Java is one of the most widely used programming languages, and the process of converting source code into an executable form is an essential aspect of Java development. This process, commonly called a “build,” is central to software development in Java. In this article, we will explore what builds in Java entail, the tools and processes involved, and how they contribute to creating robust applications.
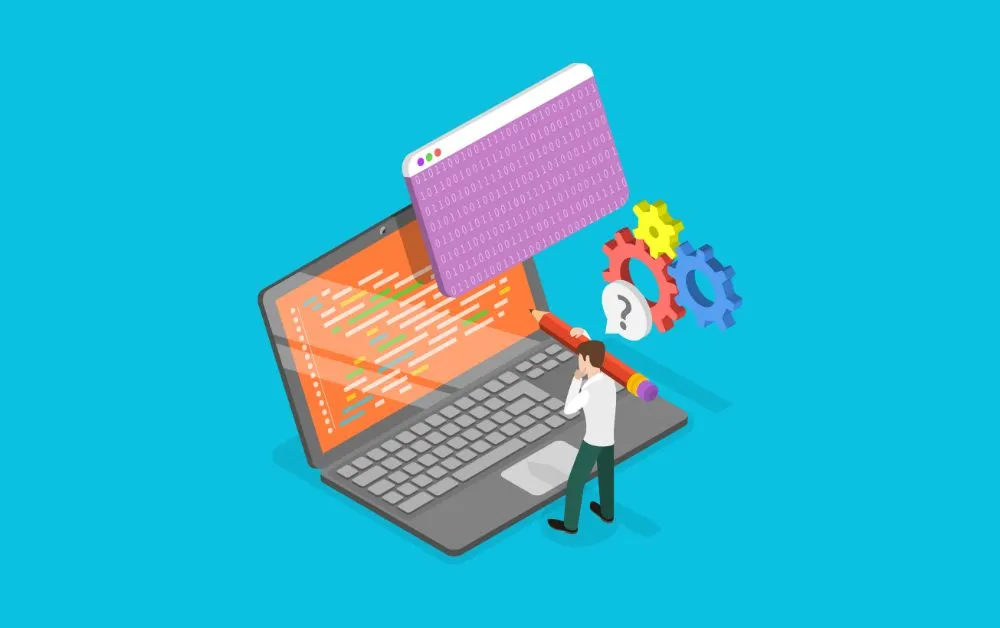
What is a Build in Java?
A build in Java is the process of compiling and packaging source code into a format that can be executed by a Java Virtual Machine (JVM). This process typically involves:
- Compiling: Converting Java source code (
.java
files) into bytecode (.class
files) using the Java compiler (javac
). - Linking and Packaging: Combining multiple
.class
files, libraries, and resources into a single deployable package like a.jar
or.war
file. - Testing: Running automated tests to ensure the build is functional and error-free.
- Deployment: Preparing the application for deployment to a server or other runtime environments.
The Build Lifecycle
A Java build lifecycle generally includes the following stages:
1. Initialization
- Setting up the project structure.
- Defining build configurations in build tools like Maven, Gradle, or Ant.
2. Compilation
- The source code is converted to bytecode using the
javac
compiler. - Syntax and semantic errors are identified during this phase.
3. Testing
- Unit tests, integration tests, and functional tests are run to validate the application’s behavior.
- Tools like JUnit, TestNG, or Mockito are often used for this purpose.
4. Packaging
- Bytecode files and resources are bundled into executable archives such as
.jar
,.war
, or.ear
files.
5. Deployment
- The final artifact is deployed to an application server (e.g., Apache Tomcat, WildFly) or a cloud environment.
6. Cleanup
- Temporary files and artifacts generated during the build process are removed to maintain a clean workspace.
Java Build Tools
Several tools are available to manage the build process in Java, each offering unique features:
1. Apache Maven
- A powerful build automation tool based on the Project Object Model (POM).
- Handles dependency management, building, and testing.
- Example
pom.xml
configuration:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
2. Gradle
- A modern and flexible build tool with a focus on performance.
- Uses Groovy or Kotlin for build scripts.
- Example
build.gradle
script:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
testImplementation 'junit:junit:4.13.2'
}
3. Apache Ant
- A simple and older build tool that uses XML-based build scripts.
- Example
build.xml
configuration:
<project name="MyApp" basedir="." default="compile">
<target name="compile">
<javac srcdir="src" destdir="build/classes"/>
</target>
</project>
Benefits of a Build System
Using a structured build system in Java offers several advantages:
- Consistency: Ensures consistent builds across different environments.
- Automation: Automates repetitive tasks like compiling, testing, and packaging.
- Dependency Management: Handles external library dependencies seamlessly.
- Scalability: Makes it easier to manage large and complex projects.
- Integration: Integrates with Continuous Integration/Continuous Deployment (CI/CD) pipelines for faster delivery.
Best Practices for Java Builds
- Use a Build Tool: Choose a build tool like Maven or Gradle based on your project needs.
- Maintain Dependencies: Regularly update and manage dependencies to avoid vulnerabilities.
- Automate Testing: Incorporate automated tests in the build process.
- Minimize Artifacts: Keep only necessary files in the final build artifact.
- Optimize for Performance: Use incremental builds to reduce build times.
Common Challenges and Solutions
Challenge | Solution |
---|---|
Dependency conflicts | Use dependency management features of Maven or Gradle to resolve conflicts. |
Long build times | Optimize build configurations and use caching or parallel builds. |
Build failures in CI/CD | Use containerized builds for a consistent environment. |
Outdated build tools/plugins | Regularly update tools and plugins to the latest versions. |
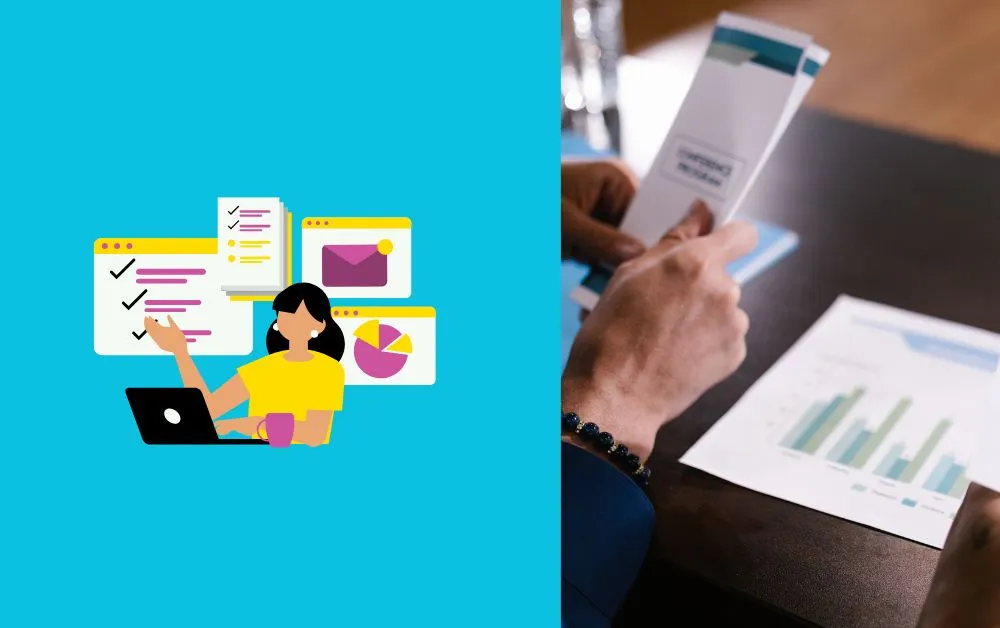
Conclusion
Builds are the backbone of Java development, enabling developers to transform source code into deployable applications. By understanding the build lifecycle, leveraging modern tools like Maven and Gradle, and adhering to best practices, you can streamline your development process and ensure the delivery of high-quality Java applications.
For more insights on Java development, visit Makemychance.com, where we provide tutorials, tips, and resources for developers.
Would you like help tailoring this content further for your needs?
Programming Projects for Beginners Java
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.