When working with objects in JavaScript, you often need to determine whether a property belongs directly to an object or if it is inherited through its prototype chain. The hasOwnProperty()
method is a built-in method designed specifically for this purpose. In this article, we’ll explore how it works, its benefits, and real-world use cases.
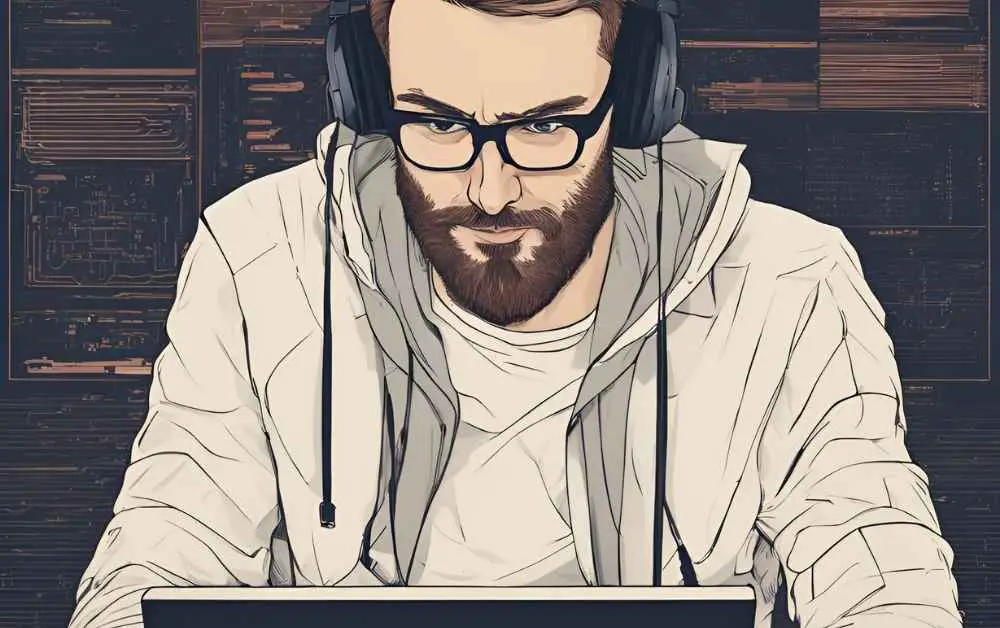
What is the hasOwnProperty()
Method?
The hasOwnProperty()
method checks whether an object has a property as its own (direct) property instead of being inherited from its prototype chain.
It is a method available on all JavaScript objects via the Object.prototype
.
Syntax
obj.hasOwnProperty(property)
obj
: The object on which you want to check the property.property
: The name of the property (as a string) to check for.
This method returns a boolean:
true
if the property exists directly on the object.false
if the property is inherited or does not exist.
Example Usage
Basic Example
const car = {
brand: "Toyota",
model: "Corolla"
};
console.log(car.hasOwnProperty("brand")); // true
console.log(car.hasOwnProperty("toString")); // false (inherited from Object.prototype)
In the example above, brand
is a direct property of the car
object, while toString
is inherited from the Object.prototype
.
Inherited vs Own Properties
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
return `Hello, ${this.name}!`;
};
const person1 = new Person("Alice");
console.log(person1.hasOwnProperty("name")); // true
console.log(person1.hasOwnProperty("greet")); // false (inherited from Person.prototype)
Why Use hasOwnProperty()
?
When iterating over an object using a for...in
loop, properties from the prototype chain are also included. Using hasOwnProperty()
allows you to filter out these inherited properties.
Example with for...in
Loop
const animal = {
type: "Mammal"
};
const dog = Object.create(animal);
dog.name = "Bulldog";
dog.bark = function() {
return "Woof!";
};
for (let key in dog) {
if (dog.hasOwnProperty(key)) {
console.log(key); // Logs: "name", "bark"
}
}
Without hasOwnProperty()
, the inherited type
the property would also be logged.
Common Use Cases
- Safeguarding Code Against Prototype Pollution
- In scenarios where objects might have malicious properties injected via prototypes, using
hasOwnProperty()
ensures that only direct properties are considered.
- In scenarios where objects might have malicious properties injected via prototypes, using
- Filtering Object Properties
- When iterating over objects with
for...in
, it’s important to exclude inherited properties to avoid unintended side effects.
- When iterating over objects with
- Debugging and Testing Object Structures
- Useful for checking the structure of an object in unit tests or debugging scenarios.
Potential Pitfalls
Shadowing of hasOwnProperty()
If an object has a property named hasOwnProperty
, it can overshadow the native method. To avoid this, you can call the method from Object.prototype
directly:
const obj = {
hasOwnProperty: function() {
return false;
},
key: "value"
};
// This would return false due to shadowing
console.log(obj.hasOwnProperty("key")); // false
// Correct approach
console.log(Object.prototype.hasOwnProperty.call(obj, "key")); // true
Non-Object Data Types
hasOwnProperty()
is not directly available on primitive values. Attempting to use it on a primitive will result in an error:
const num = 42;
// num.hasOwnProperty("toString"); // TypeError
You can avoid this by ensuring the value is an object or by using Object()
to wrap it:
console.log(Object(num).hasOwnProperty("toString")); // false
Alternatives to hasOwnProperty()
Object.keys()
To get an array of an object’s own properties:
const obj = { a: 1, b: 2 };
console.log(Object.keys(obj)); // ["a", "b"]
Object.hasOwn()
(Introduced in ES2022)
A newer method, Object.hasOwn()
, behaves similarly to hasOwnProperty()
but avoids issues with shadowing:
const obj = { a: 1 };
console.log(Object.hasOwn(obj, "a")); // true
Conclusion
The hasOwnProperty()
method is a robust way to check for direct properties of an object. It is crucial in ensuring safe and predictable code, especially when working with complex object structures or prototype inheritance. While newer alternatives like Object.hasOwn()
provide similar functionality with added safety, hasOwnProperty()
remains widely supported and valuable in modern JavaScript development.
Start incorporating hasOwnProperty()
in your projects today to ensure your code is both reliable and maintainable!
Follow US – Dev Community