If you’ve ever sought to create unique identifiers in a system that don’t rely on sequence-based or database-stored values, then UUID (Universally Unique Identifier) holds the key. In the realm of JavaScript, UUID fills a crucial role in safeguarding data uniqueness across varying systems. Understanding the complexities of UUID, from its unique properties to its critical roles, lays the foundation for exploring the reach of data comprehensiveness and synchronization in JavaScript.
Understand the Concept of UUID
Understanding UUID in JavaScript
UUID stands for Universally Unique Identifier, and in JavaScript, it is typically used to provide a unique identity for data or objects. Each UUID is a 128-bit number, represented as a 36-character string such as “123e4567-e89b-12d3-a456-426655440000”.
UUIDs are designed to be sufficiently random, which ensures each UUID is distinct from every other UUID generated, providing unique identifiers across time and space.
Importance of UUID
In software applications, managing unique identities is essential, and UUID helps in fulfilling that need. It ensures every element in the system has a unique identifier, guaranteeing data uniqueness.
Typically, UUIDs are necessary when data is distributed across multiple systems or databases. In JavaScript (and many other programming languages), they are often used in scenarios such as setting unique keys in databases, generating random file names for uploaded files, creating authentication tokens, or any situation where a unique string is required.
Why do UUIDs maintain data uniqueness across systems?
UUIDs are unique to each system and maintain the data’s uniqueness throughout multiple systems due to their design and the randomization process. This functionality makes them very scalable and a preferred choice for distributed systems, as systems can generate UUIDs independently of each other while still maintaining the uniqueness guarantee.
Despite being randomly generated, the probability of one UUID colliding with another is incredibly low to the point of being virtually impossible, ensuring each UUID generated remains distinct.
The application of UUIDs in JavaScript, or any programming language, plays an important role in maintaining data integrity and order, leading to more efficient and robust software systems.
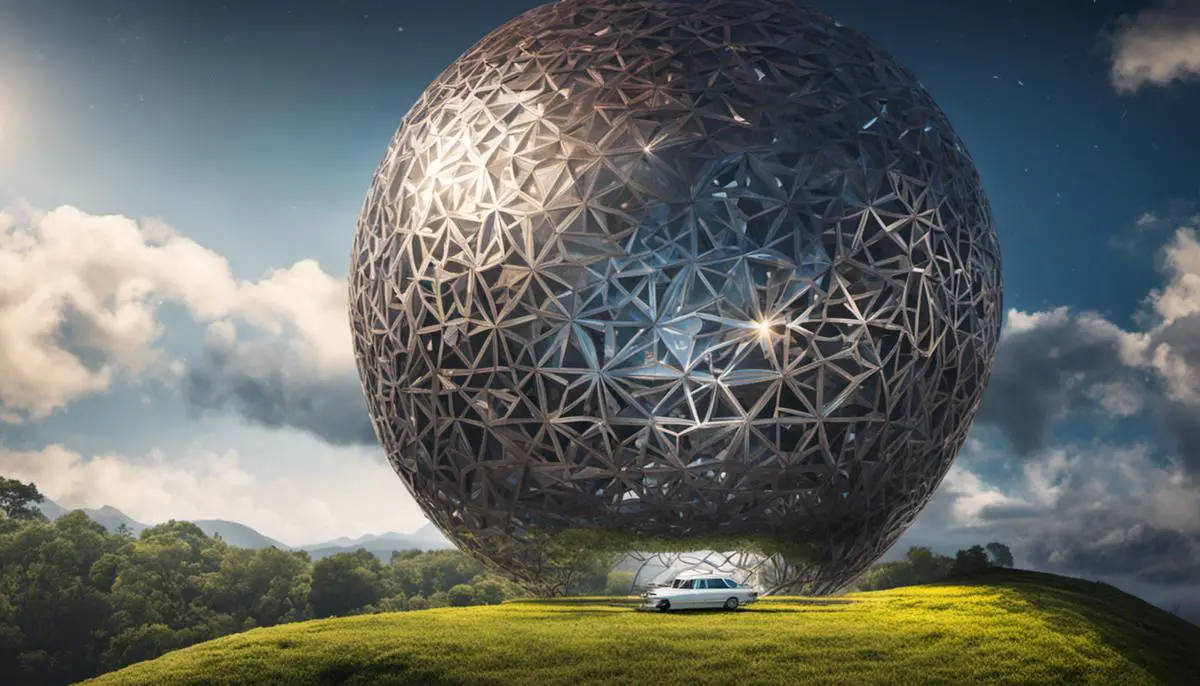
Implement UUID Generation in JavaScript
Understanding UUID in JavaScript
UUID stands for Universal Unique Identifier. As its name suggests, it is used to uniquely identify information in a distributed environment. In JavaScript, a UUID is a string of characters which follows a certain structure and is intended to have a statistically negligible chance of collision, thereby making it unique.
Generating UUID with ‘uuid’ Library
One of the simplest ways to generate UUID in JavaScript is by using the ‘uuid’ library. You can install ‘uuid’ library via npm by running the following command in your terminal:
npm install uuid
After you’ve installed ‘uuid’, generating a UUID in JavaScript is easy. Here is an example of how to do it:
const uuid = require('uuid');
let uniqueId = uuid.v4(); // Generate a v4 UUID
console.log(uniqueId);
In the above example, uuid.v4()
command is used. The ‘v4’ here refers to version 4 of UUID which is based on random numbers. When you run this program, ‘uuid’ will generate a unique string every time.
Generating UUID without Any Library
If you want to generate a UUID in JavaScript without using any libraries, you can do it by leveraging the in-built Math.random() function. Here’s how you can do it:
function generateUUID() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = Math.random() * 16 | 0, v = c === 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
console.log(generateUUID());
In the code snippet above, we’re first defining a string with the structure that a UUID follows. We then replace each ‘x’ or ‘y’ in the format string with a hexadecimal digit. Here, ‘y’ is replaced by a random hexadecimal digit from set [8, 9, A, or B]. Math.random() function is used to generate random numbers.
You can also use the `crypto` module in Node.js to generate a cryptographically secure UUID.
Here’s an example:
const { randomUUID } = require('crypto');
// Generate a UUID
const myUUID = randomUUID();
console.log(myUUID);
This is a recommended way
This `randomUUID()` function from the `crypto` module in Node.js generates a Version 4 UUID, which is based on random numbers. It’s more secure than using a simple random number generator for UUID generation.
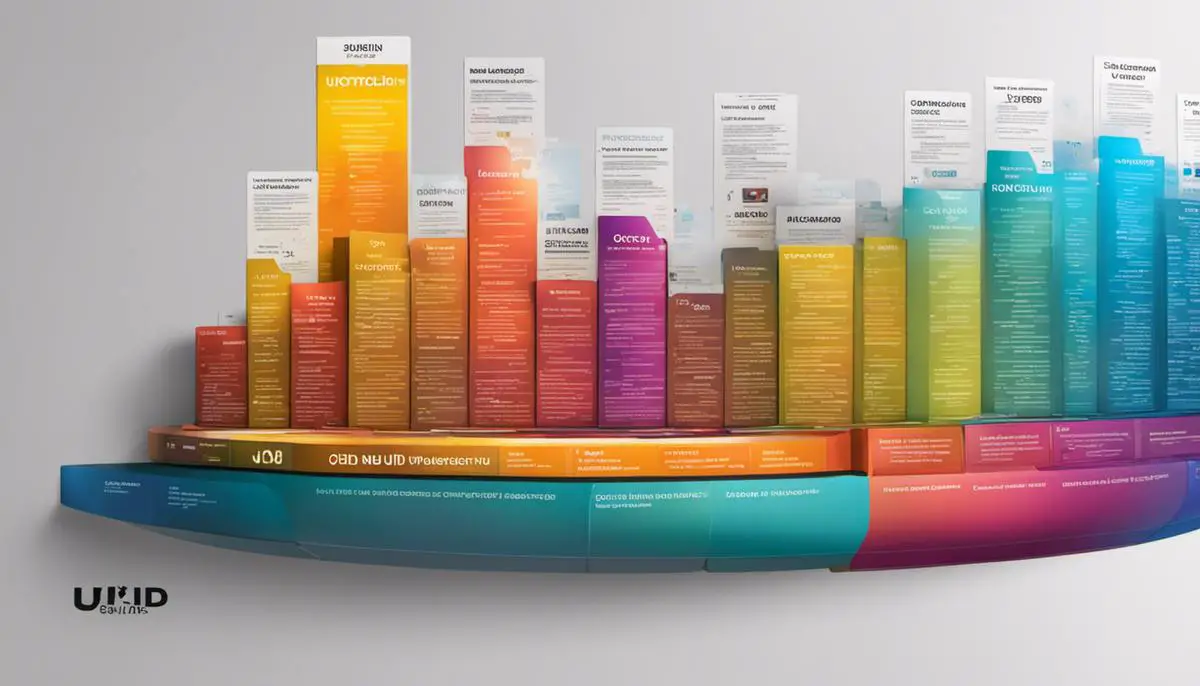
The journey into generating UUIDs in JavaScript opens doors to numerous possibilities for maintaining data singularity. As you’ve journeyed through using ‘uuid’ modules or even without relying on libraries, you’ve collected substantial knowledge and developed practical skills. From this, you can ensure your JavaScript projects maintain data uniqueness across various systems, bringing you one step closer to mastering JavaScript processes and systems integrations.