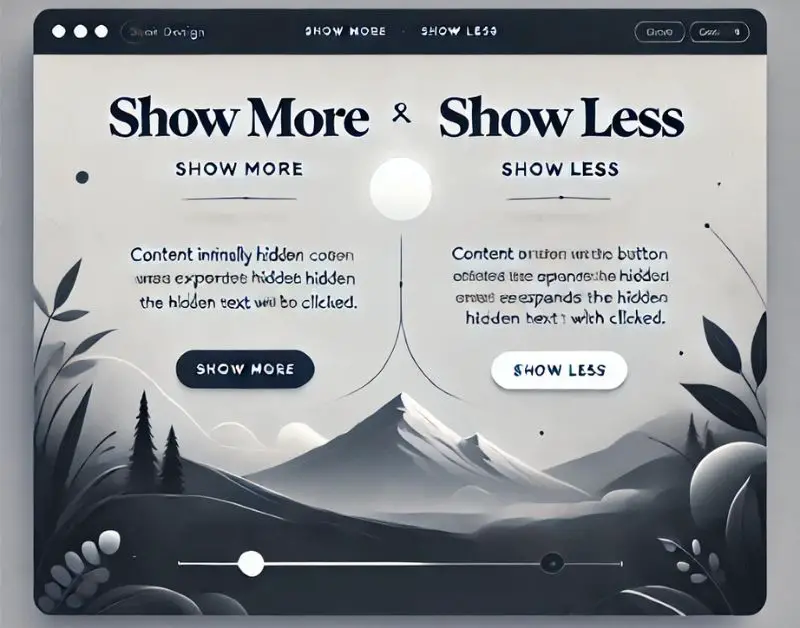
In this article, we will walk through how to create a “Show More” and “Show Less” feature using CSS.
Why Use CSS for Show More/Show Less?
Using CSS to implement a “Show More/Show Less” functionality is advantageous because:
- It doesn’t require any JavaScript.
- It’s lightweight and loads faster.
- It keeps your codebase simpler.
- It works well for static content that doesn’t change dynamically.
Let’s dive into the step-by-step guide.
Step-by-Step Implementation
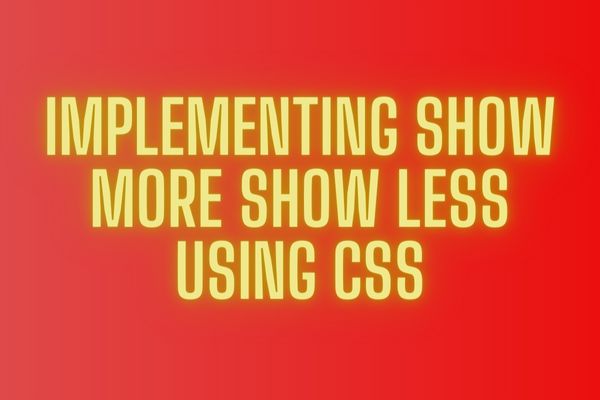
HTML Structure
We need to define the structure of the content that will be displayed or hidden. The content is placed inside a container, with some initially hidden and some visible.
<div class="content">
<input type="checkbox" id="toggle">
<p class="text">
This is the visible part of the text.
<span class="more">This part of the text will be shown when you click "Show More".</span>
</p>
<label for="toggle" class="show-more">Show More</label>
<label for="toggle" class="show-less">Show Less</label>
</div>
Explanation of HTML
<input type="checkbox">
: A hidden checkbox that controls the visibility of additional content. By default, it’s unchecked.<p class="text">
: The visible part of the content, and a hidden<span class="more">
element that will only be displayed when the checkbox is checked.<label>
: Two labels (Show More and Show Less) that act as buttons, linked to the checkbox using thefor
attribute.
CSS Styling
Now, let’s use CSS to manage the visibility of the extra content and style the labels as clickable buttons.
.content {
position: relative;
width: 300px;
font-family: Arial, sans-serif;
}
.text {
margin: 0;
}
.more {
display: none; /* Initially hide the extra content */
}
#toggle {
display: none; /* Hide the checkbox */
}
.show-more, .show-less {
display: inline-block;
color: blue;
cursor: pointer;
margin-top: 10px;
}
.show-less {
display: none; /* Initially hide the 'Show Less' button */
}
/* When the checkbox is checked, show the extra content and 'Show Less' button */
#toggle:checked ~ .text .more {
display: inline;
}
#toggle:checked ~ .show-more {
display: none; /* Hide the 'Show More' button */
}
#toggle:checked ~ .show-less {
display: inline; /* Show the 'Show Less' button */
}
Explanation of CSS
display: none
: Hides elements by default. We initially hide the.more
content, the checkbox, and the “Show Less” button.:checked
: A pseudo-class that targets the checkbox when it is checked. When the checkbox is checked, the.more
content becomes visible, the “Show More” label hides, and the “Show Less” label appears.
Final Output
By using the above CSS, you now have a simple “Show More/Show Less” functionality where users can click “Show More” to reveal hidden text, and “Show Less” to hide it again.
How It Works
- Initially, the
.more
content and the “Show Less” label are hidden. - When the checkbox is checked (when you click the “Show More” label), CSS rules come into play:
- The hidden content (
.more
) is revealed. - The “Show More” label is hidden, and the “Show Less” label becomes visible.
- When the checkbox is unchecked (when you click the “Show Less” label), everything returns to the initial state.
Check The output in Codepan
Conclusion
This method is simple and effective for static websites where JavaScript might be overkill. It can be especially useful for FAQ sections, blog summaries, or any place where you want to show only a portion of the content initially. By using CSS alone, you can enhance the user experience and keep the page lightweight.
If you’re looking to implement a dynamic version, you can pair this technique with JavaScript for more complex scenarios. However, for most basic use cases, this CSS approach will work perfectly.
Feel free to visit Makemychance.com for more CSS tutorials and web development tips!