Introduction to the HTML onclick
Attribute
The onclick attribute is a foundational building block in web development. It enables developers to create dynamic and interactive web pages. By adding JavaScript functionality directly to HTML elements, onclick facilitates actions like button clicks, form submissions, and more. Let’s explore in detail how this feature works and its importance in web development.
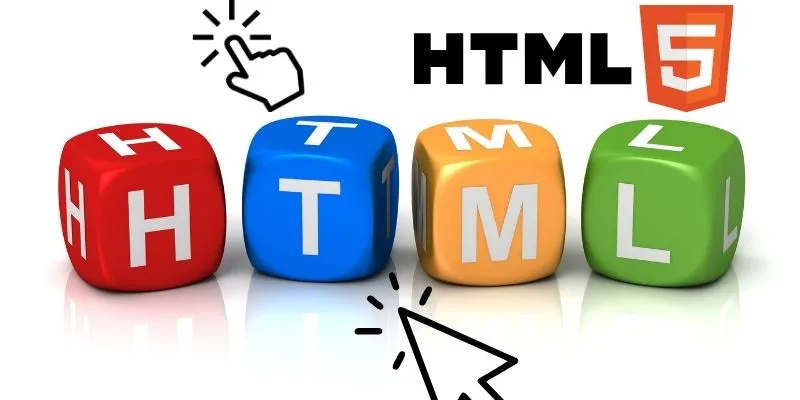
What is the onclick
Attribute?
The onclick
attribute is a built-in feature of HTML that allows developers to specify a JavaScript function or code snippet to execute when a user clicks on an element. It’s commonly used to add interactivity to web pages, such as opening a modal, toggling visibility, or validating forms.
Key Features:
- Event-driven interaction: Executes code in response to user actions.
- Versatility: Can be used on various HTML elements like buttons, links, and divs.
- Ease of use: Requires no additional libraries or frameworks.
For example, here’s a simple onclick
implementation:
<button onclick="alert('Button clicked!')">Click Me</button>
This snippet displays an alert box whenever the button is clicked.
Basic Syntax of the onclick
Attribute
The syntax for using the onclick
attribute is straightforward. You attach it as an attribute within an HTML element, and it accepts JavaScript code or a function as its value.
Syntax:
<element onclick="JavaScriptCode"></element>
Examples of Usage:
- Inline JavaScript:
<button onclick="console.log('Button clicked!')">Log Message</button>
- Calling a Function:
<button onclick="myFunction()">Run Function</button>
Here,myFunction
is a JavaScript function defined elsewhere in the code.
Using onclick
with Buttons
One of the most common applications of the onclick
attribute is with buttons. Buttons are often used to trigger specific actions like submitting forms, opening pop-ups, or changing the page’s content.
Example:
<button onclick="alert('You clicked the button!')">Click Me</button>
When the button is clicked, the alert()
function is executed, displaying a message to the user.
Explanation:
- The
onclick
attribute is directly placed within the<button>
tag. - The JavaScript code inside the quotes runs whenever the button is clicked.
Attaching JavaScript Functions to onclick
Instead of writing JavaScript code inline, you can link onclick
to external JavaScript functions. This improves code readability and reusability.
Example:
<!DOCTYPE html>
<html>
<head>
<script>
function greetUser() {
alert("Hello, User!");
}
</script>
</head>
<body>
<button onclick="greetUser()">Greet</button>
</body>
</html>
Why Use External Functions?
- Cleaner Code: Keeps HTML and JavaScript separate.
- Reusability: Allows the same function to be used across multiple elements.
- Scalability: Easier to debug and maintain.
onclick
in Links (Anchor Tags)
The onclick
attribute can also be applied to links to override their default behavior or add functionality.
Example:
<a href="#" onclick="alert('Link clicked!')">Click This Link</a>
In this case, clicking the link will trigger the alert but won’t navigate to a new page.
Explanation:
- The
href="#"
prevents the link from navigating. onclick
ensures the JavaScript code executes instead.- To entirely suppress navigation, use
event.preventDefault()
.
Dynamic Content Creation Using onclick
One of the most powerful uses of the onclick
attribute is to dynamically manipulate or create content on the webpage. This allows developers to build interactive user interfaces where elements can be added, modified, or removed without needing to reload the page.
Example:
Let’s look at how onclick
can dynamically add a new paragraph element to a webpage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dynamic Content Example</title>
<script>
function addParagraph() {
var newPara = document.createElement("p");
var text = document.createTextNode("This is a dynamically added paragraph.");
newPara.appendChild(text);
document.body.appendChild(newPara);
}
</script>
</head>
<body>
<button onclick="addParagraph()">Add Paragraph</button>
</body>
</html>
Explanation:
- When the button is clicked, the
addParagraph()
function is executed. - This function creates a new
<p>
element, adds some text to it, and then appends it to the body of the document. - No page reloads are necessary; the content is added dynamically.
This is just one example of what can be done with the onclick
attribute. By integrating JavaScript, onclick
can perform many other actions like hiding or showing elements, changing styles, or even interacting with external APIs.
Interactive Forms with onclick
Forms are another area where the onclick
attribute shines. You can use it to trigger JavaScript functions that validate user inputs, modify form values, or even prevent form submission if certain conditions aren’t met.
Example:
Here’s a basic form with onclick
validation:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Validation</title>
<script>
function validateForm() {
var name = document.getElementById("name").value;
if (name === "") {
alert("Name must be filled out!");
return false;
}
return true;
}
</script>
</head>
<body>
<form onsubmit="return validateForm()">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
</body>
</html>
Explanation:
- When the form is submitted, the
validateForm()
function is called. - It checks whether the name field is empty. If so, it prevents the form from submitting and alerts the user.
- If the name is entered, the form submits as usual.
This method allows you to perform simple or complex validation before actually submitting data, ensuring that your user inputs are correct and complete.
onclick
for Event Propagation and Delegation
Event propagation is an important concept in JavaScript. By default, events triggered on an element propagate up or down the DOM tree (this is known as bubbling and capturing). Using onclick
, you can take advantage of event propagation to handle events more efficiently.
Event Bubbling:
In event bubbling, an event triggered on a child element will propagate to its parent elements. This is useful when handling multiple similar events on the same parent element. Instead of attaching an event listener to each child, you can listen for events at the parent level and filter them based on the target.
Example of Event Delegation:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Delegation Example</title>
<script>
function handleClick(event) {
alert("You clicked on " + event.target.textContent);
}
</script>
</head>
<body>
<ul onclick="handleClick(event)">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</body>
</html>
Explanation:
- The
onclick
event is attached to the<ul>
element, the parent of the list items. - When any
<li>
item is clicked, the event propagates to the<ul>
, where thehandleClick()
function is executed. event.target
refers to the specific item that was clicked, and the alert displays the clicked item’s text.
This technique is highly efficient when dealing with large numbers of elements or dynamically generated content.
onclick
vs. Event Listeners
While the onclick
attribute is simple and straightforward, there are cases where it’s better to use JavaScript’s addEventListener()
method. Both approaches allow you to attach an event handler to an element, but they have key differences.
onclick
Attribute:
- Inline JavaScript: The code is written directly in the HTML element.
- One Event Handler: You can only assign one handler to an event at a time. If you define multiple
onclick
attributes for the same element, the last one will overwrite the others.
addEventListener()
Method:
- External JavaScript: You attach event listeners in external scripts, keeping the HTML clean.
- Multiple Handlers: You can attach multiple event listeners to the same event on the same element, which is useful for more complex logic.
- More Flexibility:
addEventListener()
offers better control, such as handling both capturing and bubbling phases.
Example Using addEventListener()
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Using addEventListener</title>
<script>
function showAlert() {
alert("Button clicked using addEventListener!");
}
window.onload = function() {
var button = document.getElementById("btn");
button.addEventListener("click", showAlert);
}
</script>
</head>
<body>
<button id="btn">Click Me</button>
</body>
</html>
Explanation:
- The event listener is added after the window loads, keeping the HTML free of inline JavaScript.
- The
showAlert()
function is triggered when the button is clicked, but you could also add additional event listeners to the same button without overwriting the previous one.
Inline vs. External JavaScript for onclick
When implementing onclick
, you have two main options: placing the JavaScript code inline within the HTML element or using an external JavaScript file. Both approaches have their pros and cons.
Inline JavaScript:
- Pros:
- Simple and easy for small scripts or quick demos.
- No need for an external file.
- Cons:
- Can clutter the HTML.
- Difficult to maintain and debug as projects grow larger.
- Makes it harder to separate structure (HTML), style (CSS), and behavior (JavaScript), violating the principle of separation of concerns.
External JavaScript:
- Pros:
- Keeps HTML clean and separate from the behavior code.
- Easier to maintain, especially in larger projects.
- Allows the same JavaScript code to be reused across multiple pages.
- Cons:
- Requires an additional HTTP request, which might slightly impact loading times.
- The external file must be correctly linked to the HTML document.
Best Practice:
For larger, production-level websites, it’s generally recommended to use external JavaScript files to keep your code organized and maintainable.
Accessing DOM Elements Using onclick
One of the most common uses of the onclick
attribute is to access and manipulate DOM (Document Object Model) elements. With onclick
, you can easily get references to elements on your webpage and modify their properties, styles, or content.
Example: Changing the Text of an Element
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Access DOM Elements</title>
<script>
function changeText() {
var element = document.getElementById("demo");
element.textContent = "Text has been changed!";
}
</script>
</head>
<body>
<p id="demo">This is the original text.</p>
<button onclick="changeText()">Change Text</button>
</body>
</html>
Explanation:
- When the button is clicked, the
changeText()
function runs. - The function uses
document.getElementById()
to select the paragraph with the IDdemo
. - The
textContent
property is updated, changing the paragraph’s text dynamically.
This approach is helpful for creating responsive and interactive web pages.
Triggering Multiple Functions with onclick
Sometimes, you might want a single click event to trigger multiple functions. This can be done by calling multiple functions within the onclick
attribute or using more advanced JavaScript techniques like chaining.
Example: Calling Multiple Functions Inline
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Multiple Functions</title>
<script>
function firstFunction() {
alert("First function executed!");
}
function secondFunction() {
alert("Second function executed!");
}
</script>
</head>
<body>
<button onclick="firstFunction(); secondFunction();">Click Me</button>
</body>
</html>
Explanation:
- Both
firstFunction()
andsecondFunction()
are executed sequentially when the button is clicked. - The semicolon (
;
) separates the function calls.
Advanced Approach: Chaining Functions
In more complex applications, you can use JavaScript event listeners and chaining for better readability and flexibility.
Preventing Default Behaviors Using onclick
By default, some HTML elements have predefined behaviors. For instance, clicking a link navigates to a new page, and submitting a form sends data to the server. You can use onclick
to override these default actions.
Example: Preventing Link Navigation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Prevent Default</title>
<script>
function preventLink(event) {
event.preventDefault();
alert("Link click prevented!");
}
</script>
</head>
<body>
<a href="https://example.com" onclick="preventLink(event)">Click Me</a>
</body>
</html>
Explanation:
- The
event.preventDefault()
method stops the link from navigating to itshref
destination. - This is useful when you want to add custom behavior to a link without changing its default appearance.
Debugging onclick
Errors
Debugging issues with onclick
can sometimes be tricky, especially when dealing with complex applications. Here are some common errors and how to address them:
Common Issues:
- JavaScript Syntax Errors:
- Ensure your JavaScript code inside
onclick
is free of typos or missing semicolons.
- Ensure your JavaScript code inside
- Function Not Defined:
- Make sure the JavaScript function you’re calling exists and is correctly linked to the HTML page.
- Incorrect Element References:
- Double-check that your
id
,class
, or other selectors match the elements you’re targeting.
- Double-check that your
Tips for Debugging:
- Use browser developer tools (F12) to check for errors in the console.
- Log messages with
console.log()
to understand what your code is doing. - Test your code incrementally to isolate problems.
Security Considerations with onclick
While the onclick
attribute is powerful, it also comes with potential security risks. Improper use can make your website vulnerable to attacks like Cross-Site Scripting (XSS).
Potential Risks:
- Inline JavaScript Injection: Attackers could inject malicious JavaScript into your
onclick
handlers. - Unvalidated User Input: Relying on user input without proper validation can open up vulnerabilities.
How to Mitigate Risks:
- Escape User Input:
- Always sanitize and validate input from users before using it in your
onclick
logic.
- Always sanitize and validate input from users before using it in your
- Avoid Inline JavaScript:
- Use external scripts or event listeners to separate your JavaScript logic from HTML.
- Content Security Policies (CSP):
- Implement CSP headers to restrict the execution of inline scripts on your website.
Conclusion and Best Practices
The onclick
attribute is a cornerstone of interactive web development. It allows developers to trigger actions with a simple click, enhancing the user experience. Whether you’re building a simple button or a complex application, understanding how to use onclick
effectively is crucial.
Best Practices:
- Use external JavaScript files to keep your code clean and maintainable.
- Leverage
addEventListener()
for complex or scalable projects. - Always validate and sanitize user inputs to prevent security vulnerabilities.
- Keep your code modular by breaking down functionality into reusable functions.
With these tips and examples, you’re ready to create dynamic, responsive, and secure web applications using the onclick
attribute.
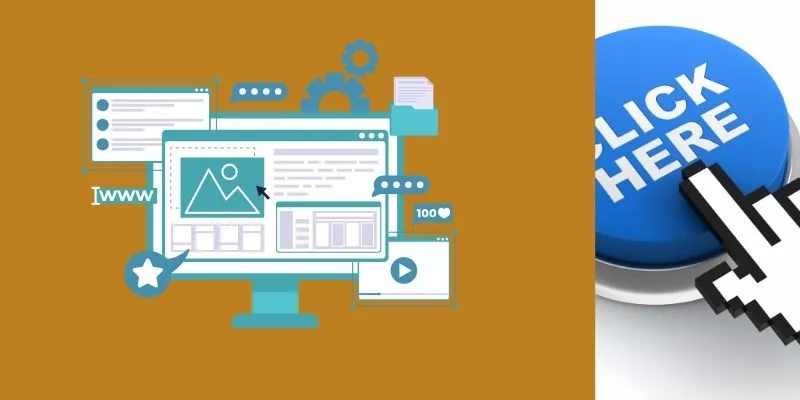
FAQs about the onclick
Attribute
1. Can I use multiple onclick
attributes on the same element?
No, an element can have only one onclick
attribute. If you need to attach multiple actions, consider using an external JavaScript function or the addEventListener()
method.
2. Is onclick
the only way to handle click events?
No, you can also use JavaScript’s addEventListener()
method, which provides more flexibility and supports multiple event handlers.
3. How do I remove an onclick
handler?
You can use JavaScript to remove onclick
by setting it to null
:
element.onclick = null;
4. Is onclick
secure to use?
It can be secure if used correctly. Avoid inline JavaScript and sanitize user inputs to prevent vulnerabilities like XSS.
5. Can I use onclick
with frameworks like React or Vue?
In frameworks like React, you use event binding methods instead of onclick
. For example, in React:
<button onClick={handleClick}>Click Me</button>
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.