Micro Frontends have become increasingly popular in recent years, as developers look for ways to break down monolithic applications into smaller, more manageable pieces. One popular approach is to use Angular, a powerful framework for building web applications. With Angular, it’s possible to create Micro Frontends that are easy to maintain, scalable, and highly modular.

In this article, we’ll explore an example of how to build a Micro Frontend with Angular 7. We’ll walk through the steps involved in creating a standalone Angular application, and then show how to integrate it with other Micro Frontends using Module Federation. We’ll also discuss some of the benefits and challenges of using this approach, and provide tips for overcoming common pitfalls. By the end of this article, you’ll have a solid understanding of how to build Micro Frontends with Angular 7, and be ready to start building your own modular, scalable web applications.
Understanding Micro Frontends

Micro Frontends is an architectural style that allows teams to work independently on different parts of a web application using different technologies and frameworks. It is an extension of the microservices architecture pattern, but applied to the frontend layer of a web application.
In a traditional monolithic web application, all the frontend code is bundled together and deployed as a single unit. This makes it difficult to scale and maintain as the application grows in size and complexity. With Micro Frontends, the frontend code is split into smaller, independent modules, each with its own codebase, build process, and deployment pipeline.
This approach allows teams to work independently on different parts of the application, using the technologies and frameworks that best suit their needs. For example, one team might use Angular to build a dashboard, while another team uses React to build a shopping cart. Each team can work independently without worrying about the other teams’ code or dependencies.
Micro Frontends also allows for more flexibility in deployment. Instead of deploying the entire application at once, each module can be deployed independently. This means that updates and changes can be made to specific parts of the application without affecting the rest of the application.
Overall, Micro Frontends is a powerful approach to building web applications that allows teams to work independently and deploy more flexibly. It is a great way to scale and maintain complex applications while also allowing for more innovation and experimentation.
Overview of Angular 7
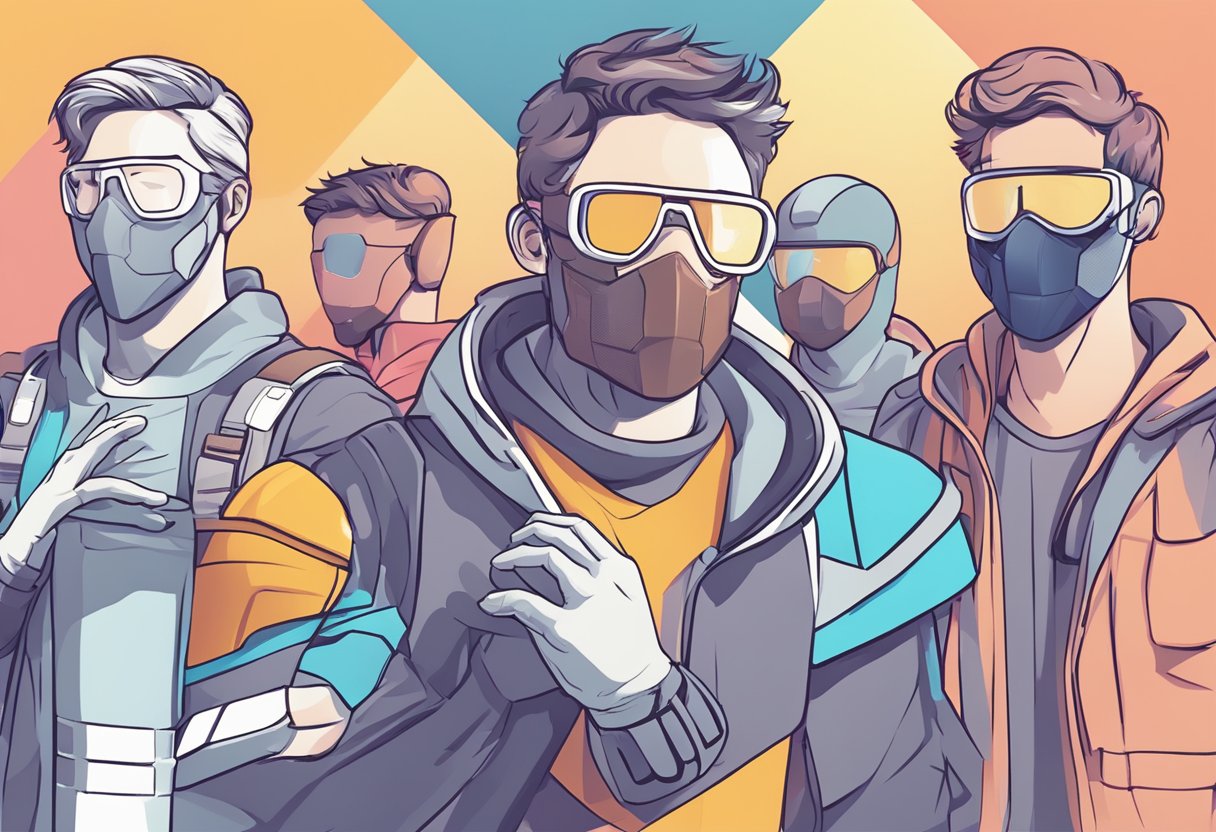
Angular 7 is a popular open-source web application framework developed by Google. It is a complete rewrite of AngularJS and was released in October 2018. Angular 7 is known for providing a robust and scalable framework for building complex web applications.
One of the key features of Angular 7 is its ability to create reusable components that can be used across multiple projects. These components are designed to be modular and can be easily integrated into any project. This makes it easier to maintain and update applications, as well as reducing development time.
Angular 7 also includes features such as improved performance, enhanced accessibility, and improved error handling. It also includes support for the latest versions of TypeScript, RxJS, and Node.js.
Another important feature of Angular 7 is its support for micro frontends. Micro frontends are small, independent components that can be built and deployed separately from the main application. This allows for greater flexibility and scalability, as well as easier maintenance and updates.
In summary, Angular 7 is a powerful and flexible web application framework that provides a wide range of features and capabilities for building complex web applications. Its support for micro frontends makes it an ideal choice for building modular and scalable applications.
Setting Up Angular 7 Environment
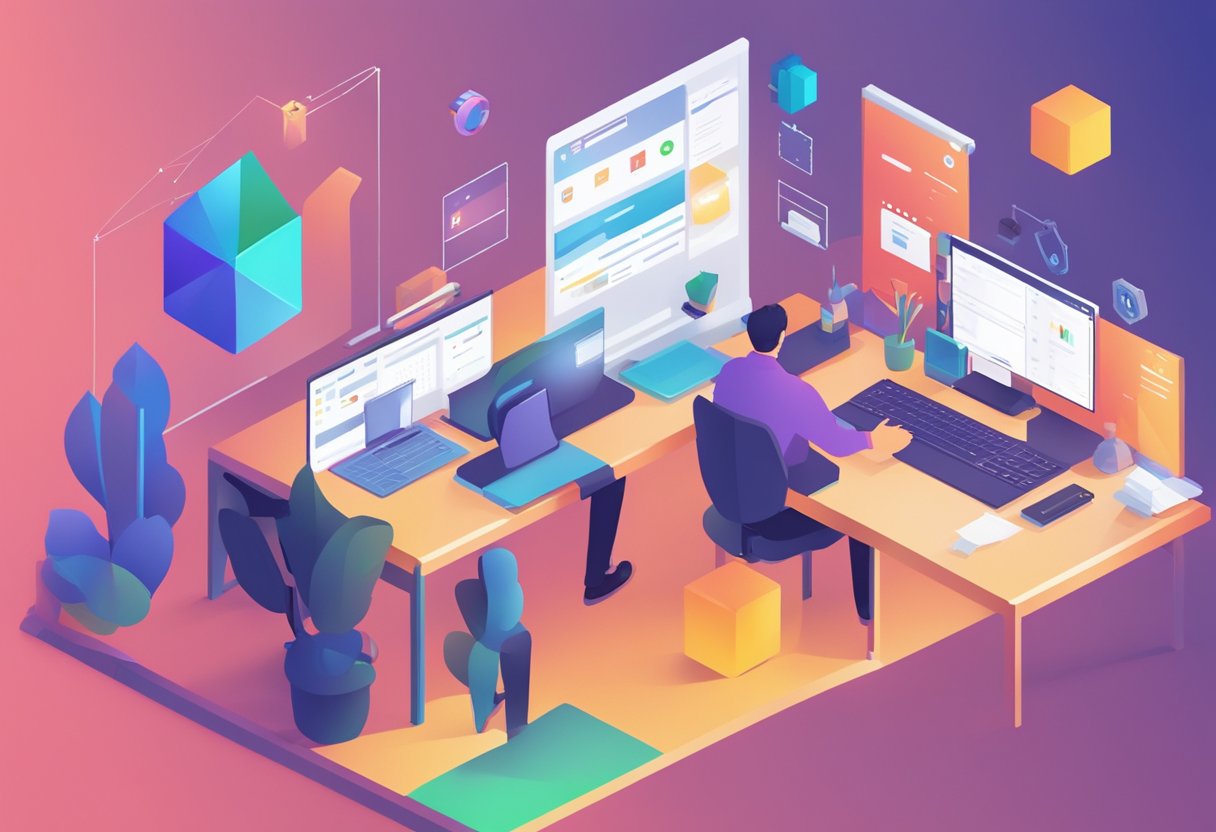
To create a Micro Frontend Angular 7 example, we need to set up our development environment. Here are the steps to set up the Angular 7 environment:
- Install Node.js and npm: Angular 7 requires Node.js version 10.9.0 or later and npm version 6.4.1 or later. You can download Node.js and npm from their official website.
- Install Angular CLI: Angular CLI is a command-line interface tool that helps us to create, manage, and build Angular applications. To install Angular CLI, open a command prompt or terminal and run the following command:
npm install -g @angular/cli
- Create a new Angular project: Once Angular CLI is installed, we can create a new Angular project by running the following command:
ng new my-micro-frontend-app
- Add a new Micro Frontend: To add a new Micro Frontend to our Angular project, we need to create a new Angular application. We can create a new application by running the following command:
ng generate application my-micro-frontend
This command will create a new Angular application inside theprojects
folder of our main Angular project. - Install dependencies: To use Micro Frontend architecture, we need to install some dependencies. We can install them by running the following command inside our newly created Micro Frontend application:
npm install --save-dev webpack webpack-cli @module-federation/client
This command will install Webpack and the Module Federation client library.
That’s it! We have set up our Angular 7 environment to create a Micro Frontend example. In the next section, we will create our Micro Frontend application.
Creating a Micro Frontend with Angular 7
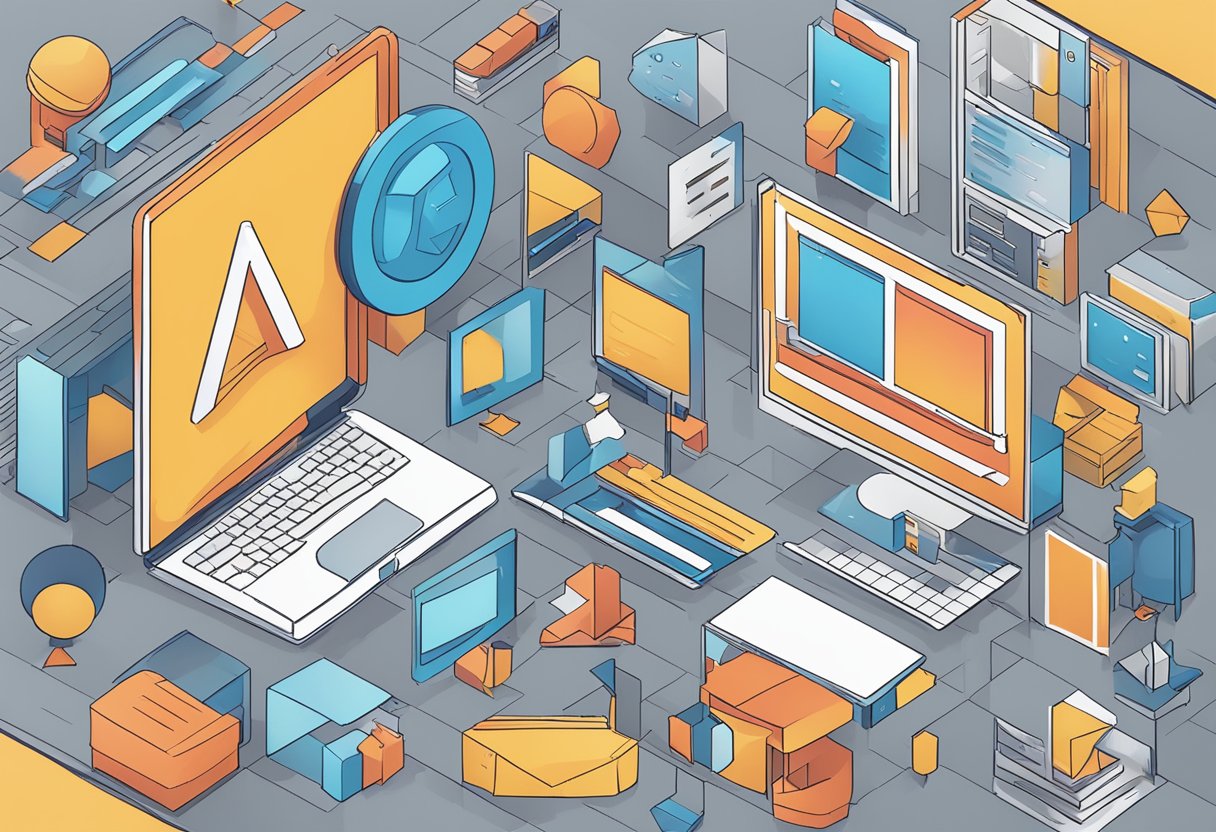
To create a micro frontend with Angular 7, we need to follow a few steps. In this section, we will describe the necessary steps to create a micro frontend with Angular 7.
Project Initialization
First, we need to create a new Angular project. We can use the Angular CLI to create a new project. To create a new project, we can run the following command:
ng new my-micro-frontend-app
This command will create a new Angular project with the name my-micro-frontend-app
.
Component Creation
Next, we need to create a new component for our micro frontend. We can use the Angular CLI to create a new component. To create a new component, we can run the following command:
ng generate component my-micro-frontend-component
This command will create a new component with the name my-micro-frontend-component
.
Routing Configuration
Finally, we need to configure the routing for our micro frontend. We can use the Angular Router to configure the routing. To configure the routing, we need to create a new module for our micro frontend. We can use the Angular CLI to create a new module. To create a new module, we can run the following command:
ng generate module my-micro-frontend-module --routing
This command will create a new module with the name my-micro-frontend-module
and also create a routing file for the module.
In the routing file, we can define the routes for our micro frontend. For example, we can define a route for our my-micro-frontend-component
component as follows:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { MyMicroFrontendComponent } from './my-micro-frontend.component';
const routes: Routes = [
{ path: '', component: MyMicroFrontendComponent }
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class MyMicroFrontendRoutingModule { }
In this way, we can create a micro frontend with Angular 7.
Integrating Micro Frontends

Integrating Micro Frontends can be a challenging task, but with Angular 7, we can make it much easier. There are a few steps that we need to follow to integrate Micro Frontends into our Angular application.
First, we need to create a new Angular project using the Angular CLI. Once we have our project set up, we can start building our Micro Frontends. We can use different frameworks and technologies to build our Micro Frontends, such as React, Vue, or even plain HTML, CSS, and JavaScript.
Next, we need to use Module Federation to integrate our Micro Frontends into our Angular application. Module Federation is a new feature introduced in Webpack 5 that allows us to share code between different applications. With Module Federation, we can dynamically load our Micro Frontends into our Angular application without the need for any additional build steps.
To use Module Federation, we need to install the necessary packages and configure our Webpack configuration file. We also need to define the Micro Frontends that we want to load into our Angular application and their corresponding entry points.
Once we have our Micro Frontends loaded into our Angular application, we need to define how they will communicate with each other. We can use different communication patterns, such as Pub/Sub or Request/Response, to enable communication between our Micro Frontends.
Overall, integrating Micro Frontends into our Angular application requires careful planning and execution. With the right tools and techniques, we can create a modular and scalable application that can adapt to changing requirements and technologies.
Testing the Micro Frontend

Once we have built our micro frontend using Angular 7, it’s important to test it to ensure that it works as expected. In this section, we will cover some of the testing strategies that we can use.
Unit Testing
Unit testing is an essential part of any software development project, and micro frontends are no exception. We can use the Angular testing framework to write unit tests for our micro frontend components and services. These tests can help us catch bugs early in the development process and ensure that our code is working as expected.
Integration Testing
Integration testing involves testing the interaction between different components of our micro frontend. We can use tools like Protractor to write integration tests for our micro frontend. These tests can help us ensure that our micro frontend is working as expected when it is integrated with other micro frontends or with the main application.
End-to-End Testing
End-to-end testing involves testing the entire micro frontend as a whole, including its interaction with other micro frontends and the main application. We can use tools like Cypress to write end-to-end tests for our micro frontend. These tests can help us ensure that our micro frontend is working as expected in a real-world scenario.
Continuous Integration and Deployment
To ensure that our micro frontend is always working as expected, we should set up a continuous integration and deployment (CI/CD) pipeline. This pipeline can automatically run our unit, integration, and end-to-end tests and deploy our micro frontend to production when all tests pass.
In conclusion, testing is an essential part of building a micro frontend using Angular 7. By using the testing strategies outlined in this section, we can ensure that our micro frontend is working as expected and catch bugs early in the development process.
Deployment and Scaling
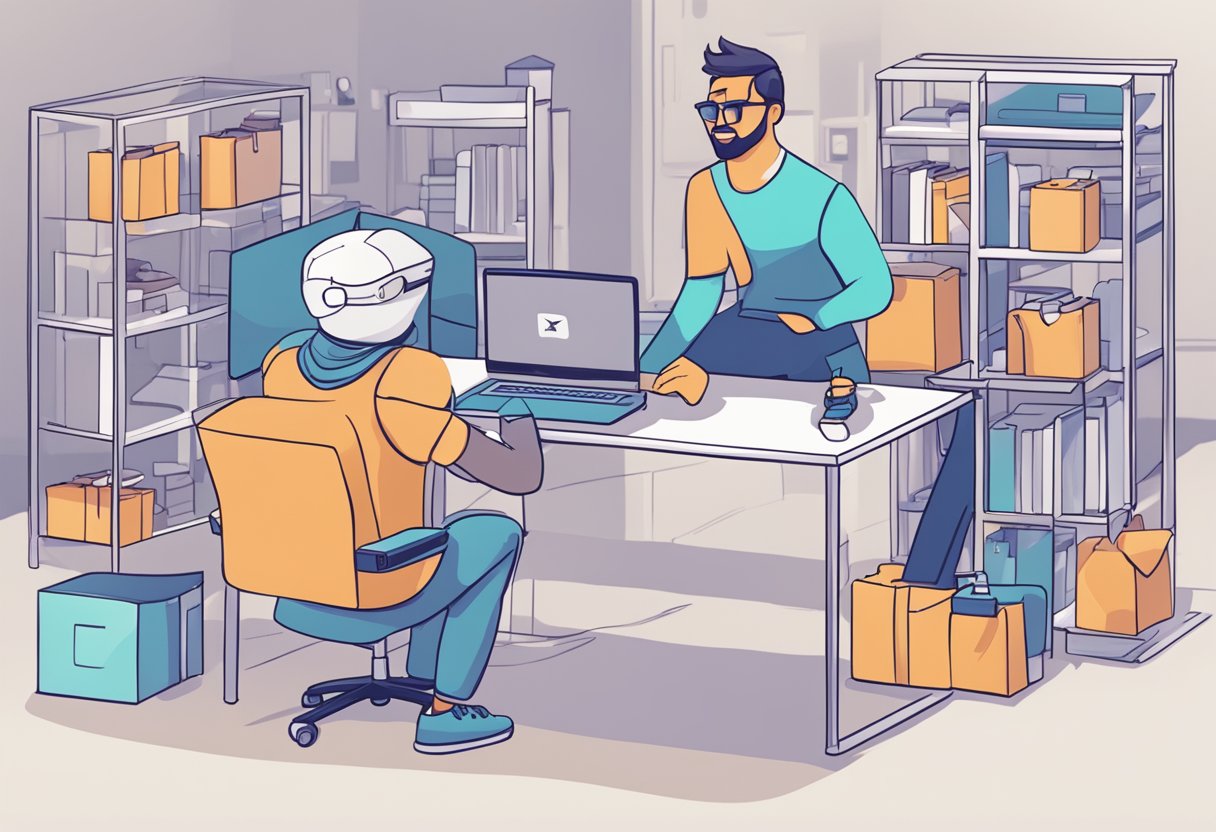
When it comes to deploying and scaling micro frontends with Angular, there are a few factors to consider. Here are some best practices that we’ve found helpful:
1. Containerization
One way to deploy micro frontends is to use containerization. This involves packaging the application and its dependencies into a container that can be easily moved between environments. Docker is a popular containerization tool that can be used to deploy micro frontends.
2. Load Balancing
Load balancing is another important consideration for scaling micro frontends. By distributing incoming traffic across multiple servers, load balancing can help ensure that the application can handle increased traffic and remain responsive. Tools like Kubernetes can be used to manage load balancing for micro frontends.
3. Continuous Integration and Deployment
To ensure that changes to micro frontends are deployed quickly and reliably, it’s important to establish a continuous integration and deployment (CI/CD) pipeline. This involves automating the build, testing, and deployment processes so that changes can be quickly and easily pushed to production.
4. Monitoring and Logging
Finally, it’s important to have a robust monitoring and logging strategy in place to ensure that issues can be quickly identified and resolved. This can involve using tools like Prometheus for monitoring and ELK stack for logging.
By following these best practices, we can ensure that our micro frontends are deployed and scaled in a way that is reliable, efficient, and scalable.
Best Practices and Challenges

Micro Frontends with Angular 7 can be a great way to deliver a modular and scalable application. However, there are some best practices and challenges that we need to consider before implementing micro frontends.
Performance Optimization
One of the main concerns when implementing micro frontends is performance. Each micro frontend is a separate application, and we need to ensure that they don’t negatively impact the overall performance of the application.
To optimize performance, we can use lazy loading to only load the micro frontend when it’s needed. We can also use server-side rendering to improve the initial load time of the application. Additionally, we can use code splitting to reduce the size of the bundles and improve the overall performance.
Security Considerations
Another challenge when implementing micro frontends is security. Each micro frontend is a separate application, and we need to ensure that they don’t introduce any security vulnerabilities.
To ensure security, we need to use proper authentication and authorization mechanisms. We also need to ensure that each micro frontend is isolated from the others to prevent any unauthorized access. Additionally, we need to ensure that each micro frontend is properly tested for security vulnerabilities.
Overall, implementing micro frontends with Angular 7 can be a great way to deliver a modular and scalable application. However, we need to ensure that we follow best practices and consider the challenges, such as performance optimization and security considerations, to ensure that our application is secure and performs well.
Frequently Asked Questions

How can Angular Module Federation be used with Micro Frontends?
Angular Module Federation can be used with Micro Frontends to enable dynamic loading of Micro Frontend components at runtime. This allows for greater flexibility in development and deployment, as well as improved performance and scalability. To learn more about using Angular Module Federation with Micro Frontends, check out this article by Okta.
What is the best course for learning about Micro Frontends with Angular?
There are several great courses available for learning about Micro Frontends with Angular. One highly recommended course is Micro Frontends with Angular on Udemy. This course covers everything from the basics of Micro Frontends to advanced techniques for building scalable and maintainable applications.
Can you provide an example of Micro Frontends with Angular using Module Federation?
Sure! One example of Micro Frontends with Angular using Module Federation can be found in this GitHub repository. This repository includes a sample application that demonstrates how to use Angular Module Federation to dynamically load Micro Frontend components at runtime.
What is the recommended framework for building Micro Frontends with Angular?
Angular is the recommended framework for building Micro Frontends with Angular. Angular provides a powerful set of tools and features for building scalable and maintainable applications, making it an ideal choice for building Micro Frontends. To learn more about building Micro Frontends with Angular, check out this article by Angular Architects.
Which Udemy course is best for learning about Micro Frontends with Angular?
One highly recommended Udemy course for learning about Micro Frontends with Angular is Micro Frontends with Angular. This course covers everything from the basics of Micro Frontends to advanced techniques for building scalable and maintainable applications.
How does single-spa work with Micro Frontends in Angular?
Single-spa is a framework for building Micro Frontends that works well with Angular. Single-spa provides a set of APIs for loading and managing Micro Frontends, making it easy to build complex applications with multiple Micro Frontends. To learn more about using Single-spa with Micro Frontends in Angular, check out this article by Bit.
ReactJS Interview Questions: A Comprehensive Guide for Success