In today’s tech-driven world, building APIs has become essential for connecting front-end and back-end systems. A RESTful API allows different applications to communicate seamlessly over the web. In this article, we’ll create a basic RESTful API using Node.js and Express in just a few steps.
1. What is a RESTful API?
A RESTful API follows the principles of Representational State Transfer (REST), enabling communication through standard HTTP methods like GET, POST, PUT, and DELETE. REST APIs are stateless, lightweight, and widely used for building scalable applications.
2. Setting Up the Environment
To get started, ensure Node.js is installed on your system. You’ll also need a code editor like VS Code.
- Initialize the project:
Open your terminal and run:
mkdir rest-api
cd rest-api
npm init -y
2.Install Express:
Express is a popular Node.js framework for building APIs.
npm install express
3. Building the API
Let’s create an API for managing a list of books.
- Create the server file:
Inside your project, create a file namedserver.js
and add:
const express = require('express');
const app = express();
app.use(express.json()); // To parse JSON bodies
const books = [
{ id: 1, title: 'Node.js Basics', author: 'John Doe' },
{ id: 2, title: 'Learn Express', author: 'Jane Smith' }
];
app.get('/books', (req, res) => res.json(books));
app.post('/books', (req, res) => {
const book = { id: books.length + 1, ...req.body };
books.push(book);
res.status(201).json(book);
});
app.listen(3000, () => console.log('Server running on http://localhost:3000'));
2.Run the server:
Start the API server by running:
node server.js
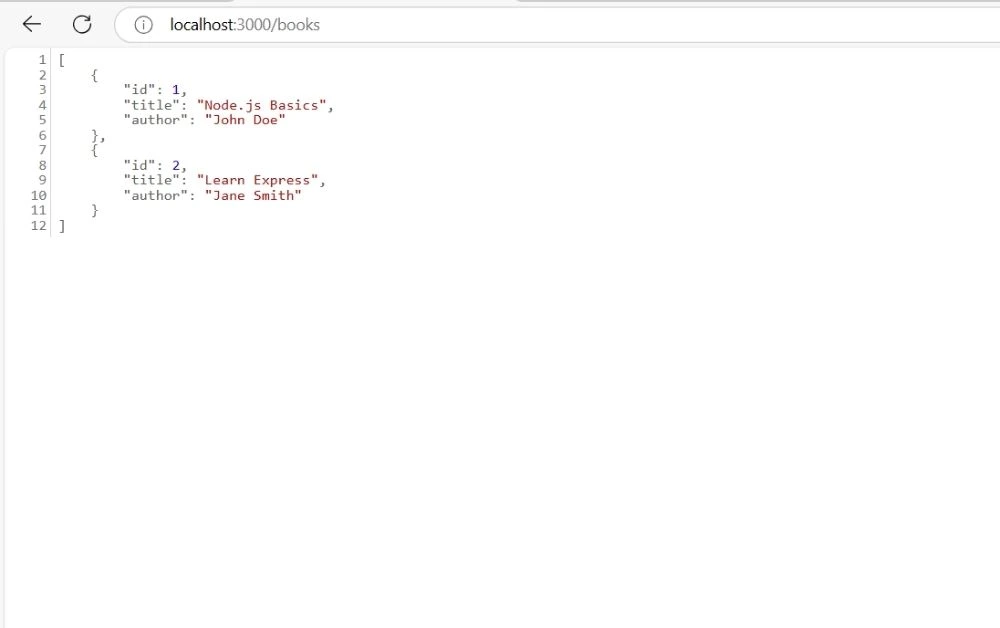
4. Testing the API
Use tools like Postman or cURL to test the API endpoints:
- GET /books: Retrieve the list of books.
- POST /books: Add a new book by sending a JSON payload like:
{
"title": "Mastering REST APIs",
"author": "Alice Johnson"
}
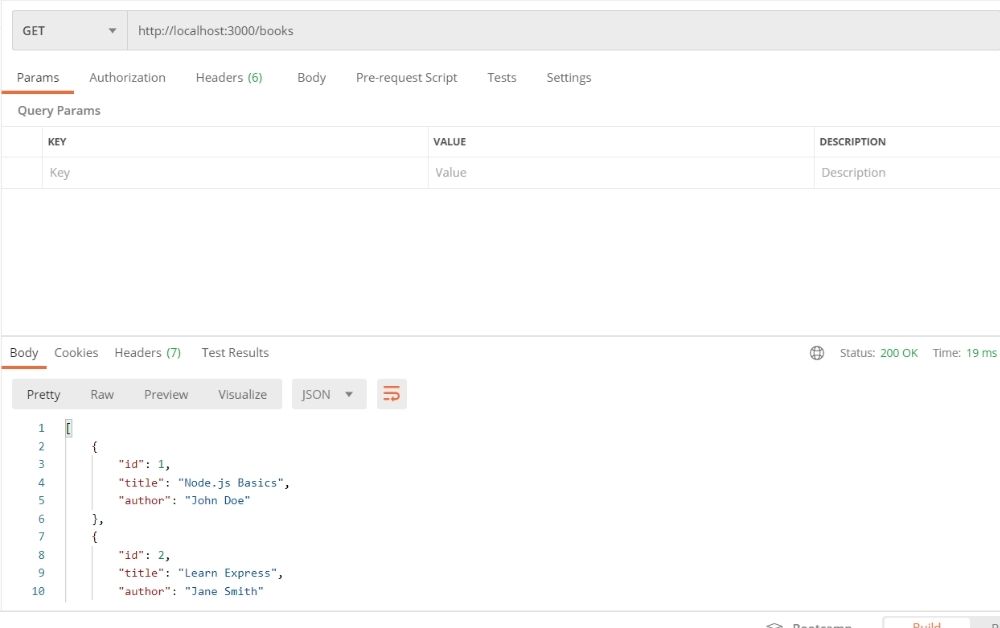
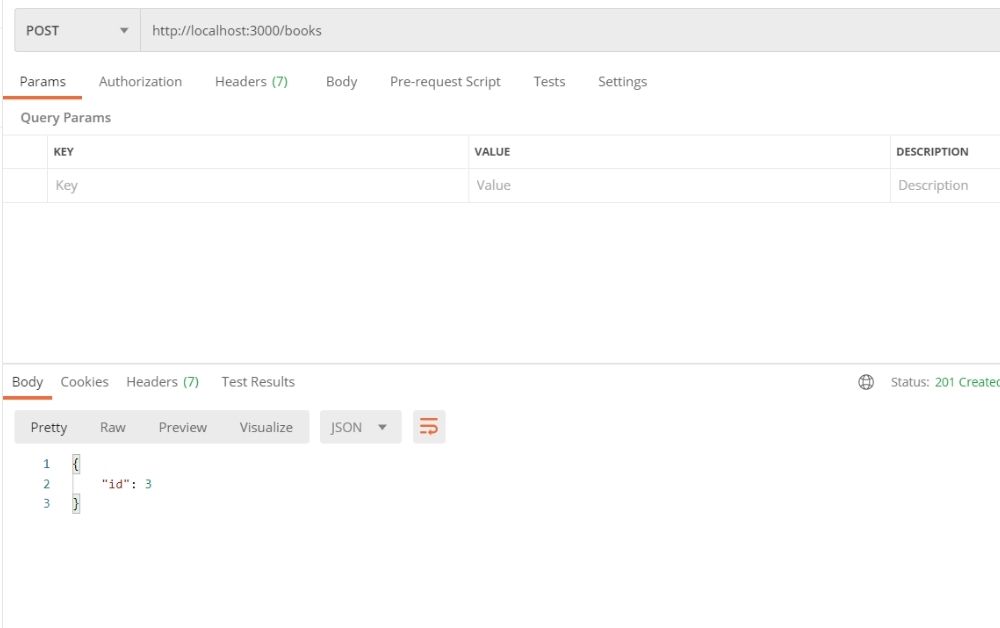
5. Conclusion
Building a RESTful API with Node.js and Express is straightforward and powerful. This basic setup is just the beginning—enhance it with database integration, authentication, and error handling for production-ready applications.
Ready to explore more? Dive deeper into API development and unlock the full potential of Node.js.
Originally published on Makemychance.com
HTTP Status Code: Understanding the Basics
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.