In the world of programming, data structures play an important role in managing and manipulating data effectively. One such dynamic and widely used data structure in Java is the ArrayList. This article explores the intricacies of Java ArrayList, from its fundamentals to its practical applications.
What is an ArrayList in Java?
An ArrayList is a part of the Java Collections Framework, which extends the capabilities of arrays. Unlike arrays, ArrayLists can dynamically grow in size, making them highly flexible. They belong to the java.util
package and provide numerous methods for data manipulation.
Benefits of Using ArrayList
ArrayLists offer several advantages:
- Dynamic Sizing: They can expand or shrink as needed.
- Versatility: Storing objects of any type is possible.
- Easy Retrieval: Elements can be accessed using their index.
- Powerful Methods: Methods like
add
,remove
, andsort
simplify operations.
Declaring and Initializing an ArrayList
To create an ArrayList, you need to declare it and then initialize it. Here’s a basic example:
import java.util.ArrayList;
ArrayList<String> names = new ArrayList<>();
This creates an ArrayList named names
to store strings.
Adding Elements to an ArrayList
You can add elements to an ArrayList using the add
method. For instance:
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Removing Elements from an ArrayList
Removing elements is as straightforward as adding them. The remove
method helps with that.
names.remove("Bob");
Accessing Elements in an ArrayList
To retrieve elements, you can use their index.
String firstPerson = names.get(0); // Retrieves "Alice"
ArrayList Size and Capacity
The size of an ArrayList represents the number of elements currently stored, while the capacity is the total number of elements it can hold before resizing.
Iterating Through an ArrayList
Iterating through an ArrayList is essential for various operations. The enhanced for-loop simplifies this task.
for (String name : names) {
System.out.println(name);
}
Sorting an ArrayList
To sort the elements in an ArrayList, you can use the Collections.sort
method.
import java.util.Collections;
Collections.sort(names);
Common Operations with ArrayList
Other common operations include checking if an ArrayList is empty, clearing all elements, determining its size, and ensuring it is imported into the code.
boolean isEmpty = names.isEmpty();
names.clear();
int listSize = names.size();
ArrayList vs. Arrays
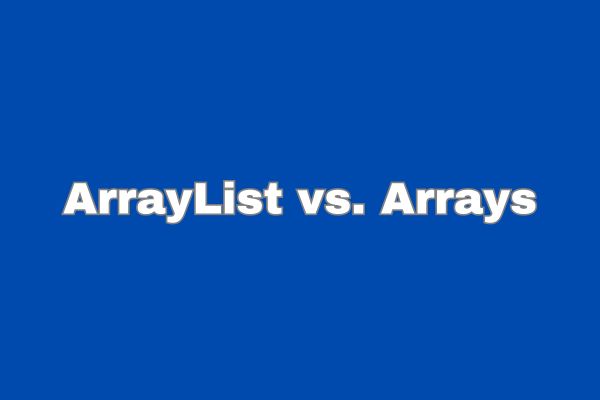
ArrayLists offer more flexibility and functionality compared to traditional arrays. However, they consume more memory.
Best Practices for Using ArrayList
To make the most of ArrayLists, follow these best practices:
- Specify the data type.
- Use the diamond operator for type inference.
- Consider capacity when adding elements in bulk.
- Use enhanced for-loops for iteration.
Java ArrayList Use Cases
ArrayLists are versatile and can be used in various scenarios, such as maintaining lists of user information, managing inventory items, and more.
Conclusion
In the world of Java programming, the ArrayList is a valuable tool that simplifies data management. With dynamic sizing, powerful methods, and extensive use cases, it’s a data structure that every Java developer should be well-versed in.
FAQs
- What is the difference between ArrayList and arrays in Java?
ArrayLists are dynamic, can store objects of any type, and provide extensive methods for data manipulation, whereas arrays have a fixed size and are limited in functionality. - How do I add elements to an ArrayList?
You can use theadd
method to add elements to an ArrayList. - Can I sort an ArrayList in Java?
Yes, you can use theCollections.sort
method to sort the elements in an ArrayList. - What are some best practices for using ArrayList in Java?
It’s advisable to specify the data type, use the diamond operator, consider capacity when adding elements in bulk, and use enhanced for-loops for iteration. - What are some common use cases for ArrayList in Java?
ArrayLists are used in various scenarios, such as managing lists of user information, maintaining inventory items, and more.