Flowbite React, a versatile React-based library, bridges the gap between rapid development and modern design, offering developers pre-designed components and utilities that align perfectly with Tailwind CSS. This article dives deep into Flowbite React, covering its features, installation, use cases, and why it’s a valuable tool for modern developers.
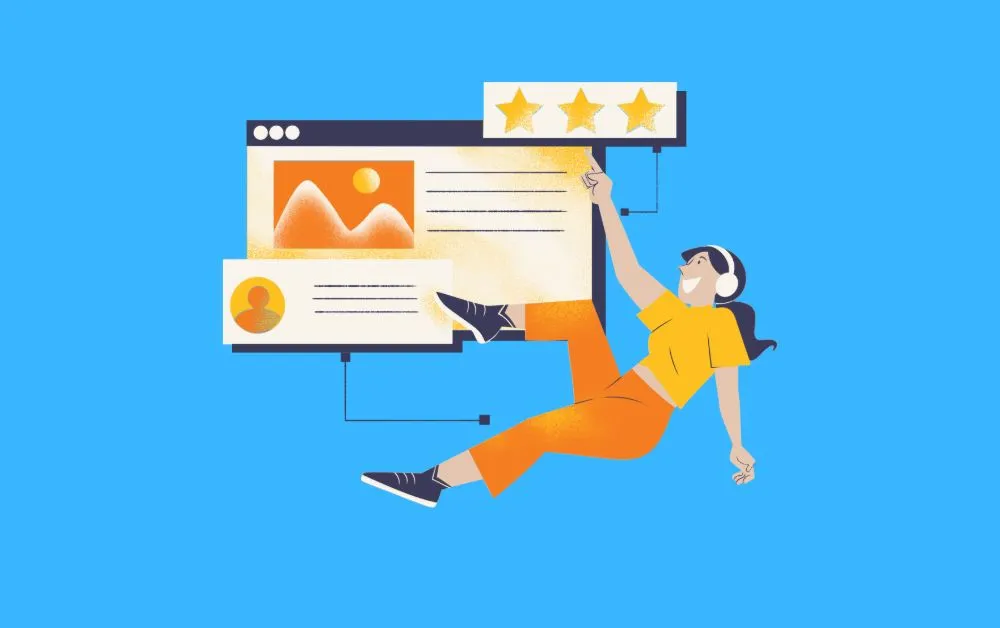
What is Flowbite React?
Flowbite React is the React integration of Flowbite, a popular component library built on Tailwind CSS. It simplifies the creation of responsive, accessible, and aesthetically pleasing interfaces by providing a collection of reusable components like buttons, modals, carousels, and more. Designed to integrate seamlessly with React, Flowbite React allows developers to focus on functionality without compromising on design.
Why Choose Flowbite React?
Here are some reasons why Flowbite React stands out:
- Tailwind CSS Compatibility
Flowbite React uses Tailwind CSS classes, making it ideal for projects already using Tailwind. You can easily customize components by extending your Tailwind configuration. - Accessibility
All components adhere to WAI-ARIA standards, ensuring that your application is accessible to a wide audience. - Customization
Flowbite React components are easily customizable, allowing you to maintain consistency with your brand’s design language. - Ease of Use
With intuitive syntax and a rich documentation set, Flowbite React is beginner-friendly yet robust enough for experienced developers.
Installation and Setup
Getting started with Flowbite React is straightforward. Here’s a step-by-step guide:
Step 1: Install Tailwind CSS
First, ensure you have Tailwind CSS installed in your React project. You can follow Tailwind’s official installation guide for this.
Step 2: Install Flowbite and Flowbite React
Run the following commands to install Flowbite and its React package:
npm install flowbite-react
Step 3: Configure Tailwind CSS
Add Flowbite to your Tailwind configuration file (tailwind.config.js
):
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
"./node_modules/flowbite-react/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [require("flowbite/plugin")],
};
Step 4: Import Components
Import the required Flowbite React components in your project:
import { Button } from 'flowbite-react';
Key Components of Flowbite React
1. Button
Flowbite React provides a wide variety of buttons that can be customized for different use cases.
import { Button } from 'flowbite-react';
function App() {
return (
<Button gradientDuoTone="purpleToBlue">Click Me</Button>
);
}
2. Navbar
Create responsive navigation bars effortlessly:
import { Navbar } from 'flowbite-react';
function App() {
return (
<Navbar fluid rounded>
<Navbar.Brand href="/">
<img
src="/logo.png"
className="mr-3 h-6 sm:h-9"
alt="Flowbite Logo"
/>
<span className="self-center whitespace-nowrap text-xl font-semibold dark:text-white">
Flowbite
</span>
</Navbar.Brand>
</Navbar>
);
}
3. Modal
Add interactive modals with ease:
import { Modal, Button } from 'flowbite-react';
function App() {
const [open, setOpen] = React.useState(false);
return (
<>
<Button onClick={() => setOpen(true)}>Open Modal</Button>
<Modal show={open} onClose={() => setOpen(false)}>
<Modal.Header>Modal Title</Modal.Header>
<Modal.Body>This is the modal content.</Modal.Body>
</Modal>
</>
);
}
4. Carousel
Implement dynamic carousels:
import { Carousel } from 'flowbite-react';
function App() {
return (
<Carousel>
<img src="/image1.jpg" alt="Slide 1" />
<img src="/image2.jpg" alt="Slide 2" />
<img src="/image3.jpg" alt="Slide 3" />
</Carousel>
);
}
Advanced Customization
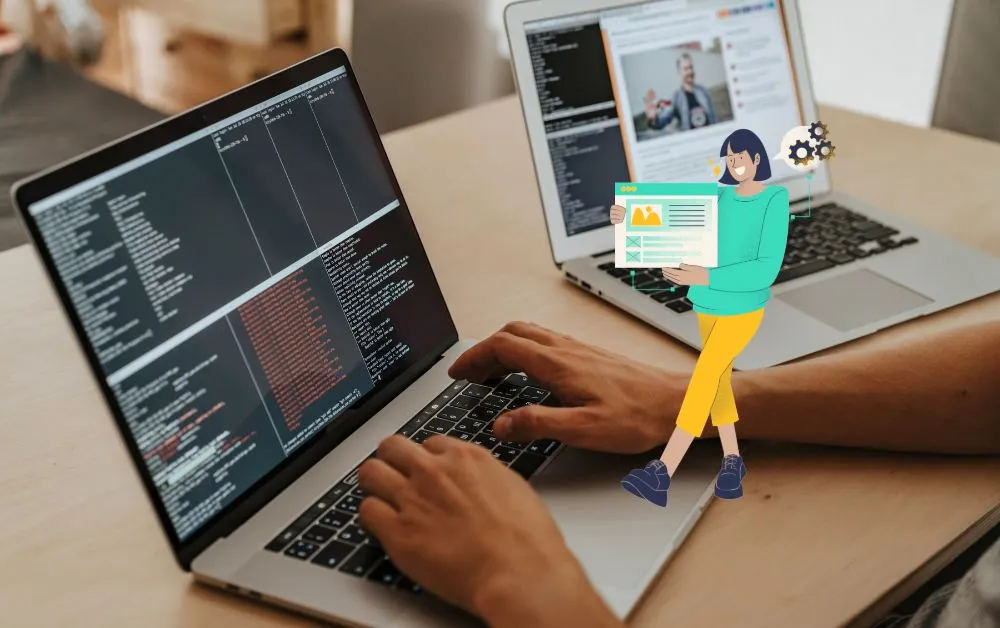
Theming
Flowbite React supports custom themes, enabling you to define unique styles. Extend the Tailwind configuration to include custom color schemes or typography.
Utility Integration
Leverage Tailwind’s utility-first approach to fine-tune component styling without leaving your markup.
Use Cases of Flowbite React
- Dashboards
Build complex dashboards with components like data tables, modals, and sidebars. - E-commerce Sites
Create attractive product displays and carousels effortlessly. - Marketing Websites
Use Flowbite’s call-to-action buttons and hero sections for landing pages. - Admin Panels
Simplify the creation of admin interfaces with pre-designed layouts and components.
Final Thoughts
Flowbite React is a powerful tool for developers seeking to streamline the UI development process while maintaining design consistency and accessibility. Its seamless integration with Tailwind CSS, extensive component library, and customization options make it an excellent choice for modern web applications. Whether you’re building a simple landing page or a complex dashboard, Flowbite React has you covered.
For more information and in-depth documentation, visit the Flowbite React official website.
Author’s Note: If you’re looking for more articles about React or Tailwind CSS, check out Makemychance.com for additional resources!