The tech world is changing fast, and machine learning is playing a big role. Developers using JavaScript are especially interested. Before, R and Python were the go-to languages for machine learning. But now, JavaScript is becoming a key player, thanks to its large community and built-in security.
JavaScript also runs faster than many other languages. This makes it great for web apps that need machine learning. With libraries like TensorFlow.js, web developers can now build complex machine learning apps right in the browser.
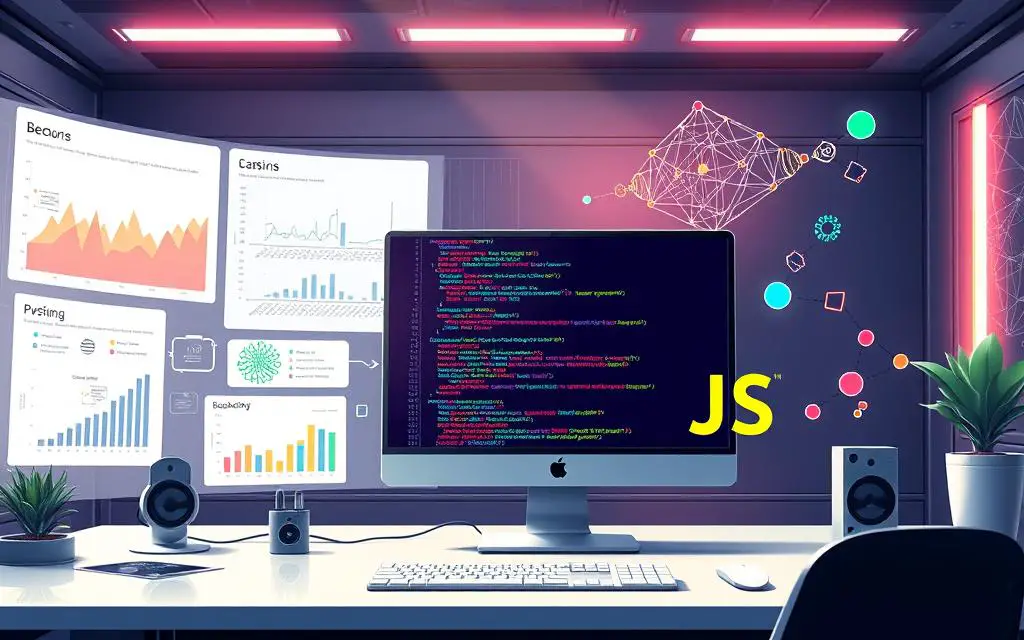
JavaScript is flexible and widely used, making it perfect for machine learning. This guide will cover the basics of machine learning in JavaScript. We’ll look at popular libraries and show you how to apply them in your projects.
Key Takeaways
- Machine learning in JavaScript expands opportunities for web developers.
- JavaScript is increasingly viable for running complex machine learning models alongside web applications.
- TensorFlow.js is a cornerstone library for javascript machine learning, enhancing user experience without sacrificing speed.
- Pre-trained models can be utilized in-browser, facilitating efficient inference without the need for extensive training.
- JavaScript’s adaptability ranges from web to mobile applications and beyond, presenting a versatile platform for machine learning innovation.
Introduction to Machine Learning in JavaScript
Machine learning is now a key skill in tech, often seen as complex and tied to Python. But, developers can use JavaScript for machine learning, opening up new web development chances.
JavaScript libraries for machine learning keep getting better. They make it easier to use complex algorithms without learning another language. Frameworks like TensorFlow.js, Brain.js, and ml5.js let you add machine learning to web apps.
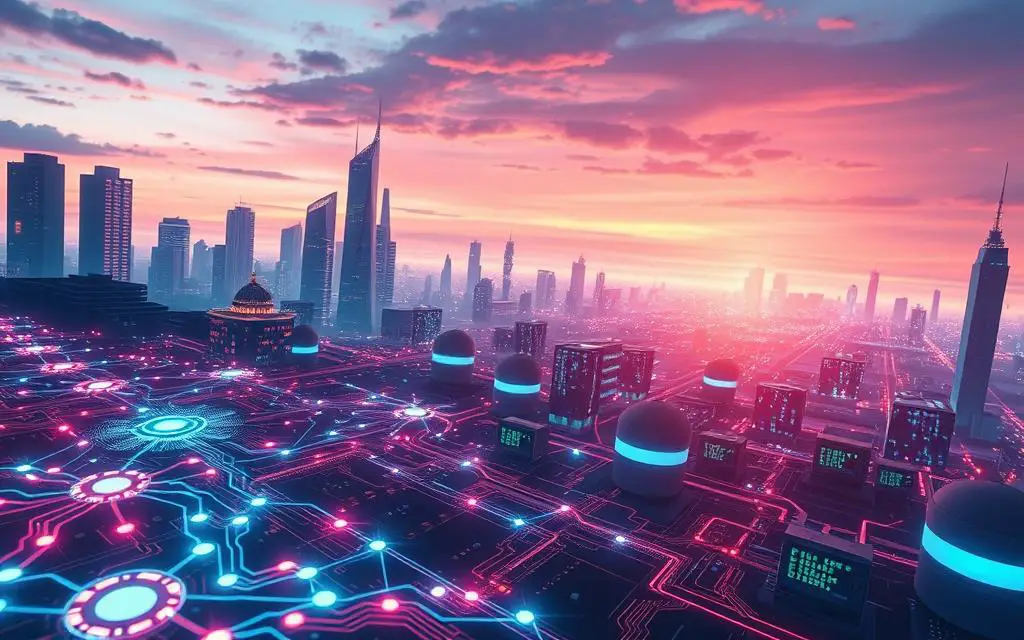
To start, you just need to know the basics of machine learning. It includes supervised, unsupervised, semi-supervised, and reinforcement learning. Developers can use pre-trained models for tasks like image recognition and text analysis.
Getting hands-on with machine learning makes learning easier. You can try projects like object detection and face detection. Tools like Brain.js make neural networks easier to understand, making learning less scary.
As more web developers need machine learning, understanding its value is important. By exploring machine learning in JavaScript, developers can make new, exciting apps that change how we interact online.
The Importance of Machine Learning for Web Developers
Machine learning is changing web development. It makes user experiences better and solves problems in new ways. For web developers, knowing machine learning algorithms in JavaScript is key to staying ahead.
JavaScript is becoming more important in AI and machine learning. It can run AI models right in the browser. Libraries like TensorFlow.js and Brain.js help developers build and use machine learning models easily.
Using AI in web apps is no longer just a trend. By 2024, it’s a must for developers. JavaScript and machine learning are just starting to work together. This opens up many opportunities for developers to explore.
Developers can make web apps smarter with machine learning. They can add features like predictive models and image recognition. JavaScript is becoming essential for both front-end and back-end development, making data processing more efficient.
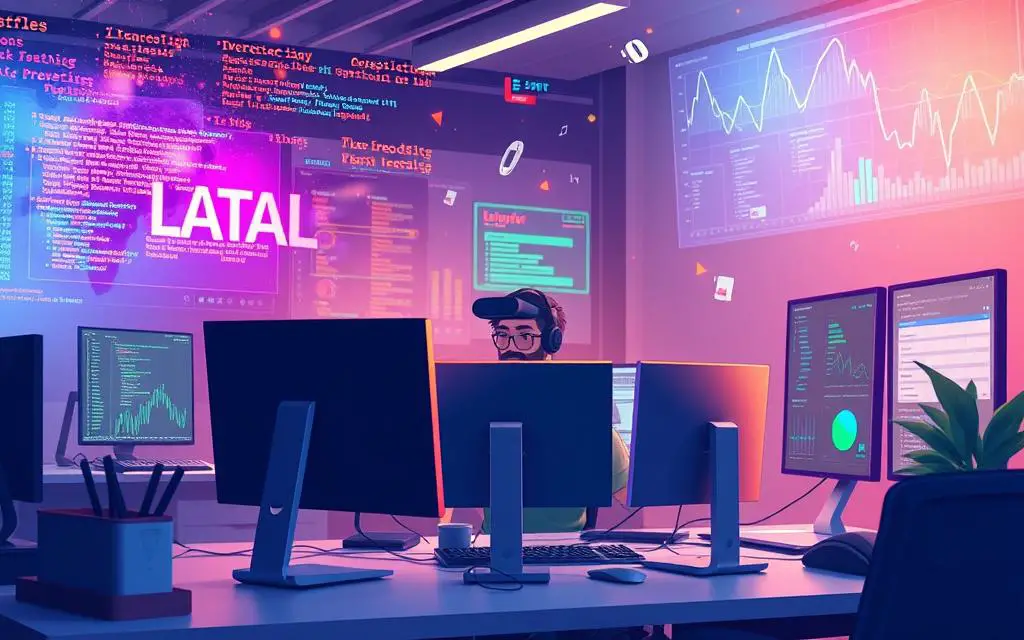
In summary, knowing how to use machine learning in JavaScript is crucial. It opens up new possibilities for innovation and growth in web development. Developers who embrace this technology will help create smarter, more responsive apps for the future.
Popular JavaScript Libraries for Machine Learning
JavaScript has become key in machine learning, thanks to powerful libraries. These tools let developers use machine learning in web apps. We’ll look at three top libraries with unique features.
TensorFlow.js Overview
TensorFlow.js is a machine learning library for the browser. It supports tasks like image classification, language translation, and reinforcement learning. It’s great for adding complex models to web apps without losing performance.
It uses GPU acceleration through WebGL for fast computations. This is a big plus for handling large data sets.
Brain.js: Simplifying Neural Networks
Brain.js is known for making javascript neural networks for machine learning easy. It supports different network types, like feedforward and recurrent. It’s perfect for those who want to build models fast and with little effort.
Neural networks with Brain.js can be set up in just a few lines of code. This makes it great for quick projects.
ml5.js: Making Machine Learning Accessible
ml5.js makes machine learning easy for all, no matter their experience. It has a simple API for training and using models in the browser. It supports both supervised and unsupervised learning.
This makes it a solid base for both simple and complex projects. Its focus on accessibility has made it popular among educators and hobbyists.
Implementing Machine Learning in JavaScript
Starting a machine learning project in JavaScript can seem hard. But with the right tools, it’s easy to get started. This guide will help you set up your project using TensorFlow.js and ml5.js.
Setting Up Your Development Environment
To begin, create a Node.js project and add TensorFlow.js. Here’s how:
- Install Node.js from the official website.
- Create a new project directory and navigate to it.
- Run npm init to set up your project.
- Install TensorFlow.js by running npm install @tensorflow/tfjs in your terminal.
- Prepare your code editor to start writing machine learning tutorials using JavaScript.
By following these steps, you’ll have a ready environment for machine learning. JavaScript’s clear documentation makes it easy to start.
Creating Your First Prediction Model
Now, let’s build a simple prediction model. Use TensorFlow.js to classify handwritten digits from the MNIST dataset. Here’s what you need to do:
- Load the MNIST dataset.
- Normalize the data and split it into training and testing sets.
- Define the model architecture, including layers with specific activation functions such as ‘relu’ and ‘softmax’.
- Compile the model with appropriate optimizers and loss functions.
- Train the model for a set number of epochs, adjusting as necessary based on accuracy.
The table below shows important parts of the implementation:
Element | Description |
---|---|
Layers | 128 units in the first layer, 64 in the second, and 10 in the output layer. |
Activation Functions | ‘relu’ for hidden layers and ‘softmax’ for output layer. |
Training Parameters | 20 epochs with an ‘adam’ optimizer and ‘categoricalCrossentropy’ loss function. |
Validation Set | 2,000 samples for evaluating the model’s generalization. |
After training, save the model and use it in a web app for real-time predictions. This shows how to start with machine learning in JavaScript.
Understanding Machine Learning Algorithms in JavaScript
Machine learning algorithms in JavaScript are key to making smart apps. They help improve user experience and automate tasks in web apps. There are two main types: supervised and unsupervised learning.
Supervised learning uses labeled data to make predictions. It includes methods like linear regression and decision trees. Unsupervised learning finds patterns without labels, using clustering and dimensionality reduction. Knowing these types is crucial for using machine learning in JavaScript.
Data preprocessing is vital for machine learning in JavaScript. It cleans and organizes data to improve model performance. Choosing the right features is important. Libraries like Math.js help with data manipulation.
The JavaScript world uses machine learning for real-time apps. Libraries like TensorFlow.js, Brain.js, and ml5.js make it easy. TensorFlow.js lets developers train models in the browser or Node.js. Brain.js makes neural networks simple. ml5.js makes machine learning easy for everyone, even for tasks like image classification.
The table below shows the main machine learning libraries in JavaScript. It highlights their key features:
Library | Key Features | Best For |
---|---|---|
TensorFlow.js | Build and train models in-browser, extensive community support | Complex models and deep learning applications |
Brain.js | Simplifies neural networks, easy to use | Beginner-friendly network creations |
ml5.js | High-level abstractions, minimal code required | Accessibility for non-experts in ML |
ConvNetJS | Deep learning in-browser, supports various learning techniques | Advanced users interested in deep learning |
Synaptic | Architecture-agnostic neural networks, actively maintained | Flexible network development |
Using machine learning in JavaScript makes app development faster. The ecosystem is growing fast. It offers tools for creating new apps, even in areas like natural language processing and image recognition.
JavaScript Neural Networks for Machine Learning
Neural networks are a powerful tool in solving complex problems in machine learning with JavaScript. TensorFlow.js makes it easy for developers to work with neural networks in JavaScript. This section will show how to build a basic neural network for practical tasks.
Using TensorFlow.js to Create Neural Networks
Let’s start with a simple neural network. Imagine a network with 2 input neurons and 4 output neurons. The connections have random weights between 0 and 1, ready for learning.
The output layer’s biases are set to zero. The sigmoid function is used to scale the output between 0 and 1. During training, the weights are adjusted based on the difference between expected and actual outputs.
JavaScript has basic algorithms like linear regression and logistic regression. These help with machine learning tasks from scratch. The math.js package adds to these capabilities, making calculations easier.
The deeplearn.js library makes creating and running neural networks in JavaScript easier. It’s similar to TensorFlow and uses the GPU for faster calculations.
One use of this neural network is to find the best font color for different background colors. This improves web accessibility. Training sets are made from random RGB colors, linking inputs to outputs.
Feature | Description |
---|---|
Network Structure | 2 input neurons, 4 output neurons |
Weight Initialization | Random values between 0 and 1 |
Bias Configuration | Array of zeros for output neurons |
Activation Function | Sigmoid function for output scaling |
Training Method | Weight adjustment based on output errors |
Data Generation | Random RGB sets for training and testing |
Scaling RGB color channels to 0 to 1 makes the neural network work better. It shows how efficient JavaScript neural networks are for machine learning.
Practical Machine Learning Tutorials Using JavaScript
Working on practical machine learning tutorials with JavaScript can really boost your skills. You get to apply what you’ve learned to real projects. This hands-on experience is crucial for becoming good at machine learning.
Here are some fun project ideas to try:
- Image Classifier: Create a simple image classifier with TensorFlow.js. Begin with a basic dataset and then add more images to make it harder.
- Football Match Prediction Model: Make a model that predicts football game results based on past games. Use stats and machine learning together.
- Text Sentiment Analysis: Build a program that reads and sorts text feelings. This can make user interactions better.
Tutorials stress the need for coding, data handling, and math skills. Sites like TensorFlow have lessons for all levels. They cover everything from ML basics to advanced ideas.
For in-depth learning, check out books like “AI and Machine Learning for Coders” by Laurence Moroney and “Deep Learning with Python” by Francois Chollet. They help you understand how to do it and why it works.
Getting your hands dirty is the best way to get better at machine learning. The more you practice, the better you’ll get. Using all the resources out there will help you grow your skills and confidence.
Benefits of Using JavaScript for Machine Learning
JavaScript is a big deal in AI because it’s easy to use. Many web developers already know JavaScript. This makes it easier for them to start working on machine learning projects.
JavaScript also works well with web apps. It lets developers add machine learning to websites easily. This makes websites more interactive and fun to use.
More developers are using JavaScript for machine learning. This has led to new tools and libraries. Libraries like Brain.js and Synaptic help build predictive models and neural networks.
JavaScript works on all modern devices. This is great for mobile apps. Libraries like TensorFlow.js let developers use machine learning in apps without needing to write native code.
JavaScript is good at handling big data. It’s perfect for creating dynamic apps. Tools like SmartScripter make AI development easier for everyone.
Conclusion
Machine learning in JavaScript is changing the game for web developers. It lets them build smarter, more interactive web apps. With frameworks like TensorFlow.js and Brain.js, developers can add cool features like real-time image recognition and personalized content.
This makes it easy to use machine learning without needing to know other programming languages. It’s a big win for developers who want to make their apps more engaging.
JavaScript libraries for machine learning make complex tasks like natural language processing and predictive analytics easier. Developers can try out different algorithms and models with tools like Synaptic and ml5.js. This encourages innovation and can really boost user engagement and business success.
Even though there are challenges like performance issues and data privacy, the future looks bright. As more people want intelligent apps, machine learning in JavaScript will become even more important.
Learning about machine learning can give developers a big advantage. It helps them stay ahead in the tech world. By embracing machine learning, developers can thrive in a rapidly changing tech landscape.
FAQ
What is machine learning in JavaScript?
Machine learning in JavaScript means using the JavaScript language to make smart apps. It lets web developers add intelligence to their work. This can be done right in the browser or on a server with Node.js.
Which JavaScript libraries are best for machine learning?
Top choices include TensorFlow.js for advanced ML and GPU help; Brain.js for easy neural networks; and ml5.js for quick prototyping.
How can I implement a machine learning model in JavaScript?
First, set up a Node.js environment. Then, install TensorFlow.js. Next, write code to train a model with your data. There are many tutorials to help you.
What are the key algorithms used in JavaScript machine learning?
Important algorithms are supervised, unsupervised, and reinforcement learning. JavaScript makes these efficient for real-time use.
Can I create neural networks with JavaScript?
Yes, with libraries like TensorFlow.js. They help build, train, and use neural networks in web apps.
What are some practical project ideas for using machine learning in JavaScript?
Ideas include making an image classifier, a chatbot, or a sports prediction model. These projects improve your machine learning skills.
Why should web developers learn machine learning?
Learning machine learning boosts your skills. It lets you make more advanced, data-driven apps. This makes your work more valuable and keeps you up-to-date in tech.
Top JavaScript Tools for Developers
Top JavaScript Libraries for Data Visualization
Using OpenAI for Data Analysis and Visualization
Source Links
- A Web Developer’s Guide to Machine Learning in JavaScript
- Welcome to the Machine: a gentle introduction to Machine Learning in JavaScript 🤖
- Learn Machine Learning in JavaScript with TensorFlow.js
- W3Schools.com
- How AI and Machine Learning are Shaping the Future of JavaScript
- AI and Machine Learning with JavaScript: Powering Intelligent Web Apps
- Best Javascript Machine Learning Libraries in 2024
- 10 Best JavaScript Machine Learning Libraries in 2024
- #21 Using JavaScript for Machine Learning
- Revolutionize Your Web Applications: Implementing Machine Learning Models in JavaScript with…
- Machine Learning in JavaScript Using TensorFlow JS | Technology | Blog
- 10 Machine Learning Examples in JavaScript
- Machine Learning with JavaScript – GeeksforGeeks
- 🧠 An AI / neural network…in vanilla JS! 😱 With no libraries! 🤯
- Neural Networks in JavaScript with deeplearn.js
- Project Based Learning
- Machine learning education | TensorFlow
- 4 reasons to learn machine learning with JavaScript – TechTalks
- JavaScript and AI: Mastering Machine Learning with TensorFlow.js
- Exploring Machine Learning in JavaScript
- How to do machine learning in JavaScript?
- JavaScript for Machine Learning? Weighing The Pros and Cons