In the realm of interactive web design, CSS Transitions and React have emerged as crucial elements in creating powerful, user-focused applications. The fusion of both can significantly enhance a user’s interface experience, further driving engagement and prolonging on-site time. This understanding becomes more impactful when we dive deep into CSS Transitions, a technology that allows web designers to create smooth and adaptive visual effects. Through judicious use of these innovative techniques, we can create fluid, visually stunning, and highly responsive web experiences. This journey doesn’t stop at simply understanding CSS Transitions; there’s an exhilarating exploration of implementing these capabilities within a React-based application, providing answers to how to supercharge an application’s interactivity.
Understanding CSS Transitions
Drawn in by the multiplicity of the most innovative tech trends? If so, technology enthusiasts, we are here to elucidate CSS Transitions – a splendid tool for enhancing web interactivity and dynamism. Without further ado, here’s how you can leverage the influential capabilities of CSS transitions for a website’s user interface.
To begin, it’s a no-brainer that an interactive website engages more users. Therefore, CSS, short for Cascading Style Sheets, has transcended from styling HTML documents to enabling smooth, elegant transitions. CSS transitions breathe life into static interfaces, setting up an interpolation between two stages – an initial state and an altered state.
In technical terms, CSS transitions are the gradual evolvement from one style configuration to another over time. A transition occurs when an element’s property alters in any way, shape, or form. The result? Increased website interactivity.
A closer look at the CSS transition property involves four sub-properties: property, duration, timing function, and delay.
- The
property
defines what attribute of an element will change. For example, you may choose to change the opacity of a link or button when hovered over. - The
duration
is the length of time the transition will take from start to end, usually implemented in seconds or milliseconds. A longer duration will slow the change, while a shorter one will speed it up. - The
timing function
determines the speed of the transition effect over the duration. This can be set as linear for a consistent pace, ease for an accelerating-then-decelerating pace, or other pre-set options, all serving to transform the user experience. - Last but no less crucial,
the delay
property specifies when the transition effect will begin.
These properties are used in conjunction with CSS pseudo-classes to control when the transitions occur.
Here is a sample usage of CSS Transition:
div {
width: 100px;
height: 100px;
transition: width 2s;
}
div:hover {
width: 200px;
}
In this example, the div
element will smoothly change its width over a span of 2 seconds when hovered over.
In essence, what makes CSS transitions a boon for tech-lovers is their ability to infuse charisma into common website elements, upgrading them from being simply functional to also being visually engaging. They boast the potential to make your website more effective, impressive, and user-friendly, an inevitable formula to boost user engagement and retention. So, for those striving for excellence in web design, one cannot afford to overlook the influence of CSS transitions in enhancing web interactivity.
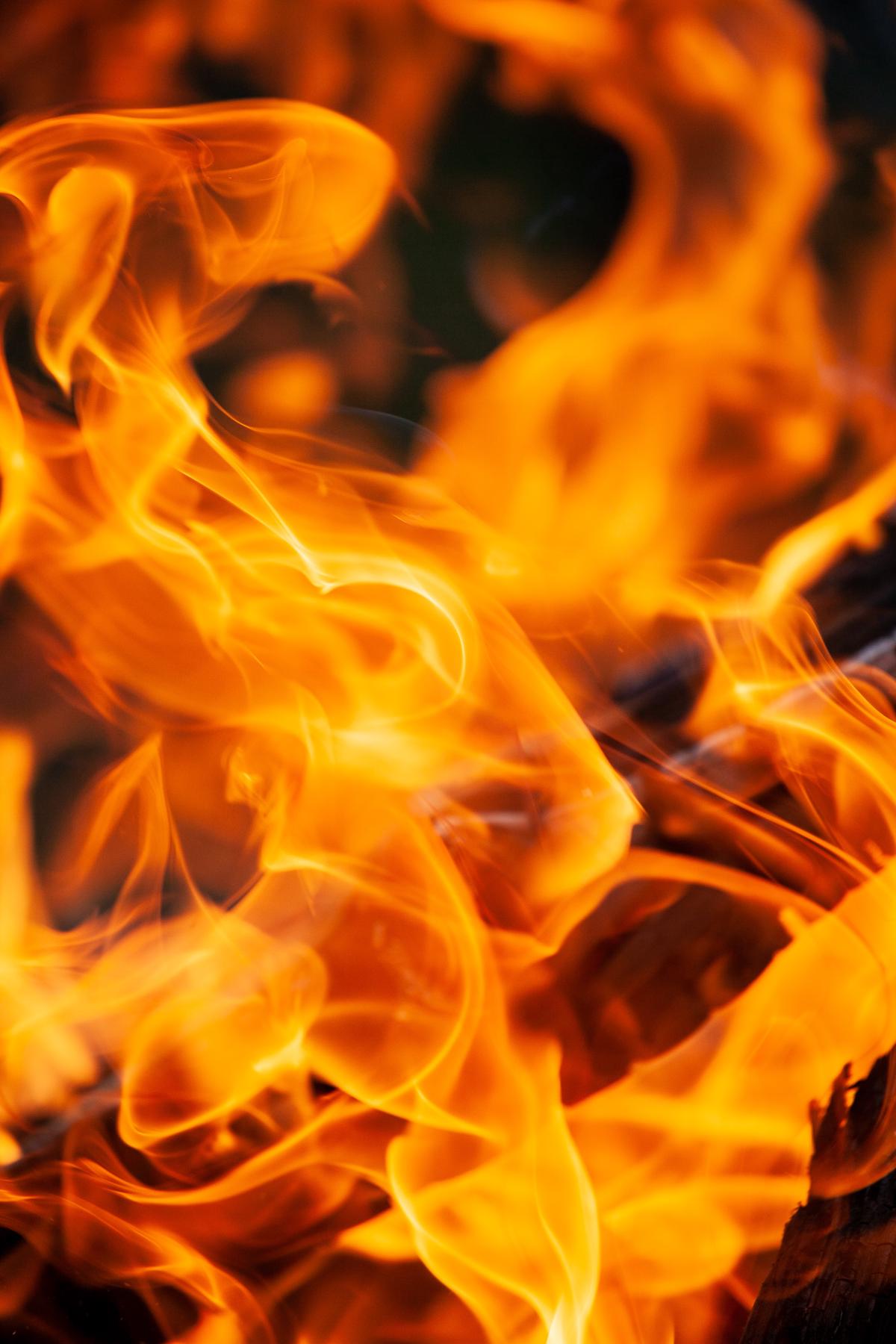
Photo by maxim_tajer on Unsplash
Implementing CSS Transitions with React
In your web development journey, you may find yourself asking, how can I effectively integrate CSS transitions into my React applications? React, while powerful in managing state and handling data, doesn’t directly address CSS transitions. But that doesn’t mean it cannot be done. Let’s directly delve into integrating these technologies.
First off, let’s clarify one thing: React itself does not inherently support CSS transitions. We have to reach for additional libraries that simplify this integration. One exceptionally pleasant library to work with is ‘react-transition-group’. This library is built and maintained by the team at React, ensuring seamless integration and up-to-date features.
Firstly, install this package into your project by running npm install react-transition-group
in your project terminal. This will add the necessary codebase into your project, allowing you to access the powerful transition properties provided by this library.
Once installed, you have access to four main components, but for the purpose of this tutorial, our focus is on the CSSTransition
component.
To include a CSS transition in your React application, you’ll need to first import the CSSTransition
component from ‘react-transition-group’. For example, import { CSSTransition } from 'react-transition-group';
at the top of your file.
Following this, you can then utilize the CSSTransition
component in your render method.
Here’s a brief example of how that might look with a simple fadeIn and fadeOut effect on a modal:
<import CSSTransition from 'react-transition-group';>
function Example() {
const [inProp, setInProp] = useState(false);
return (
<div>
<CSSTransition in={inProp} timeout={200} classNames="my-node">
<div>
{"I'll fade in and out when you click the button!"}
</div>
</CSSTransition>
<button type="button" onClick={() => setInProp(!inProp)}>
Click to Enter
</button>
</div>
);
}
And the corresponding CSS for the transition would look like this:
.my-node-enter {
opacity: 0;
}
.my-node-enter-active {
opacity: 1;
transition: opacity 200ms;
}
.my-node-exit {
opacity: 1;
}
.my-node-exit-active {
opacity: 0;
transition: opacity 200ms;
}
The ‘classNames’ prop helps link your React component with corresponding CSS styles that control the transition. The ‘enter’ and ‘exit’ classes along with their ‘-active’ counterparts allow you to control the entering and exiting states of your components, creating seamless transitions.
But remember, managing transitions in React necessitates strategic handling of state. The “in” prop here controls whether the component should be in the entering or exiting state, letting you control your component’s visibility with React’s state and lifecycle methods.
Implementing CSS transitions in React can give you the best of both worlds: the robust state management of React and the smooth, visual transitions of CSS. So why delay? Dive straight into the world where CSS transitions meet React and give your application that smooth interactivity it deserves.
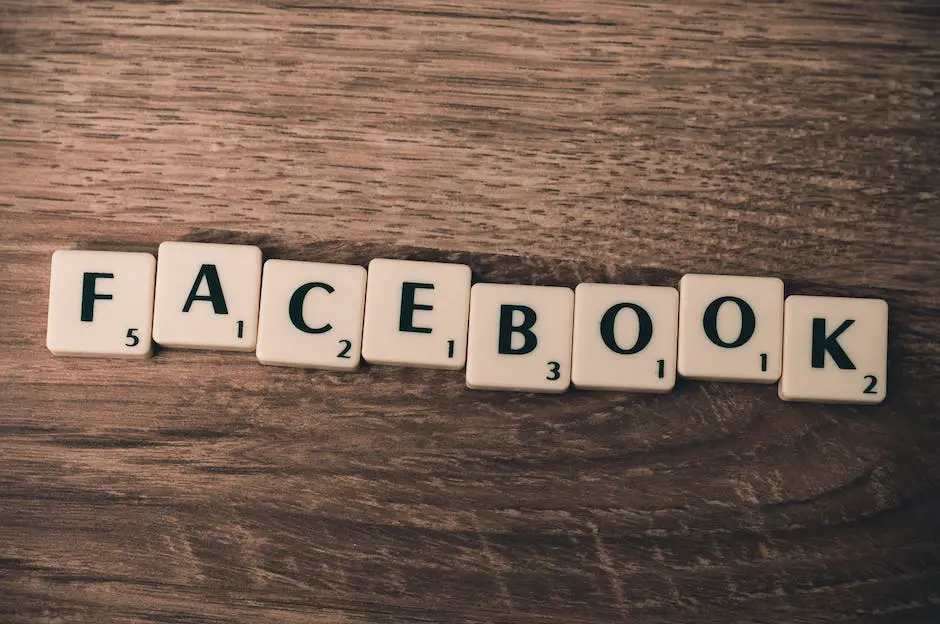
Troubleshooting CSS Transitions in React
Despite the impressive benefits bestowed upon web design and user engagement by CSS transitions, it is not quite a walk in the park when it comes to integrating them with React. We know React does not directly support CSS transitions, necessitating the use of third-party libraries like the popular ‘react-transition-group’. This additional task casts a few potential hurdles on our path. In adoption of the tech enthusiast spirit we are armed with, let’s uncover common problems that can occur when using CSS transitions in React and delve into the pragmatic solutions for them.
First and foremost, performance issues can be a nuisance. High-complexity animations might lead to reduced performance in the React application, particularly in less powerful devices or browsers with subpar GPU acceleration. Enter ‘will-change’, a CSS property that informs the browser that an element will animate in the future. By using this property, we can help the browser prepare and optimize for the transition, essentially elevating the application’s performance during intense animations.
Next up, managing transition entry and exit states can become cumbersome in large scale applications. Using the ‘CSSTransition’ component from the ‘react-transition-group’ library, we can couple the CSS transitions with the component’s lifecycle. However, this coupling might become hard to manage as the app grows. The solution is to break down the transitions into smaller, manageable components, thereby encapsulating their state and behavior. This way, we can maintain a clean and modular codebase that is easier to reason about.
Unpredictable rendering order is another issue. Since React’s rendering behavior is optimized for performance and not predictability, it sometimes leads to unexpected transition behavior. To counter this, we could use the ‘TransitionGroup’ component, which manages a set of ‘CSSTransition’ or ‘Transition’ components in a list. With ‘TransitionGroup’, any ‘CSSTransition’ or ‘Transition’ child elements and their order can be controlled deterministically.
Finally, back and forth communication with designers about pixel-perfect details can be time-consuming and difficult to implement with code alone. The ‘react-spring’ library plays the hero in this situation. The library allows us to control the animation’s physics properties, translating designer’s needs into useable code, by leveraging the power of physics-based animations.
To conclude, the integration of CSS transitions in React may pose challenges; but with deep understanding of the involved hurdles and utilizing the right tools, we can tackle them effectively and efficiently. Striving for seamless animations and transitions not only enhances the interactive experience but also marks an applaudable testament to our problem-solving abilities as tech aficionados. Diving into the tech universe with vigor, let’s continue to solve problems, automate, analyze, and innovate. Because, isn’t that what being a tech-enthusiast is all about?
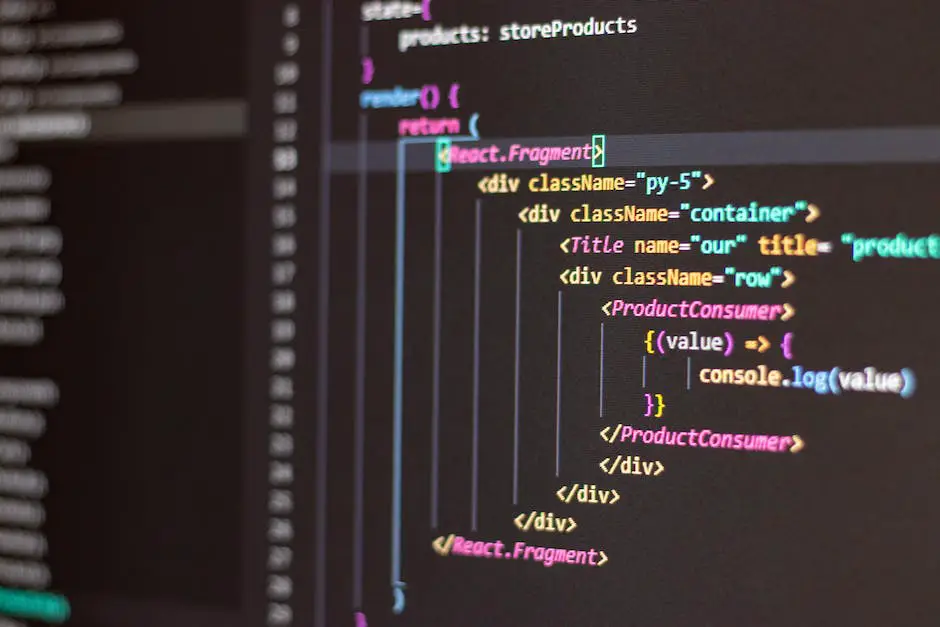
The world of CSS Transitions in React is extensive and occasionally daunting, but with the right information and guidance, troubleshooting potential issues becomes less intimidating. Overcoming challenges in a React environment and debugging them effectively might seem like navigating uncharted territories. However, equipped with the implicit knowledge shared here, each stumbling block can turn into a stepping stone towards mastery. Thus, a thorough understanding of CSS Transitions, coupled with the ability to implement them efficiently and tackle potential issues in a React _environment, can spell the difference between a good and a great web development professional.