Identify Conflict Source
To resolve the “npm error ERESOLVE” with jsdom and canvas on Vercel, start by examining your project’s dependencies. Check your package.json
file for conflicting versions of these packages. Use npm ls jsdom canvas
to list where they’re used and identify mismatches.
Review issue trackers on GitHub for jsdom or node-canvas repositories. There may be discussions about known conflicts or bugs with certain versions.
To fix the issue, try aligning the versions. Set compatible versions of jsdom and canvas in your package.json
. Run npm install jsdom@x.x.x canvas@x.x.x --legacy-peer-deps
to avoid peer dependency conflicts.
For Vercel deployments, ensure local and production environments match. Verify your Node.js version in package.json
with a setting like "engines": { "node": "18.x" }
for consistency.
If conflicts persist, consider adding a post-install script:
"scripts": {
"postinstall": "npm install --legacy-peer-deps"
}
This forces npm to install dependencies while ignoring peer dependency conflicts.
Remember: Vercel might handle dependencies differently than your local setup, especially for serverless functions. With node-canvas, certain libraries might be unavailable in environments like AWS Lambda.
Install Legacy Peer Dependencies
Using the --legacy-peer-deps
flag can help resolve dependency conflicts. This makes npm handle package installations more flexibly, bypassing strict version requirements.
To implement this, add the following to your package.json
scripts section:
"scripts": {
"postinstall": "npm install --legacy-peer-deps"
}
This instructs npm to apply the legacy peer dependencies policy after the initial installation, helping to prevent potential conflicts.
While this approach improves compatibility, use it cautiously. Ensure your application still functions correctly after installation, as legacy installations differ from standard dependency handling.
Adjust Node.js Version
Maintaining a consistent Node.js version between your local setup and Vercel deployment environment is crucial. This prevents ERESOLVE errors from version discrepancies and ensures predictable code behavior.
Edit your package.json
and add an "engines"
field to set the Node.js version:
"engines": {
"node": "18.x"
}
This instructs the deployment environment to use this version, maintaining consistency with your local setup.
- After setting this, redeploy your application to refresh everything under the new settings.
- This approach doesn’t prevent using newer Node.js versions—it ensures synchronization with your local tests.
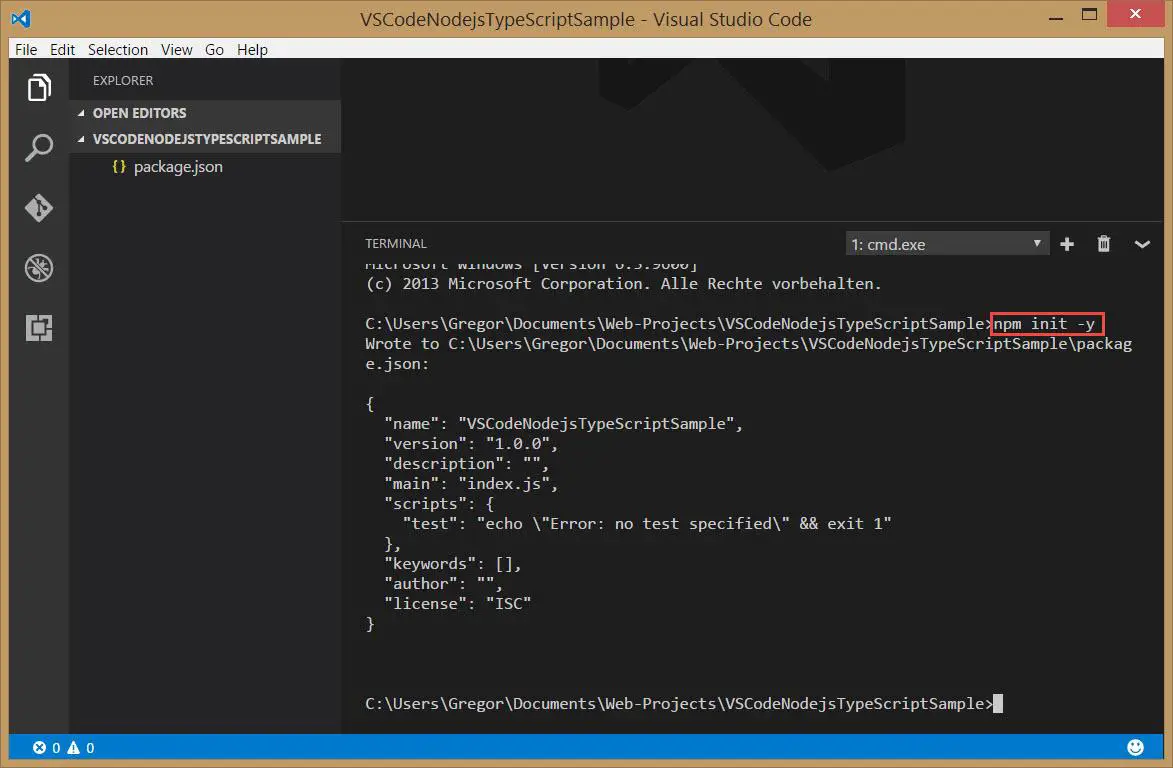
Remove Redux DevTools
If the redux-devtools-extension
is causing issues, consider removing it. Run:
npm uninstall redux-devtools-extension
Then, adjust your Redux store setup. Replace any composeWithDevTools
usage with a simple middleware setup:
import { createStore, applyMiddleware } from 'redux';
import rootReducer from './reducers';
import thunk from 'redux-thunk';
const store = createStore(
rootReducer,
applyMiddleware(thunk)
);
This simplification reduces potential bugs from outdated or incompatible devtools and ensures your Redux setup remains stable.
By following these steps, resolving dependency conflicts becomes more manageable. Aligning your environments and dependencies is key to maintaining smooth project operations.
Experience the future of content creation with Writio, the AI writer for blogs and websites. This article was crafted by Writio.
- GitHub. Issues – kkomelin/isomorphic-dompurify. 2023.
- Vercel. Next.js Discussions. 2023.
How I Fixed the “Module Not Found” Error When Deploying a Vite Project on Vercel
🛠️ Solving “Deprecation Warning [legacy-js-api]” in Dart Sass While Building a React Project
Arsalan Malik is a passionate Software Engineer and the Founder of Makemychance.com. A proud CDAC-qualified developer, Arsalan specializes in full-stack web development, with expertise in technologies like Node.js, PHP, WordPress, React, and modern CSS frameworks.
He actively shares his knowledge and insights with the developer community on platforms like Dev.to and engages with professionals worldwide through LinkedIn.
Arsalan believes in building real-world projects that not only solve problems but also educate and empower users. His mission is to make technology simple, accessible, and impactful for everyone.