Understanding how to effectively manipulate data structures is crucial for developers. Fold, often referred to as reduce in JavaScript, offers a streamlined approach to data processing. By applying a function across an array, it simplifies operations and enhances code readability.
Understanding Fold as a Higher-Order Function
Fold, a fundamental higher-order function in programming, is called reduce in JavaScript. It works by applying an accumulator function to all items in an array, producing a single cumulative result. The versatility of fold makes it useful for various data processing needs.
Consider these examples:
// Summing numbers
[1, 2, 3, 4, 5].reduce((memo, num) => memo + num, 0) // Result: 15
// Multiplying numbers
[1, 2, 3, 4, 5].reduce((memo, num) => memo * num, 1) // Result: 120
// Joining letters
['h', 'e', 'l', 'l', 'o'].reduce((memo, letter) => memo + letter) // Result: "hello"
// Counting occurrences
['apple', 'banana', 'apple', 'orange', 'banana', 'apple'].reduce((acc, fruit) => {
acc[fruit] = (acc[fruit] || 0) + 1;
return acc;
}, {}) // Result: { apple: 3, banana: 2, orange: 1 }
Fold is straightforward because it mirrors thinking patterns most developers already use. You start with a base value, apply logic on each item, and smoothly glide to an end result.
Implementing Fold with Array.prototype.reduce
JavaScript’s Array.prototype.reduce serves as a powerful alternative to traditional loops, catering to operations like summing, multiplying, and string concatenation. It simplifies and improves code readability, transforming loops into concise expressions.
Here are some practical implementations:
// Summing numbers
const numbers = [1, 2, 3, 4, 5];
const total = numbers.reduce((accumulator, current) => accumulator + current, 0);
// Multiplying numbers
const product = numbers.reduce((accumulator, current) => accumulator * current, 1);
// Joining letters
const letters = ['h', 'e', 'l', 'l', 'o'];
const word = letters.reduce((accumulator, current) => accumulator + current);
// Counting occurrences
const fruits = ['apple', 'banana', 'apple', 'orange', 'banana', 'apple'];
const fruitCount = fruits.reduce((acc, fruit) => {
acc[fruit] = (acc[fruit] || 0) + 1;
return acc;
}, {});
By embracing this approach, developers can streamline operations, effectively replace loops, and maintain clear, concise code.
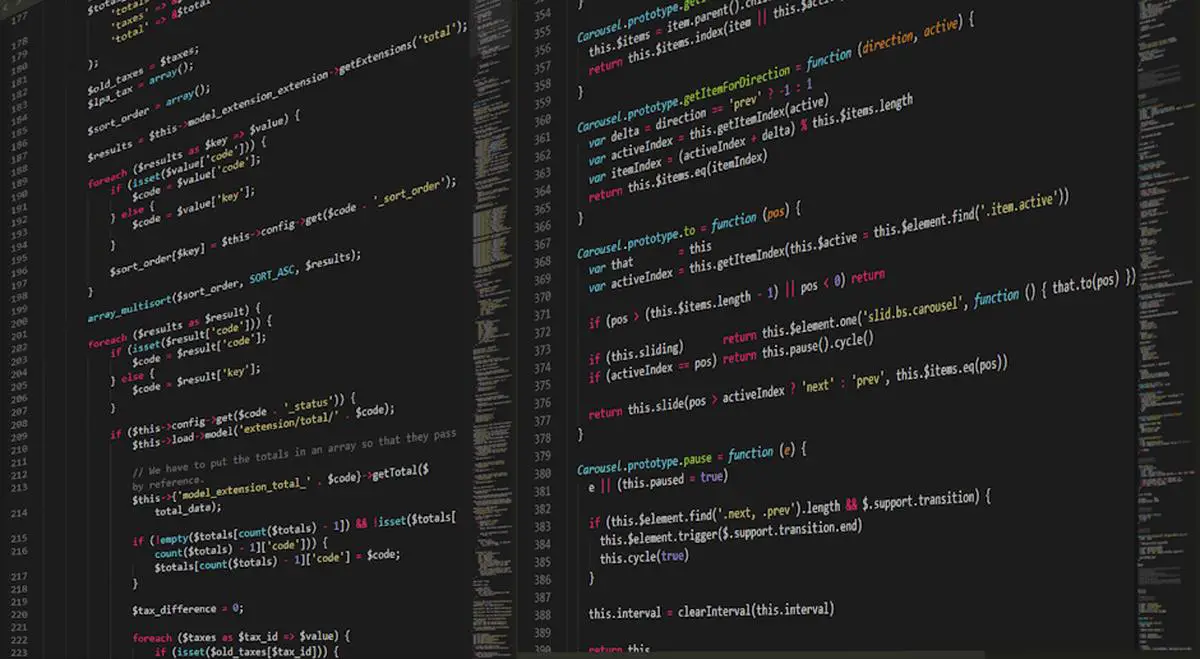
Common Use Cases and Examples
Fold, or reduce, shines in various practical scenarios:
- Vote counting:
const votes = ['Alice', 'Bob', 'Alice', 'Carol', 'Bob', 'Alice'];
const voteCount = votes.reduce((acc, vote) => {
acc[vote] = (acc[vote] || 0) + 1;
return acc;
}, {}); - Finding maximum value:
const attendances = [250, 300, 150, 400, 350];
const maxAttendance = attendances.reduce((max, current) => Math.max(max, current), -Infinity); - Creating a dictionary from user data:
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Carol' }
];const userDictionary = users.reduce((acc, user) => {
acc[user.id] = user.name;
return acc;
}, {});
These examples demonstrate reduce’s prowess in data aggregation, finding extremes, and data structure creation.
Advantages of Using Fold
Using fold offers several benefits:
- Cleaner and more expressive code: Fold replaces complex loops with concise, understandable expressions.
- Improved readability: The accumulator pattern makes the intent clear, distilling complex actions into simple instructions.
- Reusability: Fold encapsulates operation logic into a reusable format, adaptable for various tasks.
- Encourages functional programming: It aligns with modern best practices and promotes thinking composably.
- Efficiency: By eliminating loop variables and counters, fold allows developers to focus on core logic.
These advantages make fold a valuable tool for transforming how developers approach problem-solving in JavaScript, leading to smarter and more sophisticated code.
Potential Pitfalls and Considerations
While fold is powerful, it’s important to be aware of potential issues:
- Initial value: Choosing the wrong starting point can skew results. Ensure the initial value aligns with the desired output.
- Reducer function: Misjudging the reducer’s logic can lead to unwanted data transformations or errors. Shape reducers thoughtfully to guide the accumulator precisely.
- Applicability: Fold isn’t suitable for every problem. Consider alternative approaches for scenarios requiring multiple data passes or operations with dependencies between iterations.
- Performance: For large or complex datasets, fold might not be the most efficient option, especially if the reducer function is computationally intensive.
- Complexity: While fold can simplify code, it may sometimes make logic harder to follow for those unfamiliar with functional programming concepts.
It’s crucial to choose the right tool for each task, using fold where it shines and considering other strategies when appropriate.
Fold transforms complex data manipulation into straightforward tasks, making it an essential tool for developers. Its ability to simplify code while maintaining clarity and efficiency underscores its value in programming.
JavaScript in Operator: Test Object Properties
Master Event Emitter in JavaScript
- Hutton G. A tutorial on the universality and expressiveness of fold. J Funct Program. 1999;9(4):355-372.
- Bird R. A simple proof of the universality of fold. J Funct Program. 1998;8(5):551-554.
- Meijer E, Fokkinga M, Paterson R. Functional programming with bananas, lenses, envelopes and barbed wire. In: Hughes J, ed. Functional Programming Languages and Computer Architecture. Springer; 1991:124-144.