Java Socket Creation
Creating a socket in Java enables data sharing between computers. The java.net.Socket class is used to connect to a server using its IP address and port number. To establish a connection, use this syntax:
Socket socket = new Socket("example.com", 80);
This connects to a server at “example.com” on port 80, often used for web traffic.
To send data, use the socket’s output stream:
OutputStream out = socket.getOutputStream();
out.write("Hello, server!".getBytes());
out.flush();
To receive data, use the input stream:
InputStream in = socket.getInputStream();
int data = in.read();
Finally, close the socket to end the connection:
socket.close();
Understanding IP addresses, ports, and input/output streams is essential for establishing solid connections for various applications.
Data Transmission in Java Sockets
After connecting to a server, the next step is sending data packets. The OutputStream is used to send data:
OutputStream out = socket.getOutputStream();
byte[] message = "Hello again!".getBytes();
out.write(message);
out.flush();
The flush()
method ensures data is sent immediately rather than being buffered. This is important for timely data transmission.
Data buffering optimizes network performance by sending data only when there’s enough to transmit efficiently. However, this can sometimes delay crucial data. Using flush()
ensures prompt delivery.
Handling Java Socket Data
Reading data from a Java socket uses the InputStream:
InputStream in = socket.getInputStream();
Data is read byte by byte:
int data;
while ((data = in.read()) != -1) {
// Process the incoming data
}
For asynchronous data transfers, consider using a separate thread:
new Thread(() -> {
try {
int byteData;
while ((byteData = in.read()) != -1) {
// Handle byteData
}
} catch (IOException e) {
e.printStackTrace();
}
}).start();
Understanding when the server is transmitting or has disconnected allows for creating resilient applications ready for dynamic data exchange.
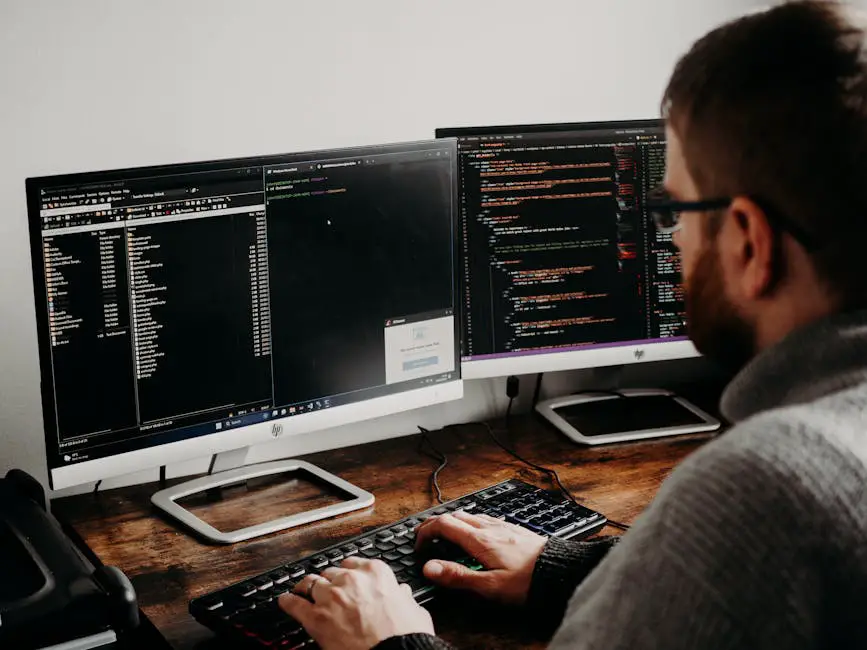
Java Socket Closure
Closing a Java socket is essential for maintaining system health and preventing resource leaks. To close a socket:
socket.close();
It’s also important to close the input and output streams:
inputStream.close();
outputStream.close();
Handle potential exceptions during closure:
try {
inputStream.close();
outputStream.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
Properly closing sockets helps protect your system from potential vulnerabilities and unforeseen data issues.
Common Java Socket Issues
One common issue is the OverflowError
when handling signed byte arrays. To address this, use:
byte b = (byte) ((value + 128) % 256 - 128);
Data type mismatches can occur during socket communication. Ensure proper conversion methods are used, such as getBytes()
for strings.
Always use try-catch blocks to handle unexpected issues gracefully. This allows you to identify and resolve problems while maintaining a functional application.
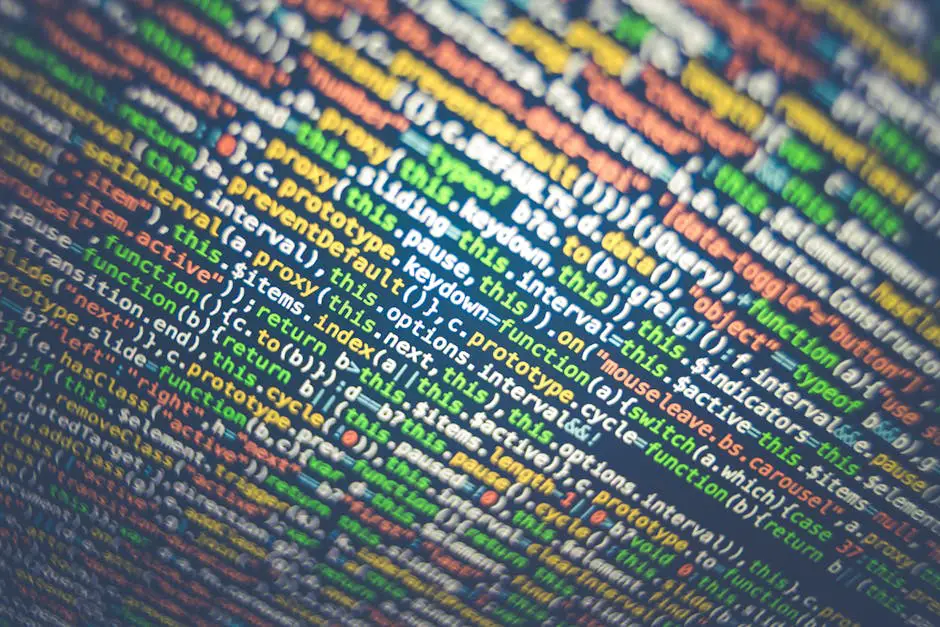
Mastering Java sockets involves understanding the basics of network communication. By focusing on creating, managing, and closing connections effectively, you establish a strong foundation for reliable data exchange.
Revolutionize your content with Writio – an AI writer for website publishers. This article was written by Writio.
- Oracle Corporation. Java Documentation: Networking. Oracle Technology Network.
- Jenkov J. Java Networking: Socket Programming. Jenkov.com.
- Journal Dev. Java Socket Programming. JournalDev.com.
- GeeksforGeeks. Socket Programming in Java. GeeksforGeeks.org.