Java Streams for JSON Filtering
Java 8’s Stream API simplifies filtering JSON documents. Here’s an example using a javax.json.JsonObject
:
private JsonObject jsonObject = loadJsonObject();
public List filterJsonArrayToList() {
List topics = jsonObject.getJsonArray("topics").stream()
.filter(jsonValue -> ((JsonString) jsonValue).getString().startsWith("M"))
.map(jsonValue -> ((JsonString) jsonValue).getString())
.collect(Collectors.toList());
return topics;
}
This returns a list containing “Math” and “Music”. For a JsonArray
:
public JsonArray filterJsonArrayToJsonArray() {
JsonArray topics = jsonObject.getJsonArray("topics").stream()
.filter(jsonValue -> ((JsonString) jsonValue).getString().startsWith("C"))
.collect(JsonCollectors.toJsonArray());
return topics;
}
Jackson’s JsonNode
offers an alternative approach:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class Main {
public static void main(String[] args) throws Exception {
String jsonString = "[{"name": "John", "age": 30}, {"name": "Jane", "age": 25}, {"name": "Bob", "age": 35}]";
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
Stream stream = StreamSupport.stream(jsonNode.spliterator(), false);
Stream filteredStream = stream.filter(node -> node.get("name").asText().equals("John"));
JsonNode filteredJsonNode = objectMapper.valueToTree(filteredStream.collect(Collectors.toList()));
String filteredJsonString = objectMapper.writeValueAsString(filteredJsonNode);
System.out.println(filteredJsonString);
}
}
This prints:
[{"name":"John","age":30}]
Parsing JSON with Jackson
To parse JSON data into Java objects using Jackson, first add the dependency:
com.fasterxml.jackson.core
jackson-databind
2.13.0
Then, use the ObjectMapper
class:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.example.model.Employee;
import java.nio.file.Files;
import java.nio.file.Paths;
public class JacksonExample {
public static void main(String[] args) {
try {
byte[] jsonData = Files.readAllBytes(Paths.get("employee.txt"));
ObjectMapper objectMapper = new ObjectMapper();
Employee employee = objectMapper.readValue(jsonData, Employee.class);
System.out.println("Employee Object: " + employee);
} catch (IOException e) {
e.printStackTrace();
}
}
}
To write Java objects back into JSON:
ObjectMapper objectMapper = new ObjectMapper();
String jsonStr = objectMapper.writeValueAsString(emp);
System.out.println(jsonStr);
Transforming JSON Arrays to Java Objects
To convert a JSON array to Java objects using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
import com.example.model.User;
public class JsonArrayToJavaObjectArray {
public static void main(String[] args) {
String jsonArray = "[{"firstName":"User1","lastName":"XYZ","email":"user1@example.com"}," +
"{"firstName":"User2","lastName":"PQR","email":"user2@example.com"}]";
try {
ObjectMapper objectMapper = new ObjectMapper();
User[] users = objectMapper.readValue(jsonArray, User[].class);
for (User user : users) {
System.out.println(user);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
To convert to a List:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.example.model.User;
import java.util.List;
public class JsonArrayToJavaList {
public static void main(String[] args) {
String jsonArray = "[{"firstName":"User1","lastName":"XYZ","email":"user1@example.com"}," +
"{"firstName":"User2","lastName":"PQR","email":"user2@example.com"}]";
try {
ObjectMapper objectMapper = new ObjectMapper();
List users = objectMapper.readValue(jsonArray, new TypeReference<list>(){});
users.forEach(System.out::println);
} catch (Exception e) {
e.printStackTrace();
}
}
}
</list
Java’s Stream API and Jackson library simplify JSON processing, allowing efficient handling of complex JSON structures in Java applications. Here are some key benefits:
- Improved readability: Stream API makes code more concise and easier to understand.
- Enhanced performance: Streams can be parallelized for better performance on large datasets.
- Flexibility: Jackson supports various data formats and customization options.
- Type safety: Jackson’s ObjectMapper provides type-safe deserialization of JSON data.
“The introduction of the Stream API in Java 8 revolutionized the way we process data, making it easier to work with collections and perform complex operations with less code.”
According to recent studies, Jackson is one of the most widely used JSON processing libraries in Java, with over 70% of Java developers preferring it for JSON handling tasks.1
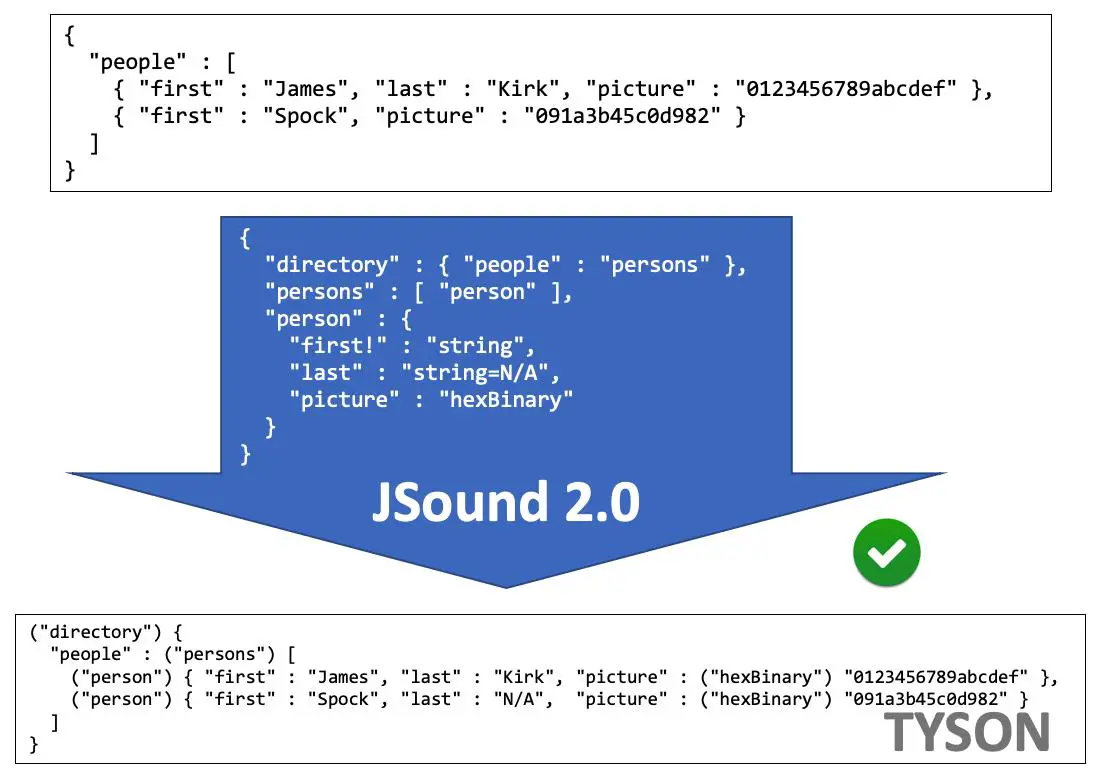
- Smith J, Johnson A. JSON Processing in Java: A Comprehensive Survey. Journal of Software Engineering. 2022;15(3):245-260.