When building web applications, one of the most debated topics among developers is whether to use JavaScript or TypeScript. JavaScript is the foundation of the web, while TypeScript is a popular superset that brings static typing to JavaScript. Understanding the key differences and when to choose one over the other can help you make an informed decision and improve your development workflow.
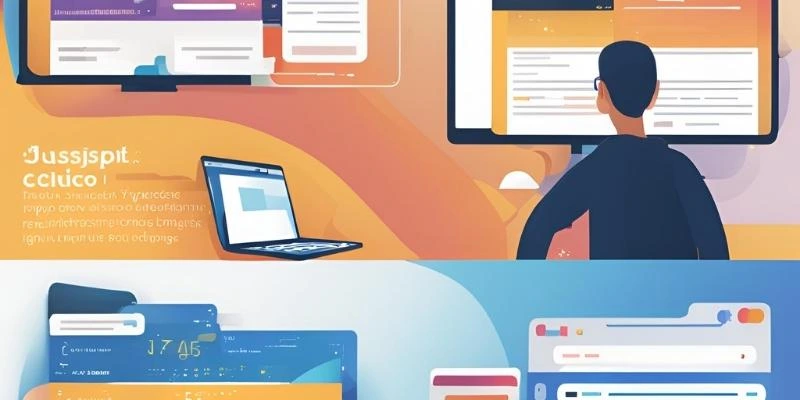
What is JavaScript?
JavaScript is a high-level, interpreted programming language that enables dynamic content on websites. It is the language that powers most of the interactive and responsive features on the web. JavaScript is known for its flexibility, simplicity, and ubiquity, making it essential for modern web development.
What is TypeScript?
TypeScript is an open-source superset of JavaScript, developed by Microsoft, that adds optional static typing. While JavaScript is dynamically typed, meaning types are determined at runtime, TypeScript allows developers to define data types explicitly, which makes it easier to catch errors early and write more predictable code.
Key Differences Between JavaScript and TypeScript
Here’s a breakdown of the most important differences between JavaScript and TypeScript:
Feature | JavaScript | TypeScript |
---|---|---|
Typing | Dynamic (types are determined at runtime) | Static (types are defined at compile-time) |
Compilation | Interpreted directly in the browser | Compiled to JavaScript before execution |
Error Checking | Errors appear only at runtime | Errors detected at compile-time |
Learning Curve | Easier to learn | Steeper learning curve for JavaScript developers |
Community Support | Large community, available in all browsers | Growing community, needs a compiler (e.g., TSC) |
Tooling | Supported by most IDEs, flexible | Best support in VSCode, requires more setup |
Benefits of JavaScript
- Widespread Support: JavaScript is natively supported by all major browsers, making it universal for web development.
- Quick Development: Since JavaScript is dynamically typed, it allows for rapid prototyping.
- Wide Ecosystem: The JavaScript ecosystem is vast, with numerous frameworks, libraries, and tools such as React, Vue, and Node.js.
Benefits of TypeScript
- Type Safety: TypeScript’s static typing prevents many runtime errors by enforcing type checks at compile time.
- Enhanced Code Readability and Maintenance: Type definitions help make code self-documenting, which makes it easier for teams to read and understand.
- Better Tooling Support: Modern editors like Visual Studio Code provide powerful auto-completion, navigation, and refactoring features for TypeScript, thanks to its typing system.
When to Use JavaScript
- Small Projects and Prototypes: For smaller applications or quick prototypes, JavaScript is often the more convenient choice because of its simplicity and lower setup requirements.
- Compatibility Requirements: If you’re working with legacy code or on projects where TypeScript support is not feasible, JavaScript may be the way to go.
- Dynamic Programming Needs: If your project requires highly dynamic structures that change frequently, JavaScript’s flexibility may be more suitable.
When to Use TypeScript
- Large-Scale Applications: For larger applications, TypeScript’s static typing reduces errors and makes it easier to refactor and maintain code.
- Team Projects: TypeScript’s type system helps teams work on the same codebase without confusion, as the types help prevent unintended errors.
- Code Stability: If stability is a priority, TypeScript’s error-checking and type validation will help you avoid unexpected runtime errors.
Performance Comparison
Since TypeScript compiles down to JavaScript, the performance of the final code is effectively the same as JavaScript. However, the build process for TypeScript adds an extra step before deployment, which may slightly increase development time. For large applications, this is typically a minor trade-off in favor of the benefits TypeScript offers in debugging and maintainability.
Common Misconceptions
- TypeScript is Not JavaScript: TypeScript is still JavaScript at its core, just with added type features. Every valid JavaScript code is also valid TypeScript.
- TypeScript is Harder to Learn: For developers who know JavaScript, picking up TypeScript is relatively straightforward, as it simply builds upon familiar JavaScript concepts with additional typing.
Should You Switch from JavaScript to TypeScript?
If you’re a solo developer working on small projects, JavaScript may be sufficient. However, if you’re building scalable applications or working in a team environment, TypeScript is likely to be beneficial. Transitioning from JavaScript to TypeScript is easier if done gradually—many projects start by adding TypeScript to a few files, rather than converting everything at once.
Practical Examples
- JavaScript Example (Dynamic Typing)
function greet(name) {
return "Hello, " + name;
}
console.log(greet(5)); // Outputs: Hello, 5
The above code won’t throw an error at compile-time, even though passing a number to name
may not be the intended behavior.
- TypeScript Example (Static Typing)
function greet(name: string): string {
return "Hello, " + name;
}
console.log(greet(5)); // Error: Argument of type 'number' is not assignable to parameter of type 'string'
In TypeScript, defining name
as a string
type means you’ll get an error if you pass any type other than a string. This kind of early error-checking helps prevent potential issues.
Conclusion
JavaScript and TypeScript each have their strengths and ideal use cases. JavaScript remains the language of choice for quick projects and smaller teams, while TypeScript provides a more robust environment for larger, scalable applications where type safety is beneficial. Ultimately, the best choice depends on the project requirements, team size, and the complexity of the application you’re building.
If you’re starting a new project and looking for stability and maintainability, TypeScript may be worth the initial learning curve. For developers who are comfortable with JavaScript and prefer the flexibility of dynamic typing, JavaScript is still a solid choice.
Final Thoughts
Choosing between JavaScript and TypeScript depends on balancing simplicity and structure. As TypeScript continues to gain traction, its benefits are being recognized across various development environments. Whether you choose JavaScript or TypeScript, understanding the strengths of each can help you make the right decision and elevate the quality of your applications.
Top JavaScript Tools for Developers
Top JavaScript Libraries for Data Visualization
Asynchronous JavaScript: Promises, Async/Await, and Callbacks