In modern web development, animations play a crucial role in enhancing user experience by adding an interactive feel to web pages. While CSS offers basic animation capabilities, there’s often a need for more complex, physics-based effects that mimic natural movements, such as bouncing, elasticity, and smooth transitions. Enter CSS Spring, an NPM package that leverages spring physics to deliver smooth, lifelike animations with ease.
This article will dive into what CSS Spring is, how it works, and how to implement it in your projects.
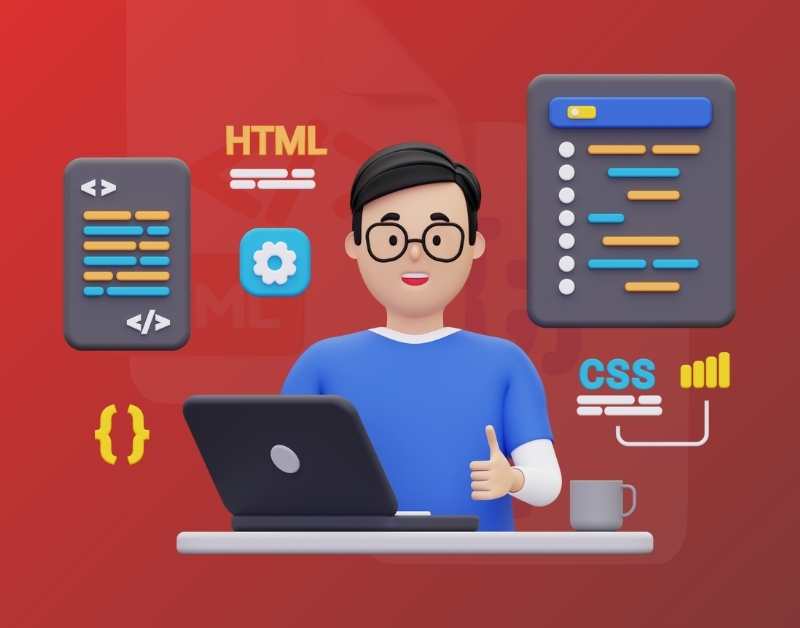
What is CSS Spring?
CSS Spring is a lightweight NPM package that simplifies the process of creating spring-based animations in your web projects. Instead of manually configuring multiple keyframes or using JavaScript libraries to simulate physics, CSS Spring provides an intuitive and clean API to create spring animations with minimal effort.
Springs are physics-based models used in animations to simulate natural motion, giving elements more dynamic and organic movements. For example, when you click a button, it can “spring” back into place, offering a smoother, more natural interaction compared to simple CSS animations.
Why Use CSS Spring?
- Smooth, natural animations: CSS Spring animations mimic real-world physics, creating more engaging, natural-feeling interactions.
- Lightweight: It’s designed to be lightweight and easy to integrate, making it an excellent choice for performance-conscious developers.
- Flexible: With minimal configuration, you can achieve advanced animations like bouncing, overshooting, and elastic effects.
- Customizable: CSS Spring allows you to tweak the spring parameters to achieve the desired level of tension, friction, and mass, offering fine control over your animations.
Getting Started with CSS Spring
Let’s go through the process of setting up and using CSS Spring in a project.
Step 1: Installation
First, install the CSS Spring package via NPM:
npm install css-spring
Alternatively, if you’re using Yarn:
yarn add css-spring
Step 2: Importing CSS Spring
Once installed, you can import CSS Spring into your JavaScript or TypeScript file:
import { spring } from 'css-spring';
Step 3: Creating Your First Spring Animation
To demonstrate how CSS Spring works, let’s animate a simple box that springs into place when clicked.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Spring Example</title>
<style>
#box {
width: 100px;
height: 100px;
background-color: coral;
margin-top: 100px;
}
</style>
</head>
<body>
<div id="box"></div>
<script type="module">
import { spring } from 'css-spring';
const box = document.getElementById('box');
box.addEventListener('click', () => {
// Define the spring animation
spring(box, {
from: { transform: 'translateY(0px)' },
to: { transform: 'translateY(-150px)' },
tension: 170, // Controls stiffness of the spring
friction: 26, // Controls damping (smoothness)
mass: 1, // Controls the weight of the spring
onUpdate: (props) => {
box.style.transform = props.transform;
},
onComplete: () => {
console.log('Animation completed!');
}
});
});
</script>
</body>
</html>
In the example above:
- We use the
spring()
function to animate thebox
element. - The animation makes the box “spring” upwards by changing its
translateY
property. - Parameters like
tension
,friction
, andmass
control the spring physics, allowing for fine-tuning the animation’s stiffness and smoothness.
Step 4: Customizing Spring Animations
You can further customize your animations by adjusting the spring parameters. Here’s a breakdown of the key parameters:
tension
: The stiffness of the spring. Higher values make the animation faster and snappier.friction
: Controls how much resistance the spring faces as it moves. Higher friction values make the animation slower and more dampened.mass
: The weight of the animated element. Increasing the mass makes the animation feel heavier, slowing down its movement.onUpdate
: A callback that updates the properties of the element during the animation.onComplete
: A callback that triggers when the animation finishes.
Example: Elastic Button Animation
To see another example, let’s create a button that springs back to its original position after being clicked:
<button id="springButton">Click Me!</button>
<script type="module">
import { spring } from 'css-spring';
const button = document.getElementById('springButton');
button.addEventListener('click', () => {
spring(button, {
from: { transform: 'scale(1)' },
to: { transform: 'scale(1.3)' },
tension: 150,
friction: 30,
mass: 1,
onUpdate: (props) => {
button.style.transform = props.transform;
}
});
});
</script>
This code snippet animates the button to grow slightly larger when clicked and then “springs” back to its original size.
Practical Use Cases of CSS Spring
- Interactive UI Elements: Create buttons, toggles, and sliders that respond to user actions with spring-like movements.
- Modal Animations: Instead of having modals just pop up, you can use CSS Spring to have them slide in naturally, improving the overall user experience.
- Card Hover Effects: Use spring animations to create more engaging hover effects for cards or interactive elements.
- Page Transitions: Implement smooth page transitions that feel responsive and lively using spring-based motion.
Conclusion
CSS Spring offers an easy-to-use solution for developers looking to create sophisticated, physics-based animations in their web projects. Its lightweight nature and simplicity make it an attractive choice for anyone aiming to enhance their website’s interactivity and appeal.
By leveraging spring physics, you can create animations that feel natural and intuitive, offering a more engaging user experience. Whether you’re animating a simple button or creating complex UI transitions, CSS Spring has you covered.
Reference
For more details and advanced usage of CSS Spring, visit the official NPM package page here.