When working with authentication systems in Node.js, bcrypt
is a popular library used for hashing and comparing passwords. However, a common issue developers face is that bcrypt.compare
always seems to return false
, even when the inputs appear correct. Let’s explore the reasons behind this problem and how to fix it.
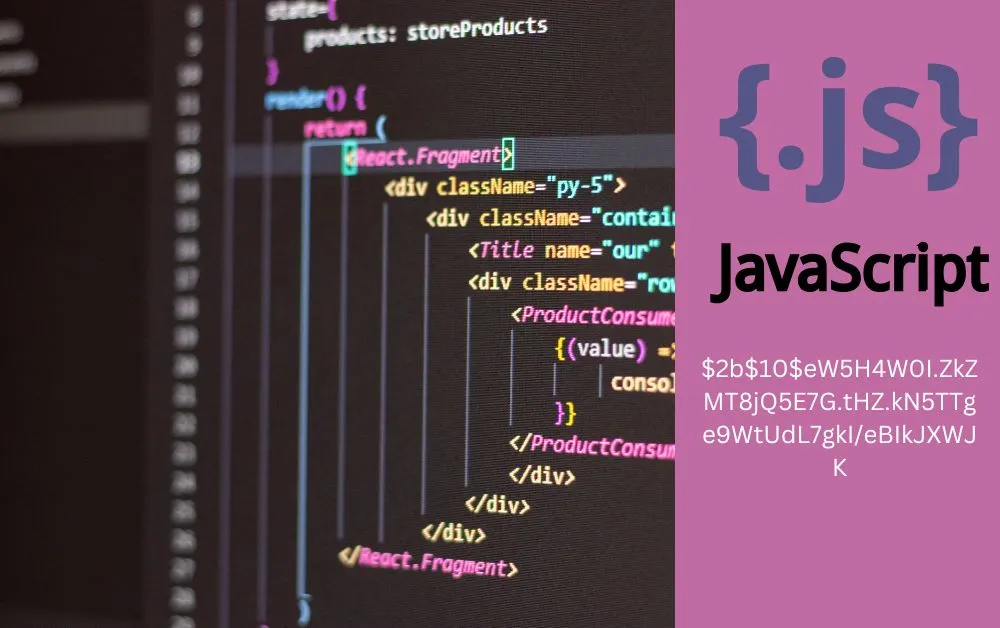
Understanding bcrypt.compare
The bcrypt.compare
function is used to compare a plain text password with a hashed password to verify if they match. Here’s how it works:
const bcrypt = require('bcrypt');
const password = 'mypassword';
const hash = '$2b$10$eW5H4W0I.ZkZMT8jQ5E7G.tHZ.kN5TTge9WtUdL7gkI/eBIkJXWJK'; // Example hash
bcrypt.compare(password, hash, (err, result) => {
if (err) {
console.error('Error comparing:', err);
} else if (result) {
console.log('Passwords match!');
} else {
console.log('Passwords do not match.');
}
});
If bcrypt.compare
always returns false
, there’s likely an issue with one of the inputs or the hashing process.
Common Reasons Why bcrypt.compare
Always Returns False
1. Incorrect Password or Hash
- Issue: The plain text password or hash provided to
bcrypt.compare
is incorrect. - Solution: Double-check that the password and hash being compared are the correct ones. Ensure you are passing the hash generated during user registration.
Learn more about bcrypt.compare.
2. Rehashing the Password
- Issue: Hashing the plain text password again before storing or comparing. Remember,
bcrypt
hashes are not reversible, and hashing a password twice creates a different hash. - Solution: Compare the plain text password directly with the stored hash without rehashing it.
Example:
// Incorrect approach (rehashing password):
const hash = bcrypt.hashSync(password, saltRounds);
bcrypt.compare(bcrypt.hashSync(password, saltRounds), hash); // Will always return false
// Correct approach:
bcrypt.compare(password, hash);
For an in-depth guide on how bcrypt
hashing works, visit BCrypt Algorithm Explained.
3. Hash Generated with a Different Salt
- Issue: The hash was generated with a different salt or algorithm settings than the one used during comparison.
- Solution: Ensure the salt rounds used for generating the hash are consistent across your application.
Example:
const saltRounds = 10; // Must remain consistent
const hash = bcrypt.hashSync('mypassword', saltRounds);
Refer to salt rounds best practices to understand their importance.
4. Password and Hash Data Types
- Issue: The password or hash is being passed in the wrong data type (e.g., hash as a plain string or password as a number).
- Solution: Ensure both inputs are strings.
Example:
// Incorrect data types:
const password = 123456; // Should be a string
const hash = someNonStringValue; // Should be a valid bcrypt hash string
// Correct data types:
const password = '123456';
const hash = '$2b$10$eW5H4W0I.ZkZMT8jQ5E7G.tHZ.kN5TTge9WtUdL7gkI/eBIkJXWJK';
5. Corrupted or Truncated Hash
- Issue: The hash has been altered, corrupted, or truncated, making it invalid.
- Solution: Verify that the hash stored in your database or application is complete and unchanged.
To better handle database interactions, refer to storing and retrieving hashes using Sequelize.
6. Using Asynchronous and Synchronous Functions Incorrectly
- Issue: Mixing synchronous and asynchronous
bcrypt
functions can lead to unexpected results. - Solution: Stick to either the asynchronous or synchronous functions consistently.
Example:
// Async:
bcrypt.compare(password, hash, (err, result) => {
console.log(result); // true or false
});
// Sync:
const result = bcrypt.compareSync(password, hash);
console.log(result); // true or false
7. Version Mismatch
- Issue: A mismatch between the
bcrypt
version used to generate the hash and the version used to compare it may cause errors. - Solution: Ensure the same version of
bcrypt
is used across your application or during hash generation and comparison.
Check the latest version of bcrypt and upgrade if necessary.
Best Practices to Avoid bcrypt.compare
Errors
- Consistent Hashing Strategy
Use the same salt rounds and hashing algorithm consistently. - Store Password Hashes Correctly
Store password hashes securely in your database and ensure they are not altered. - Validate Inputs
Ensure the inputs tobcrypt.compare
are valid strings and non-null. - Debugging
Log the inputs to verify the password and hash values being compared.
Example of Proper Implementation
Here’s a complete example of how to implement password hashing and comparison with bcrypt
:
const bcrypt = require('bcrypt');
const password = 'mypassword';
const saltRounds = 10;
// Hash the password
bcrypt.hash(password, saltRounds, (err, hash) => {
if (err) {
console.error('Error hashing password:', err);
return;
}
console.log('Hash:', hash);
// Compare the password with the hash
bcrypt.compare(password, hash, (err, result) => {
if (err) {
console.error('Error comparing password:', err);
} else if (result) {
console.log('Passwords match!');
} else {
console.log('Passwords do not match.');
}
});
});
For more examples and troubleshooting tips, visit the bcrypt GitHub repository.
Conclusion
When bcrypt.compare
always returns false
, it’s usually due to issues with the inputs or hashing process. By understanding how bcrypt
works and following best practices, you can effectively debug and fix this issue in your application. Always test your authentication system thoroughly to ensure a smooth user experience and secure password handling.