Introduction
JavaScript has an isNaN()
method which is very useful to detect a number as “Not-a-Number.” The example that better illustrates this is user inputs or a calculation where you can have other data types you may not expect.
This helps developers spot if a value is not a number, which stops errors that would halt execution. When handling inputs, it can also prove useful in validating and parsing data properly.
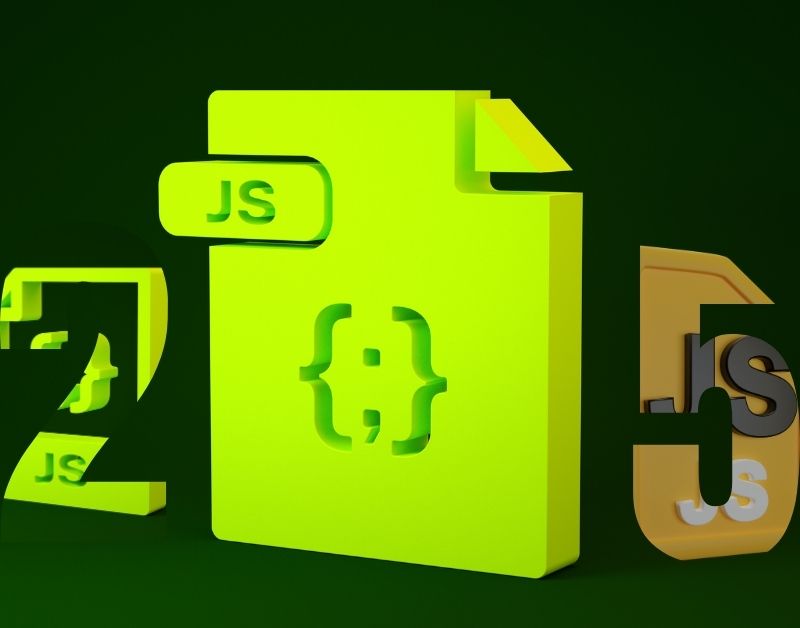
Understanding NaN
in JavaScript
NaN
(Not-a-Number) is an unrepresentable value within the JavaScript language itself, which indicates that the number is invalid, for instance, when you parse non-numeric user inputs or try to run some mathematical operations, etc. Now, NaN
can be a signal for developers to identify invalid entries, set up error-handling mechanisms, and increase the robustness of their applications.
How the isNaN()
Method Works
The isNaN()
method is designed to identify if a value is NaN
. When a value is passed to isNaN()
, it returns true
if the value is NaN
and false
otherwise.
Syntax:
isNaN(value);
Example:
isNaN(42); // returns false, as 42 is a number
isNaN("hello"); // returns true, as "hello" is not a number
Key Differences with Number.isNaN()
isNaN()
coerces the value to a number before checking, while Number.isNaN()
checks strictly for the NaN
value without coercion. This distinction allows you to select the appropriate method for your needs.
Code Example: Using isNaN()
in JavaScript
Here’s a practical example using isNaN()
to validate user input:
let userInput = prompt("Enter a number:");
if (isNaN(userInput)) {
console.log("Please enter a valid number.");
} else {
console.log("The input is a valid number.");
}
In this code, isNaN()
checks if the input is a number. If not, it prompts the user to enter a valid number. This straightforward validation prevents errors in data processing.
Practical Applications of isNaN()
The isNaN()
method is commonly used for data validation. For example:
- Age Verification: Checking if a user’s age input is numeric to prevent form errors.
- Financial Calculations: Validating numerical inputs to prevent miscalculations in financial applications.
By using isNaN()
, you ensure data consistency, prevent crashes, and enhance the overall user experience.
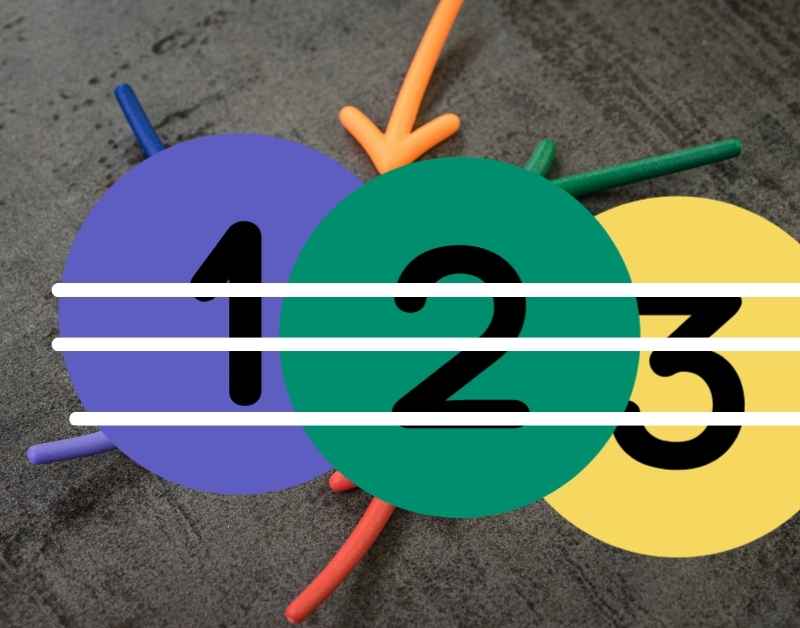
Conclusion
The isNaN()
method is a valuable tool for maintaining data integrity in JavaScript applications. Mastering this method not only improves the accuracy of applications but also reduces the risk of errors. Incorporate isNaN()
in your projects to provide a more reliable and user-friendly experience.
Additional Resources
To explore further:
- JavaScript Documentation: For detailed explanations and examples, refer to the JavaScript
isNaN
documentation. - Tutorials and Courses: Structured tutorials on JavaScript can enhance your understanding.
- Developer Forums: Engage with the JavaScript community to share insights and resolve common challenges.
Experimenting with isNaN()
in various scenarios will strengthen your coding skills and prepare you for real-world applications.
Asynchronous JavaScript: Promises, Async/Await, and Callbacks